Week 2 - C Programming Fundamentals PDF
Document Details
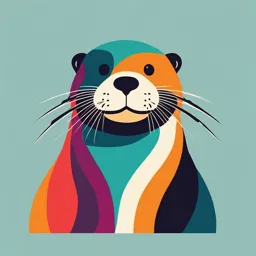
Uploaded by LuxuryAbundance
Algonquin College
Tags
Summary
This document is a set of lecture notes on C programming for Algonquin College. It covers fundamental concepts like data types, control flow, and functions.
Full Transcript
CST8234 – C Programming Week 2 - The basics of language. Objectives Write Simple C Programs Use I/O Statements Learn about Fundamental Data Types Write simple Decision-Making Statements Learn about Control Specifiers About Macros Intro to Arrays 2 ...
CST8234 – C Programming Week 2 - The basics of language. Objectives Write Simple C Programs Use I/O Statements Learn about Fundamental Data Types Write simple Decision-Making Statements Learn about Control Specifiers About Macros Intro to Arrays 2 Basics of the C language The main function. Using other files and libraries (#include). C program structure. Variables. Control Flow. Conditionals. Iteration (loops). Functions. 3 Typical C program structure A typical C program includes: 1. Preprocessor directives: Lines beginning with # used for including libraries or defining macros. 2. Function definitions: Including the main function and any other functions. 3. Statements and expressions: The actual code that performs operations. 4 Example of a Typical C program #include int add(int a, int b) { return a + b; } int main() { int result = add(5, 3); printf("The result is %d\n", result); return 0; } 5 Example of a Typical C program #include // function main begins program execution int main() { printf("Welcome to C!\n"); } // end function main OUTPUT: Welcome to C! 6 Typical C project structure The project is divided into several implementation files “.c” and header files that expose their functionality called “.h” files. A program can consist of any number of these files, no limitations. Though, when dividing the program into smaller files, it is best practice to group the functionality logically. For example, if we are to build a calculator program, we might have the following files main.c: The entry point of the program. operations.h: File that include the definitions for the various operations the user can do. operations.c: The actual implementations for the operations User-input.h: File that has common functionality to handle user input. User-input.c: The actual implementations for the common functionality. We will look at the header files later in the class. 7 C #include Preprocessor Directive o Used to include the contents of a file within another file before the actual compilation of the code begins. o This is particularly useful for including libraries and headers that contain declarations of functions, macros, and type definitions which are essential for the program. o In C, in order to use someone else’s code, we need to include the library that has the implementation. o Syntax: #include Or #include “lb-name.h” o The difference between and “”: : is used to tell the complier to find the library in pre-defined location. “”: is used to tell the complier to find the library in source program location. 8 (#include) The #include directive is used to include the contents of another file. It’s commonly used to include standard libraries or header files. For instance: #include : This includes the Standard Input Output library, which provides functions like printf and scanf #include “myheader.h” : This includes a user-defined header file located in the same directory as the source file. Header files typically contain function prototypes, macros, and type definitions. 9 How #include works 1) Preprocessing Stage: When the preprocessor encounters the #include directive, it replaces the directive with the contents of the specified file. This happens before the actual compilation of the program. 2) Inclusion of Content: After replacing the #include directive with the contents of the specified file, the compiler processes this content as if it were part of the original source file. This includes function prototypes, macro definitions, and type declarations. 3) Avoiding Multiple Inclusions: To prevent issues with multiple inclusions of the same header file, you can use include guards. Include guards ensure that the header file is included only once per compilation. 1 0 Avoiding Multiple Inclusions - Example #ifndef MYHEADER_H // Checks if MYHEADER_H has not been defined #define MYHEADER_H // Defines MYHEADER_H to prevent re- inclusion. // Declarations and definitions #endif // MYHEADER_H // Ends the conditional inclusion Refer to Slide 6: #include Includes the standard I/O header, allowing the use of the printf function. printf("Hello, World!\n") : Calls the printf function to print the string "Hello, World!" to the standard output (usually the console). 