Fundamentals of C Programming PDF
Document Details
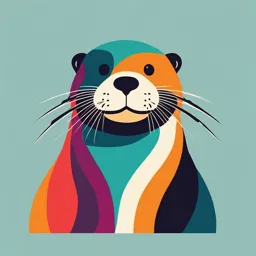
Uploaded by PeerlessRhodolite5211
Tags
Summary
This document provides a historical overview of C language, from its origins to its standardization. It explores the reasons behind the standardization and explains why C is a prevalent and powerful programming language for various applications, including systems programming. It's suitable for readers learning about C programming.
Full Transcript
History of C Language The root of all modern languages is ALGOL (Algorithmic Language). ALGOL was the first computer programming language to use a block structure, and it was introduced in 1960. In 1967, Martin Richards developed a language called BCPL (Basic Combined Programming Lan...
History of C Language The root of all modern languages is ALGOL (Algorithmic Language). ALGOL was the first computer programming language to use a block structure, and it was introduced in 1960. In 1967, Martin Richards developed a language called BCPL (Basic Combined Programming Language). BCPL was derived from ALGOL. In 1970, Ken Thompson created a language using BCPL called B. Both BCPL and B programming languages were typeless. After that, C was developed using BCPL and B by Dennis Ritchie at the Bell lab in 1972. So, in terms of history of C language, it was used in mainly academic environments, but at long last with the release of many C compilers for commercial use and the increasing popularity of UNIX, it began to gain extensive support among professionals. History of C Language In 1969, Ken Thompson and Dennis Ritchie designed a file system for Bell Laboratories in order to provide a more convenient environment for programming. This file system evolved into an early version of the UNIX Operating System. Later, this file system was implemented on a PDP-7 computer, which was found lying idle, and in 1971, on PDP- 11. In an attempt to write a FORTRAN language compiler for the new system, Thompson instead developed the language B. History of C Language B was interpreter-based, and thus had performance drawbacks; so in 1972 Ritchie developed the language C, which was compiler- based. In 1973, the new file system was rewritten in C language giving a thrust to its acceptance among an increasing number of users. History of C Language Why Was C Language Standardized? C was standardized by the American National Standards Institute (ANSI) and International Organization for Standardization (ISO) in 1983(ANSI)/1090(ISO). The purpose behind it was to ensure the compatibility and portability of C programs across different computer systems and hardware platforms. The standardization process aimed to define a common, standardized version of the language that would be recognized and supported by all manufacturers and users. Prior to standardization, there were several different versions of C in use, each with slightly different syntax and libraries, which made it difficult for programs to be ported from one system to another. The ANSI standardization of C provided a single, widely accepted definition of the language, which allowed programs to be written and compiled on one platform and then run on other platforms without modification. It had a major impact on the language, leading to its widespread adoption and greater portability of programs. Today, the ANSI standard remains the most widely recognized definition of C, and it is supported by most C compilers and libraries. Why C Language ? C covers all the basic concepts of programming. It's good for learners to learn programming. It's a base or mother programming language to learn object-oriented programming like C++, Java,.Net, etc. Many modern programming languages, such as C++, Java, and Python, have borrowed syntax and concepts from C. It provides fine-grained control over hardware, making it highly efficient. As a result, C is commonly used to develop system-level programs, like designing Operating Systems, OS kernels, etc., and also used to develop applications like Text Editors, Compilers, Network Drivers, etc. C programs are portable; hence they can run on different platforms without significant modifications. Still a significant demand for C programmers in various industries. Introduction to C Low level programming languages are used for system programming because they have powerful features such as low-level access to memory. Similarly, high-level languages are used for application programming because they are flexible and easy to use. C language combines the power of low-level languages and the flexibility and simplicity of high-level languages. Therefore, it is a widely used computer language. C as a Second and Third Generation Language C language possesses the powerful low-level features of second generation languages such as pointers, memory allocation, and bit-manipulation. It also supports conditional constructs, loop constructs, a rich list of operators, and a variety of data structures as in third generation languages. The combined features of second and third generation languages make C language a very powerful and flexible language. These features of C language make it possible to use the language for systems programming, like development of compilers, interpreters, operating systems, graphics and general utilities, and also for a host of applications in the commercial environment. C language is so powerful