Introduction to MATLAB Plotting PDF
Document Details
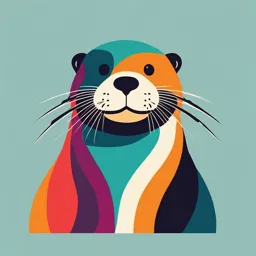
Uploaded by FeatureRichCedar
Dr. Nur ad-Din M. Salem
Tags
Summary
This document provides an introduction to plotting in MATLAB. It explains the basic plot command, including how to create plots of *y* versus *x*, along with options to adjust lines and markers. Several examples are included, illustrating different types of plots and how formatting commands like xlabel, ylabel, and title can be used to improve the appearance and readability of graphs.
Full Transcript
# Introduction to Computer and Programming ## CH.3 Introduction to MatLab The arguments *x* and *y* are each a vector (one-dimensional array). Both vectors must have the same number of elements. When the *plot* command is executed, a figure appears in the **Figure Window**, which opens automatic...
# Introduction to Computer and Programming ## CH.3 Introduction to MatLab The arguments *x* and *y* are each a vector (one-dimensional array). Both vectors must have the same number of elements. When the *plot* command is executed, a figure appears in the **Figure Window**, which opens automatically. The figure has a single curve with the *x* values on the horizontal axis (independent axis) and the *y* values on the vertical axis (dependent axis). The curve is constructed of straight line segments that connect the points whose coordinates are defined by the elements of the vectors *x* and *y*. The vector that is typed first in the *plot* command is used for the horizontal axis, and the vector that is typed second is used for the vertical axis. For example, if a vector *x* has the elements 0, 1, 2, 4, 7, 5, 8, 8, 9, 10, and a vector *y* has the elements 8, 9, 7, 6, 6, 2, 3, 8, 5, 9, a simple plot of *y* versus *x* can be produced by typing the following in the **Command Window**: ``` >> x = [0 1 2 4 7 5 8 8 9 10]; >> y = [8 9 7 6 6 2 3 8 5 9]; >> plot (x, y) ``` Once the *plot* command is executed, the plot that is shown in Fig. 3.5 is displayed in the **Figure Window**. **Fig. 3.5: A simple example of using the *plot* command** An image of a plot with *x* values on the horizontal axis ranging from 0 to 10 and *y* values on the vertical axis ranging from 0 to 9. The line plotted connects the points (0, 8), (1, 9), (2, 7), (4, 6), (7,6), (5, 2), (8, 3), (8, 8 ), (9, 5), (10, 9). Other example; to plot the function *y* = 2*x*³ for 0 ≤ *x* ≤ 1, *x* should be in the range from 0 to 1. For each value of *x*, the corresponding *y* is calculated from *y* = 2*x*³. Once by typing and executing the following commands: ``` >> x = [0 : 0.1 : 1]; >> y = 2 * x .^ 3; % Note the use of the operator .^ because of the use of vectors >> plot (x, y) ``` the plot that is shown in Fig. 3.6 is displayed in the **Figure Window** replacing the old one. **Fig. 3.6: The graph of the function *y* = 2*x*³** An image of a plot with *x* values on the horizontal axis ranging from 0 to 1 and *y* values on the vertical axis ranging from 0 to 2. The plotted line connects the points (0, 0), (0.1, 0.002), (0.2, 0.032), (0.3, 0.162), (0.4, 0.512), (0.5, 1.25), (0.6, 2.592), (0.7, 4.802), (0.8, 8.192), (0.9, 14.58), (1, 2). The *plot* command has additional optional arguments that can be used to specify the color and style of the line and the color and type of markers, if any are desired. With these options the command has the form: ``` plot(x,y, 'line specifiers') ``` Line specifiers can be used to define the style and color of the line and the type of markers (if markers are desired). The line style specifiers are: |Line Style | Specifier | |-----------------------|-----------| | solid (default) | - | | dotted | : | | dashed | -- | | dash-dot | -. | The line color specifiers are: | Line Color | Specifier | |---------------------|-----------| | red | r | | green | g | | blue | b | | cyan | c | | magenta | m | | yellow | y | | black | k | | white | w | The marker type specifiers are: | Marker | Specifier | |------------------------|-----------| | plus sign | + | | circle | o | | asterisk | * | | point | . | | square | s | | diamond | d | The specifiers are typed inside the *plot* command within a single quote and in any order. The specifiers are optional. This means that none, one, two, or all the three can be included in a command. The following are some examples of the use of the specifiers: * **plot (x, y)** A blue solid line connects the points with no markers (default). * **plot (x, y, 'r')** A red solid line connects the points. * **plot (x, y, ' - - y')** A yellow dashed line connects the point. * **plot (x, y , ‘*’)** No line between the points, but the points are marked with * * **plot (x, y, 'g : d')** A green dotted line connects the points that are marked with diamond markers. The *plot* command creates bare plots. The plot can be modified to include axis labels, a title, and other features. Plots can be formatted by using MATLAB commands that follow the *plot* command. The formatting commands for adding axis labels and a title are: ``` xlabel('text as string') ylabel('text as string') title('text as string') ``` For example, the program listed below produces the plot that is displayed in Fig. 3.7. The data is plotted with a dashed red line and asterisk markers, and the figure includes axis labels and a title. ``` >> yr = [1988:1:1994]; >> sle = [8 12 20 22 18 24 27]; >>plot(yr,sle,'--r*', 'linewidth',2, 'markersize', 12) >>xlabel('YEAR') >>ylabel('SALES (Millions)') >>title('Sales Records') ``` **Fig. 3.7: Formatted plot.