java-software-solutions_compress.pdf
Document Details
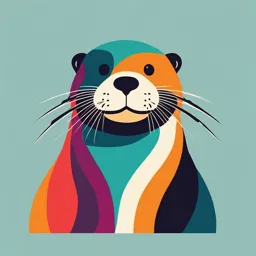
Uploaded by AmazedAllusion
University of South Carolina Upstate
Related
Full Transcript
Java™ Software Solutions Foundations of Program Design Ninth Edition John Lewis Virginia Tech William Loftus Accenture 330 Hudson Street, NY NY 10013 Director, Portfolio Management: Engineering, Computer Science & Global Editions: Julian Partridge Specialist, Higher Ed Portfolio Management: Ma...
Java™ Software Solutions Foundations of Program Design Ninth Edition John Lewis Virginia Tech William Loftus Accenture 330 Hudson Street, NY NY 10013 Director, Portfolio Management: Engineering, Computer Science & Global Editions: Julian Partridge Specialist, Higher Ed Portfolio Management: Matt Goldstein Portfolio Management Assistant: Kristy Alaura Managing Content Producer: Scott Disanno Content Producer: Carole Snyder Web Developer: Steve Wright Rights and Permissions Manager: Ben Ferrini Manufacturing Buyer, Higher Ed, Lake Side Communications Inc (LSC): Maura Zaldivar-Garcia Inventory Manager: Ann Lam Product Marketing Manager: Yvonne Vannatta Field Marketing Manager: Demetrius Hall Marketing Assistant: Jon Bryant Cover Designer: Joyce Wells Project Management: Louise C. Capulli, Lakeside Editorial Services, L.L.C. Full-Service Project Management: Revathi Viswanathan, Cenveo Publisher Services Credits and acknowledgments borrowed from other sources and reproduced, with permission, appear on the Credits page at the end of the front matter of this textbook. Copyright © 2017, 2015, 2012, 2009 Pearson Education, Inc. All rights reserved. Printed in the United States of America. This publication is protected by Copyright, and permission should be obtained from the publisher prior to any prohibited reproduction, storage in a retrieval system, or transmission in any form or by any means, electronic, mechanical, photocopying, recording, or likewise. For information regarding permissions, request forms and the appropriate contacts within the Pearson Education Global Rights & Permissions department, please visit www.pearsonhighed.com/permissions/. Many of the designations by manufacturers and sellers to distinguish their products are claimed as trademarks. Where those designations appear in this book, and the publisher was aware of a trademark claim, the designations have been printed in initial caps or all caps. The programs and applications presented in this book have been included for their instructional value. They have been tested with care, but are not guaranteed for any particular purpose. The publisher does not offer any warranties or representations, nor does it accept any liabilities with respect to the programs or applications. Library of Congress Cataloging-in-Publication Data Names: Lewis, John, 1963- author. | Loftus, William, author. Title: Java software solutions : foundations of program design / John Lewis, Virginia Tech; William Loftus, Accenture. Description: Ninth edition. | Boston : Pearson, 2017. Identifiers: LCCN 2016054660| ISBN 9780134462028 | ISBN 0134462025 Subjects: LCSH: Java (Computer program language) | Object-oriented programming (Computer science) Classification: LCC QA76.73.J38 L49 2017 | DDC 005.1/17–dc23 LC record available at https://lccn.loc.gov/2016054660 2013047763 10 9 8 7 6 5 4 3 2 1—DOC—15 14 13 12 11 ISBN 10: 0-13-446202-5 ISBN 13: 978-0-13-446202-8 This book is dedicated to our families. Sharon, Justin, Kayla, Nathan, and Samantha Lewis and Veena, Isaac, and Dévi Loftus Preface Welcome to the Ninth Edition of Java Software Solutions: Foundations of Program Design. We are pleased that this book has served the needs of so many students and faculty over the years. This edition has been tailored further to improve the coverage of topics key to introductory computing. New to This Edition The biggest change to this edition of Java Software Solutions is a sweeping overhaul of the Graphics Track sections of the book to fully embrace the JavaFX API. Swing is no longer actively supported by Oracle. JavaFX is now the preferred approach for developing graphics and graphical user interfaces (GUIs) in Java, and we make use of it throughout this text. The changes include the following: Coverage of JavaFX graphical shapes. Coverage of JavaFX controls, including buttons, text fields, check boxes, radio buttons, choice boxes, color pickers, date pickers, dialog boxes, sliders, and spinners. Use of Java 8 method references and lambda expressions to define event handlers. An exploration of the JavaFX class hierarchy. An explanation of JavaFX properties and property binding. Revised end-of-chapter exercises and programming projects. A new appendix (Appendix G ) that presents an overview of JavaFX layout panes. A new appendix (Appendix H ) that introduces the JavaFX Scene Builder software. There are two exciting aspects to embracing JavaFX. First, it provides a much cleaner approach to GUI development than Swing did. Equivalent programs using JavaFX are shorter and more easily understood. Second, the JavaFX approach embraces core object-oriented principles better than Swing did. For example, all graphic shapes are represented by classes with fundamental data elements, such as a Circle class with a radius. Early on (Chapter 3 ), the shape classes provide a wealth of basic, well-designed classes, just when students need to understand what classes and objects are all about. The use of Java 8 method references provides an easy-to-understand approach to defining event handlers. The use of the (underlying) lambda expressions is also explored as an alternative approach. JavaFX layout panes are used and explained as needed in examples, with a full overview of layout panes provided in a new appendix. We think this works better than the way we treated Swing layout managers, as a separate topic in a chapter. All GUI development in the book is done “by hand” in straight Java code, which is important for beginning students. The JavaFX drag- and-drop Scene Builder is discussed in a new appendix, but it is not used in the book itself. In addition to the changes related to JavaFX, we also updated examples and discussions in various places throughout the book as needed to bring them up-to-date and improve their pedagogy. We’re excited about the opportunities this new edition of Java Software Solutions provides for both students and instructors. As always, questions and comments are welcome. Cornerstones of the Text This text is based on the following basic ideas that we believe make for a sound introductory text: True object-orientation. A text that really teaches a solid object- oriented approach must use what we call object-speak. That is, all processing should be discussed in object-oriented terms. That does not mean, however, that the first program a student sees must discuss the writing of multiple classes and methods. A student should learn to use objects before learning to write them. This text uses a natural progression that culminates in the ability to design real object-oriented solutions. Sound programming practices. Students should not be taught how to program; they should be taught how to write good software. There’s a difference. Writing software is not a set of cookbook actions, and a good program is more than a collection of statements. This text integrates practices that serve as the foundation of good programming skills. These practices are used in all examples and are reinforced in the discussions. Students learn how to solve problems as well as how to implement solutions. We introduce and integrate basic software engineering techniques throughout the text. The Software Failure vignettes reiterate these lessons by demonstrating the perils of not following these sound practices. Examples. Students learn by example. This text is filled with fully implemented examples that demonstrate specific concepts. We have intertwined small, readily understandable examples with larger, more realistic ones. There is a balance between graphics and nongraphics programs. The VideoNotes provide additional examples in a live presentation format. Graphics and GUIs. Graphics can be a great motivator for students, and their use can serve as excellent examples of object- orientation. As such, we use them throughout the text in a well- defined set of sections that we call the Graphics Track. The book fully embraces the JavaFX API, the preferred and fully-supported approach to Java graphics and GUIs. Students learn to build GUIs in the appropriate way by using a natural progression of topics. The Graphics Track can be avoided entirely for those who do not choose to use graphics. Chapter Breakdown Chapter 1 (Introduction) introduces computer systems in general, including basic architecture and hardware, networking, programming, and language translation. Java is introduced in this chapter, and the basics of general program development, as well as object-oriented programming, are discussed. This chapter contains broad introductory material that can be covered while students become familiar with their development environment. Chapter 2 (Data and Expressions) explores some of the basic types of data used in a Java program and the use of expressions to perform calculations. It discusses the conversion of data from one type to another and how to read input interactively from the user with the help of the standard Scanner class. Chapter 3 (Using Classes and Objects) explores the use of predefined classes and the objects that can be created from them. Classes and objects are used to manipulate character strings, produce random numbers, perform complex calculations, and format output. Enumerated types are also discussed. Chapter 4 (Writing Classes) explores the basic issues related to writing classes and methods. Topics include instance data, visibility, scope, method parameters, and return types. Encapsulation and constructors are covered as well. Some of the more involved topics are deferred to or revisited in Chapter 6. Chapter 5 (Conditionals and Loops) covers the use of boolean expressions to make decisions. Then the if statement and while loop are explored in detail. Once loops are established, the concept of an iterator is introduced and the Scanner class is revisited for additional input parsing and the reading of text files. Finally, the ArrayList class introduced, which provides the option for managing a large number of objects. Chapter 6 (More Conditionals and Loops) examines the rest of Java’s conditional ( switch ) and loop ( do , for ) statements. All related statements for conditionals and loops are discussed, including the enhanced version of the for loop. The for-each loop is also used to process iterators and ArrayList objects. Chapter 7 (Object-Oriented Design) reinforces and extends the coverage of issues related to the design of classes. Techniques for identifying the classes and objects needed for a problem and the relationships among them are discussed. This chapter also covers static class members, interfaces, and the design of enumerated type classes. Method design issues and method overloading are also discussed. Chapter 8 (Arrays) contains extensive coverage of arrays and array processing. The nature of an array as a low-level programming structure is contrasted to the higher-level object management approach. Additional topics include command-line arguments, variable length parameter lists, and multidimensional arrays. Chapter 9 (Inheritance) covers class derivations and associated concepts such as class hierarchies, overriding, and visibility. Strong emphasis is put on the proper use of inheritance and its role in software design. Chapter 10 (Polymorphism) explores the concept of binding and how it relates to polymorphism. Then we examine how polymorphic references can be accomplished using either inheritance or interfaces. Sorting is used as an example of polymorphism. Design issues related