MATLAB Programming for Engineers PDF
Document Details
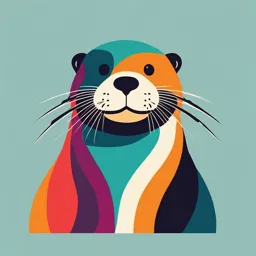
Uploaded by SoulfulBauhaus
Tomorrow University
Tags
Related
Summary
This document is a set of MATLAB programming problems and solutions, focusing on programming basics, matrices, arrays and plotting. Includes questions and examples for programming practices.
Full Transcript
Programming for Engineers Module – 1 || Lab – 1&2 Introduction to Programming Basics Faculty: Dr. Rupendra Kumar Pachauri Module-1: Lab-1 session on Introduction to Programming Basics More Practice Questions: Basic Operations: How do you create a variable in MATLAB? Write...
Programming for Engineers Module – 1 || Lab – 1&2 Introduction to Programming Basics Faculty: Dr. Rupendra Kumar Pachauri Module-1: Lab-1 session on Introduction to Programming Basics More Practice Questions: Basic Operations: How do you create a variable in MATLAB? Write a command to perform element-wise multiplication of two vectors A and B. What is the difference between.* and * in MATLAB? Matrices and Arrays: How do you create a 3x3 identity matrix? Write a command to concatenate two matrices A and B vertically. How can you access the element in the second row, third column of a matrix M? Functions and Scripts: What is the difference between a script and a function in MATLAB? Write a simple function that takes two inputs and returns their sum. How do you call a function named myFunction with inputs 3 and 5? Plotting: How do you create a basic plot of a sine wave? Write commands to label the x-axis as "Time" and the y-axis as "Amplitude". How can you add a legend to a plot? More Practice Questions: Control Flow: How do you write a for loop that iterates from 1 to 10? Write an if-else statement that checks if a variable x is positive, negative, or zero. How do you use a while loop to repeatedly prompt a user for input until they enter a negative number? File Input/Output: How do you read data from a file named data.txt? Write a command to save a variable A to a file named output.mat. How can you append new data to an existing text file? Advanced Topics (optional): How do you create a handle to a function in MATLAB? What is the purpose of the profiler in MATLAB? Write a command to perform element-wise logical AND operation between two logical arrays. Practice Questions 1. Introduction to Basics of Computer Programming and Terminologies Problem-1: Design a program that calculates the resistance of a series circuit consisting of three resistors with given values. Take the values of the resistors as input from the user and output the total resistance. Solution: R1 = input('Enter the value of R1: '); % Take input for R1 R2 = input('Enter the value of R2: '); % Take input for R2 R3 = input('Enter the value of R3: '); % Take input for R3 Rtotal = R1 + R2 + R3; % Calculate total resistance fprintf('The total resistance is: %.2f ohms\n', Rtotal); Problem 2: Create a MATLAB function that converts a temperature from Celsius to Fahrenheit. Solution: function F = celsiusToFahrenheit(C) % Convert Celsius to Fahrenheit F = (C * 9/5) + 32; end % Example usage: C = input('Enter temperature in Celsius: '); F = celsiusToFahrenheit(C); fprintf('Temperature in Fahrenheit: %.2f\n', F); 2. Getting Started with MATLAB Interface and Workspace Problem 3: Write a MATLAB script to calculate and display the kinetic energy of a moving object given its mass and velocity. Solution: mass = input('Enter the mass of the object (in kg): '); % Mass input velocity = input('Enter the velocity of the object (in m/s): '); % Velocity input kinetic_energy = 0.5 * mass * velocity^2; % Calculate kinetic energy fprintf('The kinetic energy of the object is: %.2f J\n', kinetic_energy); % Display result Problem 4: Create a MATLAB script to plot the trajectory of a projectile given initial velocity and angle. Solution: g = 9.81; % Acceleration due to gravity (m/s^2) t = linspace(0, t_flight, 100); % Trajectory v0 = input('Enter the initial velocity (in m/s): '); % Initial velocity equations theta = input('Enter the launch angle (in degrees): '); x = v0 * cos(theta_rad) * t; % Launch angle y = v0 * sin(theta_rad) * t - 0.5 * g * t.