EDP 101 - GROUP 10.pdf
Document Details
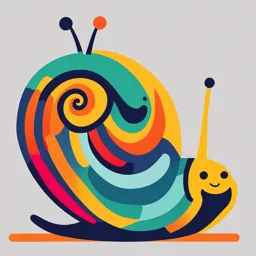
Uploaded by SteadyBixbite
Tags
Related
- C++ Programming Fundamentals Lecture 3 PDF
- Lecture 1: Introduction to C++ Programming and Computer Science PDF
- Programming Fundamentals: Lecture 1 PDF
- Computer Fundamentals and Programming Lecture Module 2 PDF
- C++ Programming Essentials Lecture 1 PDF
- Chapter 1: Introduction to Computers and Programming PDF
Full Transcript
PROGRAMMING FUNDAMENTALS PROGRAMMING w i t h C + + Presentation by Students #1 - #5 PROGRAMMING FUNDAMENTALS GET STARTED with C++ C++ is a general-purpose programming language that supports object-oriented programming. PROGRAMMING FUNDAMENT...
PROGRAMMING FUNDAMENTALS PROGRAMMING w i t h C + + Presentation by Students #1 - #5 PROGRAMMING FUNDAMENTALS GET STARTED with C++ C++ is a general-purpose programming language that supports object-oriented programming. PROGRAMMING FUNDAMENTALS THE LEVELS OF Programming Languages High-Level Assembly Machine Hardware Image 1. A simple “Hello World!” program using C++. PROGRAMMING FUNDAMENTALS FUNDAMENTALS Presentation by Students #1 - #5 PROGRAMMING FUNDAMENTALS PROGRAMMING FUNDAMENTALS Description stands for input/output stream PROGRAMMING FUNDAMENTALS Description and Function `` is a header file in C++ that provides the core input and output (I/O) functionality. It includes classes like `std::cin`, `std::cout`, `std::cerr`, and `std::clog` for handling standard input, output, error output, and logging, respectively. These classes are used for reading from the keyboard and writing to the console in a C++ program. Example. Usage of PROGRAMMING FUNDAMENTALS KEYWORDS KEYWORDS A keyword (sometimes called reserved word) is an identifier that is reserved by the C++ language and cannot be redefined by the programmer or the library of the language. We show in color the keywords in the text and in programs to distinguish them from other identifiers. PROGRAMMING FUNDAMENTALS Description sizeof operator The ‘sizeof’ operator in C++, a unary compile-time operator, returns the size, in bytes, of a data type or object. PROGRAMMING FUNDAMENTALS Function sizeof operator The ‘sizeof’ operator is used to determine the size of variables, data types, and constants in bytes at compile time. PROGRAMMING FUNDAMENTALS Syntax sizeof operator Example. Finding size of integer types PROGRAMMING FUNDAMENTALS Description namespace A namespace is a declarative region that provides a scope to group related identifiers, such as variables, functions, and classes, to avoid name conflicts. It helps organize code and prevents naming collisions, especially in large projects or when using multiple libraries. For example, the standard library is contained within the ‘std’ namespace. PROGRAMMING FUNDAMENTALS Function namespace The function of a namespace in C++ is to organize code and prevent naming conflicts by grouping related identifiers (like variables, functions, and classes) into distinct scopes. This allows the same names to be used in different contexts without collision. Example. C++ program structure PROGRAMMING FUNDAMENTALS Description return keyword In programming, the `return` keyword is used to exit a function and optionally pass a value back to the caller. PROGRAMMING FUNDAMENTALS Function return keyword The ‘return’ keyword finishes the execution of a method, and can be used to return a value from a method. Example. Usage of ‘return’ keyword PROGRAMMING FUNDAMENTALS Description and Function static cast This is the simplest type of cast that can be used. It is a compile-time cast. It does things like implicit conversions between types (such as int to float, or pointer to void*), and it can also call explicit conversion functions. Example. Usage of the static cast PROGRAMMING FUNDAMENTALS HEADER FILE/ PREPROCESSOR PROGRAMMING FUNDAMENTALS Description preprocessor A preprocessor directive is a command to the compiler to take some action before it compiles the program. PROGRAMMING FUNDAMENTALS Description header file These are those files that store predefined functions. It contains definitions of functions that you can include or import using a preprocessor directive include. This preprocessor directive tells the compiler that the header file needs to be processed prior to the compilation. PROGRAMMING FUNDAMENTALS Function header file Header files in C and C++ are used to declare functions, macros, constants, and types that can be shared across multiple source files. They help in organizing code and promoting modularity. By including a header file in a source file, you can use the declarations and definitions contained in the header file without having to rewrite them. Example. A preprocessor directive PROGRAMMING FUNDAMENTALS MANIPULATORS WITH ARGUMENTS PROGRAMMING FUNDAMENTALS Description and Function setprecision() The ‘setprecision()’ is used only for fixed (not scientific) floating-point values. The integer inside the parentheses (n) defines the number of digits after the decimal point. PROGRAMMING FUNDAMENTALS Description and Function setprecision() By using the ‘setprecision()’, we can get the desired precise value of a floating-point or a double value by providing the exact number of decimal places. If an argument n is passed to the ‘setprecision()’ function, then it will give n significant digits of the number without losing any information. Example. Usage of ‘setprecision()’ PROGRAMMING FUNDAMENTALS Description and Function setw(n) This manipulator is used to define the size of the field that we want our value to occupy. Note that this manipulator does not change the state of the stream. It needs to be set for each value individually. Example. Usage of ‘setw()’ PROGRAMMING FUNDAMENTALS Description and Function setfill(ch) This manipulator shows how we can fill the field with padding if the actual size of our value is less than the size defined by setw (n). The argument inside parentheses is a literal character used as padding. The manipulators