to polymorphism are examined as well. Chapter 11 (Exceptions) explores the class hierarchy from the Java standard library used to define exceptions, as well as the ability to define our own exception objects. We also discuss the use of exceptions when dealing with input and output and examine an example that writes a text file. Chapter 12 (Recursion) covers the concept, implementation, and proper use of recursion. Several examples from various domains are used to demonstrate how recursive techniques make certain types of processing elegant. Chapter 13 (Collections) introduces the idea of a collection and its underlying data structure. Abstraction is revisited in this context and the classic data structures are explored. Generic types are introduced as well. This chapter serves as an introduction to a CS2 course. Supplements Student Online Resources These student resources can be accessed at the book’s Companion Website, “www.pearsonhighered.com/cs-resources” Source Code for all the programs in the text Links to Java development environments VideoNotes: short step-by-step videos demonstrating how to solve problems from design through coding. VideoNotes allow for self- paced instruction with easy navigation including the ability to select, play, rewind, fast-forward, and stop within each VideoNote exercise. Margin icons in your textbook let you know when a VideoNote video is available for a particular concept or homework problem. Online Practice and Assessment with MyProgrammingLab MyProgrammingLab helps students fully grasp the logic, semantics, and syntax of programming. Through practice exercises and immediate, personalized feedback, MyProgrammingLab improves the programming competence of beginning students who often struggle with the basic concepts and paradigms of popular high-level programming languages. A self-study and homework tool, MyProgrammingLab consists of hundreds of small practice exercises organized around the structure of this textbook. For students, the system automatically detects errors in the logic and syntax of their code submissions and offers targeted hints that enable students to figure out what went wrong—and why. For instructors, a comprehensive gradebook tracks correct and incorrect answers and stores the code submitted by students for review. MyProgrammingLab is offered to users of this book in partnership with Turing’s Craft, the makers of the CodeLab interactive programming exercise system. For a full demonstration, to see feedback from instructors and students, or to get started using MyProgrammingLab in your course, visit www.myprogramminglab.com. Instructor Resources The following supplements are available to qualified instructors only. Visit the Pearson Education Instructor Resource Center (www.pearsonhighered.com/irc) for information on how to access them: Presentation Slides—in PowerPoint. Solutions to end-of-chapter Exercises. Solutions to end-of-chapter Programming Projects. Features Key Concepts Throughout the text, the Key Concept boxes highlight fundamental ideas and important guidelines. These concepts are summarized at the end of each chapter. Listings All programming examples are presented in clearly labeled listings, followed by the program output, a sample run, or screen shot display as appropriate. The code is colored to visually distinguish comments and reserved words. Syntax Diagrams At appropriate points in the text, syntactic elements of the Java language are discussed in special highlighted sections with diagrams that clearly identify the valid forms for a statement or construct. Syntax diagrams for the entire Java language are presented in Appendix L. Graphics Track All processing that involves graphics and graphical user interfaces is discussed in one or two sections at the end of each chapter that we collectively refer to as the Graphics Track. This material can be skipped without loss of continuity, or focused on specifically as desired. The material in any Graphics Track section relates to the main topics of the chapter in which it is found. Graphics Track sections are indicated by a brown border on the edge of the page. Summary of Key Concepts The Key Concepts presented throughout a chapter are summarized at the end of the chapter. Self-Review Questions and Answers These short-answer questions review the fundamental ideas and terms established in the preceding section. They are designed to allow students to assess their own basic grasp of the material. The answers to these questions can be found at the end of the book in Appendix N. Exercises These intermediate problems require computations, the analysis or writing of code fragments, and a thorough grasp of the chapter content. While the exercises may deal with code, they generally do not require any online activity. Programming Projects These problems require the design and implementation of Java programs. They vary widely in level of difficulty. MyProgrammingLab Through practice exercises and immediate, personalized feedback, MyProgrammingLab improves the programming competence of beginning students who often struggle with the basic concepts and paradigms of popular high-level programming languages. VideoNotes Presented by the author, VideoNotes explain topics visually through informal videos in an easy-to-follow format, giving students the extra help they need to grasp important concepts. Look for this VideoNote icon to see which in-chapter topics and end-of-chapter Programming Projects are available as VideoNotes. Software Failures These between-chapter vignettes discuss real-world flaws in software design, encouraging students to adopt sound design practices from the beginning. Acknowledgments I am most grateful to the faculty and students from around the world who have provided their feedback on previous editions of this book. I am pleased to see the depth of the faculty’s concern for their students and the students’ thirst for knowledge. Your comments and questions are always welcome. I am particularly thankful for the assistance, insight, and attention to detail of Robert Burton from Brigham Young University. For years, Robert has consistently provided valuable feedback that helps shape and evolve this textbook. Bradley Richards, at the University of Applied Sciences in Northwestern Switzerland, provided helpful advice and resources during the transition to JavaFX. Brian Fraser of Simon Fraser University also has provided some excellent feedback that helped clarify some issues. Such interaction with computing educators is incredibly valuable. I also want to thank Dan Joyce from Villanova University, who developed the original Self-Review questions, ensuring that each relevant topic had enough review material, as well as developing the answers to each. I continue to be amazed at the talent and effort demonstrated by the team at Pearson. Matt Goldstein, our editor, has amazing insight and commitment. His assistant, Kristy Alaura, is a source of consistent and helpful support. Marketing Manager Demetrius Hall makes sure that instructors understand the pedagogical advantages of the text. The cover was designed by the skilled talents of Joyce Wells. Scott Disanno and Carole Snyder led the production effort. Louise Capulli, of Lakeside Editorial Services, was the Project Manager for this edition and a huge help to the author on a daily basis. We thank all of these people for ensuring that this book meets the highest quality standards. Special thanks go to the following people who provided valuable advice to us about this book via their participation in focus groups, interviews, and reviews. They, as well as many other instructors and friends, have provided valuable feedback. They include: Elizabeth Adams James Madison University Hossein Assadipour Rutgers University David Atkins University of Oregon Lewis Barnett University of Richmond Thomas W. Bennet Mississippi College Gian Mario Besana DePaul University Hans-Peter Bischof Rochester Institute of Technology Don Braffitt Radford University Robert Burton Brigham Young University John Chandler Oklahoma State University Robert Cohen University of Massachusetts, Boston Dodi Coreson Linn Benton Community College James H. Cross II Auburn University Eman El-Sheikh University of West Florida Sherif Elfayoumy University of North Florida Christopher Eliot University of Massachusetts, Amherst Wanda M. Eanes Macon State College Stephanie Elzer Millersville University Matt Evett Eastern Michigan University Marj Feroe Delaware County Community College, Pennsylvania John Gauch University of Kansas Chris Haynes Indiana University James Heliotis Rochester Institute of Technology Laurie Hendren McGill University Mike Higgs Austin College Stephen Hughes Roanoke College Daniel Joyce Villanova University Saroja Kanchi Kettering University Gregory Kapfhammer Allegheny College Karen Kluge Dartmouth College Jason Levy University of Hawaii Peter MacKenzie McGill University Jerry Marsh Oakland University Blayne Mayfield Oklahoma State University Gheorghe Muresan Rutgers University Laurie Murphy Pacific Lutheran University Dave Musicant Carleton College Faye Navabi-Tadayon Arizona State University Lawrence Osborne Lamar University Barry Pollack City College of San Francisco B. Ravikumar University of Rhode Island David Riley University of Wisconsin (La Crosse) Bob Roos Allegheny College Carolyn Rosiene University of Hartford Jerry Ross Lane Community College Patricia Roth Southeastern Polytechnic State University Carolyn Schauble Colorado State University Arjit Sengupta Georgia State University Bennet Setzer Kennesaw State University Vijay Srinivasan JavaSoft, Sun Microsystems, Inc. Stuart Steiner Eastern Washington University Katherine St. John Lehman College, CUNY Alexander Stoytchev Iowa State University Ed Timmerman University of Maryland, University College Shengru Tu University of New Orleans Paul Tymann Rochester Institute of Technology John J. Wegis JavaSoft, Sun Microsystems, Inc. Ken Williams North Carolina Agricultural and Technical University Linda Wilson Dartmouth College David Wittenberg Brandeis University Wang-Chan Wong California State University (Dominguez Hills) Thanks also go to my friends and former colleagues at Villanova University who have provided so much wonderful feedback. They include Bob Beck, Cathy Helwig, Anany Levitin, Najib Nadi, Beth Taddei, and Barbara Zimmerman. Thanks also to Pete DePasquale, formerly of The College of New Jersey and now with SailThru, Inc. Many other people have helped in various ways. They include Ken Arnold, Mike Czepiel, John Loftus, Sebastian Niezgoda, and Saverio Perugini. Our apologies to anyone we may have omitted. The ACM Special Interest Group on Computer Science Education (SIGCSE) is a tremendous resource. Their conferences provide an opportunity for educators from all levels and all types of schools to share ideas and materials. If you are an educator in any area of computing and are not involved with SIGCSE, you’re missing out. Contents Preface v Chapter 1 Introduction 1 1.1 Computer Processing 2 Software Categories 3 Digital Computers 5 Binary Numbers 7 1.2 Hardware Components 10 Computer Architecture 11 Input/Output Devices 12 Main Memory and Secondary Memory 13 The Central Processing Unit 17 1.3 Networks 19 Network Connections 20 Local-Area Networks and Wide-Area Networks 21 The Internet 22 The World Wide Web 24 Uniform Resource Locators 25 1.4 The Java Programming Language 26 A Java Program 27 Comments 29 Identifiers and Reserved Words 30 White Space 33 1.5 Program Development 35 Programming Language Levels 36 Editors, Compilers, and Interpreters 38 Development Environments 40 Syntax and Semantics 40 Errors 41 1.6 Object-Oriented Programming 43 Problem Solving 44 Object-Oriented Software Principles 45 Chapter 2 Data and Expressions 55 2.1 Character Strings 56 The print and println Methods 56 String Concatenation 58 Escape Sequences 61 2.2 Variables and Assignment 63 