and flexible that C compilers are being written in earlier versions of C language. C is also used to write other applications, such as other language compilers, databases, spreadsheets, and word processors. Out of a total of 13,000 lines of code for the Unix Operating System, all except 800 lines have been written in C language. Features of the C Language C language has various features that make it a widely-used language. Some of the important features are: Pointers Memory Allocation Recursion Bit-manipulation Features of the C Language Pointers C language, like other languages, allows reference to a memory location by a name assigned to it. In addition, C allows a user to refer to a memory location (by its internal address or byte number) by using pointers. Memory Allocation In almost all programming languages, memory is assigned to a variable name at the time of definition. C language also allows dynamic allocation of memory, i.e. a program itself can request the operating system to release memory for the use of the program at the time of execution. Features of the C Language Recursion A function may call itself. This feature, called recursion, is supported by C language. Bit-Manipulation Unlike other languages, C allows manipulation of data in its lowest form of storage - BITS. For example, consider multiplication of a number, m by 2. A familiar method to do so is to compute (m * 2). Another method is to shift the BITS of memory location m to the left by one position, as shown in the following table. Features of the C Language Value in m BIT representation BIT representation Value after BIT shift of value in memory of value in memory after BIT shift 1 0000 0001 0000 0010 2 2 0000 0010 0000 0100 4 3 0000 0011 0000 0110 6 Effect of Shifting Bits Advantages and Disadvantages of C Programming Advantages of C Programming Language 1) Building block for many other programming languages C is considered to be the most fundamental language that needs to be studied if you are beginning with any programming language. Many programming languages such as Python, C++, Java, etc are built with the base of the C language. 2) Powerful and efficient language C is a robust language as it contains many data types and operators to give you a vast platform to perform all kinds of operations. 3) Portable language C is very flexible, or we can say machine independent that helps you to run your code on any machine without making any change or just a few changes in the code. 4) Built-in functions There are only 32 keywords in ANSI C, having many built-in functions. These functions are helpful when building a program in C. 5) Quality to extend itself Another crucial ability of C is to extend itself. C language has its own set of functions in the C library. So, it becomes easy to use these functions. We can add our own functions to the C Standard Library and make code simpler. 6) Structured programming language C is structure-based. It means that the issues or complex problems are divided into smaller blocks or functions. This modular structure helps in easier and simpler testing and maintenance. 7) Middle-level language C is a middle-level programming language that means it supports high-level programming as well as low-level programming. It supports the use of kernels and drivers in low-level programming and also supports system software applications in the high-level programming language. 8) Implementation of algorithms and data structures The use of algorithms and data structures in C has made program computations very fast and smooth. Thus, the C language can be used in complex calculations and operations such as MATLAB. 9) Procedural programming language C follows a proper procedure for its functions and subroutines. As it uses procedural programming, it becomes easier for C to identify code structure and to solve any problem in a specific series of code. In procedural programming C variables and functions are declared before use. 10) Dynamic memory allocation C provides dynamic memory allocation that means you are free to allocate memory at run time. For example, if you don’t know how much memory is required by objects in your program, you can still run a program in C and assign the memory at the same time. 11) System-programming C follows a system based programming system. It means the programming is done for the hardware devices. So, with this, we are aware of why C considered a very powerful language and why is it important to know the advantages of C? Disadvantages of C Programming language 1. Concept of OOPs C is a very vast language, but it does not support the concept of OOPs (Inheritance, Polymorphism, Encapsulation, Abstraction, Data Hiding). C simply follows the procedural programming approach. 2. Run-time checking In the C programming language, the errors or the bugs aren’t detected after each line of code. Instead, the compiler shows all the errors after writing the program. It makes the checking of code very complex in large programs. 3. Concept of namespace C does not implement the concept of namespaces. A namespace is structured as a chain of commands to allow the reuse of names in different contexts. Without namespaces, we cannot declare two variables of the same name. But, C programming lacks in this feature, and hence you cannot define a variable with the same name in C. 4. Lack of Exception Handling Exception Handling is one of the most important features of programming languages. While compiling the code, various anomalies and bugs can occur. Exception Handling allows you to catch the error and take appropriate responses. However, C does not exhibit this important feature. 