OOP - JAVA Object-Oriented Programming PDF
Document Details
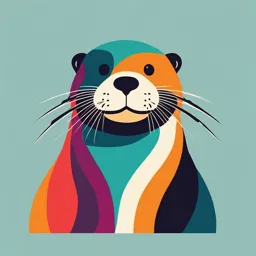
Uploaded by SmoothestRationality9460
Sapientia Hungarian University of Transylvania
2024
Margit Antal
Tags
Summary
This document is a set of lecture notes on Object-Oriented Programming (OOP) in Java. The topics covered include fundamental concepts like objects, classes, and methods. It also includes examples and explanations for several Java features.
Full Transcript
OOP - JAVA Object-Oriented Programming Java Margit ANTAL Sapientia Hungarian University of Transylvania 2024 Margit Antal Goals...
OOP - JAVA Object-Oriented Programming Java Margit ANTAL Sapientia Hungarian University of Transylvania 2024 Margit Antal Goals 1. Java Language 2. Objects and classes 3. Static Members 4. Relationships between classes 5. Inheritance and Polymorphism 6. Interfaces and Abstract Classes 7. Exceptions 8. Nested Classes 9. Threads 10. GUI Programming 11. Collections and Generics 12. Serialization Margit Antal Module 1 Java language Margit Antal Java language History Java technology: JDK, JRE, JVM Properties Hello world application Garbage Collection Margit Antal Short History 1991 - Green Project for consumer electronics market (Oak language → Java) 1994 – HotJava Web browser 1995 – Sun announces Java 1996 – JDK 1.0 1997 – JDK 1.1 RMI, AWT, Servlets 1998 – Java 1.2 Reflection, Swing, Collections 2004 – J2SE 1.5 (Java 5) Generics, enums 2014 – Java SE 8 Lambdas - functional programming Margit Antal Short History 2017 - Java SE 9 2018 - Java SE 10, Java SE 11 2019 - Java SE 12, Java SE 13 2020 - Java SE 14, Java SE 15 2021 - Java SE 16, Java SE 17 2022 - Java SE 18, Java SE 19 2023 - Java SE 20 2024 - Java SE 21 Margit Antal Java technology JDK – Java Development Kit JRE – Java Runtime Environment JVM – Java Virtual Machine JDK javac, jar, debugging JRE java, libraries JVM Margit Antal Properties Object-oriented Interpreted Portable Secure and robust Scalable Multi-threaded Dynamic capabilities (reflection) Distributed Margit Antal Hello World Application 1. Write the source code: HelloWorld.java public class HelloWorld{ public static void main( String args[] ){ System.out.println(“Hello world”); } } 2. Compile: javac HelloWorld.java 3. Run: java HelloWorld Margit Antal Hello World Application HelloWorld.java bytecode javac HelloWorld.java HelloWorld.class java HelloWorld Runtime JVM Margit Antal Garbage Collection Dynamically allocated memory Deallocation Programmer's responsibility (C/C++) System responsibility (Java): ○ Is done automatically (system-level thread) ○ Checks for and frees memory no longer needed Margit Antal Remember JVM, JRE, JDK Compilers vs. interpreters Bytecode Garbage collection Portability Margit Antal Module 2 Object-Oriented Programming Margit Antal Object-oriented programming Classes and Objects Class Attributes and methods Object (instance) Information hiding Encapsulation Constructors Packages Margit Antal Class Is a user-defined type − Describes the data (attributes) − Defines the behavior (methods) } members Instances of a class are objects Margit Antal Declaring Classes Syntax * class { * * * } Example public class Counter { private int value; public void inc(){ ++value; } public int getValue(){ return value; } } Margit Antal Declaring Attributes Syntax * [= ]; Examples public class Foo { private int x; private float f = 0.0; private String name =”Anonymous”; } Margit Antal Declaring Methods Syntax * ( * ){ * } Examples public class Counter { public static final int MAX = 100; private int value; public void inc(){ if( value < MAX ){ ++value; } } public int getValue(){ return value; } } Margit Antal Accessing Object Members Syntax. Examples public class Counter { Counter c = new Counter(); public static final int MAX = 100; c.inc(); private int value = 0; int i = c.getValue(); public void inc(){ if( value < MAX ){ ++value; } } public int getValue(){ return value; } } Margit Antal Information Hiding The problem: Client code has direct access to internal data struct Date { Date d; int year, month, day; d.day = 32; //invalid day }; d.month = 2; d.day = 30; // invalid data d.day = d.day + 1; // no check Margit Antal Information Hiding The solution: Client code must use setters and getters to access internal data // Java language public class Date { Date d = new Date(); private int year, month, day; // no assignment public void setDay(int d){..} d.setDay(32); public void setMonth(int m){..} // month is set public void setYear(int y){..} d.setMonth(2); public int getDay(){...} // no assignment public int getMonth(){...} d.day = 30; public int getYear(){...