Variables 63 The Assignment Statement 65 Constants 67 2.3 Primitive Data Types 69 Integers and Floating Points 69 Characters 71 Booleans 72 2.4 Expressions 73 Arithmetic Operators 73 Operator Precedence 74 Increment and Decrement Operators 78 Assignment Operators 79 2.5 Data Conversion 81 Conversion Techniques 83 2.6 Interactive Programs 85 The Scanner Class 85 Software Failure: NASA Mars Climate Orbiter and Polar Lander 96 Chapter 3 Using Classes and Objects 99 3.1 Creating Objects 100 Aliases 102 3.2 The String Class 104 3.3 Packages 108 The import Declaration 110 3.4 The Random Class 112 3.5 The Math Class 115 3.6 Formatting Output 118 The NumberFormat Class 118 The DecimalFormat Class 120 The printf Method 121 3.7 Enumerated Types 124 3.8 Wrapper Classes 127 Autoboxing 129 3.9 Introduction to JavaFX 129 3.10 Basic Shapes 133 3.11 Representing Colors 140 Chapter 4 Writing Classes 147 4.1 Classes and Objects Revisited 148 4.2 Anatomy of a Class 150 Instance Data 155 UML Class Diagrams 155 4.3 Encapsulation 157 Visibility Modifiers 158 Accessors and Mutators 159 4.4 Anatomy of a Method 160 The return Statement 162 Parameters 163 Local Data 163 Bank Account Example 164 4.5 Constructors Revisited 169 4.6 Arcs 170 4.7 Images 173 Viewports 175 4.8 Graphical User Interfaces 176 Alternate Ways to Specify Event Handlers 179 4.9 Text Fields 180 Software Failure: Denver Airport Baggage Handling System 189 Chapter 5 Conditionals and Loops 191 5.1 Boolean Expressions 192 Equality and Relational Operators 193 Logical Operators 194 5.2 The if Statement 197 The if-else Statement 200 Using Block Statements 203 Nested if Statements 207 5.3 Comparing Data 210 Comparing Floats 210 Comparing Characters 211 Comparing Objects 212 5.4 The while Statement 214 Infinite Loops 218 Nested Loops 220 The break and continue Statements 223 5.5 Iterators 225 Reading Text Files 226 5.6 The ArrayList Class 229 5.7 Determining Event Sources 232 5.8 Managing Fonts 234 5.9 Check Boxes 237 5.10 Radio Buttons 241 Software Failure: Therac-25 253 Chapter 6 More Conditionals and Loops 255 6.1 The switch Statement 256 6.2 The Conditional Operator 260 6.3 The do Statement 261 6.4 The for Statement 265 The for-each Loop 268 Comparing Loops 270 6.5 Using Loops and Conditionals with Graphics 271 6.6 Graphic Transformations 276 Translation 276 Scaling 276 Rotation 277 Shearing 278 Applying Transformations on Groups 279 Chapter 7 Object-Oriented Design 289 7.1 Software Development Activities 290 7.2 Identifying Classes and Objects 291 Assigning Responsibilities 293 7.3 Static Class Members 293 Static Variables 294 Static Methods 294 7.4 Class Relationships 298 Dependency 298 Dependencies Among Objects of the Same Class 298 Aggregation 304 The this Reference 308 7.5 Interfaces 310 The Comparable Interface 315 The Iterator Interface 316 7.6 Enumerated Types Revisited 317 7.7 Method Design 320 Method Decomposition 321 Method Parameters Revisited 326 7.8 Method Overloading 331 7.9 Testing 333 Reviews 334 Defect Testing 334 7.10 GUI Design 337 7.11 Mouse Events 338 7.12 Key Events 343 Software Failure: 2003 Northeast Blackout 352 Chapter 8 Arrays 355 8.1 Array Elements 356 8.2 Declaring and Using Arrays 357 Bounds Checking 360 Alternate Array Syntax 365 Initializer Lists 365 Arrays as Parameters 366 8.3 Arrays of Objects 368 8.4 Command-Line Arguments 378 8.5 Variable Length Parameter Lists 380 8.6 Two-Dimensional Arrays 384 Multidimensional Arrays 388 8.7 Polygons and Polylines 389 8.8 An Array of Color Objects 392 8.9 Choice Boxes 395 Software Failure: LA Air Traffic Control 405 Chapter 9 Inheritance 407 9.1 Creating Subclasses 408 The protected Modifier 411 The super Reference 414 Multiple Inheritance 417 9.2 Overriding Methods 419 Shadowing Variables 421 9.3 Class Hierarchies 422 The Object Class 424 Abstract Classes 425 Interface Hierarchies 427 9.4 Visibility 427 9.5 Designing for Inheritance 430 Restricting Inheritance 431 9.6 Inheritance in JavaFX 432 9.7 Color and Date Pickers 434 9.8 Dialog Boxes 438 File Choosers 441 Software Failure: Ariane 5 Flight 501 449 Chapter 10 Polymorphism 451 10.1 Late Binding 452 10.2 Polymorphism via Inheritance 453 10.3 Polymorphism via Interfaces 466 10.4 Sorting 468 Selection Sort 469 Insertion Sort 475 Comparing Sorts 476 10.5 Searching 477 Linear Search 477 Binary Search 479 Comparing Searches 483 10.6 Designing for Polymorphism 483 10.7 Properties 485 Change Listeners 488 10.8 Sliders 491 10.9 Spinners 493 Chapter 11 Exceptions 501 11.1 Exception Handling 502 11.2 Uncaught Exceptions 503 11.3 The try-catch Statement 504 The finally Clause 508 11.4 Exception Propagation 509 11.5 The Exception Class Hierarchy 513 Checked and Unchecked Exceptions 516 11.6 I/O Exceptions 517 11.7 Tool Tips and Disabling Controls 521 11.8 Scroll Panes 525 11.9 Split Panes and List Views 528 Chapter 12 Recursion 537 12.1 Recursive Thinking 538 Infinite Recursion 538 Recursion in Math 539 12.2 Recursive Programming 540 Recursion vs. Iteration 543 Direct vs. Indirect Recursion 543 12.3 Using Recursion 544 Traversing a Maze 545 The Towers of Hanoi 550 12.4 Tiled Images 555 12.5 Fractals 559 Chapter 13 Collections 573 13.1 Collections and Data Structures 574 Separating Interface from Implementation 574 13.2 Dynamic Representations 575 Dynamic Structures 575 A Dynamically Linked List 576 Other Dynamic List Representations 581 13.3 Linear Collections 583 Queues 583 Stacks 584 13.4 Non-Linear Data Structures 587 Trees 587 Graphs 588 13.5 The Java Collections API 590 Generics 590 Appendix A Glossary 597 Appendix B Number Systems 621 Appendix C The Unicode Character Set 629 Appendix D Java Operators 633 Appendix E Java Modifiers 639 Appendix F Java Coding Guidelines 643 Appendix G JavaFX Layout Panes 649 Appendix H JavaFX Scene Builder 659 Appendix I Regular Expressions 669 Appendix J Javadoc Documentation Generator 671 Appendix K Java Syntax 677 Appendix L Answers to Self-Review Questions 691 Index 745 VideoNote Overview of program elements. 28 Comparison of Java IDEs. 40 Examples of various error types. 42 Developing a solution for PP 1.2. 53 Example using strings and escape sequences. 61 Review of primitive data and expressions. 74 Example using the Scanner class. 89 Developing a solution of PP 2.10. 94 Creating objects. 101 Example using the Random and Math classes. 115 Developing a solution of PP 3.6. 145 Dissecting the Die class. 152 Discussion of the Account class. 166 Developing a solution of PP 4.2. 186 Examples using conditionals. 205 Examples using while loops. 217 Developing a solution of PP 5.4. 250 Examples using for loops. 266 Developing a solution of PP 6.2. 285 Exploring the static modifier. 293 Examples of method overloading. 332 Developing a solution of PP 7.1. 349 Overview of arrays. 359 Discussion of the LetterCount example. 364 Developing a solution of PP 8.5. 403 Overview of inheritance. 413 Example using a class hierarchy. 425 Exploring the Firm program. 454 Sorting Comparable objects. 474 Developing a solution of PP 10.1. 498 Proper exception handling. 509 Developing a solution of PP 11.1. 534 Tracing the MazeSearch program. 548 Exploring the Towers of Hanoi. 551 Developing a solution of PP 12.1. 569 Example using a linked list. 576 Implementing a queue. 584 Developing a solution of PP 13.3. 594 Credits Cover: Photocase Addicts GmbH/Alamy Stock Photo Figure 2.1 : NASA EOS Earth Observing System Figure 4.2 : Susan Van Etten/PhotoEdit Figure 5.1 : David Joel/The Image Bank/Getty Images Figures 4.1a , 4.1b , 6.1a , 6.1b , 6.2 , 11.3 , 11.8, 11.9, 11.10, 12.5 , APG.1a, APG.1b: Pixabay Figures 7.1a and 7.1b : Anarres/Openclipart Figures 7.2a and 7.2b : National Oceanic and Atmospheric Administration Figure 8.1 : Matthew McVay/The Image Bank/Getty Images Figure 9.1 : Mario Fourmy/Redux Pictures Figure H.1 : NASA 1 Introduction Chapter Objectives Describe the relationship between hardware and software. Define various types of software and how they are used. Identify the core hardware components of a computer and explain their roles. Explain how the hardware components interact to execute programs and manage data. Describe how computers are connected into networks to share information. Introduce the Java programming language. Describe the steps involved in program compilation and execution. Present an overview of object-oriented principles. This book is about writing well-designed software. To understand software, we must first have a fundamental understanding of its role in a computer system. Hardware and software cooperate in a computer system to accomplish complex tasks. The purpose of various hardware components and the way those components are connected into networks are important prerequisites to the study of software development. This chapter first discusses basic computer processing and then begins our exploration of software development by introducing the Java programming language and the principles of object- oriented programming. 1.1 Computer Processing All computer systems, whether it’s a desktop, laptop, tablet, smartphone, gaming console, or a special-purpose device such as a car’s navigation system, share certain characteristics. The details vary, but they all process data in similar ways. While the majority of this book deals with the development of software, we’ll begin with an overview of computer processing to set the context. It’s important to establish some fundamental terminology and see how key pieces of a computer system interact. A computer system is made up of hardware and software. The hardware components of a computer system are the physical, tangible pieces that support the computing effort. They include chips, boxes, wires, keyboards, speakers, disks, memory cards, universal serial bus (USB) flash drives (also called jump drives), cables, plugs, printers, mice, monitors, routers, and so on. If you can physically touch it and it can be considered part of a computer system, then it is computer hardware. Key Concept A computer system consists of hardware and software that work in concert to help us solve problems. The hardware components of a computer are essentially useless without instructions to tell them what to do. A program is a series of instructions that the hardware executes one after another. Software consists of programs and the data that programs use. Software is the intangible counterpart to the physical hardware components. Together they form a tool that we can use to help solve problems. The key hardware components in a computer system are central processing unit (CPU) input/output (I/O) devices main memory secondary memory devices Each of these hardware components is described in detail in the next section. For now, let’s simply examine their basic roles. The central processing unit (CPU) is a device that executes the individual commands of a program. Input/output (I/O) devices , such as the keyboard, mouse, trackpad, and monitor, allow a human being to interact with the computer. Programs and data are held in storage devices called memory, which fall into two categories: main memory and secondary memory. Main memory is the storage device that holds the software while it is being processed by the CPU. Secondary memory devices store software in a relatively permanent manner. The most important secondary memory device of a typical computer system is the hard disk that resides inside the main computer box. A USB flash drive is also an important secondary memory device. A typical USB flash drive cannot store nearly as much information as a hard disk. USB flash drives have the advantage of portability; they can be removed temporarily or moved from computer to computer as needed. Figure 1.1 shows how information moves among the basic hardware components of a computer. Suppose you have an executable program you wish to run. The program is stored on some secondary memory device, such as a hard disk. When you instruct the computer to execute your program, a copy