Lec6-Control Structure part1.pdf
Document Details
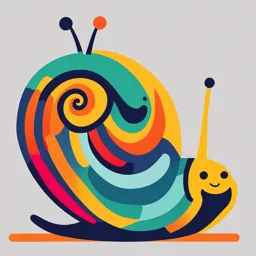
Uploaded by InfallibleSagacity6104
National University
Full Transcript
Control Structure part1 Learning Outcomes Be able to use Java conditional constructs, including if, if-else, and switch, as well as the nested version of those constructs correctly. Be able to perform equality testing appropriate with each primitive data type. Be able to design program...
Control Structure part1 Learning Outcomes Be able to use Java conditional constructs, including if, if-else, and switch, as well as the nested version of those constructs correctly. Be able to perform equality testing appropriate with each primitive data type. Be able to design programs that require the inclusion of decisions. 2 Control structures Control structures – allows us to change the ordering of how the statements in our programs are executed Two types of Control Structures – decision control structures allows us to select specific sections of code to be executed – repetition control structures allows us to execute specific sections of the code several times Decision control structures – Java statements that allows us to select and execute specific blocks of code while skipping other sections Types: if-statement if-else-statement If-else if-statement 4 if Statement The if -construct stipulates that a block of code will be performed if and only if a certain Boolean statement is true. if Statement if Statement The if-statement has the form, if( boolean_expression ) statement; or if( boolean_expression ){ statement1; statement2;... } Example 1 8 Example 2 9 Example 3 PRESENTATION TITLE 10 Example 4 PRESENTATION TITLE 11 Coding Guidelines 1. The boolean_expression part of a statement should evaluate to a boolean value. That means that the execution of the condition should either result to a value of true or a false. 2. Indent the statements inside the if-block. For example, if( boolean_expression ){ //statement1; //statement2; } If else Statement It is used when you want to execute a certain statement if a condition is true, and a different statement if the condition is false. If else Statement If else Statement The if-else statement has the form, if( boolean_expression ) statement; else statement; or can also be written as, if( boolean_expression ){ statement1; statement2;... } else{ statement1; statement2;... } Example1 PRESENTATION TITLE 16 Example2 PRESENTATION TITLE 17 If-else-if Statement The statement in the else-clause of an if-else block can be another if-else structures. This cascading of structures allows us to make more complex selections. If-else-if Statement If-else-if Statement The if-else if statement has the form, if( boolean_expression1 ) statement1; else if( boolean_expression2 ) statement2; else statement3; If-else-if Statement can also be written as, if( boolean_expression1 ){ statement1; statement2;... } else if( boolean_expression2 ){ statement1; statement2;... } else{ statement1; statement2;... } Example1 22 Example2 Exercise Create a program that checks if a given number is divisible by 6 and 7. Sample Outputs Scenario1 Scenario2 Scenario3 Scenario4 Num = -18 Num=21 Num=10 Num=42 Divisible by 6 Divisible by 7 Not divisible Divisible by 6 and 7 Answer 25 Example3 PRESENTATION TITLE 26 Example 4 27 Example 5 28 Example 6 PRESENTATION TITLE 29 Exercise Create a program that determines whether a user's input is a consonant, a vowel, or a number. 30 Answer 9/8/20XX PRESENTATION TITLE 31 Laboratory Activity 2 32 Questions?? Lecture Activity Prepare 1/4 sheet of yellow paper. What is the best definition of if-else-if statement? a. When you have another condition to evaluate when the first condition is false. b. When you have another condition to evaluate when the first condition is true. c. When you must evaluate multiple conditions. d. When you evaluate a condition and when it's false there is statements to execute. 9/8/20XX PRESENTATION TITLE 38 You need to create a Java program that has Rap move if the front is clear, but if it isn't clear, Rap will do nothing. Which control structure do you need to use? a. If statement b. If else statement c. if else if statement d. Nested if 9/8/20XX PRESENTATION TITLE 40 Select all true statements from the following statements. a. There must be at least one else statement after else if statement. b. There must be at least one else statement after if statement. c. else statement must have corresponding if statement. d. Curly brackets are mandatory for if, else if and else statements 9/8/20XX PRESENTATION TITLE 42 What is the output of the Java program? int marks=70; if(marks >= 80) System.out.println("DISTINCTION"); else if(marks >=65) System.out.println("PASSED"); else System.out.println("FAILED"); a. Distinction b. Passed c. Failed d. Distinction Passed 9/8/20XX PRESENTATION TITLE 44 What is the output of the Java program? int x = 3; int y = 2; if (x > 2) x++; if (y > 1) y++; if (x > 2) System.out.print(“SCS "); if (y < 3) System.out.print(“NU