5. Constructor or destructor C does not have any constructor or destructor. Constructors & Destructors support basic functionality of Object Oriented Programming. Both are member functions that are created as soon as an object of the class is created. You will be studying constructor and destructor in detail later on. 6. Low level of abstraction C is a small and core machine language that has minimum data hiding and exclusive visibility that affects the security of this language. Applications of C language System Programming − C language is used to develop system software which are close to hardware such as operating systems, firmware, language translators, etc. Embedded Systems − C language is used in embedded system programming for a wide range of devices such as microcontrollers, industrial controllers, etc. Compiler and Interpreters − C language is very common to develop language compilers and interpreters. Database Systems − Since C language is efficient and fast for low-level memory manipulation. It is used for developing DBMS and RDBMS engines. Applications of C language Networking Software − C language is used to develop networking software such as protocols, routers, and network utilities. Game Development − C language is widely used for developing games, gaming applications, and game engines. Scientific and Mathematical Applications − C language is efficient in developing applications where scientific computing is required. Applications such as simulations, numerical analysis, and other scientific computations are usually developed in C language. Text Editor and IDEs − C language is used for developing text editors and integrated development environments such as Vim and Emacs. Tokens in C Tokens in C is the most important element to be used in creating a program in C. We can define the token as the smallest individual element in C. For example, we cannot create a sentence without using words, similarly, we cannot create a program in C without using tokens in C. Therefore, we can say that tokens in C is the building block or the basic component for creating a program in C language. Classification of tokens in C C Keywords and Identifiers Keywords and Identifiers in the C language are the building block of any program. Keywords are predefined, which means the C language has a list of words that are Keywords, while an Identifier is user-defined, which means you while writing the C language program can specify identifiers. Let's see what these two are. What are Keywords? Every programming language has some reserved words that are used internally and have some meaning for the language, such words are called Reserved Keywords or just Keywords. C language has 32 keywords. Keywords in C Programming Language auto double int struct break else long switch case enum register typedef char extern return union const short float unsigned continue for signed void default goto sizeof volatile do if static while C Identifiers In the C language identifiers are the names given to variables, constants, functions, structures, pointers, or any other user-defined data, to identify them. Because it's up to the user to define the Identifiers, hence there are some rules that one has to follow, which are defined by the C language, to avoid unnecessary errors in the compiler. Rules for defining an Identifier: An identifier can only have alphanumeric characters(a-z , A-Z , 0-9) and underscore(_). The first character of an identifier can only contain alphabet(a-z, A-Z) or underscore (_). Identifiers are case-sensitive in the C language. For example, name and Name will be treated as two different identifiers. Keywords are not allowed to be used as Identifiers. Identifiers should be written in such a way that it is meaningful, short, and easy to read. The length of the identifiers should not be more than 31 characters. No special characters, such as semicolon, period, whitespaces, slash, or comma (? - + ! @ # % ^ & * ( ) [ ] { }. , ; : ’ ‘/ and \. ) are permitted to be used in or as an Identifier. What is variable in C ? A variable is a name given to a memory location to store values in a computer program. It is used to store information that can be referenced and manipulated in a program. We can choose any name for the variable, but it must follow the programming semantics. Let's say there are two values, 10 and 20, that we want to store and use in our program. For this, we need to use a variable, and we will do the below steps: Steps to create variable in C First, we will create or declare a variable with a suitable name. Assign those values to the variables to store them. Once these values are stores, we can use these variables with their name in our program. for example: int myvariable = 101; Here myvariable is the name or identifier for the variable which stores the value 101 in it. And int is the keyword. And all of them are tokens. Example Let's have another example, int money; double salary; Example of Valid Identifiers: Here are some valid identifiers, total, avg1, difference_1; Example of Invalid Identifiers: Here are some invalid identifiers, $myvar; // incorrect x!y; // again incorrect Using the