of the program is brought in from secondary memory and stored in main memory. The CPU reads the individual program instructions from main memory. The CPU then executes the instructions one at a time until the program ends. The data that the instructions use, such as two numbers that will be added together, also are stored in main memory. They are either brought in from secondary memory or read from an input device such as the keyboard. During execution, the program may display information to an output device such as a monitor. Figure 1.1 A simplified view of a computer system Key Concept The CPU reads the program instructions from main memory, executing them one at a time until the program ends. The process of executing a program is fundamental to the operation of a computer. All computer systems basically work in the same way. Software Categories Software can be classified into many categories using various criteria. At this point, we will simply differentiate between system programs and application programs. The operating system is the core software of a computer. It performs two important functions. First, it provides a user interface that allows the user to interact with the machine. Second, the operating system manages computer resources such as the CPU and main memory. It determines when programs are allowed to run, where they are loaded into memory, and how hardware devices communicate. It is the operating system’s job to make the computer easy to use and to ensure that it runs efficiently. Key Concept The operating system provides a user interface and manages computer resources. Several popular operating systems are in use today. The Windows operating system was developed for personal computers by Microsoft, which has captured the lion’s share of the operating systems market. Various versions of the Unix operating system are also quite popular, especially in larger computer systems. A version of Unix called Linux was developed as an open-source project, which means that many people contributed to its development and its code is freely available. Because of that Linux has become a particular favorite among some users. Mac OS is an operating system used for computing systems developed by Apple Computers. Operating systems are often specialized for mobile devices such as smartphones and tablets. The iOS operating system from Apple is used on the iPhone, iPad, and iPod. It is similar in functionality and appearance to the desktop Mac OS, but tailored for the smaller devices. The Android operating system developed by Google currently dominates the smartphone market. An application (often shortened in conversation to “app”) is a generic term for just about any software other than the operating system. Word processors, missile control systems, database managers, Web browsers, and games all can be considered application programs. Each application program has its own user interface that allows the user to interact with that particular program. The user interface for most modern operating systems and applications is a graphical user interface (GUI , pronounced “gooey”), which, as the name implies, makes use of graphical screen elements. Among many others, these elements include windows, which are used to separate the screen into distinct work areas icons , which are small images that represent computer resources, such as a file menus, checkboxes, and radio buttons, which provide the user with selectable options sliders , which allow the user to select from a range of values buttons , which can be “pushed” with a mouse click to indicate a user selection A mouse or trackpad is the primary input device used with GUIs; thus, GUIs are sometimes called point-and-click interfaces. The screenshot in Figure 1.2 shows an example of a GUI. Figure 1.2 An example of a graphical user interface (GUI) Key Concept As far as the user is concerned, the interface is the program. The interface to an application or operating system is an important part of the software because it is the only part of the program with which the user interacts directly. To the user, the interface is the program. Throughout this book, we discuss the design and implementation of graphical user interfaces. The focus of this book is the development of high-quality application programs. We explore how to design and write software that will perform calculations, make decisions, and present results textually or graphically. We use the Java programming language throughout the text to demonstrate various computing concepts. Digital Computers Two fundamental techniques are used to store and manage information: analog and digital. Analog information is continuous, in direct proportion to the source of the information. For example, an alcohol thermometer is an analog device for measuring temperature. The alcohol rises in a tube in direct proportion to the temperature outside the tube. Another example of analog information is an electronic signal used to represent the vibrations of a sound wave. The signal’s voltage varies in direct proportion to the original sound wave. A stereo amplifier sends this kind of electronic signal to its speakers, which vibrate to reproduce the sound. We use the term analog because the signal is directly analogous to the information it represents. Figure 1.3 graphically depicts a sound wave captured by a microphone and represented as an electronic signal. Figure 1.3 A sound wave and an electronic analog signal that represents the wave Key Concept Digital computers store information by breaking it into pieces and representing each piece as a number. Digital technology breaks information into discrete pieces and represents those pieces as numbers. The music on a compact disc is stored digitally, as a series of numbers. Each number represents the voltage level of one specific instance of the recording. Many of these measurements are taken in a short period of time, perhaps 44,000 measurements every second. The number of measurements per second is called the sampling rate. If samples are taken often enough, the discrete voltage measurements can be used to generate a continuous analog signal that is “close enough” to the original. In most cases, the goal is to create a reproduction of the original signal that is good enough to satisfy the human senses. Figure 1.4 shows the sampling of an analog signal. When analog information is converted to a digital format by breaking it into pieces, we say it has been digitized. Because the changes that occur in a signal between samples are lost, the sampling rate must be sufficiently fast. Figure 1.4 Digitizing an analog signal by sampling Sampling is only one way to digitize information. For example, a sentence of text is stored on a computer as a series of numbers, where each number represents a single character in the sentence. Every letter, digit, and punctuation symbol has been assigned a number. Even the space character is assigned a number. Consider the following sentence: Hi, Heather. The characters of the sentence are represented as a series of 12 numbers, as shown in Figure 1.5. When a character is repeated, such as the uppercase 'H' , the same representation number is used. Note that the uppercase version of a letter is stored as a different number from the lowercase version, such as the 'H' and 'h' in the word Heather. They are considered distinct characters. Figure 1.5 Text is stored by mapping each character to a number Modern electronic computers are digital. Every kind of information, including text, images, numbers, audio, video, and even program instructions, is broken into pieces. Each piece is represented as a number. The information is stored by storing those numbers. Binary Numbers A digital computer stores information as numbers, but those numbers are not stored as decimal values. All information in a computer is stored and managed as binary values. Unlike the decimal system, which has 10 digits (0 through 9), the binary number system has only two digits (0 and 1). A single binary digit is called a bit. All number systems work according to the same rules. The base value of a number system dictates how many digits we have to work with and indicates the place value of each digit in a number. The decimal number system is base 10, whereas the binary number system is base 2. Appendix B contains a detailed discussion of number systems. Key Concept Binary is used to store and move information in a computer because the devices that store and manipulate binary data are inexpensive and reliable. Modern computers use binary numbers because the devices that store and move information are less expensive and more reliable if they have to represent only one of two possible values. Other than this characteristic, there is nothing special about the binary number system. Computers have been created that use other number systems to store and move information, but they aren’t as convenient. Some computer memory devices, such as hard drives, are magnetic in nature. Magnetic material can be polarized easily to one extreme or the other, but intermediate levels are difficult to distinguish. Therefore, magnetic devices can be used to represent binary values quite effectively—a magnetized area represents a binary 1 and a demagnetized area represents a binary 0. Other computer memory devices are made up of tiny electrical circuits. These devices are easier to create and are less likely to fail if they have to switch between only two states. We’re better off reproducing millions of these simple devices than creating fewer, more complicated ones. Binary values and digital electronic signals go hand in hand. They improve our ability to transmit information reliably along a wire. As we’ve seen, an analog signal has continuously varying voltage with infinitely many states, but a digital signal is discrete, which means the voltage changes dramatically between one extreme (such as +5 volts) and the other (such as –5 volts). At any point, the voltage of a digital signal is considered to be either “high,” which represents a binary 1, or “low,” which represents a binary 0. Figure 1.6 compares these two types of signals. Figure 1.6 An analog signal vs. a digital signal As a signal moves down a wire, it gets weaker and degrades due to environmental conditions. That is, the voltage levels of the original signal change slightly. The trouble with an analog signal is that as it fluctuates, it loses its original information. Since the information is directly analogous to the signal, any change in the signal changes the information. The changes in an analog signal cannot be recovered because the degraded signal is just as valid as the original. A digital signal degrades just as an analog signal does, but because the digital signal is originally at one of two extremes, it can be reinforced before any information is lost. The voltage may change slightly from its original value, but it still can be interpreted correctly as either high or low. The number of bits we use in any given situation determines the number of unique items we can represent. A single bit has two possible values, 0 and 1, and therefore can represent two possible items or situations. If we want to represent the state of a light bulb (off or on), one bit will suffice, because we can interpret 0 as the light bulb being off and 1 as the light bulb being on. If we want to represent more than two things, we need more than one bit. Two bits, taken together, can represent four possible items because there are exactly four permutations of two bits: 00, 01, 10, and 11. Suppose we want to represent the gear that a car is in (park, drive, reverse, or neutral). We would need only two bits, and could set up a mapping between the bit permutations and the gears. For instance, we could say that 00 represents park, 01 represents drive, 10 represents reverse, and 11 represents neutral. In this case, it wouldn’t matter if we switched that