1 1 The main function The main function is the entry point of the program. When the program is executed, main is the first function called. main has two prototypes (variants) 1. int main(int args, char* argv[]); This version accepts command-line arguments. argc: (argument count) is an integer representing the number of command-line arguments. argv: (argument vector) is an array of strings (character pointers) representing the command-line arguments 2. int main(); In both cases, it must return an integer at the end of program execution. Returning 0 (program terminated successfully) Returning non-zero value (Error) 1 2 The main function (Example) #include int main(int argc, char *argv[]) { printf(“Welcome Aboard!\n"); return 0; // Success } Escape Sequences backslash (\) is an escape character. Compiler combines a backslash with the next character to form an escape sequence. \n means newline Comments Begin with // , Can also use multi-line comments Comments do not cause the computer to perform actions 1 3 Escape Sequence Description \n Moves the cursor to the beginning of the next line. \t Moves the cursor to the next horizontal tab stop. Produces a sound or visible alert without changing the current \a cursor position. Because the backslash has special meaning in a string, \\ is \\ required to insert a backslash character in a string. Because strings are enclosed in double quotes, \” is required \” to insert a double-quote character in a string. © Pearson Education 1 4 Example #include // function main begins program execution int main(void) { printf("Welcome\nto\nFallTerm!\n"); } // end function main OUTPUT: Welcome to FallTerm! 1 5 Variables o Variables in C are used to store data. o They need to be declared before they can be used. o You can place each variable definition anywhere in main before that variable’s first use in the code o Here’s how you declare different types of variables: somename; int index; //integers char letter; // single chars double distance; // double-precision floating-point nos float temp; // single-precision floating-point nos NOTE: C is case sensitive, so a1 and A1 are different identifiers 1 6 Examples Variables must be declared with a data type, and optionally initialized at the same time. int age = 25; char initial = 'A’; float height = 5.9; double weight = 72.5; 1 7 Variables Definition Vs Declaration o Definition: o Variables: Specifies memory allocation and initial values o Functions: Provides the complete implementation, including the body of the function. o Declaration o Variables: Provides type and indicates that the actual definition is elsewhere. No memory is allocated here. o Functions: Provides the function signature (return type and parameters) without the body. Indicates that the implementation is elsewhere. 1 8 Scope and Lifetime of Variables o Global variables: o Definition: Variables declared outside any function or block o Scope: Visible throughout the entire file (translation unit) in which they are declared. They can be accessed by any function within the same file. o Lifetime: Exists for the entire duration of the program’s execution. o Local Variables o Definition: Variables declared inside a function or a block (e.g., loops, conditional statements). o Scope: Limited to the function or block in which they are declared. o Lifetime: Exists only during the execution of the function or block. The memory for these variables is allocated when the function/block is entered and deallocated when it is exited. 1 9 Global variables - Example #include const int institutionID = 257367; // Global variable void printInstitutionID() { printf("Institution ID: %d\n", institutionID); } int main() { printInstitutionID(); // Can access global variable return 0; } institutionID is a global variable and can be accessed by both printInstitutionID and main 2 0 Local variables - Example #include void resizeClass(int classID, int numStudents) { long timeRegistration; // Local variable to the function while (numStudents-- > 0) { int studentID = 0; // Local variable to the loop printf("school %d, class %d: student %d registered\n", classID, classID, studentID); } } int main() { resizeClass(101, 5); // Calling the function return 0; } is a local variable to the function resizeClass. It can be timeRegistration accessed throughout the function but not outside it. studentID is a local variable to the whilee loop. It is only accessible within the loop’s block. 2 1 Data Types o In C, data types are crucial because they specify the type and size of data that can be stored and manipulated within a program. BASIC DATA TYPES 1) int (Integer): Represents whole numbers. Typically, 4 bytes (32 bits) on most systems, but this can vary depending on the compiler and system. int age = 30; 2) char (Character): Represents a single character. Typically, 1 byte (8 bits) Usually from -128 to 127 (signed) or 0 to 255 (unsigned) char initial = 'A'; 2 2 Basic Data Types 3) float (Single-Precision Floating-Point): Represents real numbers with a fractional part. Typically, 4 bytes (32 bits). float temperature = 23.5; 4) double (Double-Precision Floating-Point): Represents real numbers with more precision than float. Typically, 8 bytes (64 bits) double distance = 123456.789; 2 3 Derived Data Types 1) void: Represents the absence of type. It is used for functions that do not return a value. Often used in function declarations and definitions where no value is returned. void printMessage(void) { printf(“Welcome Aboard!