** An image of a line plot with data points marked with red asterisk symbols connected by a red dashed line. The *x* values are on the horizontal axis ranging from 1988 to 1994 and the *y* values are on the vertical axis ranging from 0 to 30. The *y* axis label is "SALES (Millions)" and the *x* axis label is "YEAR". The title is "Sales Records". ### 3.14-1 Plotting multiple graphs in the same plot There are two methods to add multiple graphs to an existing figure: 1. Using the *plot* command: by typing pairs of vectors inside the *plot* command. In this case, the syntax of the command is: ``` plot (x1, y1, X2, У2, Хз, Уз) ``` The above command creates three graphs: *y*1 versus *x*1, *y*2 versus *x*2, and *y*3 versus *x*3, all in the same plot. MATLAB automatically plots the graphs in different colors so that they can be identified. It is also possible to add line specifiers following each pair. For example the command ``` plot (x1, y1,' - b', x2, y2, - -r', x3, уз,': g') ``` plots *y*1 versus *x*1 with a solid blue line, *y*2 versus *x*2 with a dashed red line and *y*3 versus *x*3 with a dotted green line. For example, the program listed below produces the plot that is displayed in Fig. 3.8 for the following three functions: - *y*1 = 2*x* - *y*2 = 4*x*2 - *y*3 = *e*x2 ``` >> x = linspace (0, 1, 10); % ten points are used to create the vector x >> % defining the three dependent vectors y1, y2, and y3 >> y1 = 2 * x; >> y2 = 4 * x .^2; % Because x is a vector, element-by-element exponentiation is used >> y3 = exp(x.^2); >> plot (x, y1, x, y2, x, y3) ``` **Fig. 3.8: The three graphs plotted in the same Figure Window.** An image of a plot with *x* values on the horizontal axis ranging from 0 to 1 and *y* values on the vertical axis ranging from 0 to 4. Three lines are plotted on the same graph. The first line is blue and connects the points (0, 0), (0.1, 0.2), (0.2, 0.4), (0.3, 0.6), (0.4, 0.8), (0.5, 1), (0.6, 1.2), (0.7, 1.4), (0.8, 1.6), (0.9, 1.8), (1, 2), representing the function *y*1 = 2 * *x*. The second line is green and connects the points (0, 0), (0.1, 0.04), (0.2, 0.16), (0.3, 0.36), (0.4, 0.64), (0.5, 1), (0.6, 1.44), (0.7, 1.96), (0.8, 2.56), (0.9, 3.24), (1, 4), representing the function *y*2 = 4 * *x*2. The third line is red and connects the points (0, 1), (0.1, 1.01), (0.2, 1.04), (0.3, 1.11), (0.4, 1.21), (0.5, 1.37), (0.6, 1.6), (0.7, 1.93), (0.8, 2.34), (0.9, 2.86), (1, 3.5), representing the function *y*3 = *e*x2. To better identify the graphs representing the above three functions is to use line specifiers for each graph by typing the following commands: ``` >> x = linspace (0, 1, 10); % ten points are used to create the vector x >> % defining the three dependent vectors y1, y2, and y3 >> y1 = 2 * x; >> y2 = 4 * x .^2; % Because x is a vector, element-by-element exponentiation is used >> y3 = exp(x.^2); >> plot (x, y1, '-r+', x, y2, '--g*', x, y3, '-.bo') ``` **Figure 3.9 is the Figure Window for plotting the three functions with the use of line specifiers, where the graph representing the first function is plotted with a solid red line with red plus (+) markers, the graph representing the second function is plotted with a dashed green line with green star (*) markers, and the final graph representing the third function is plotted with a blue dash-dot line with blue circle (o) markers.** An image containing a plot of three lines with different line styles, red +, green *, and blue o. ### 2. Using the *hold on*, *hold off* commands: Several graphs can be added to an existing graph using the *hold* command. One graph is plotted first with the *plot* command, and then the *hold on* command is typed. This keeps the **Figure Window** with the first plot open. Additional graphs can be added with *plot* commands that are typed next. Each *plot* command comes after the *hold on* command creates a graph added to the present figure. The *hold off* command stops this process. It returns to the default mode in which the *plot* command erases the previous graph. The following example represents the plotting of the three functions: - *y*1 = sin *x* - *y*2 = cos *x* - *y*3 = sin *x* + cos *x* in the interval 0 ≤ *x* ≤ 2π, in the same figure using the *hold on* and *hold off* commands (Fig. 3.10). ``` >> x = [0: pi/20 : 2 * pi]; >> y1 = sin(x); >> y2 = cos(x); >> y3 = sin(x) + cos(x); >> plot(x, y1, 'r+') % The first graph is created >> hold on >> plot (x, y2, 'g*') % The second graph is created and added to the first >> plot (x, y3, 'bo') % The third graph is created and added to the first and the second >> hold off ``` **Fig. 3.10: The three graphs plotted using the *hold on*, and *hold off* commands.