chap 1.pdf
Document Details
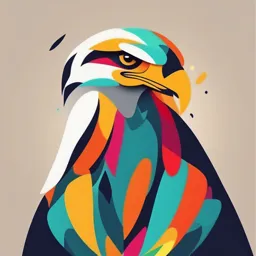
Uploaded by SupremeClematis
University of South Africa
Full Transcript
# Data Types in Python Python has a rich set of fundamental data types. The operations that are applicable on an object depend on its data type (i.e., an object's data type determines which operations are applicable on it). The list of data types are as follows: - **Integers:** Integers are 32 bit...
# Data Types in Python Python has a rich set of fundamental data types. The operations that are applicable on an object depend on its data type (i.e., an object's data type determines which operations are applicable on it). The list of data types are as follows: - **Integers:** Integers are 32 bits long, and their range is from -2<sup>32</sup> to 2<sup>32</sup> - 1 (i.e., from -2,147,483,648 to 2,147,483,647). - **Long Integers:** It has unlimited precision, subject to the memory limitations of the computer. - **Floating Point Numbers:** Floating-point numbers are also known as double-precision numbers and use 64 bits. - **Boolean:** It can hold only one of two possible values: True or False. - **Complex Number:** A complex number has a real and an imaginary component, both represented by float types in Python. An imaginary number is a multiple of the square root of minus one, and is denoted by `j`. For instance, 2+3j is a complex number, where 3 is the imaginary component and is equal to 3 × √-1. - **Strings:** Sequences of Unicode characters. - **Lists:** Ordered sequences of values. - **Tuples:** Ordered, immutable sequences of values. - **Sets:** Unordered collections of values. - **Dictionaries:** Unordered collections of key-value pairs. **Note** Unicode is a standard that uses 16-bit characters to represent characters on your computer. Unlike ASCII (American Standard Code for Information Interchange), which consists of 8 bits, Unicode uses 16 bits and represents characters by integer value denoted in base 16. A number does not include any punctuation and cannot begin with a leading zero (0). Leading zeros are used for base 2, base 8, and base 16 numbers. For example, a number with a leading `0b` or `0B` is binary, base 2, and uses digits 0 and 1. Similarly, a number with a leading `0o` is octal, base 8, and uses the digits 0 to 7, and a number with a leading `0x` or `0X` is hexadecimal, base 16, and uses the digits 0 through 9, plus `a`, `A`, `b`, `B`, `c`, `C`, `d`, `D`, `e`, `E`, `f`, and `F`. **Note** An object that can be altered is known as a mutable object, and one that cannot be altered is an immutable object. # Basic Elements in a Program Every program consists of certain basic elements, a collection of literals, variables, and keywords. The next few sections explain what these terms mean. ## Literals A literal is a number or string that appears directly in a program. The following are all literals in Python: - `10` # Integer literal - `10.50` # Floating-point literal - `10.50j` # Imaginary literal - `'Hello'` # String literal - `"World!"` #2 String literal - `'''Hello World! It might rain today # Triple-quoted string literal Tomorrow is Sunday'''` In Python you can use both single and double quotes to represent strings. The strings that run over multiple lines are represented by triple quotes. ## Variables Variables are used for storing data in a program. To set a variable, you choose a name for your variable, and then use the equals sign followed by the data that it stores. Variables can be letters, numbers, or words. For example: - `l = 10` - `length = 10` - `length_rectangle = 10.0` - `k="Hello World!"` You can see in the preceding examples the variable can be a single character or a word or words connected with underscores. Depending on the data stored in a variable, they are termed as integer, floating point, string, boolean, and list or tuple variables. Like in above examples, the variables `l` and `length` are integer variables, `length_rectangle` is a floating-point variable, and `k` is a string variable. Following are examples of boolean, list, and tuple variables: - `a=True` # Boolean variable - `b=[2,9,4]` # List variable - `c=('apple', 'mango', 'banana')` # tuple variable A tuple in python language refers to an ordered, immutable (non changeable) set of values of any data type. ## Keywords Python has 30 keywords, which are identifiers that Python reserves for special use. Keywords contain lowercase letters only. You cannot use keywords as regular identifiers. Following are the keywords of Python: `and assert break class continue def del elif else except exec finally for from global if import in is lambda nonlocal not or pass raise return try while