Chapter Three Introduction to MATLAB PDF
Document Details
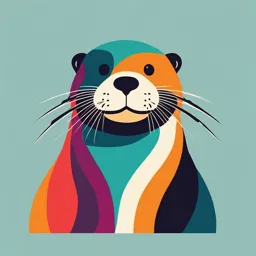
Uploaded by FeatureRichCedar
Tags
Summary
This document is an introduction to MATLAB, a programming language for technical computing. It describes how to use MATLAB for basic calculations and the different commands that are available. This document also provides some examples.
Full Transcript
# Chapter Three Introduction to MATLAB At the end of this chapter, the students will be able to: - Use MatLab as a calculator (a tool for performing math tasks). - Create a vector in MatLab with different method. - Create a two-dimensional array in MatLab. - Manipulate data using arrays in MATLAB....
# Chapter Three Introduction to MATLAB At the end of this chapter, the students will be able to: - Use MatLab as a calculator (a tool for performing math tasks). - Create a vector in MatLab with different method. - Create a two-dimensional array in MatLab. - Manipulate data using arrays in MATLAB. - Write Matlab codes to solve engineering problems. - Perform basic plotting tasks. - Change plot specifics and plot modification. # CH.3 Introduction to MatLab ## 3.1 What is MATLAB? MATLAB is a high-performance language for technical computing. It integrates computation, visualization, and programming in an easy-to-use environment where problems and solutions are expressed in familiar mathematical notation. Typical uses include: - Math and computation - Algorithm development - Modeling, simulation, and prototyping - Data analysis, exploration, and visualization - Scientific and engineering graphics - Application development, including Graphical User Interface building MATLAB is a commercial product of The MathWorks Company (http://www.mathworks.com/). The name MATLAB stands for Matrix LABoratory. MATLAB is an interactive system whose basic data element is an array that does not require dimensioning. This allows you to solve many technical computing problems, especially those with matrix and vector formulations, in a fraction of the time it would take to write a program in a scalar non-interactive language such as C or FORTRAN. Programs written using MATLAB can be interfaced with programs written in other programming languages including C, C++, Java, and FORTRAN. ## 3.2 MATLAB as a calculator 1. As an example of a simple interactive calculation, just type the following expression 1 + 2 × 3 at the prompt command `>>` and then press the Enter key to compute it. You obtain the following window in Figure 3.1. ``` >>1+2*3 ans = 7 ``` 2. Note that if you do not specify an output variable, MATLAB uses a default variable `ans`, short for answer, to store the results of the current computation. 3. The variable `ans` is overwritten, if there is more than one computation. It always stores the result of the last calculation, as one can see from Fig. 3.2 which shows simple calculations and displaying `ans` after the prompt command and pressing the Enter key. ``` >>1+2*3 ans = 7 >>3+5 ans = 8 ``` 4. To avoid this, you may assign a value to a variable as you see in Fig. 3.3. 5. Note from Fig. 3.3 that the variable `x` can always be used to refer to because it is stored in the computer memory in a location named `x`. Therefore the expression `y =x`, assigns what is in the location `x` to another location in the memory designated by the variable named `y`. Both these two variables `x` and `y` can be used in a new expression `z = x + y`. All the values of variables `x`, `y`, and `z` are stored in computer memory locations named `x`, `y`, and `z` respectively, as in Fig. 3.4. ``` >>x = 1+ 2*3 x = 7 >>y =x y = 7 >>x = 1+ 2*3 x = 7 >>y =x y = >>z = x + y z = 14 ``` ## 3.3 Guidelines for working in the Command Window 1. The command is executed once the Enter key is pressed. 2. Several commands can be typed in the same line but must be separated by a comma and they are executed in order from left to right. 3. Use the up arrow key (↑) to go back to a previously command line in the Command Window if you want to modify it and then re-execute it. 4. Use the down arrow key (↓) to move down to the previously typed command. 5. If a command is too long to fit in one line, type three dots ... to make it continue to the next line and then press the Enter key. ## 3.4 The semicolon symbol (;) 1. When a semicolon (;) is typed at the end of a command the output of the command is not displayed when the Enter key is pressed. This is useful when the output is obvious or very large. 