left, internal, and right that we discussed before can then determine where the padding is located. Example. Usage of ‘setfill()’ PROGRAMMING FUNDAMENTALS NO-ARGUMENT MANIPULATORS PROGRAMMING FUNDAMENTALS Description and Function showpoint The showpoint function is a manipulator that sets the showpoint flag, which tells an output stream to write a decimal point for floating-point output, even if the point is unnecessary. Example. Usage of the showpoint manipulator PROGRAMMING FUNDAMENTALS Description and Function fixed format There are two ways to show a floating-point type value: fixed or scientific. PROGRAMMING FUNDAMENTALS Description and Function fixed format In the fixed format, a floating-point value is shown as an integer part and the fraction part separated by a decimal point such as (ddd.ddd) in which each d is a digit. The number is preceded by a minus sign if it iss a negative. Example. Usage of the fixed format PROGRAMMING FUNDAMENTALS Description and Function adjusting numbers in a field PROGRAMMING FUNDAMENTALS Description and Function adjusting numbers in a field In the left format (default), the number and sign are located at the left of the field and the rest of field is filled with padding (as we explain shortly). In the internal format, the sign occupies the leftmost part of the field, the number occupies the rightmost parts, and the remaining part is filled with padding. In the right format, the sign and the number occupy the rightmost part and the left part is filled with padding. PROGRAMMING FUNDAMENTALS LINKER PROGRAMMING FUNDAMENTALS Description and Function linker In computing, a linker or link editor is a computer system program that takes one or more object files (generated by a compiler or an assembler) and combines them into a single executable file, library file, or another "object" file. Example. Usage of ‘return’ keyword PROGRAMMING FUNDAMENTALS COMPILER & INTERPRETER PROGRAMMING FUNDAMENTALS Description and Function compiler A compiler is a program that converts an entire program from high level source code into some lower level representation (assembly or machine code for example). Example. Writing, editing, and executing a program PROGRAMMING FUNDAMENTALS Description and Function interpreter An interpreter is a program that directly executes the instructions in a high-level language, without converting it into machine code. It reads and executes the source code of a program in real-time. Instead of translating the entire program into machine code at once (as a compiler does), it processes the code incrementally. Example. Compiler vs. Interpreter PROGRAMMING FUNDAMENTALS DEBUGGER PROGRAMMING FUNDAMENTALS Description and Function debugger A debugger is a crucial tool in programming that helps developers identify, diagnose, and fix issues or "bugs" in their code. It provides various functionalities to inspect the state of a program, control its execution, and analyze its behavior. PROGRAMMING FUNDAMENTALS SYNTAX & SYMANTIC ERRORS PROGRAMMING FUNDAMENTALS Description and Function syntax errors Syntax errors occur when the code violates the rules of the programming language. These errors are typically detected by the compiler or interpreter when the code is being parsed. Syntax errors must be corrected before the code can be successfully compiled or executed. Example. Syntax error PROGRAMMING FUNDAMENTALS Description and Function symantic errors Semantic errors occur when the code is syntactically correct but does not do what the programmer intended. These errors are related to the logic of the code, and they do not prevent the program from running, but they lead to incorrect results. Example. Symantic error PROGRAMMING FUNDAMENTALS COMMENTS PROGRAMMING FUNDAMENTALS Description and Function comments Programmers use comments to annotate code and clarify its purpose, making the code easier to read and maintain. PROGRAMMING FUNDAMENTALS Description and Function comments Comments in programming serve a function for explaining the purpose of particular areas of the code, explaining what the code does, and aiding identify problems during debugging. PROGRAMMING FUNDAMENTALS Description and Function comments There are usually two syntactic ways to comment. The first is called a single line comment and, as implied, only applies to a single line in the "source code" (the program). The second is called a block comment usually refers to a paragraph of text. SYNTACTIC WAYS to comment Single Line Block Starts with two forward (Multi-line) Starts with. The purpose of this is to To describe larger provide a short sections of code or explanation of a particular provide detailed line or section of code. documentation. Example. Single line comments Example. Block comments PROGRAMMING FUNDAMENTALS PROGRAMMING FUNDAMENTALS Description The is a library in C++ which helps us in manipulating the output of any C++ program. There are many functions in this library that help in manipulating the output. FUNCTIONS OF std::setw(int n): Sets the width of the next input/output field. std::setfill(char c): Sets the fill character used to pad the output field. std::setprecision(int n): Sets the number of decimal places for floating-point numbers. std::fixed: Forces floating-point numbers to be displayed in fixed-point notation. std::scientific: Forces floating-point numbers to be displayed in scientific notation. FUNCTIONS OF std::hex: Sets integer output to hexadecimal format. std::dec: Sets integer output to decimal format (default). std::oct: Sets integer output to octal format. std::left: Left-aligns the output within the field. std::right: Right-aligns the output within the field. Example. Usage of SALFORD & CO. MEET OUR TEAM ABDULHALIM, SHERIFA SACOR I. ABELGAS, RICHMON LAWRENCE AKUT, VANNE JOSEPH 1st Year - BSCE 1st Year - BSCE 1st Year - BSCE SALFORD & CO. MEET OUR TEAM ALBUERA, APRILLE NICOLE ARZAGA, JONIEL 1st Year - BSCE 1st Year - BSCE PROGRAMMING FUNDAMENTALS THANK YOU Presentation by Students #1 - #5