\n"); } 2) Array: A collection of elements of the same type. Size depends on the number of elements and the size of each element int numbers = {1, 2, 3, 4, 5}; 2 4 Derived Data Types 3) pointer: Holds the memory address of another variable. Typically, 4 bytes on a 32-bit system and 8 bytes on a 64-bit system. int value = 10; int *ptr = &value; 4) struct (Structure): A composite data type that groups variables of different types into a single unit. Size depends on the number and type of members typedef struct { char name; int age; float height; } Person; Person john; 2 5 Derived Data Types 5) union: Similar to structures, but members share the same memory location. Only one member can be accessed at a time. Size: Size of the largest member. typedef union { int intValue; float floatValue; } Data; Data data; 2 6 Modified Data Types 1) Signed and Unsigned: Modifiers that affect the range of values. ‘Signed’ allows both negative and positive values, while ‘unsigned’ only allows positive values and zero. unsigned int positiveNumber = 10; signed int negativeNumber = -10; 2) Short and Long: Modifiers that adjust the size of the integer type. ‘short’ typically uses 2 bytes, while ‘long’ can use 4 or 8 bytes depending on the system. short smallNumber = 32767; long largeNumber = 2147483647L; 3) long long: An extended version of ‘long’ that guarantees at least 8 bytes. long long veryLargeNumber = 9223372036854775807LL; 2 7 const Keyword The const keyword in C is used to define variables whose values cannot be changed after initialization. This is useful for defining values that should remain constant, such as configuration parameters or fixed values. const datatype variableName = value; const short numStudents = 500; ‘const’ variables are allocated memory and can be used in expressions. Their values are stored in memory, and they have a specific memory address. 2 8 Macro The #define directive is used to define macros or constants at the preprocessing stage. It performs a global search-and-replace for text in the code before actual compilation begins. #define NAME value #define NUM_STUDENTS 500 NUM_STUDENTS : Just a literal, and there is no type associated with it. Does not occupy any memory; it is replaced with the literal value at preprocessing time. The replacement happens before the actual compilation, so it doesn’t have a memory location or type information 2 9 const Vs ‘#define’ 1) Type Safety const: Type-safe, as it requires a specific type declaration #define: No type checking since it’s a simple text substitution 2) Memory Allocation const: Allocates memory for the constant variable #define: No memory allocation; the macro is replaced by its value during preprocessing. 3) Scope const: Scope is limited to the block or file in which it is defined (unless declared ‘extern’ #define: Global scope throughout the file after its definition 3 0 const Vs ‘#define’ 4) Modifiability const: The value cannot be modified after initialization #define: The value is a literal text replacement, but can be modified using #undef, for example: #define VALUE 5 #undef VALUE #define VALUE 6 5) Use Case const: Preferred for defining constants with a specific type and scope within your code. #define: Useful for defining constants or macros that need to be available throughout the file or project, and for simple text substitutions. 3 1 const Vs ‘#define’ Example #include #define MAX_STUDENTS 500 // Preprocessor macro const short numStudents = 500; // Constant variable int main(void) { // numStudents = 600; // This would generate a compile-time error printf("Max students: %d\n", MAX_STUDENTS); printf("Number of students: %d\n", numStudents); return 0; } 3 2 Macro Example SIMPLE MACRO: #define TRUE 1 #define MAX 10 #define MAX 10; // Incorrect: the semicolon is part of the replacement text int array[MAX]; // Results in int array[10;]; which is invalid MACROS WITH ARGUMENTS: #define SUM(x, y) ((x) + (y)) int s = SUM(4, 5); // Expands to int s = ((4) + (5)); which evaluates to 9 NESTING OF MACROS: #define PI 3.14 #define PISQUARE (PI * PI) float k = PISQUARE; // Expands to float k = (3.14 * 3.14); which evaluates to 9.8596 3 3 Control Specifier in scanf and printf ‘scanf’ and ‘printf’ are standard functions used for formatted input and output, respectively. They use format strings to specify the types and formats of the data being read or written. SCANF FUNCTION Reads input from the standard input (usually the keyboard) and stores the values in the provided variables. The format string controls how the input is parsed and interpreted. scanf("control string", &var1, &var2,...); control string: Specifies the format of the input data &var1, &var2,... : Addresses of variables where the input values will be stored 3 4 Scanf Example Reading an int and float: int marks; float term; scanf("%d:%f", &marks, &term); Input: 1500:20.5 marks will store 1500 and term will store 20.5 Reading a String: char str; scanf("%s", str); Input: HelloWorld str will HelloWorld 3 5 Scanf Example Field Width Specifier : int a, b; scanf("%2d%3d", &a, &b); For the input: 6903901 Stores 69 in "a" and 039 (the next 3 characters, which becomes the integer 39) in "b". The rest of the input (“01”) will be kept for a future input reading. For the input: 690 3901 The first 2 characters are stored in "a" (69). Afterwards, it'll put only the 0 in "b" (since there's a space after the 0 which is used as a separator). The rest of the input is kept for a future reading. 