} } Verify days in month Margit Antal Encapsulation Bundling of data with the methods that operate on that data (restricting of direct access to some of an object's components) Hides the implementation details of a class Forces the user to use an interface to access data Makes the code more maintainable Margit Antal UML - Graphical Class Representation class name Person “-” means -firstName: String state private access “+” means +getfirstName(): String behaviour public access Margit Antal Declaring Constructors Syntax: []( *){ * } public class Date { private int year, month, day; public Date( int y, int m, int d) { if( verify(y, m, d) ){ year = y; month = m; day = d; } } private boolean verify(int y, int m, int d){ //... } } Margit Antal Constructors Role: object initialization Name of the constructor must be the same as that of class name. Must not have return type. Every class should have at least one constructor. ○ If you don't write constructor, compiler will generate the default constructor. Constructors are usually declared public. ○ Constructor can be declared as private → You can't use it outside the class. One class can have more than one constructors. ○ Constructor overloading. Margit Antal The Default Constructors There is always at least one constructor in every class. If the programmer does not supply any constructors, the default constructor is generated by the compiler ○ The default constructor takes no argument ○ The default constructor's body is empty public class Date { private int year, month, day; default public Date( ){ constructor } } Margit Antal Objects Objects are instances of classes Are allocated on the heap by using the new operator Constructor is invoked automatically on the new object Counter c = new Counter(); Date d1 = new Date( 2016, 9, 23); Person p = new Person(“John”,”Smith”); Margit Antal Packages Help manage large software systems Contain ○ Classes ○ Sub-packages java lang awt Math Graphics String Button Thread Margit Antal The package statement Syntax: package [.]*; Examples: package java.lang; - statement at the beginning of the source file public class String{ - only one package declaration per //... source file } - if no package name is declared → the class is placed into the default package Margit Antal The import statement Syntax: package [.]*; Usage: import [.]*.*; Examples: import java.util.List; -precedes all class declarations import java.io.*; -tells the compiler where to find classes Margit Antal Remember Class, encapsulation Class members: ○ attributes ○ methods Object, instance Constructor Package Import statement Margit Antal Object-oriented programming Types Primitive types Reference Type Parameter Passing The this reference Variables and Scope Casting Margit Antal Java Types − Primitive (8) Logical: boolean Textual: char Integral: byte, short, int, long Floating: double, float − Reference All others Margit Antal Logical - boolean − Characteristics: Literals: − true − false Examples: − boolean cont = true; − boolean exists = false; Margit Antal Textual - char − Characteristics: Represents a 16-bit Unicode character Literals are enclosed in single quotes (' ') Examples: − 'a' - the letter a − '\t' - the TAB character − '\u0041' - a specific Unicode character ('A') represented by 4 hexadecimal digits Margit Antal Integral – byte, short, int, and long − Characteristics: Use three forms: − Decimal: 67 − Octal: 0103 (1x8^2+0x8^1+3x8^0) − Hexadecimal: 0x43 Default type of literal is int. Literals with the L or l suffix are of type long. Margit Antal Integral – byte, short, int, and long − Ranges: Type Length Range byte 1 byte -27..27-1 short 2 byte -215..215-1 int 4 byte -231..231-1 long 8 byte -263..263-1 Margit Antal Floating Point – float and double − Characteristics: Size: − float – 4 byte − double – 8 byte Decimal point − 9.65 (double, default type) − 9.65f or 9.65F (float) − 9.65D or 9.65d (double) Exponential notation − 3.41E20 (double) Margit Antal Java Reference Types public class MyDate { private int day = 26; private int month = 9; private int year = 2016; public MyDate( int day, int month, int year){... } } MyDate date1 = new MyDate(20, 6, 2000); Margit Antal Constructing and Initializing Objects MyDate date1 = new MyDate(20, 6, 2000); Margit Antal Constructing and Initializing Objects MyDate date1 = new MyDate(20, 6, 2000); new MyDate(20, 6, 2000); 1) Memory is allocated for the object 2) Explicit attribute initialization is performed 3) A constructor is executed 4) The object reference is returned by the new operator Margit Antal Constructing and Initializing Objects MyDate date1 = new MyDate(20, 6, 2000); new MyDate(20, 6, 2000); 1) Memory is allocated for the object 2) Explicit attribute initialization is performed 3) A constructor is executed 4) The object reference is returned by the new operator date1 = object reference 5) The reference is assigned to a variable Margit Antal (1) Memory is allocated for