2. If several commands are typed in the same line, the output from any of the commands will not be displayed if a semicolon is typed between the commands instead of a comma. ## 3.5 The percent symbol (%) 1. When the symbol % is typed at the beginning of a line, the line is designated as a comment i.e. when the Enter key is pressed the line is not executed. 2. Comments are used in programs to add description or to explain the program. Usually there is no need for comments in the Command Window. ## 3.6 The Percent-Brace %{ %} 1. The %{ and %} symbols enclose a block of comments that extend beyond one line. 2. Enclose any multiline comments (called block comment) with percent followed by an opening or closing brace as follows: ``` >> %{ The purpose of this example is to compute y = f(x) %} ``` 3. The %{ and %} operators must appear alone on the lines that immediately precede and follow the block of comments. Do not include any other text on these lines. ## 3.7 The clc command 1. Typing `clc` after the prompt (>>) and pressing the Enter key clears the Command Window. 2. The `clc` command does not change anything that was done before; if some variables were defined previously they still exist and can be used. 3. You can use the up arrow key to recall commands that were typed before. ## 3.8 The arithmetic operations with numbers 1. Numbers can be assigned to variables which can subsequently be used in calculation. 2. There are three kinds of numbers used in MATLAB: - integers - real numbers - complex numbers 3. Integer numbers have no fractional part or decimal point. Integers are entered without the decimal point as follows: ``` >>xi = 10 xi = 10 ``` 4. Real numbers have fractional part or decimal point. They are entered with the decimal point as follows: ``` >>xr = 10.0321 xr = 10.0321 ``` 5. Complex numbers are constructed from real and imaginary components. The complex number c = x+ yi is represented in MATLAB as c= complex (x, y) where x, and y are the real and imaginary components respectively. The symbol i or j is the imaginary unit √-1. The following example illustrates the representation of a complex number in MATLAB and how to obtain the real and the imaginary component of a complex number using real (), and imag () respectively. ``` >>c = complex (3, 4) c = 3.0000 + 4.0000i >>x = real (c) x = 3 >>y = imag (c) y = 4 ``` 6. The six basic arithmetic operations with numbers used in MATLAB are presented in Table 3.2. 7. Table 3.3 presents the order of precedence of the arithmetic operations. | Operation | Symbol | Example | |--------------------|--------|--------------------| | Addition | + | `>> 6 + 2` | | | | `ans =` | | | | `8` | | Subtraction | - | `>> 6 - 2` | | | | `ans = ` | | | | `4` | | Multiplication | * | `>> 6 * 2` | | | | `ans =` | | | | `12` | | Left division | / | `>> 6/2` | | | | `ans =` | | | | `3` | | Right division | \ | `>> 6\2` | | | | `ans =` | | | | `0.3333` | | Exponentiation | ^ | `>> 6^2` | | | | `ans =` | | | | `36` | Table 3.3: Order of precedence of arithmetic operations | Precedence | Mathematical operation | |-------------|------------------------------------| | First | Parentheses | | | For nested parentheses, the innermost are executed first | | Second | Exponentiation | | Third | Multiplication and division both are equal precedence | | Fourth | Addition and subtraction | 8. In a mathematical expression that has several operations; higher precedence operations are executed before lower precedence operations. If two or more operations have the same precedence, the expression is executed from left to right. Parentheses are used to change the order of calculations. ## Examples The purpose of the following examples is to illustrate the order of precedence of the arithmetic operations in the calculation of mathematical expressions and some of the discussed commands and features. ``` >>3 + 4/2 % 4/2 is executed first ans = 5 >> (3 + 4)/2 % (3 + 4) is executed first ans = 3.5000 >>3 + 4/2 - 2 % 4/2 is executed first, then addition and subtraction ``` ``` >> %{ The following example calculate the expression 9^(1/2) + 27^2/3 1½ is executed first, 9 ^ (1/2), then 27 ^ 2 and 27 ^ 2 / 3 are executed next and + is executed last. %} >> 9^(1/2) + 27^2/3 ans = 246 ``` ``` >> % Using the three periods ... to continue to the next line >> 4*2 + (6+8)/4 + 4*2^2 + 2*3^2 - 2*52 /63 +4 + 3/ (15 -8/2) ... + 6/3 % Leave a single space after (15 - 8/2), then type ... and press Enter ans = 50.1219 ``` ``` >> % the comma is used when we need to calculate more than one >> %expression in one line >> 4/3 +5* 2,7-9 + 27/8 ans = 11.3333 ans = 1.3750 >> % The semicolon is used to prevent the calculation of the >> % expression in the command window. >> 5*9 + 8^2; ``` ## 3.9 Variables in MATLAB 1. The name of variable consists of a letter, followed by any number of letters, digits, or underscores. 