mapping around, though in some cases the relationships between the bit permutations and what they represent are important. Three bits can represent eight unique items, because there are eight permutations of three bits. Similarly, four bits can represent 16 items, five bits can represent 32 items, and so on. Figure 1.7 shows the relationship between the number of bits used and the number of items they can represent. In general, N bits can represent 2N unique items. For every bit added, the number of items that can be represented doubles. Key Concept There are exactly 2N permutations of N bits. Therefore, N bits can represent up to 2N unique items. Figure 1.7 The number of bits used determines the number of items that can be represented We’ve seen how a sentence of text is stored on a computer by mapping characters to numeric values. Those numeric values are stored as binary numbers. Suppose we want to represent character strings in a language that contains 256 characters and symbols. We would need to use eight bits to store each character because there are 256 unique permutations of eight bits (28 equals 256). Each bit permutation, or binary value, is mapped to a specific character. How many bits would be needed to represent 195 countries of the world? Seven wouldn’t be enough, because 27 equals 128. Eight bits would be enough, but some of the 256 permutations would not be mapped to a country. Ultimately, representing information on a computer boils down to the number of items there are to represent and determining the way those items are mapped to binary values. Self-Review Questions (see answers in Appendix L ) SR 1.1 What is hardware? What is software? SR 1.2 What are the two primary functions of an operating system? SR 1.3 The music on a CD is created using a sampling rate of 44,000 measurements per second. Each measurement is stored as a number that represents a specific voltage level. How many such numbers are used to store a three-minute long song? How many such numbers does it take to represent one hour of music? SR 1.4 What happens to information when it is stored digitally? SR 1.5 How many unique items can be represented with the following? a. 2 bits b. 4 bits c. 5 bits d. 7 bits SR 1.6 Suppose you want to represent each of the 50 states of the United States using a unique permutation of bits. How many bits would be needed to store each state representation? Why? 1.2 Hardware Components Let’s examine the hardware components of a computer system in more detail. Consider the desktop computer described in Figure 1.8. What does it all mean? Is the system capable of running the software you want it to? How does it compare with other systems? These terms are explained throughout this section. Figure 1.8 The hardware specification of a particular computer Computer Architecture The architecture of a house defines its structure. Similarly, we use the term computer architecture to describe how the hardware components of a computer are put together. Figure 1.9 illustrates the basic architecture of a generic computer system. Information travels between components across a group of wires called a bus. Figure 1.9 Basic computer architecture Key Concept The core of a computer is made up of main memory, which stores programs and data, and the CPU, which executes program instructions one at a time. The CPU and the main memory make up the core of a computer. As we mentioned earlier, main memory stores programs and data that are in active use, and the CPU methodically executes program instructions one at a time. The CPU in the computer described in Figure 1.8 is manufactured by the Intel Corporation, which supplies processors for many computer systems. Suppose we have a program that computes the average of a list of numbers. The program and the numbers must reside in main memory while the program runs. The CPU reads one program instruction from main memory and executes it. If an instruction needs data, such as a number in the list, to perform its task, the CPU reads that information as well. This process repeats until the program ends. The average, when computed, is stored in main memory to await further processing or long-term storage in secondary memory. Almost all devices in a computer system other than the CPU and main memory are called peripherals ; they operate at the periphery, or outer edges, of the system (although they may be in the same box). Users don’t interact directly with the CPU or main memory. Although they form the essence of the machine, the CPU and main memory would not be useful without peripheral devices. Controllers are devices that coordinate the activities of specific peripherals. Every device has its own particular way of formatting and communicating data, and part of the controller’s role is to handle these idiosyncrasies and isolate them from the rest of the computer hardware. Furthermore, the controller often handles much of the actual transmission of information, allowing the CPU to focus on other activities. Input/output (I/O) devices and secondary memory devices are considered peripherals. Another category of peripherals consists of data transfer devices , which allow information to be sent and received between computers. The computer specified in Figure 1.8 includes a network card that uses the 802.11 standard for wireless radio communication (or WiFi) to a computer network. In some ways, secondary memory devices and data transfer devices can be thought of as I/O devices because they represent a source of information (input) and a place to send information (output). For our discussion, however, we define I/O devices as those devices that allow the user to interact with the computer. Input/Output Devices Let’s examine some I/O devices in more detail. The most common input devices are the keyboard and the mouse or trackpad. Others include bar code readers, such as the one used at a retail store checkout microphones, used by voice recognition systems that interpret voice commands virtual reality devices, such as handheld devices that interpret the movement of the user’s hand scanners, which convert text, photographs, and graphics into machine-readable form cameras, which capture still pictures and video, and can also be used to process special input such as QR codes Monitors and printers are the most common output devices. Others include plotters, which move pens across large sheets of paper (or vice versa) speakers, for audio output goggles, for virtual reality display Some devices can provide both input and output capabilities. A touch screen system can detect the user touching the screen at a particular place. Software can then use the screen to display text and graphics in response to the user’s touch. Touch screens have become commonplace for handheld devices. The computer described in Figure 1.8 includes a monitor with a 15.6-inch display area (measured diagonally). A picture is represented in a computer by breaking it up into separate picture elements, or pixels. This monitor can display a grid of 1366 by 768 pixels. Main Memory and Secondary Memory Main memory is made up of a series of small, consecutive memory locations , as shown in Figure 1.10. Associated with each memory location is a unique number called an address. Key Concept An address is a unique number associated with a memory location. Figure 1.10 Memory locations When data is stored in a memory location, it overwrites and destroys any information that was previously stored at that location. However, the process of reading data from a memory location does not affect it. On many computers, each memory location consists of eight bits, or one byte, of information. If we need to store a value that cannot be represented in a single byte, such as a large number, then multiple, consecutive bytes are used to store the data. The storage capacity of a device such as main memory is the total number of bytes it can hold. Devices can store thousands or millions of bytes, so you should become familiar with larger units of measure. Because computer memory is based on the binary number system, all units of storage are powers of two. A kilobyte (KB) is 1024, or 210, bytes. Some larger units of storage are a megabyte (MB), a gigabyte (GB), a terabyte (TB), and a petabyte (PB) as listed in Figure 1.11. It’s usually easier to think about these capacities by rounding them off. For example, most computer users think of a kilobyte as approximately one thousand bytes, a megabyte as approximately one million bytes, and so forth. Figure 1.11 Units of binary storage Key Concept Main memory is volatile, meaning the stored information is maintained only as long as electric power is supplied. Many personal computers have 4 GB of main memory, such as the system described in Figure 1.8. A large main memory allows large programs or multiple programs to run efficiently, because they don’t have to retrieve information from secondary memory as often. Main memory is usually volatile, meaning that the information stored in it will be lost if its electric power supply is turned off. When you are working on a computer, you should often save your work onto a secondary memory device such as a USB flash drive in case the power goes out. Secondary memory devices are usually nonvolatile; the information is retained even if the power supply is turned off. The cache is used by the CPU to reduce the average access time to instructions and data. The cache is a small, fast memory that stores the contents of the most frequently used main memory locations. Contemporary CPUs include an instruction cache to speed up the fetching of executable instructions and a data cache to speed up the fetching and storing of data. The most common secondary storage devices are hard disks and USB flash drives. A typical USB flash drive stores between 1 and 256 GB of information. The storage capacities of hard drives vary, but on personal computers, capacities typically range between 500 and 750 GB, such as in the system described in Figure 1.8. Some hard disks can store much more. A USB flash drive consists of a small printed circuit board carrying the circuit elements and a USB connector, insulated electrically and protected inside a plastic, metal, or rubberized case, which can be carried in a pocket or on a key chain, for example. A disk is a magnetic medium on which bits are represented as magnetized particles. A read/write head passes over the spinning disk, reading or writing information as appropriate. A hard disk drive might actually contain several disks in a vertical column with several read/write heads, such as the one shown in Figure 1.12. Figure 1.12 A hard disk drive with multiple disks and read/write heads Before disk drives were common, magnetic tapes were used as secondary storage. Tapes are considerably slower than hard disk and USB flash drives because of the way information is accessed. A hard disk is a direct access device since the read/write head can move, in general, directly to the information needed. A USB flash drive is also a direct access device, but nothing moves mechanically. The terms direct access and random access are often used interchangeably. However, information on a tape can be accessed only after first getting past the intervening data. A tape must be rewound or fast- forwarded to get to the appropriate position. A tape is therefore considered a sequential access device. For these reasons, tapes are no longer used as a computing storage device, just as audio cassettes were supplanted by compact discs. Two other terms are used to describe memory devices: random access memory (RAM) and read-only memory (ROM). It’s important to understand these terms because they are used often and their names can be misleading. The terms RAM and main memory are basically interchangeable, describing the memory where active programs and data are stored. ROM can refer to chips on the computer motherboard or to portable storage such as a