the object MyDate date1 = new MyDate(20, 6, 2000); reference date1 ??? Implicit initialization object day 0 month 0 year 0 Margit Antal (2) Explicit Attribute Initialization MyDate date1 = new MyDate(20, 6, 2000); reference date1 ??? public class MyDate{ private int day = 26; private int month = 9; private int year = 2016; object day 26 } month 9 year 2016 Margit Antal (3) Executing the constructor MyDate date1 = new MyDate(20, 6, 2000); reference date1 ??? public class MyDate{ private int day = 26; private int month = 9; private int year = 2016; object day 20 } month 6 year 2000 Margit Antal (4) The object reference is returned MyDate date1 = new MyDate(20, 6, 2000); The address of the object reference date1 ??? object day 20 month 6 0x01a2345 year 2000 Margit Antal (5) The reference is assigned to a variable MyDate date1 = new MyDate(20, 6, 2000); The reference points to the object reference date1 0x01a2345 0x01a2345 object day 20 month 6 year 2000 Margit Antal Assigning References Two variables refer to a single object MyDate date1 = new MyDate(20, 6, 2000); MyDate date2 = date1; date1 0x01a2345 date2 0x01a2345 0x01a2345 day 20 object month 6 year 2000 Margit Antal Parameter Passing Pass-by-Value public class PassTest{ public void changePrimitive(int value){ ++value; } public void changeReference(MyDate from, MyDate to){ from = to; } public void changeObjectDay(MyDate date, int day){ date.setDay( day ); } } Margit Antal Parameter Passing Pass-by-Value PassTest pt = new PassTest(); int x = 100; pt.changePrimitive( x ); System.out.println( x ); MyDate oneDate = new MyDate(3, 10, 2016); MyDate anotherDate = new MyDate(3, 10, 2001); pt.changeReference( oneDate, anotherDate ); System.out.println( oneDate.getYear() ); pt.changeObjectDay( oneDate, 12 ); System.out.println( oneDate.getDay() ); Output: 100 2016 12 Margit Antal The this Reference Usage: − To resolve ambiguity between instance variables and parameters − To pass the current object as a parameter to another method Margit Antal The this Reference public class MyDate{ private int day = 26; private int month = 9; private int year = 2016; public MyDate( int day, int month, int year){ this.day = day; this.month = month; this.year = year; } public MyDate( MyDate date){ this.day = date.day; this.month = date.month; this.year = date.year; } public MyDate creteNextDate(int moreDays){ MyDate newDate = new MyDate(this); //... add moreDays return newDate; } } Margit Antal Java Coding Conventions Packages ○ ro.sapientia.ms Classes ○ SavingsAccount Methods ○ getAmount() Variables ○ amount Constants ○ NUM_CLIENTS Margit Antal Variables and Scope Local variables are Defined inside a method Created when the method is executed and destroyed when the method is exited Not initialized automatically Created on the execution stack Margit Antal Margit Antal Default Initialization Default values for attributes: Type Value byte 0 short 0 int 0 long 0L float 0.0f double 0.0d char '\u0000' boolean false refrence null Margit Antal Operators Logical operators Bitwise operators ( ~, ^, &, |, >>, >>>, { final Point origo = new Point(0,0); return Double.compare(p1.distanceTo(origo), p2.distanceTo(origo)); } ); for (Point point : points) { System.out.println(point); } Margit Antal Module 7 Exceptions Margit Antal Exceptions Define exceptions Exception handling: try, catch, and finally Throw exceptions: throw, throws Exception categories User-defined exceptions Margit Antal Exception Example public class AddArguments { public static void main(String[] args) { int sum = 0; for( String arg: args ){ sum += Integer.parseInt( arg ); } System.out.println( "Sum: "+sum ); } } java AddArguments 1 2 3 Sum: 6 java AddArguments 1 foo 2 3 Exception in thread "main" java.lang.NumberFormatException: For input string: "foo" at java.lang.NumberFormatException.forInputString(NumberFormatException.java:65) at java.lang.Integer.parseInt(Integer.java:580) at java.lang.Integer.parseInt(Integer.java:615) at addarguments.AddArguments.main(AddArguments.java:line_number) Java Result: 1 Margit Antal The try-catch statement public class AddArguments2 { public static void main(String[] args) { try{ int sum = 0; for( String arg: args ){ sum += Integer.parseInt( arg ); } System.out.println( "Sum: "+sum ); } catch( NumberFormatException e ){ System.err.println(“Non-numeric argument”); } } } java AddArguments2 1 foo 2 3 Non-numeric argument Margit Antal The try-catch statement public class AddArguments3 { public static void main(String[] args) { int sum = 0; for( String arg: args ){ try{ sum += Integer.parseInt( arg ); } catch( NumberFormatException e ){ System.err.println(arg+”is not an integer”); } } System.out.println( "Sum: "+sum ); } } java AddArguments3 1 foo 2 3 foo is not an integer Sum: 6 Margit Antal The try-catch