Data Types Available in C language The types of data structures provided by C can be classified under the following categories: Fundamental data types Derived data types Fundamental Data Types Fundamental data types include the data types at the lowest level, i.e. those which are used for actual data representation in the memory. All other data types are based on the fundamental data types. Fundamental Data Types The fundamental data types are: char: For characters and strings int: For integers float: For numbers with decimals such as amounts, quotients, and salary Since the preceding data types are fundamental, i.e. at the machine level, the storage requirement is hardware dependent. The storage requirements of the fundamental data types are shown in the following table. Data Number of bytes on a Minimum value Maximum value type 32-bit (HORIZON) computer char 1 -128 127 int 4 -2,147,483,648 2,147,483,647 float 4 6 digits of 6 digits of precision precision Derived Data Types Derived data types are based on fundamental data types, i.e. a derived data type is represented in the memory as a fundamental data type. Some of the derived data types with their storage requirements are shown in the following table. Data type Number of Minimum value Maximum value bytes on a32- bit (HORIZON) computer short int 2 -2^15 (2^15) – 1 -32768 to 32767 long int 4 -2^31 (2^31) - 1 double 8 12 digits of 12 digits of float precision precision Storage Requirements of Derived Data Types Defining Data Definition of memory for any data, both fundamental and derived data types, is done in the following format: [data type] [variable name],...; All data is normally defined in the beginning of a function. The definition: char alph; defines a memory location of size one byte, of character type, referred to as alph. Definitions of various data types are shown in the following table. Data Data type Memory Size (bytes) Value definition defined assigned char a, c; char ac 1 - 1 - char a = 'Z'; char a 1 Z int count; int count 4 - int a, count int a count 4 - 10 =10; 4 float fnum; float fnum 4 - float fnum1, float fnum1 4 - 93.63 fnum2 = fnum2 4 93.63; It is evident from the preceding table that the contents of a variable are undefined, unless it is assigned a value by initializing it, or by using an input function. Practice (Just A Minute) Write the appropriate definitions for defining the following variables: 1. num to store integers. 2. chr to store a character and assign the character Z to it. 3. num to store a number and assign the value 8.93 to it. 4. i, j to store integers and assign the value 0 to j. Strings in C : Strings in C are always represented as an array of characters having null character '\0' at the end of the string. This null character denotes the end of the string. Strings in C are enclosed within double quotes, while characters are enclosed within single characters. The size of a string is a number of characters that the string contains. Ex:- char str=“CIMAGE”; char str[ ]=“CIMAGE”; char str={‘C’,’I’,’M’,’A’,’G’,’E’,’\0’}; Special characters in C Some special characters are used in C, and they have a special meaning which cannot be used for another purpose. Square brackets [ ]: The opening and closing brackets represent the single and multidimensional subscripts. Simple brackets ( ): It is used in function declaration and function calling. For example, printf() is a pre-defined function. Curly braces { }: It is used in the opening and closing of the code. It is used in the opening and closing of the loops. Comma (,): It is used for separating for more than one statement and for example, separating function parameters in a function call, separating the variable when printing the value of more than one variable using a single printf statement. Hash/pre-processor (#): It is used for pre- processor directive. It basically denotes that we are using the header file. Asterisk (*): This symbol is used to represent pointers and also used as an operator for multiplication. Period (.): It is used to access a member of a structure or a union. Example : #include int main() { const int a; const int b = 12; printf("The default value of variable a : %d", a); printf("The value of variable b : %d", b); return 0; } Compilation Process of C Programe Compiler, Linker, Assembler, and Loader Compiler : A compiler is a specialized system tool that translates a program written in a specific programming language into the assembly language code. The assembly language code is specific to each machine and is governed by the CPU of the machine. Assembler: The assembler enters the arena after the compiler has played its part. The assembler translates our assembly code to the machine code and then stores the result in an object file. This file contains the binary representation of our program. Moving further, the assembler gives a memory location to each object and instruction in our code. The memory location can be physical as well as virtual. A virtual location is an offset that is relative to the base address of the first instruction. Linker: Next, we move to the linker module. The linker spawns to action after the assembler has done its job. The linker combines all external programs (such as libraries and other shared components) with our program to create a final executable. At the end of the linking step, we get the executable for our program. So, the linker takes all