with yield` # Comments Comments are the lines that are for documentation purposes and are ignored by the interpreter. The comments inform the reader what the program is all about. A comment begins by a hash sign (`#`). All characters after the `#` and up to the physical line end are part of the comment. For example: ```Python # This program computes area of rectangle a=b+c # values of b and c are added and stored in a ``` # Continuation Lines A physical line is a line that you see in a program. A logical line is a single statement in Python terms. In Python, the end of a physical line marks the end of most statements, unlike in other languages, where usually a semicolon (`;`) is used to mark the end of statements. When a statement is too long to fit on a single line, you can join two adjacent physical lines into a logical line by ensuring that the first physical line has no comment and ends with a backslash (`\`). Besides this, Python also joins adjacent lines into one logical line if an open parenthesis (`(`), bracket (`[` ), or brace (`{`) is not closed. The lines after the first one in a logical line are known as continuation lines. The indentation is not applied to continuation lines but only to the first physical line of each logical line. # Printing For printing messages and results of computations, the `print()` function is used with the following syntax, `print(["message"][variable list])` where `message` is the text string enclosed either in single or double quotes. The `variable list` may be one or more variables containing the result of computation, as shown in these examples: - `print("Hello World!")` - `print(10)` - `print(1)` - `print("Length is ", 1)` You can display a text message, constant values, and variable's values through the `print` statement, as shown in the preceding examples. After printing the desired message/value, the `print()` function also prints the newline character, meaning the cursor moves onto the next line after displaying the required message/value. As a result, the message/value displayed through the next `print()` function appears on the next line. To suppress printing of the newline character, end the `print` line with `end=''` followed by a comma (`,`) after the expression so that the `print()` function prints an extra space instead of a newline character. For example, the strings displayed via the next two `print()` functions will appear on the same line with a space in between: - `print("Hello World!", end=" ")` - `print('It might rain today')` You can also concatenate strings on output by using either a plus sign (`+`) or comma (`,`) between strings. For example, the following `print` function will display the two strings on the same line separated by a space: - `print('Hello World!', 'It might rain today')` The following statement merges the two messages and displays them without any space in between: - `print('Hello World!'+'It might rain today')` In order to get a space in between the strings, you have to concatenate a white space between the strings: - `print('Hello World!'+ ' '+ 'It might rain today')` You can also use a comma for displaying values in the variables along with the strings: - `print("Length is ", 1, " and Breadth is ", b)` Assuming the values of variables `l` and `b` are 8 and 5, respectively, the preceding statement will display the following output: `Length is 8 and Breadth is 5` You can also use format codes (`%`) for substituting values in the variables at the desired place in the message: - `print ("Length is %d and Breadth is %d" %(l,b))` where `%d` is a format code that indicates an integer has to be substituted at its place. That is, the values in variables `l` and `b` will replace the respective format codes. **Note** If the data type of the values in the variables doesn't match with the format codes, auto conversion takes place. The list of format codes is as shown in Table 1.1. You will be learning to apply format codes in the next chapter. | Format Code | Usage | |---|---| |`%s`| Displays in string format. | |`%d`| Displays in decimal format. | |`%e`| Displays in exponential format. | |`%f`| Displays in floating-point format. | |`%o`| Displays in octal (base 8) format. | |`%x`| Displays in hexadecimal format. | |`%c`| Displays ASCII code. | The following program demonstrates using the `print()` function for displaying different output: ```Python printex.py print (10) print('Hello World! \ It might rain today. \ Tomorrow is Sunday.') print('''Hello World! It might rain today. Tomorrow is Sunday.''') ``` **Output:** ``` 10 Hello World! It might rain today. Tomorrow is Sunday. Hello World! It might rain today. Tomorrow is Sunday ```