3 6 printf Function Outputs formatted data to the standard output (usually the console). The format string specifies how the data should be formatted and displayed Basic Syntax: printf("control string", var1, var2,...); control string: Specifies the format of the output data. var1, var2,... : Values or variables to be printed 3 7 printf Example Printing a floating-point number: float flo = 3.14159; printf("%f", flo); Output: 3.14159 (default precision for %f is 6 decimal places) Formatted Output with Field Width: int a = 26, b = 5; printf("a=%3d, b=%4d", a, b); Output: a= 26, b= 5 If the number of characters is less than the field width, the output is right-justified and padded with spaces 3 8 printf Example Formatting Floating-Point Nos: float x = 8.0, y = 5.9; printf("x=%4.1f, y=%7.2f", x, y); Output: x= 8.0, y= 5.90 %4.1f : specifies a field width of 4 with 1 digit after the decimal point %7.2f : specifies a field width of 7 with 2 digits after the decimal point Handling Input Lengths: float a, b; scanf("%3f%4f", &a, &b); Input: 5.965.87 Stores 5.9 in "a" (the first 3 characters) and 65.8 in "b" (the next 4 characters). The rest of the input (“7”) will be kept for a future input reading. 3 9 printf Example float x = 15.231, y = 65.875948; printf("x=%4.1f, y=%7.2f", x, y); Output: x=15.2, y= 65.88 %4.1f rounds 15.231 to 15.2 %7.2f rounds 65.875948 to 65.88 and ensures at least 7 characters wide, including the decimal point and digits 4 0 Control Flow Control flow in C is managed using conditionals and loops CONDITIONALS Conditionals allow you to execute different blocks of code based on certain conditions. if statement: Executes a block of code if a condition is true else statement: Executes a block of code if the ‘if’ condition is false Else-if : Provides additional conditions to test Switch : Provides a way to execute code based on the value of a variable Each of the above checks if some condition(s) is true to decide what part of the program to run. 4 1 if statement Checks whether some condition(s) is true or not. If the condition is true, then it executes the code in the statement block. Syntax: if () { if (index == 10) { printf(“true”); } index = index + 1; } If you have only one line to execute for the if-statement, you can remove the curly brackets if (index == 10) printf(“true”); 4 2 else statement If the condition in ‘if’ is FALSE, then it executes the code in the statement block. Syntax: if (x > 0) { printf("x is positive\n"); } else { printf("x is not positive\n"); } 4 3 if-(else-if)-else statement In some cases you may need to check for mutually exclusive conditions Syntax: if () { } else if() { } else { } 44 else if statement if (x > 0) { printf("x is positive\n"); } else if (x == 0) { printf("x is zero\n"); } else { printf("x is negative\n"); } 4 5 Switch case In other cases, you may find using a switch-case statement more readable for mutually exclusive conditions: Syntax: switch () { case const-expr: statements; break; case const-expr: statements; break; default: statements; break; } Please note break is needed at the end of every case, not to let the code fall through. 46 switch statement switch (day) { case 1: printf("Monday\n"); break; case 2: printf("Tuesday\n"); break; default: printf("Other day\n"); break; } 4 7 Iteration (Loops) Loops are used to repeat a block of code multiple times for Loop: Used when the number of iterations is known int i for (i = 0; i < 5; i++) { printf("%d\n", i); } while Loop: Repeats a block of code while a condition is true do-while Loop : Like ‘while’, but guarantees that the code block is executed at least once 4 8 for loop Syntax for (expr1; expr2; expr3) { } The most used case of for loop is when the number of repetitions is known. int index; for (index = 0; index < 10; index++) { } Please note: ANSI C is strict about where to define “index”. Therefore, it is best practice to define index before the for loop. 49 while and do-while Loop int i = 0; while (i < 5) { printf("%d\n", i); i++; } int i = 0; do { printf("%d\n", i); i++; } while (i < 5); 5 0 Logical operators Usually used with conditional statements, but not necessarily the only case. They are used to combine a number of conditions together, and evaluate the result Called Logical AND. The result is only true if and only if both && conditions are true Called Logical OR. The result is only false if and only if both || conditions are false Called Logical NOT. The result is always the opposite of the ! condition truthiness 5 1 Relational operators Used to check the relation between two different operands. The result of these operators is logical value (true or false) “==” “” Equal Less than Greater than “!=” “=“ Not equal Less than or equal Greater than or equal 5 2 Example: conditional statement with operators if ( speed >= 50 && speed