object files as input, resolves all memory references, and finally merges these object files to make an executable file. Loader: The loader is a specialized operating system module that comes last in the picture. It loads the final executable code into memory. Afterward, it creates the program and data stack. Then, it initializes various registers and finally gives control to the CPU so that it can start executing the code. Identifying the Structure of C Functions In C language, the functions can be categorized in the following categories: Single-level functions Multiple-level functions Single-Level Functions The following function is a single-level function: main() { printf("Welcome to C"); } In a C program, main() is always the first function to be executed by the computer and should be present in all programs. It is like any other function available in the C library, or like one developed by a user. Parenthesis () are used for passing parameters (if any) to a function. The brackets {} mark the beginning and end of a function and are mandatory in all functions. enclose a comment line in a function, which is useful for documenting various parts of a function. The comment line may be in a separate line or part of an executable line. The comment line is optional. The semicolon is used for marking the end of an executable line. In the preceding code, printf() is a C function for printing (displaying) constant or variable data and Welcome to C is the parameter passed to it. This function will be discussed in detail in due course. Just A Minute Identify any erroneous or missing component(s) in the following functions: 1) main() { printf("This function seems to be okay") } 2) main() { printf("This function is perfect"; } 3) main() } printf("This function seems to be okay") { 4) main() { This is a perfect comment line printf("Is it okay?"); } Multiple-Level Functions The following is an example showing functions at multiple levels - one function being called by another function main() { printf("Welcome to C."); disp_msg(); printf("for good learning."); } disp_msg() { printf("All the best "); } The output of the preceding program is: Welcome to C. All the best for good learning. In the preceding program,.. disp_msg()is a user-defined function that is called from the main() function. The () can be used for passing values to functions, depending on whether the receiving function is expecting any parameter. As mentioned earlier, executable lines are terminated by a semi-colon (;). A statement calling/executing a function needs to be terminated by a semi-colon, but a statement marking the beginning of a function-definition does not have a semi-colon at the end. Using the Input-Output Functions The C environment assumes that the standard input device (Keyboard), the standard output device (VDU), and the standard error device (VDU) are always linked to the environment. For standard input-output operations, the C environment uses stdin, stdout, and stderr as references for accessing the devices. The C language, as such, does not provide for any input-output operations as part of the language. They exist as functions written in C and are available in the standard C library along with other functions. These input and output functions may be used by any programmer. Any input or output operation takes place as a stream of characters. The standard input-output functions may be dealt with character-based input-output functions and string- based (stream of characters) input-output functions. The standard input-output functions are buffered, i.e. each device has an associated buffer through which any input or output operation takes place. After an input operation from the standard input device, care must be taken to clear the standard input buffer lest the previous contents of the buffer interfere with subsequent input operations. The output buffer is cleared only if a newline character is used or if an input function is used or the buffer is explicitly cleared. Character-Based Input-Output Functions The following function accepts a single character from the keyboard (standard input device) and displays it on the VDU (standard output device): #include main() { char alph; alph=getc(stdin); putc(alph,stdout); } The function main() first defines a memory location, alph, of char type, and then invokes the functions getc() and putc(). The function getc() accepts as parameter the reference of device, and allows one character to be read from this device during program execution. In this case, the device is stdin. The character read is returned and assigned to alph. The function putc() accepts as parameters the character to be output, and the reference of the output device (stdout in this case) to which one character is to be output. The function fflush() clears the buffer associated with a specified input or output device (stdin in this case). It is a good programming practice to clear the buffer even if there are no subsequent input operations in a function. The statement #include includes at the time of compilation, the contents of the standard input-output file, stdio.h, which contains definitions of stdin, stdout, and stderr. Whenever the references stdin, stdout, and stderr are used in a function, this statement has to be used. Practice Write a function to input a character and display the character input twice. The function to input and display a