Laguna"); System.out.print(“BSCS BSIS"); a. SCS b. NU Laguna c. SCS, NU Laguna d. SCS, BSCS BSIS 9/8/20XX PRESENTATION TITLE 46 What is the value of grade when the following code executes, and score is 85? if (score >= 90) grade = "A"; if (score >= 80) grade = "B"; if (score >= 70) grade = "C"; else grade = “D"; System.out.println(grade); a. A b. B c. C d. D 9/8/20XX PRESENTATION TITLE 48 What is the output of this program? int var1=5; int var2=6; if((var2=3)==var1) System.out.println(var2); else System.out.println(++var2); a. 6 b. 7 c. 4 d. 3 9/8/20XX PRESENTATION TITLE 50 Here is the segment of a program int x=3, y=2, n=2; if (n>1) x=x+1; y=y-1; What will be the values of x and y if n=2? a. x=4 , y =1 b. x=0 , y =0 c. x=1 , y =1 d. x= 3, y =2 9/8/20XX PRESENTATION TITLE 52 At a certain college school students receive letter grades based on the following scale: 93 or above is an A, 84 to 92 is a B, 75 to 83 is a C, and below 75 is an F. Which of the following code segments will assign the correct string to grade for a given integer score? a. if (score >= 93) b. if (score >= 93) c. if (score >= 93) grade = "A"; grade = "A"; grade = "A"; if (score >= 84 && score = 84) else if (score >= 84) grade = "B"; grade = "B"; grade = "B"; if (score >=75 && score =75) else if (score >=75) grade = "C"; grade = "C"; grade = "C"; if (score < 75) if (score < 75) else grade = "F"; grade = "F"; grade = "F"; 9/8/20XX PRESENTATION TITLE 54 What is the output of this program? double baseSalary = 50000.0; int experience = 5; if (experience > 10) { bonus = baseSalary * 0.20; } else if (experience > 5) { bonus = baseSalary * 0.10; } else if (experience > 2) { bonus = baseSalary * 0.05; } else { bonus = 0; } double totalSalary = baseSalary + bonus; System.out.println("Total Salary: Php" + totalSalary); A) Total Salary: Php55,000.0 B) Total Salary: Php52,500.0 C) Total Salary: Php50,000.0 D) Total Salary: Php60,000.0 55 9/8/20XX PRESENTATION TITLE 56 What is the best definition of if-else-if statement? a. When you have another condition to evaluate when the first condition is false. b. When you have another condition to evaluate when the first condition is true. c. When you must evaluate multiple conditions. d. When you evaluate a condition and when it's false there is statements to execute. You need to create a Java program that has Rap move if the front is clear, but if it isn't clear, Rap will do nothing. Which control structure do you need to use? a. If statement b. If else statement c. if else if statement d. Nested if Select all true statements from the following statements. a. There must be at least one else statement after else if statement. b. There must be at least one else statement after if statement. c. else statement must have corresponding if statement. d. Curly brackets are mandatory for if, else if and else statements What is the output of the Java program? int marks=70; if(marks >= 80) System.out.println("DISTINCTION"); else if(marks >=65) System.out.println("PASSED"); else System.out.println("FAILED"); a. DISTINCTION b. PASSED c. FAILED d. DISTINCTION PASSED What is the output of the Java program? int x = 3; int y = 2; if (x > 2) x++; if (y > 1) y++; if (x > 2) System.out.print(“SCS "); if (y < 3) System.out.print(“NU Laguna"); System.out.print(“BSCS BSIS"); a. SCS b. NU Laguna c. SCS, NU Laguna d. SCS, BSCS BSIS What is the value of grade when the following code executes, and score is 85? if (score >= 90) grade = "A"; if (score >= 80) grade = "B"; if (score >= 70) grade = "C"; else grade = “D"; System.out.println(grade); a. A b. B c. C d. D What is the output of this program? int var1=5; int var2=6; if((var2=3)==var1) System.out.println(var2); else System.out.println(++var2); a. 6 b. 7 c. 4 d. 3 Here is the segment of a program int x=3, y=2, n=2; if (n>1) x=x+1; y=y-1; What will be the values of x and y if n=2? a. x=4 , y =1 b. x=0 , y =0 c. x=1 , y =1 d. x= 3, y =2 At a certain college school students receive letter grades based on the following scale: 93 or above is an A, 84 to 92 is a B, 75 to 83 is a C, and below 75 is an F. Which of the following code segments will assign the correct string to grade for a given integer score? a. if (score >= 93) b. if (score >= 93) c. if (score >= 93) grade = "A"; grade = "A"; grade = "A"; if (score >= 84 && score = 84) else if (score >= 84) grade = "B"; grade = "B"; grade = "B"; if (score >=75 && score =75) else if (score >=75) grade = "C"; grade = "C"; grade = "C"; if (score < 75) if (score < 75) else grade = "F"; grade = "F"; grade = "F"; What is the output of this program? double baseSalary = 50000.0; int experience = 5; if (experience > 10) { bonus = baseSalary * 0.20; } else if (experience > 5) { bonus = baseSalary * 0.10; } else if (experience > 2) { bonus = baseSalary * 0.05; } else { bonus = 0; } double totalSalary = baseSalary + bonus; System.out.println("Total Salary: Php" + totalSalary); A) Total Salary: Php55,000.0 B) Total Salary: Php52,500.0 C) Total Salary: Php50,000.0 D) Total Salary: Php60,000.0 66