** An image containing three graphs plotted on top of each other. The first plot is a red solid line with a + marker, the second plot is a green line with a * marker, and the third plot is a blue circle with a o marker. ## 3.14-2 The *legend* command The *legend* command places a legend on the plot. The legend shows a sample of the line style of each graph that is plotted, and places a label beside the line sample. The syntax of the command is: ``` legend('text1 string', 'text2 string', ...) ``` The *text*1, *text*2 are the labels that are placed next to the line sample. Their order corresponds to the order that the graphs were created. ## 3.14-3 The *text* command A *text* command places a text in the figure such that the first character is positioned at the point with the coordinate *x*, *y*. The *text* command has the syntax: ``` text(x, y, 'text string') ``` ## 3.14-4 The *gtext* command The *gtext* command places the text at a position specified by the user with a mouse. When the command is executed, the **Figure Window** opens and the user specifies the position with the mouse. The *gtext* command has the syntax: ``` gtext('text string') ``` For example, the commands listed below produces the plot that is displayed in Fig. 3.11. The example plots the two functions *y* = sin *x* with a solid blue line and *y* = cos *x* with a dashed red line for -2π ≤ *x* ≤ 2π in a single figure using *legend* and *text* commands. ``` >> clear % Clear the memory from the previous defined variables. >> x = [ -2*pi: pi/100: 2*pi]; % Create the vector x >> y1 = sin(x); % Define the first function >> y2 = cos (x); % Define the second function >> plot (x, y1, '-b'); % plotting the first function >> hold on % To allow plotting in the same Figure Window >> plot (x, y2, '--r'); % plotting of the second function >> hold off % stop plotting in the same figure >> % The following commands are used for graph annotation >> xlabel('The x- axis'); >> ylabel (' The y-axis'); >> legend('sin(x)', 'cos(x)'); >> text(-4, 0.6, 'The graphs of the functions y = sin (x) and y = cos(x)'); >> title(' The plot of sin (x) versus cos (x)'); % title appears at the top of the figure ``` **Fig. 3.11: The graphs of *y*=sin *x* and *y*=cos *x* using *Legend* and *Text* commands.** An image containing a plot of a blue line representing sin (*x*) and a dashed line representing cos (*x*). There is a legend on the plot that correctly identifies each line. There is also a text box identifying the two lines, in the upper right corner of the plot. The title of the plot is "The plot of sin (*x*) versus cos (*x*)". ## 3.14-5 The *axis* limits In MATLAB the plot automatically scales the axis limits to fit the data. But if it is desired to plot a curve in a region specified by *v* = [*xmin*, *xmax*, *ymin*, *ymax*], enter the command *axis*(v), where *v* is a four-element vector, which sets the scaling on the current plot, i.e., draw the graph first, then reset the axis limits. The command *axis auto* can return to the default of automatic axis scaling. ## 3.14-6 The *grid* commands *grid* command adds/removes grid lines to/from the current graph. The grid state may be toggled. It also has the form: - **grid on** Adds grid lines to the plot - **grid off** Removes grid lines from the plot **Example** For example, the commands listed below produces the plot that is displayed in Fig. 3.12. The example plots the function *z* = sin(*t*) + *t* cos(*t*) for the range -2π ≤ *t* ≤ 2π using the *axis* and *grid* commands, in addition to other formatting commands. ``` >> t = [-2*pi : pi/50 : 2*pi]; >> z = sin(t) + t .* cos(t); >> plot (t, z) >> grid on; >> axis([-2*pi, 2*pi, -6, 6]); >> xlabel('The independent variable t'); >> ylabel ('The dependent variable z'); >> title(' The plot of z = sin(t) + t cos(t)'); >> ``` **Fig. 3.12: The graphs of *z* = sin(*t*) + *t* cos(*t*) using *grid* and *axis* commands.** An image of a line plot representing the function *z* = sin(*t*) + *t* cos(*t*) with a grid overlay. The *x* values are on the horizontal axis ranging from -6 to 6, and the *y* values are on the vertical axis ranging from -6 to 6. The *y* axis title is "The dependent variable *z*". The *x* axis title is "The independent variable variable *t*". The title of the plot is "The plot of *z* = sin(*t*) + *t* cos(*t*)".