compact disc. ROM chips typically store software called BIOS (basic input/output system) that provide the preliminary instructions needed when the computer is turned on initially. After information is stored on ROM, generally it is not altered (as the term read-only implies) during typical computer use. Both RAM and ROM are direct (or random) access devices. Key Concept The surface of a CD has both smooth areas and small pits. A pit represents a binary 1 and a smooth area represents a binary 0. A CD-ROM is a portable secondary memory device. CD stands for compact disc. It is called ROM because information is stored permanently when the CD is created and cannot be changed. Like its musical CD counterpart, a CD-ROM stores information in binary format. When the CD is initially created, a microscopic pit is pressed into the disc to represent a binary 1, and the disc is left smooth to represent a binary 0. The bits are read by shining a low-intensity laser beam onto the spinning disc. The laser beam reflects strongly from a smooth area on the disc but weakly from a pitted area. A sensor receiving the reflection determines whether each bit is a 1 or a 0 accordingly. A typical CD-ROM’s storage capacity ranges between 650 and 900 MB. Variations on basic CD technology emerged quickly. A CD- Recordable (CD-R) disk can be used to create a CD for music or for general computer storage. Once created, you can use a CD-R disc in a standard CD player, but you can’t change the information on a CD-R disc once it has been “burned.” Music CDs that you buy are pressed from a mold, whereas CD-Rs are burned with a laser. CDs were initially a popular format for music; they later evolved to be used as a general computer storage device. Similarly, the DVD format was originally created for video and is now making headway as a general format for computer data. DVD once stood for digital video disc or digital versatile disc, but now the acronym generally stands on its own. A DVD has a tighter format (more bits per square inch) than a CD and can therefore store more information. The use of CDs and DVDs as secondary storage devices for computers has declined greatly as advances in networks and external “cloud” storage provided better options. The capacity of storage devices changes continually as technology improves. A general rule in the computer industry suggests that storage capacity approximately doubles every 18 months. However, this progress eventually will slow down as capacities approach absolute physical limits. The Central Processing Unit The CPU interacts with main memory to perform all fundamental processing in a computer. The CPU interprets and executes instructions, one after another, in a continuous cycle. It is made up of three important components, as shown in Figure 1.13. The control unit coordinates the processing steps, the registers provide a small amount of storage space in the CPU itself, and the arithmetic/logic unit performs calculations and makes decisions. The registers are the smallest, fastest cache in the system. Figure 1.13 CPU components and main memory The control unit coordinates the transfer of data and instructions between main memory and the registers in the CPU. It also coordinates the execution of the circuitry in the arithmetic/logic unit to perform operations on data stored in particular registers. In most CPUs, some registers are reserved for special purposes. For example, the instruction register holds the current instruction being executed. The program counter is a register that holds the address of the next instruction to be executed. In addition to these and other special-purpose registers, the CPU also contains a set of general- purpose registers that are used for temporary storage of values as needed. The concept of storing both program instructions and data together in main memory is the underlying principle of the von Neumann architecture of computer design, named after John von Neumann, a Hungarian-American mathematician who first advanced this programming concept in 1945. These computers continually follow the fetch–decode–execute cycle depicted in Figure 1.14. An instruction is fetched from main memory at the address stored in the program counter and is put into the instruction register. The program counter is incremented at this point to prepare for the next cycle. Then, the instruction is decoded electronically to determine which operation to carry out. Finally, the control unit activates the correct circuitry to carry out the instruction, which may load a data value into a register or add two values together, for example. Figure 1.14 The continuous fetch–decode–execute cycle Key Concept The fetch-decode-execute cycle forms the foundation of computer processing. The CPU is constructed on a chip called a microprocessor, a device that is part of the main circuit board of the computer. This board also contains ROM chips and communication sockets to which device controllers, such as the controller that manages the video display, can be connected. Another crucial component of the main circuit board is the system clock. The clock generates an electronic pulse at regular intervals, which synchronizes the events of the CPU. The rate at which the pulses occur is called the clock speed, and it varies depending on the processor. The computer described in Figure 1.8 includes an Intel Dual Core i7 processor that runs at a clock speed of 3.1 GHz, or approximately 3.1 billion pulses per second. The speed of the system clock provides a rough measure of how fast the CPU executes instructions. A processor described as duel-core actually has two processors built onto one chip, and can do two things at once if the program it is executing is designed for that. However, it is difficult to write programs that can take advantage of that second processor. This will become increasingly more important for software developers, because the future of processor design is likely to be more cores, not single cores that run at faster speeds. Self-Review Questions (see answers in Appendix L ) SR 1.7 How many bytes are there in each of the following? a. 3 KB b. 2 MB c. 4 GB SR 1.8 How many bits are there in each of the following? a. 8 bytes b. 2 KB c. 4 MB SR 1.9 The music on a CD is created using a sampling rate of 44,000 measurements per second. Each measurement is stored as a number that represents a specific voltage level. Suppose each of these numbers requires two bytes of storage space. How many MB does it take to represent one hour of music? SR 1.10 What are the two primary hardware components in a computer? How do they interact? SR 1.11 What is a memory address? SR 1.12 What does volatile mean? Which memory devices are volatile and which are nonvolatile? SR 1.13 Select the word from the following list that best matches each of the following phrases: controller, CPU, main, network card, peripheral, RAM, register, ROM, secondary a. Almost all devices in a computer system, other than the CPU and the main memory, are categorized as this. b. A device that coordinates the activities of a peripheral device. c. Allows information to be sent and received. d. This type of memory is usually volatile. e. This type of memory is usually nonvolatile. f. This term basically is interchangeable with the term “main memory.” g. Where the fundamental processing of a computer takes place. 1.3 Networks A network consists of two or more computers connected together so they can exchange information. Using networks has become the normal mode of commercial computer operation. New technologies are emerging every day to capitalize on the connected environments of modern computer systems. Key Concept A network consists of two or more computers connected together so that they can exchange information. Figure 1.15 shows a simple computer network. One of the devices on the network is a printer, which allows any computer connected to the network to print a document on that printer. One of the computers on the network is designated as a file server, which is dedicated to storing programs and data that are needed by many network users. A file server usually has a large amount of secondary memory. When a network has a file server, each individual computer doesn’t need its own copy of a program. Figure 1.15 A simple computer network Network Connections If two computers are directly connected, they can communicate in basically the same way that information moves across wires inside a single machine. When connecting two geographically close computers, this solution works well and is called a point-to-point connection. However, consider the task of connecting many computers together across large distances. If point-to-point connections are used, every computer is directly connected by a wire to every other computer in the network. A separate wire for each connection is not a workable solution because every time a new computer is added to the network, a new communication line will have to be installed for each computer already in the network. Furthermore, a single computer can handle only a small number of direct connections. Figure 1.16 shows multiple point-to-point connections. Consider the number of communication lines that would be needed if two or three additional computers were added to the network. These days, local networks, such as those within a single building, often use wireless connections, minimizing the need for running wires. Figure 1.16 Point-to-point connections Compare the diagrams in Figures 1.15 and 1.16. All of the computers shown in Figure 1.15 share a single communication line. Each computer on the network has its own network address, which uniquely identifies it. These addresses are similar in concept to the addresses in main memory except that they identify individual computers on a network instead of individual memory locations inside a single computer. A message is sent across the line from one computer to another by specifying the network address of the computer for which it is intended. Sharing a communication line is cost effective and makes adding new computers to the network relatively easy. However, a shared line introduces delays. The computers on the network cannot use the communication line at the same time. They have to take turns sending information, which means they have to wait when the line is busy. Key Concept Sharing a communication line creates delays, but it is cost effective and simplifies adding new computers to the network. One technique to improve network delays is to divide large messages into segments, called packets, and then send the individual packets across the network intermixed with pieces of other messages sent by other users. The packets are collected at the destination and reassembled into the original message. This situation is similar to a group of people using a conveyor belt to move a set of boxes from one place to another. If only one person were allowed to use the conveyor belt at a time, and that person had a large number of boxes to move, the others would be waiting a long time before they could use it. By taking turns, each person can put one box on at a time, and they all can get their work done. It’s not as fast as having a conveyor belt of your own, but it’s not as slow as having to wait until everyone else is finished. Local-Area Networks and Wide- Area Networks A local-area network (LAN) is designed to span short distances and connect a relatively small number of computers. Usually, a LAN connects the machines in only one building or in a single room. LANs are convenient to install and manage and are highly reliable. As computers became increasingly small and versatile, LANs provided an inexpensive way to share information throughout an organization. However, having a LAN is like having a telephone system that allows you to call only the people in your own town. We need to be able to share information across longer distances. Key Concept A local-area