statement try{ // critical code block // code that might throw exceptions } catch( MyException1 e1 ){ // code to execute if a MyException1 is thrown } catch( MyException2 e2 ){ // code to execute if a MyException2 is thrown } catch ( Exception e3 ){ // code to execute if any other exception is thrown } finally{ // code always executed } Margit Antal Call Stack Mechanism If an exception is not handled in a method, it is thrown to the caller of that method If the exception gets back to the main method and is not handled there, the program is terminated abnormally. Margit Antal Closing resources The finally clause try{ connectDB(); doTheWork(); } catch( AnyException e ){ logProblem( e ); } finally { disconnectDB(); } The code in the finally block is always executed (even in case of return statement) Margit Antal Closing resources The try-with-resources Statement The try-with-resources statement ensures that each resource is closed at the end of the statement. static String readFirstLineFromFile(String path){ try (BufferedReader br = new BufferedReader(new FileReader(path))) { return br.readLine(); } catch(Exception e) { // exception handling } } Margit Antal Exception Categories Checked and unchecked exceptions Margit Antal The Handle or Declare Rule public static int countLines( String filename ){ int counter = 0; try (Scanner scanner = new Scanner(new File(filename))){ while (scanner.hasNextLine()) { scanner.nextLine(); ++counter; } }catch(FileNotFoundException e){ e.printStackTrace(); HANDLE } return counter; } Usage: System.out.println(ClassName.countLines(“input.txt”)); Margit Antal The Handle or Declare Rule public static int countLines(String filename) throws FileNotFoundException { try (Scanner scanner = new Scanner(new File(filename))) { int counter = 0; while (scanner.hasNextLine()) { scanner.nextLine(); ++counter; } DECLARE return counter; throws } } Usage: try{ System.out.println(ClassName.countLines(“input.txt”)); } catch( FileNotFoundException e ){ e.printStackTrace(); } Margit Antal The throws Clause void trouble1 () throws Exception1 {...} void trouble2 () throws Exception1, Exception2 {...} Principles: You do not need to declare runtime (unchecked) exceptions You can choose to handle runtime exceptions (e.g. IndexArrayOutOfBounds, NullPointerException) Margit Antal Creating Your Own Exceptions The overriding method can throw: No exceptions One or more of the exceptions thrown by the overridden method One or more subclasses of the exceptions thrown by the overridden method The overridden method cannot throw: Additional exceptions not thrown by the overridden method Superclasses of the exceptions thrown by the overridden method Margit Antal User-Defined Exception public class StackException extends Exception { public StackException(String message) { super( message ); } } Margit Antal User-Defined Exception public class Stack { private Object elements[]; private int capacity; private int size; public Stack( int capacity ){ this.capacity = capacity; elements = new Object[ capacity ]; } public void push(Object o) throws StackException { if (size == capacity) { throw new StackException("Stack is full"); } elements[size++] = o; } public Object top() throws StackException { if (size == 0) { throw new StackException("Stack is empty"); } return elements[size - 1]; } //... } Margit Antal User-Defined Exception Stack s = new Stack(3); for (int i = 0; i < 10; ++i) { try { s.push(i); } catch (StackException ex) { ex.printStackTrace(); } } Margit Antal Best practices to handle exceptions Clean up resources in a finally block or use a try-with-resource statement Prefer specific exceptions Don't ignore exceptions Don't log and throw. Instead, wrap the exception without consuming it Catch early, handle late Source: 9 Best Practices to Handle Exceptions in Java Margit Antal Module 8 Nested Classes Margit Antal Nested Classes When? − If a class is used only inside of another class (encapsulation) − Helper classes Margit Antal Nested Classes The place of nesting − Class − Method − Instruction Embedding method − Static − Non-static Margit Antal Static Nested Class public class Slist{ private Element head; public void insertFirst( Object value ){ head = new Element(value, head); } Used only inside private static class Element{ the Slist class private Object value; private Element next; public Element( Object value, Element next){ this.value = value; this.next = next; } public Element( Object value){ this.value = value; this.next = null; } } } Margit Antal The Iterator interface Package: java.util public interface Iterator{ public boolean hasNext(); public Object next(); //optional public void remove(); } Make Slist iterable using the Iterator interface!!! Margit Antal The Iterator interface Slist list = new Slist(); for( int i=0; i