character, discussed earlier, can also be written as: #include main() { char c; c=getchar(); fflush(stdin); putchar(c); } In the preceding function, getchar() is equivalent to getc(stdin) and putchar(c) is equivalent to putc(c, stdout). Practice Write a function to accept and store two characters in different memory locations, and to display them one after the other using the functions getchar() and putchar(). String-Based Input-Output Functions The functions getc(), getchar(), putc(), and putchar() perform character-based input-output. C language provides the functions gets() and puts() for string- based input-output, as shown in the following program: #include main() { char in_str; puts(“Enter a string of max 20 characters”); gets(in_str); fflush(stdin); puts(in_str); } It is a good programming practice to prompt for input by displaying a message before an input. This has been done by using the puts() function in this program. The function puts() accepts, as parameter, a string variable or a string constant (enclosed in double quotes) for display-on the standard output. The puts() function causes the cursor to jump to the next line after printing the string. The function gets() accepts the string variable into the location where the input string is to be stored. Practice 1) Write a function that prompts for and accepts a name with a maximum of 25 characters, and displays the following message. Hello. How are you? (name) 2) Write a function that prompts for a name (up to 20 characters) and address (up to 30 characters) and accepts them one at a time. Finally, the name and address are displayed in the following way. Your name is: (name) Your address is: (address) Format Specifiers for I/O Data Type Format Specifier int %d char %c float %f double %lf long double %Lf short int %hd unsigned int %u long int %li long long int %lli unsigned long int %lu unsigned long long int %llu printf() and scanf() in C The printf() and scanf() functions are used for input and output in C language. Both functions are inbuilt library functions, defined in stdio.h (header file). printf() function The printf() function is used for output. It prints the given statement to the console. The syntax of printf() function is given below: printf("format string",argument_list); The format string can be %d (integer), %c (character), %s (string), %f (float) etc. scanf() function The scanf() function is used for input. It reads the input data from the console. scanf("format string",argument_list); Program to print cube of given number. #include int main(){ int num; printf("enter a number:"); scanf("%d",&num); printf("cube of number is:%d ",num*num*num); return 0; } Escape Sequence in C An escape sequence in C language is a sequence of characters that doesn't represent itself when used inside string literal or character. It is composed of two or more characters starting with backslash \. For example: \n represents new line. Escape Sequence Name Description It is used to generate \a Alarm or Beep a bell sound in the C program. It is used to move the \b Backspace cursor backward. It moves the cursor to \n New Line the start of the next line. It moves the cursor to \r Carriage Return the start of the current line. It inserts some whitespace to the left \t Horizontal Tab of the cursor and moves the cursor accordingly. Use to insert \\ Backlash backslash character. It is used to display a \’ Single Quote single quotation mark. It is used to display \” Double Quote double quotation marks. It represents the NLL \0 NULL character. C Character I/O #include int main() { char chr; printf("Enter a character: "); scanf("%c", &chr); // When %c is used, a character is displayed printf("You entered %c.\n",chr); // When %d is used, ASCII value is displayed printf("ASCII value is %d.", chr); return 0; } C Numeric I/O #include int main() { int testInteger; float num1; double num2; printf("Enter an integer: "); scanf("%d", &testInteger); printf("Enter a Fractional number: "); scanf("%f", &num1); printf("Enter another fractional number: "); scanf("%lf", &num2); printf("num1 = %f\n", num1); printf("num2 = %lf", num2); printf("Number = %d",testInteger); return 0; } I/O Multiple Values #include int main() { int a; float b; printf("Enter integer and then a float: "); // Taking multiple inputs scanf("%d%f", &a, &b); printf("You entered %d and %f", a, b); return 0; } #include main() { char name,add,f,coll,g; int n1,n2; printf("Enter two numbers to add\t"); scanf("%d%d",&n1,&n2); getchar(); printf("Enter your Name :"); scanf("%[^\n]%*c", name); printf("Enter Your gender(1 character) "); scanf("%c",&g); getchar(); printf("Enter Colege Name "); gets(coll); printf("Enter your Fathers Name :"); fgets(f,31,stdin); printf("Enter your Address :"); scanf("%[^\n]s", add); printf("Your name is %s\n",name); printf("Your Gender is %c \n",g); printf("Your College name is %s\n",coll); printf("Your Fathers name is %s\n",f); printf("Your Address is %s\n",add); printf("Sum of two numbers is\t%d",n1+n2); } sizeof operator in C sizeof is a much-used operator in the C. It is a compile-time unary operator which can be used to compute the size of its operand. The result of sizeof is of the unsigned integral type which is usually denoted by size_t. Sizeof can be applied to any data type, including primitive types such as integer and floating-point types, pointer types, or compound data types such as Structure, union, etc. Usage