network (LAN) is an effective way to share information and resources throughout an organization. A wide-area network (WAN) connects two or more LANs, often across long distances. Usually one computer on each LAN is dedicated to handling the communication across a WAN. This technique relieves the other computers in a LAN from having to perform the details of long-distance communication. Figure 1.17 shows several LANs connected into a WAN. The LANs connected by a WAN are often owned by different companies or organizations and might even be located in different countries. Figure 1.17 LANs connected into a WAN The Internet Throughout the 1970s, an agency in the Department of Defense known as the Advanced Research Projects Agency (ARPA) funded several projects to explore network technology. One result of these efforts was the ARPANET, a wide-area network that eventually became known as the Internet. The Internet is a network of networks. The term Internet comes from the WAN concept of internetworking— connecting many smaller networks together. In 1983, there were fewer than 600 computers connected to the Internet. At the present time, the Internet serves billions of users worldwide. As more and more computers connected to the Internet, the task of keeping up with the larger number of users and heavier traffic became difficult. New technologies have replaced the ARPANET several times since the initial development, each time providing more capacity and faster processing. Key Concept The Internet is a wide-area network (WAN) that spans the globe. A protocol is a set of rules that governs how two things communicate. The software that controls the movement of messages across the Internet must conform to a set of protocols called TCP/IP (pronounced by spelling out the letters, T-C-P-I-P). TCP stands for Transmission Control Protocol, and IP stands for Internet Protocol. The IP software defines how information is formatted and transferred from the source to the destination. The TCP software handles problems such as pieces of information arriving out of their original order or information getting lost, which can happen if too much information converges at one location at the same time. Every computer connected to the Internet has an IP address that uniquely identifies it among all other computers on the Internet. An example of an IP address is 204.192.116.2. Fortunately, the users of the Internet rarely have to deal with IP addresses. The Internet allows each computer to be given a name. Like IP addresses, the names must be unique. The Internet name of a computer is often referred to as its Internet address. An example of Internet address is hector.vt.edu. Key Concept Every computer connected to the Internet has an IP address that uniquely identifies it. The first part of an Internet address is the local name of a specific computer. The rest of the address is the domain name , which indicates the organization to which the computer belongs. For example, vt.edu is the domain name for the network of computers at Virginia Tech, and hector is the name of a particular computer on that campus. Because the domain names are unique, many organizations can have a computer named hector without confusion. Individual departments might be assigned subdomains that are added to the basic domain name to uniquely distinguish their set of computers within the larger organization. For example, the cs.vt.edu subdomain is devoted to the Department of Computer Science at Virginia Tech. The last part of each domain name, called a top-level domain (TLD), usually indicates the type of organization to which the computer belongs. The TLD edu indicates an educational institution. The TLD com usually refers to a commercial business. Another common TLD is org , used by nonprofit organizations. Other top-level domains include biz , info , jobs , and name. Many computers, especially those outside of the United States, use a country-code top-level domain that denotes the country of origin, such as uk for the United Kingdom or au for Australia. When an Internet address is referenced, it gets translated to its corresponding IP address, which is used from that point on. The software that does this translation is called the Domain Name System (DNS). Each organization connected to the Internet operates a domain server that maintains a list of all computers at that organization and their IP addresses. You provide the name, and the domain server gives back a number. If the local domain server does not have the IP address for the name, it contacts another domain server that does. Initially, the primary use of interconnected computers was to send electronic mail, but Internet capabilities grew quickly. One of the most significant uses of the Internet today is the World Wide Web. The World Wide Web The Internet gives us the capability to exchange information. The World Wide Web (also known as WWW or simply the Web) makes the exchange of information easy for humans. Web software provides a common user interface through which many different types of information can be accessed with the click of a mouse. Key Concept The World Wide Web is software that makes sharing information across a network easy for humans. The Web is based on the concepts of hypertext and hypermedia. The term hypertext was coined in 1965 by Ted Nelson. It describes a way to organize information so that the flow of ideas was not constrained to a linear progression. Paul Otlet (1868–1944), considered by some to be the father of information science, envisioned that concept as a way to manage large amounts of information. The underlying idea is that documents can be linked at various points according to natural relationships so that the reader can jump from one document to another, following the appropriate path for that reader’s needs. When other media components are incorporated, such as graphics, sound, animations, and video, the resulting organization is called hypermedia. The terms Internet and World Wide Web are sometimes used interchangeably, but there are important differences between the two. The Internet makes it possible to communicate via computers around the world. The Web makes that communication a straightforward and enjoyable activity. The Web is essentially a distributed information service based on a set of software applications. A browser is a software tool that loads and formats Web documents for viewing. Mosaic, the first graphical interface browser for the Web, was released in 1993. The designer of a Web document refers to other Web information that might be anywhere on the Internet. Popular browsers include Google Chrome, Apple Safari, Mozilla Firefox, and Opera. The use of Internet Explorer, from Microsoft, has recently declined with the rise of alternatives. The Microsoft Edge browser has recently replaced Internet Explorer. A computer dedicated to providing access to Web documents is called a Web server. Browsers load and interpret documents provided by a Web server. Many such documents are formatted using the HyperText Markup Language (HTML). The Java programming language has an intimate relationship with Web processing, because links to Java programs can be embedded in HTML documents and executed through Web browsers. Uniform Resource Locators Information on the Web is found by identifying a Uniform Resource Locator (URL, pronounced by spelling out the letters U-R-L). A URL uniquely specifies documents and other information for a browser to obtain and display. The following is an example URL: http://www.google.com The Web site at this particular URL is the home of the well-known Google search engine, which enables you to search the Web for information using particular words or phrases. A URL contains several pieces of information. The first piece is a protocol, which determines the way the browser transmits and processes information. The second piece is the Internet address of the machine on which the document is stored. The third piece of information is the file name or resource of interest. If no file name is given, as is the case with the Google URL, the Web server usually provides a default page. Key Concept A URL uniquely specifies documents and other information found on the Web for a browser to obtain and display. Let’s look at another example URL: https://www.whitehouse.gov/issues/education In this URL, the protocol is https, which stands for a secure version of the HyperText Transfer Protocol. The machine referenced is www (a typical reference to a Web server), found at domain whitehouse.gov. Finally, issues/education represents a file (or a reference that generates a file) to be transferred to the browser for viewing. Many other forms for URLs exist, but this form is the most common. Self-Review Questions (see answers in Appendix L ) SR 1.14 What is a file server? SR 1.15 What is the total number of communication lines needed for a fully connected point-to-point network of five computers? Six computers? SR 1.16 Describe a benefit of having computers on a network share a communication line. Describe a cost/drawback of sharing a communication line. SR 1.17 What is the etymology of the word Internet? SR 1.18 The TCP/IP set of protocols describes communication rules for software that uses the Internet. What does TCP stand for? What does IP stand for? SR 1.19 Explain the parts of the following URLs: a. duke.csc.villanova.edu/jss/examples.html b. java.sun.com/products/index.html 1.4 The Java Programming Language Let’s now turn our attention to the software that makes a computer system useful. A program is written in a particular programming language that uses specific words and symbols to express the problem solution. A programming language defines a set of rules that determines exactly how a programmer can combine the words and symbols of the language into programming statements, which are the instructions that are carried out when the program is executed. Since the inception of computers, many programming languages have been created. We use the Java language in this book to demonstrate various programming concepts and techniques. Although our main goal is to learn these underlying software development principles, an important side effect will be to become proficient in the development of Java programs specifically. The development of the Java programming language began in 1991 by James Gosling at Sun Microsystems. The language initially was called Oak, then Green, and ultimately Java. Java was introduced to the public in 1995 and has gained tremendous popularity since. In 2010, Sun Microsystems was purchased by Oracle. There are variations of the Java Platform, including the Standard Edition, which is the mainstream version of the language; the Enterprise Edition, which includes extra libraries to support large-scale system development; and the Micro Edition, which is specifically for developing software for portable devices such as cell phones. This book focuses on the Standard Edition. Furthermore, the Java Standard Edition has gone through several revisions over the years, extending its capabilities and making changes to certain aspects of it. The table below lists the versions and some of the new features. Note that they changed the numbering technique with version 5. Readers of this book should be using version 6 or later. Version Year New Features 1.0 1996 Initial deployment 1.1 1997 Inner classes 1.2 1998 Collections framework, Swing graphics 1.3 2000 Sound framework 1.4 2002 Assertions, XML support, regular expressions 5 2004 Generics, for-each loop, autoboxing, enumerations, annotations, variable-length parameter lists 6 2006 GUI improvements, various library updates 7 2011 Use of strings in a switch, other language changes 8 2014 JavaFX, lambda expressions, date and time API Some parts of early Java technologies have been deprecated, which means they are considered old-fashioned and should not be used. When it is