2. The first character of the variable's name should not be a number. 3. The variable's name should not contain spaces. 4. MATLAB is case sensitive; it distinguishes between uppercase and lowercase letters, N and n are not the same variable. 5. The maximum number of characters of a variable's name in MATLAB R2009a is 63 characters. 6. To determine the maximum number of characters of a variable's name of the MATLAB version installed in your computer: type N = namelengthmax (namelengthmax is a built-in function) in the Command Window and press the Enter key as: ``` >> N = namelengthmax N = 63 ``` 7. The = sign in MATLAB is called the assignment operator. It takes the following form: `Variable_name = A numerical value, or computable expression` 8. The left hand side of the assignment operator can include only one variable name. 9. The right hand side of the assignment operator can be a number, or a computational expression that can include numbers and/or variables that were previously assigned numerical values. 10. When MATLAB encounters a new variable name, it automatically creates the variable and allocates the appropriate amount of memory storage. If the variable already exists, MATLAB changes its contents. For example, `num_students = 25` creates a variable named `num_students` and stores the value 25 in a memory location called `num_students`. 11. To view the value assigned to any variable, simply type the variable name and press the Enter key. MATLAB displays the variable name and its assigned value in the next two lines as ``` >> num_students = 25 num_students = 25 ``` 12. Avoid using the names of a built-in function (explained later) for a variable, because once a function name is used to define a variable, the function cannot be used. 13. The following are valid MATLAB variable assignments: ``` a = 1 speed = 1500 BeamFormer Output_Type1 = y1*Q*y2 ``` 14. These are invalid assignments: ``` 2for1 = yes (Because the first character is a number) first one = 1 (Because the variable name contains a space) ``` 15. There are a number of variables that are already defined when MATLAB is started. Some of these predefined variables are: - ans A variable that has the value of the last expression that was not assigned to a specific variable. - pi the number π, and equals 3.1416 - inf used for infinity ∞ - eps the smallest difference between two numbers, which equals to 2^(-52). It equals 2.2204e-016 - i defined as √-1 which is 0 + 10000i - j same as i - NaN stands for Not-a-Number. Used when MATLAB cannot determine a valid numeric value, as 0/0. 16. The following Command Window displays the values of the predefined variables ``` >> pi ans = 3.1416 >> eps ans = 2.2204e-016 >> inf ans = Inf >> i ans = 0 + 1.0000i >> j ans = 0 + 1.0000i >> 0/0 ans = NaN ``` ## Examples ``` >> %{ Example1: assigns 8 to the variable y %} >> y = 8 y = 8 ``` ``` >> %{ Example 2: Assigns a new value to y. The new value is 5 times the previous value of y divided by 4 %} >> y = 5*y/4 y = 10 ``` ``` >> %{ Example 3: Assigns -6 to x, 3 to y and calculates the expression z = 3*x^2*y^2. A comma is used to separate between the assignment expressions to type them all in the same line %} >> x = -6, y = 3, z = 3 *x^2 * y^2 x = -6 y = 3 z = 972 ``` ``` >> %{ Example 4: The same as example 3, but it uses the semicolon (;) between x and y to only display the value of z %} >> x = -6; y = 3; z = 3 * x^2 * y^2 z = 972 ``` ## 3.10 Commands for managing variables 1. When you define a variable at the MATLAB prompt, it is defined inside of MATLAB's "Workspace". 2. The Workspace is temporarily memory locations contain all the defined variables in the current MATLAB's session. 