Define Algorithm. Define Token with an Example. Write any two rules for Identifiers. Define Binary Search. What is sorting? List any two sorting techniques. What is an array? Give... Define Algorithm. Define Token with an Example. Write any two rules for Identifiers. Define Binary Search. What is sorting? List any two sorting techniques. What is an array? Give the syntax. Write an algorithm to exchange the values of two variables. Write a note on break and continue with an example. Illustrate the declaration and initialization of pointers with an example. Write a C program to remove the duplicate entries in a single dimensional array? How do find the smallest divisor of an integer? Write an algorithm to perform hash search on the given set of elements.
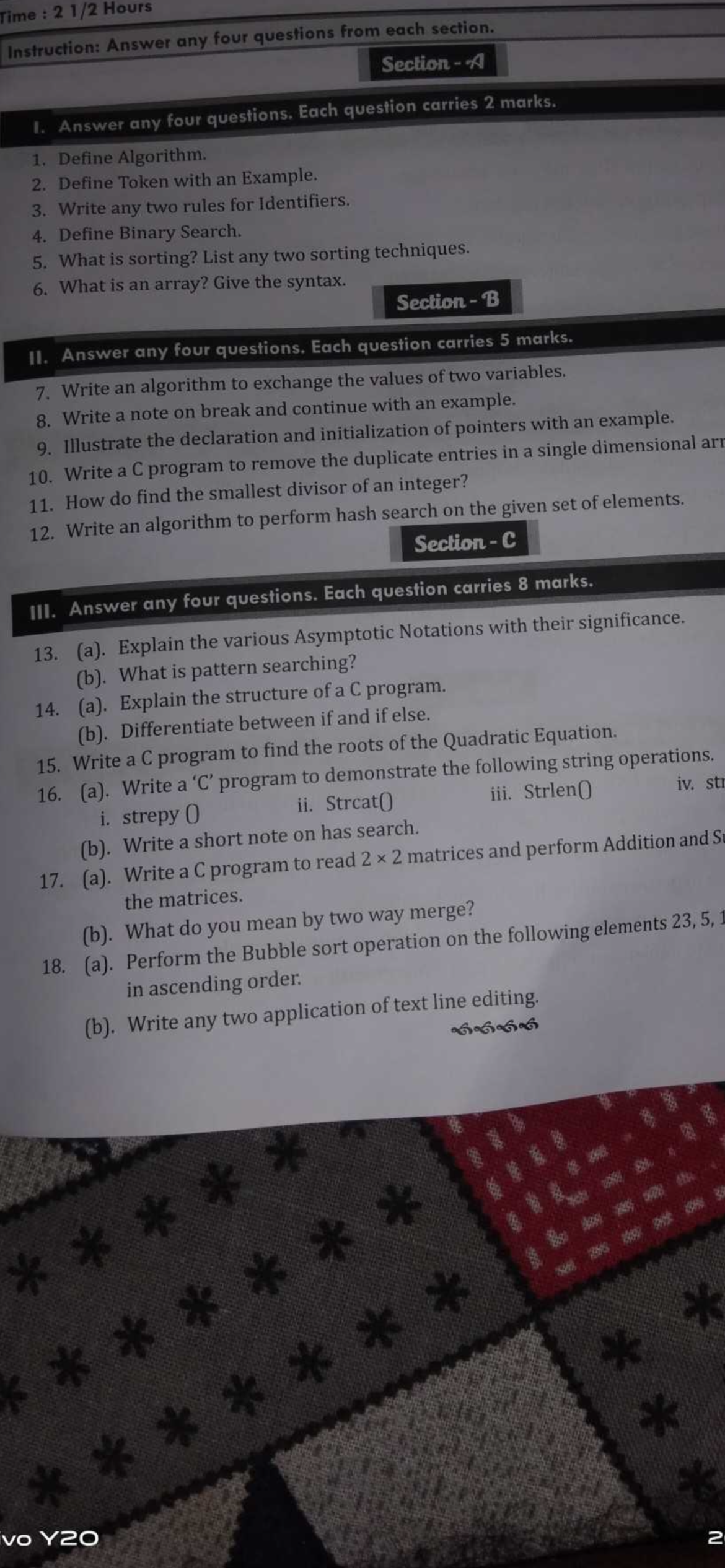
Understand the Problem
The question contains a series of exam prompts related to algorithms, data structures, and programming in C. It seeks answers to various specific questions in those domains, which involve definitions, explanations, and writing code examples.
Answer
Answer key for various programming concepts and sample solutions provided.
-
Algorithm: A set of step-by-step instructions to perform a task or solve a problem, typically by a computer.