^2; % Plot trajectory figure; % Convert angle to radians plot(x, y); theta_rad = deg2rad(theta); % Time of flight xlabel('Distance (m)'); ylabel('Height (m)'); t_flight = (2 * v0 * sin(theta_rad)) / g; % Time intervals title('Projectile Trajectory'); grid on; 3. Data Types, Variables, Operators, and Expressions Problem 5: Write a MATLAB script that calculates the roots of a quadratic equation ax2+bx+c=0ax^2 + bx + c = 0ax2+bx+c=0. The coefficients aaa, bbb, and ccc should be provided by the user. Solution: a = input('Enter the coefficient a: '); % Coefficient a b = input('Enter the coefficient b: '); % Coefficient b c = input('Enter the coefficient c: '); % Coefficient c root1 = (-b + sqrt(D)) / (2*a); % Calculate discriminant root2 = (-b - sqrt(D)) / (2*a); fprintf('The roots are: %.2f and %.2f\n', root1, root2); D = b^2 - 4*a*c; elseif D == 0 root = -b / (2*a); % Calculate roots fprintf('The root is: %.2f\n', root); if D > 0 else fprintf('The equation has no real roots.\n'); end Problem 6: Design a MATLAB script to convert a given time in hours, minutes, and seconds into total seconds. Solution: hours = input('Enter hours: '); % Hours input minutes = input('Enter minutes: '); % Minutes input seconds = input('Enter seconds: '); % Seconds input % Calculate total seconds total_seconds = hours * 3600 + minutes * 60 + seconds; fprintf('Total time in seconds: %d seconds\n', total_seconds); 4. Scripting and Debugging Techniques Problem-7: Write a MATLAB script that uses a loop to calculate the factorial of a given number. Include error checking to ensure the user inputs a non-negative integer. Solution: n = input('Enter a non-negative integer: '); % Error checking if n < 0 || mod(n, 1) ~= 0 error('Input must be a non-negative integer.'); end % Initialize factorial fact = 1; % Loop to calculate factorial for i = 2:n fact = fact * i; end fprintf('Factorial of %d is %d\n', n, fact); Problem 8: Debug the following MATLAB script that aims to calculate the sum of the first 10 natural numbers but contains an error: sum = 0; for i = 1:10 summation = summation + i; end disp(sum) Solution: % Corrected script sum = 0; for i = 1:10 sum = sum + i; % Corrected the variable name from 'summation' to 'sum' end disp(sum) % Display the result 5. Matrix Operations, Indexing, and Slicing Problem 9: Create a MATLAB script to multiply two matrices AAA and BBB of compatible dimensions and display the result. Solution: A = [1, 2; 3, 4]; % Define matrix A B = [5, 6; 7, 8]; % Define matrix B % Multiply matrices C = A * B; % Display the result disp('The result of matrix multiplication is:'); disp(C); Problem-10: Write a MATLAB script to find the inverse of a given 3x3 matrix A. Ensure that the matrix is invertible before attempting to find the inverse. Solution: A = [2, 1, 3; 0, 1, 4; 5, 6, 0]; % Define matrix A % Check if the matrix is invertible if det(A) ~= 0 A_inv = inv(A); % Calculate inverse disp('The inverse of matrix A is:'); disp(A_inv); else disp('Matrix A is not invertible.'); end 6. Vector Calculus and Applications Problem-11: Calculate the divergence of the vector field, F(x,y,z)=(x2,y2,z2)\mathbf{F}(x, y, z) = (x^2, y^2, z^2)F(x,y,z)=(x2,y2,z2) using MATLAB. Solution: syms x y z F = [x^2, y^2, z^2]; % Define the vector field % Calculate divergence div_F = divergence(F, [x, y, z]); disp('The divergence of the vector field is:'); disp(div_F); Problem-12: Write a MATLAB script to visualize the gradient of the scalar function f(x,y)=x2+y2f(x, y) = x^2 + y^2f(x,y)=x2+y2. Solution: [X, Y] = meshgrid(-5:0.5:5, -5:0.5:5); % Define the grid Z = X.^2 + Y.^2; % Define the scalar function % Calculate the gradient [dx, dy] = gradient(Z, 0.5, 0.5); % Plot the scalar field and gradient contour(X, Y, Z); hold on; quiver(X, Y, dx, dy); title('Gradient of the scalar function f(x, y) = x^2 + y^2'); xlabel('x-axis'); ylabel('y-axis'); grid on; hold off; Problem: Designing a 9x9 Sudoku matrix in MATLAB involves creating a grid that adheres to the rules of Su-Do-Ku: Each row must contain numbers 1-9 with no repetition. Each column must contain numbers 1-9 with no repetition. Each 3x3 sub-grid must contain numbers 1-9 with no repetition. Solution: function sudoku_matrix = generate_sudoku() % Initialize an empty 9x9 matrix sudoku_matrix = zeros(9, 9); % Generate the first row with numbers 1-9 shuffled sudoku_matrix(1, :) = randperm(9); % Fill in the rest of the grid following Sudoku rules for row = 2:9 sudoku_matrix(row, :) = shift_row(sudoku_matrix(row-1, :), row); end % Display the generated Sudoku grid disp('Generated Sudoku Grid:'); disp(sudoku_matrix); end function shifted_row = shift_row(previous_row, row) % Function to shift a row to the right by a specific number of columns % The number of shifts depends on the row index shift_value = mod(row-1, 3)*3 + 1; shifted_row = circshift(previous_row, [0, shift_value]); end Thank You