3. During a session of using MATLAB, one may use too many variables that cannot be remembered, and may be affect the computation of the next problem. Therefore the use of one of the following commands is good for managing variables in the Workspace - who Displays a list of variables currently in the Workspace. - whos Displays a list of variables currently in the Workspace and their sizes together with information about their bytes and class. - clear Removes all variables from the Workspace. - clear x y z Removes only variables x, y, and z. 4. Note that the command `clc` clears the screen and does not remove what are stored in the Workspace. ## Examples ``` >> a = 3 a = 3 >> b = 6, x = a+ b/a % a comma is used to type more than one expression b = 6 x = 5 >> who % who command is used to display variables in memory Your variables are: abx >> whos % whos is used to display variables“ details Name Size Bytes Class Attributes a 1x1 8 double b 1x1 8 double x 1x1 8 double >> clear x % clear command removes the variable x from the memory >> who % who command display only the two variables a and b Your variables are: ab ``` ## 3.11 Elementary math built-in functions 1. A function should have a name and accepts one or more MATLAB variables (called arguments) between parenthesis as inputs, operates on them in some way, and then returns one or more MATLAB variables as outputs. 2. Table 3.4 lists some commonly used built-in functions, where variables x and y can be numbers, a variable that has been assigned a numerical value, or a computable expression. | Function | Description | Function | Description | |---|---|---|---| | cos(x) | Cosine | abs(x) | Absolute value | | sin(x) | Sine | sign(x) | Signum function | | tan(x) | Tangent | max(x) | Maximum value | | acos(x) | Arc cosine | min(x) | Minimum value | | asin(x) | Arc sine | ceil(x) | Round towards +∞ | | atan(x) | Arc tangent | floor(x) | Round towards -∞ | | exp(x) | Exponential | round(x) | Round to nearest integer | | sqrt(x) | Square root | rem(x,y) | Remainder after division | | log(x) | Natural logarithm | angle(x) | Phase angle | | log10(x) | Common logarithm | conj(x) | Complex conjugate | Table 3.4: Elementary functions ## Examples ``` >> %Example1: Calculating the natural logarithm of 753 (In 753) >> log(753) ans = 6.6241 ``` ``` >> %Example2: Calculate y= 2*sqrt(a^2-c^2), where a=5 and c= 4 >> a = 5 % assigning 5 to a a = 5 >> c = 4 % assigning 4 to c c = 4 >> y = 2* sqrt(a^2-c^2) y = 6 >> %Example3: Example2 can be typed in a single line using the comma >> a=5, c=4, y = 2*sqrt(a^2-c^2) a = 5 c = 4 y = 6 ``` 3. Because MATLAB is a huge program; it is impossible to cover all the details of each function one by one. However, you can request information on a specific function by typing help followed by the function name in the Command Window, then pressing the Enter key as shown in the following example: ``` >> help sqrt SQRT Square root. ``` ## 3.12 Display formats in MATLAB 1. The format in which MATLAB displays output on the screen can be changed by the user. The default output format is fixed point with four decimal digits (called short). 2. The format can be changed with the format command. 3. Once the format command is entered, all the output that follows will be displayed in the specified format. 4. Several of the available formats are listed and described in Table 3.5. | Command | Description | Example | |--------------|--------------------------------------------------------------------------------------------------------------------|---------------------------------------------------------------------------------------| | format short | Fixed point with four decimal digits for: 0.001 ≤ number ≤ 1000. Otherwise display format short e. | `>> 290/7` | | | `ans =` | | | `41.4286` | | format long | Fixed point with 14 decimal digits for: 0.001 ≤ number ≤ 100. Otherwise display format long e. | `>> 290/7` | | | `ans =` | | | `41.42857142857143` | | format short e | Scientific notation with four decimal digits. | `>> 290/7` | | | `ans =` | | | `4.1429e+001` | | format long e | Scientific notation with 15 decimal digits. | `>> 290/7` | | | `ans =` | | | `4.142857142857143e+001` | | format short g | Best of 5-digit fixed or floating point. | `>> 290/7` | | | `ans =` | | | `41.429` | | format long g | Best of 15-digit fixed or floating point. | `>> 290/7` | | | `ans =` | | | `41.4285714285714` | | format bank | Two decimal digits. | `>> 290/7` | | | `ans =` | | | `41.43` | Table 3.5: Display format