-
Token in C: The smallest element of a program recognized by the compiler, e.g., keywords, identifiers, operators, etc.
-
Rules for Identifiers:
- Must begin with a letter or underscore.
- Cannot use reserved words.
-
Binary Search: An efficient algorithm to find an item's position in a sorted list by repeatedly dividing the search interval in half.
-
Sorting: Rearranging elements in a particular order. Techniques: Bubble Sort, Quick Sort.
-
Array: A collection of elements of the same type stored in contiguous memory locations. Syntax:
type name[size];
-
Algorithm to Exchange Values:
- Input variables a, b.
- temp = a.
- a = b.
- b = temp.
- Output a, b.
-
Note on Break and Continue:
-
Break: Exits the loop immediately. Example:
for(int i=0; i<10; i++) { if(i == 5) break; printf("%d ", i); }
-
Continue: Skips the current iteration and proceeds to the next. Example:
for(int i=0; i<10; i++) { if(i == 5) continue; printf("%d ", i); }
-
Break: Exits the loop immediately. Example:
-
Pointers Declaration and Initialization Example:
int a = 10; int *p = &a;
-
C Program to Remove Duplicate Entries in Array:
#include <stdio.h>
void removeDuplicates(int arr[], int n) {
int temp[n];
int j = 0;
for (int i = 0; i < n - 1; i++) {
if (arr[i] != arr[i + 1]) {
temp[j++] = arr[i];
}
}
temp[j++] = arr[n - 1];
for (int i = 0; i < j; i++) {
arr[i] = temp[i];
}
}
int main() {
int arr[] = {1, 2, 2, 3, 4, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
removeDuplicates(arr, n);
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
return 0;
}
- Smallest Divisor of an Integer:
- Check divisibility starting from the smallest integer greater than 1 up to the square root of the number.
- Hash Search Algorithm:
- Create a hash table.
- Compute hash index for each element.
- Insert keys at computed hash index.
- Search by calculating hash index of target value.
Answer for screen readers
-
Algorithm: A set of step-by-step instructions to perform a task or solve a problem, typically by a computer.
-
Token in C: The smallest element of a program recognized by the compiler, e.g., keywords, identifiers, operators, etc.
-
Rules for Identifiers:
- Must begin with a letter or underscore.
- Cannot use reserved words.
-
Binary Search: An efficient algorithm to find an item's position in a sorted list by repeatedly dividing the search interval in half.
-
Sorting: Rearranging elements in a particular order. Techniques: Bubble Sort, Quick Sort.
-
Array: A collection of elements of the same type stored in contiguous memory locations. Syntax:
type name[size];
-
Algorithm to Exchange Values:
- Input variables a, b.
- temp = a.
- a = b.
- b = temp.
- Output a, b.
-
Note on Break and Continue:
-
Break: Exits the loop immediately. Example:
for(int i=0; i<10; i++) { if(i == 5) break; printf("%d ", i); }
-
Continue: Skips the current iteration and proceeds to the next. Example:
for(int i=0; i<10; i++) { if(i == 5) continue; printf("%d ", i); }
-
Break: Exits the loop immediately. Example:
-
Pointers Declaration and Initialization Example:
int a = 10; int *p = &a;
-
C Program to Remove Duplicate Entries in Array:
#include <stdio.h>
void removeDuplicates(int arr[], int n) {
int temp[n];
int j = 0;
for (int i = 0; i < n - 1; i++) {
if (arr[i] != arr[i + 1]) {
temp[j++] = arr[i];
}
}
temp[j++] = arr[n - 1];
for (int i = 0; i < j; i++) {
arr[i] = temp[i];
}
}
int main() {
int arr[] = {1, 2, 2, 3, 4, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
removeDuplicates(arr, n);
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
return 0;
}
- Smallest Divisor of an Integer:
- Check divisibility starting from the smallest integer greater than 1 up to the square root of the number.
- Hash Search Algorithm:
- Create a hash table.
- Compute hash index for each element.
- Insert keys at computed hash index.
- Search by calculating hash index of target value.
More Information
This provides a comprehensive overview of basic programming concepts, including data structures like arrays and algorithms like binary search and sorting.
Tips
Common mistakes in algorithm design include not accounting for edge cases and inefficient time complexity.
Sources
- Binary Search Algorithm - GeeksforGeeks - geeksforgeeks.org
- Sorting Algorithms Explained with Examples - Freecodecamp - freecodecamp.org
- Tokens in C - Javatpoint - javatpoint.com
AI-generated content may contain errors. Please verify critical information