of sizeof() operator // C Program To demonstrate sizeof operator #include int main() { printf("%d\n", sizeof(char)); printf("%d\n", sizeof(int)); printf("%d\n", sizeof(float)); printf("%d\n", sizeof(double)); printf("%d\n", sizeof(long double)); printf("%d\n", sizeof(short int)); printf("%d\n", sizeof(unsigned int)); printf("%d\n", sizeof(long int)); printf("%d\n", sizeof(long long int)); printf("%d\n", sizeof(unsigned long int)); printf("%d\n", sizeof(unsigned long long int)); return 0; } Operators in C Operators in C is a special symbol used to perform the functions. The data items on which the operators are applied are known as operands. Operators are applied between the operands. Depending on the number of operands, operators are classified as follows. Unary Operator A unary operator is an operator applied to the single operand. For example: increment operator (++), decrement operator (--), sizeof, (type)* Binary Operator The binary operator is an operator applied between two operands. The following is the list of the binary operators: Ex:-Arithmetic Operators, Relational Operators, Shift Operators, Logical Operators, Bitwise Operators, Conditional Operators, Assignment Operator, Misc Operator. C Arithmetic Operators An arithmetic operator performs mathematical operations such as addition, subtraction, multiplication, division etc on numerical values (constants and variables). Operator Meaning of Operator + addition or unary plus - subtraction or unary minus * multiplication / division % remainder after division (modulo division) Example: Arithmetic Operators // Working of arithmetic operators #include int main() { int a = 9,b = 4, c; c = a+b; printf("a+b = %d \n",c); c = a-b; printf("a-b = %d \n",c); c = a*b; printf("a*b = %d \n",c); c = a/b; printf("a/b = %d \n",c); c = a%b; printf("Remainder when a divided by b = %d \n",c); return 0; } C Assignment Operators An assignment operator is used for assigning a value to a variable. The most common assignment operator is = Operator Example Same as = a=b a= b += a += b a= a+b -= a -= b a= a-b *= a *= b a= a*b /= a /= b a= a/b %= a %= b a= a%b Example : Assignment Operators // Working of assignment operators #include int main() { int a = 5, c; c = a; // c is 5 printf("c = %d\n", c); c += a; // c is 10 printf("c = %d\n", c); c -= a; // c is 5 printf("c = %d\n", c); c *= a; // c is 25 printf("c = %d\n", c); c /= a; // c is 5 printf("c = %d\n", c); c %= a; // c = 0 printf("c = %d\n", c); return 0; } C Relational Operators Relational operators are used in decision making and loops. Operator Meaning of Operator Example == Equal to 5 == 3 is evaluated to 0 > Greater than 5 > 3 is evaluated to 1 < Less than 5 < 3 is evaluated to 0 != Not equal to 5 != 3 is evaluated to 1 >= Greater than or equal to 5 >= 3 is evaluated to 1 b); printf("%d > %d is %d \n", a, c, a > c); printf("%d < %d is %d \n", a, b, a < b); printf("%d < %d is %d \n", a, c, a < c); printf("%d != %d is %d \n", a, b, a != b); printf("%d != %d is %d \n", a, c, a != c); printf("%d >= %d is %d \n", a, b, a >= b); printf("%d >= %d is %d \n", a, c, a >= c); printf("%d 2 then a will become a=a/(2^2). Thus, a=a/(2^2)=2 which can be written as 10. Explanation The below image illustrates the step-by-step shifting for 8 >> 2 Example #include int main() { int x = 100; int y; printf("Enter number of positions for the bits to Right to the left : "); // Taking input of the second operand. scanf("%d", &y); printf("After performing Right shift operation : "); printf("%d >> %d = %d", x, y, x >> y); return 0; } Loop Constructs Looping Statements in C execute the sequence of statements many times until the stated condition becomes false. A loop in C consists of two parts, a body of a loop and a control statement. The control statement is a combination of some conditions that direct the body of the loop to execute until the specified condition becomes false. The purpose of the C loop is to repeat the same code a number of times. Why looping? The looping simplifies the complex problems into the easy ones. It enables to alter the flow of the program so that instead of writing the same code again and again, we can execute the same code for a finite number of times. For example, if we need to print ‘Hello Friends’ 10- times then, instead of using the printf statement 10 times, we can use printf once inside a loop which runs up to 10 iterations. What are the advantages of Looping? 1) It provides code reusability. 2) Using loops, we do not need to write the same code again and again. 3) Using loops, we can traverse over the elements of data structures (array or linked lists). Types of C Loops There are three types of loops in C language those are given below: while Do while for Essential components of a loop Counter Initialization of the counter with initial value Condition to check with the optimum value of the counter Statement(s) to be executed by iteration Increment/decrement while loop in C The while loop in c is to be used in the scenario where the block of statements is executed in the while loop until the condition specified in the while loop is satisfied. It is also called a pre-tested loop. The syntax of while loop in c language is given below: initialisation; while(condition) { block of statements to be executed ; increment ; } Flowchart of while loop Write a C-program to print 10 natural numbers #include main() { int i=1; while(i