important, we point out deprecated elements and discuss their preferred alternatives. One reason Java attracted some initial attention was because it was the first programming language to deliberately embrace the concept of writing programs (called applets) that can be executed using the Web. Since then, the techniques for creating a Web page that has dynamic, functional capabilities have expanded dramatically. Java is an object-oriented programming language. Objects are the fundamental elements that make up a program. The principles of object-oriented software development are the cornerstone of this book. We explore object-oriented programming concepts later in this chapter and throughout the rest of the book. Key Concept This book focuses on the principles of object- oriented programming. The Java language is accompanied by a library of extra software that we can use when developing programs. This software is referred to as the Java API, which stands for Application Programmer Interface, or simply the standard class library. The Java API provides the ability to create graphics, communicate over networks, and interact with databases, among many other features. The Java API is huge and quite versatile. We won’t be able to cover all aspects of the library, though we will explore several of them. Java is used in commercial environments all over the world. It is one of the fastest growing programming technologies of all time. So not only is it a good language in which to learn programming concepts, it is also a practical language that will serve you well in the future. A Java Program Let’s look at a simple but complete Java program. The program in Listing 1.1 prints two sentences to the screen. This particular program prints a quote by Abraham Lincoln. The output is shown below the program listing. Listing 1.1 / This comment type does not use the end of a line to indicate the end of the comment. Anything between the initiating slash-asterisk ( ) is part of the comment, including the invisible newline character that represents the end of a line. Therefore, this type of comment can extend over multiple lines. No space can be between the slash and the asterisk. If there is a second asterisk following the Programmers often concentrate so much on writing code that they focus too little on documentation. You should develop good commenting practices and follow them habitually. Comments should be well written, often in complete sentences. They should not belabor the obvious but should provide appropriate insight into the intent of the code. The following examples are not good comments: System.out.println("hello"); // prints hello System.out.println("test"); // change this later The first comment paraphrases the obvious purpose of the line and does not add any value to the statement. It is better to have no comment than a useless one. The second comment is ambiguous. What should be changed later? When is later? Why should it be changed? Key Concept Inline documentation should provide insight into your code. It should not be ambiguous or belabor the obvious. Identifiers and Reserved Words The various words used when writing programs are called identifiers. The identifiers in the Lincoln program are class , Lincoln , public , static , void , main , String , args , System , out , and println. These fall into three categories: words that we make up when writing a program ( Lincoln and args ) words that another programmer chose ( String , System , out , println , and main ) words that are reserved for special purposes in the language ( class , public , static , and void ) While writing the program, we simply chose to name the class Lincoln , but we could have used one of many other possibilities. For example, we could have called it Quote , or Abe , or GoodOne. The identifier args (which is short for arguments) is often used in the way we use it in Lincoln , but we could have used just about any other identifier in its place. The identifiers String , System , out , and println were chosen by other programmers. These words are not part of the Java language. They are part of the Java standard library of predefined code, a set of classes and methods that someone has already written for us. The authors of that code chose the identifiers in that code—we’re just making use of them. Reserved words are identifiers that have a special meaning in a programming language and can only be used in predefined ways. A reserved word cannot be used for any other purpose, such as naming a class or method. In the Lincoln program, the reserved words used are class , public , static , and void. Throughout the book, we show Java reserved words in blue type. Figure 1.18 lists all of the Java reserved words in alphabetical order. The words marked with an asterisk have been reserved, but currently have no meaning in Java. Figure 1.18 Java reserved words An identifier that we make up for use in a program can be composed of any combination of letters, digits, the underscore character ( _ ), and the dollar sign ( $ ), but it cannot begin with a digit. Identifiers may be of any length. Therefore, total , label7 , nextStockItem , NUM_BOXES , and $amount are all valid identifiers, but 4th_word and coin#value are not valid. Identifier An identifier is a letter followed by zero or more letters and digits. A Java Letter includes the 26 English alphabetic characters in both uppercase and lowercase, the $ and _ (underscore) characters, as well as alphabetic characters from other languages. A Java Digit includes the digits 0 through 9. Examples: total MAX_HEIGHT num1 Keyboard System Both uppercase and lowercase letters can be used in an identifier, and the difference is important. Java is case sensitive , which means that two identifier names that differ only in the case of their letters are considered to be different identifiers. Therefore, total , Total , ToTaL , and TOTAL are all different identifiers. As you can imagine, it is not a good idea to use multiple identifiers that differ only in their case, because they can be easily confused. Although the Java language doesn’t require it, using a consistent case format for each kind of identifier makes your identifiers easier to understand. There are various Java conventions regarding identifiers that should be followed, though technically they don’t have to be. For example, we use title case (uppercase for the first letter of each word) for class names. Throughout the text, we describe the preferred case style for each type of identifier when it is first encountered. Key Concept Java is case sensitive. The uppercase and lowercase versions of a letter are distinct. While an identifier can be of any length, you should choose your names carefully. They should be descriptive but not verbose. You should avoid meaningless names such as a or x. An exception to this rule can be made if the short name is actually descriptive, such as using x and y to represent (x, y) coordinates on a two-dimensional grid. Likewise, you should not use unnecessarily long names, such as the identifier theCurrentItemBeingProcessed. The name currentItem would serve just as well. As you might imagine, the use of identifiers that are verbose is a much less prevalent problem than the use of names that are not descriptive. You should always strive to make your programs as readable as possible. Therefore, you should always be careful when abbreviating words. You might think curStVal is a good name to represent the current stock value, but another person trying to understand the code may have trouble figuring out what you meant. It might not even be clear to you two months after writing it. Key Concept Identifier names should be descriptive and readable. White Space All Java programs use white space to separate the words and symbols used in a program. White space consists of blanks, tabs, and newline characters. The phrase “white space” refers to the fact that, on a white sheet of paper with black printing, the space between the words and symbols is white. The way a programmer uses white space is important because it can be used to emphasize parts of the code and can make a program easier to read. Key Concept Appropriate use of white space makes a program easier to read and understand. Except when it’s used to separate words, the computer ignores white space. It does not affect the execution of a program. This fact gives programmers a great deal of flexibility in how they format a program. The lines of a program should be divided in logical places, and certain lines should be indented and aligned so that the program’s underlying structure is clear. Because white space is ignored, we can write a program in many different ways. For example, taking white space to one extreme, we could put as many words as possible on each line. The code in Listing 1.2 , the Lincoln2 program, is formatted quite differently from Lincoln but prints the same message. Listing 1.2 //******************************************************************** // Lincoln2.java Author: Lewis/Loftus // // Demonstrates a poorly formatted, though valid, program. //******************************************************************** public class Lincoln2{public static void main(String[]args){ System.out.println("A quote by Abraham Lincoln:"); System.out.println("Whatever you are, be a good one.");}} Output A quote by Abraham Lincoln: Whatever you are, be a good one. Taking white space to the other extreme, we could write almost every word and symbol on a different line with varying amounts of spaces, such as Lincoln3 , shown in Listing 1.3. Listing 1.3 //******************************************************************** // Lincoln3.java Author: Lewis/Loftus // // Demonstrates another valid program that is poorly formatted. //******************************************************************** public class Lincoln3 { public static void main ( String [] args ) { System.out.println ( "A quote by Abraham Lincoln:" ) ; System.out.println ( "Whatever you are, be a good one." ) ; } } Output A quote by Abraham Lincoln: Whatever you are, be a good one. Key Concept You should adhere to a set of guidelines that establish the way you format and document your programs. All three versions of Lincoln are technically valid and will execute in the same way, but they are radically different from a reader’s point of view. Both the latter examples show poor style and make the program difficult to understand. You may be asked to adhere to particular guidelines when you write your programs. A software development company often has a programming style policy that it requires its programmers to follow. In any case, you should adopt and consistently use a set of style guidelines that increase the readability of your code. Self-Review Questions (see answers in Appendix L ) SR 1.20 When was the Java programming language developed? By whom? When was it introduced to the public? SR 1.21 Where does processing begin in a Java application? SR 1.22 What do you predict would be the result of the following line in a Java program? System.out.println("Hello"); // prints hello SR 1.23 What do you predict would be the result of the following line in a Java program? // prints hello System.out.println("Hello"); SR 1.24 Which of the following are not valid Java identifiers? Why? a. RESULT b. result c. 12345 d. x12345y e. black&white f. answer_7 SR 1.25 Suppose a program requires an identifier to represent the sum of the test scores of a class of students. For each of the following names, state whether or not each is a good name to use for the identifier. Explain your answers. a. x b. scoreSum c. sumOfTheTestScoresOfTheStudents d. smTstScr SR 1.26 What is white space? How does it affect program execution? How does it affect program readability? 1.5 Program Development The process of getting a program running involves various activities. The program has to be written in the appropriate programming la