Unit 1. Web programming platforms in server environment. Characteristics of the PHP language V8.pdf
Document Details
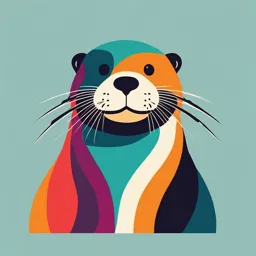
Uploaded by GrandAgate8573
Full Transcript
Index of contents 1. Characteristics of web programming 2 1.1. Static and dynamic web pages 3 1.2. Web applications 6 1.3. Execution of code on the server and on the client....
Index of contents 1. Characteristics of web programming 2 1.1. Static and dynamic web pages 3 1.2. Web applications 6 1.3. Execution of code on the server and on the client. 7 2. Technologies for server-side web programming. 10 2.1. Architectures and platforms. 11 2.2. Selection of a web programming architecture. 13 3. Languages 15 3.1. Embedded code in markup language 17 3.2. Programming tools 18 3.3. Web programming with Java 20 3.4. Web programming with PHP 22 4. PHP language features 23 4.1. PHP language elements 23 4.1.1. Variables and data types in PHP 23 4.1.2. Scope of variable use 25 4.1.3. Special PHP variables 26 4.1.4. Expressions and operators 28 4.1.5. HTML code generation 29 4.1.6. Text strings 32 4.1.7. Functions related to data types 33 4.2. Control structures 36 4.2.1. Conditionals 37 4.2.2. Loops 38 4.3. Functions 39 4.3.1. Inclusion of external files 40 4.3.2. Execution and creation of functions 41 4.3.3. Arguments 42 4.4. Compound data types 44 4.4.1. Traversing arrays (I) 46 4.4.2. Traversing arrays (II) 47 4.4.3. Functions related to compound data types 48 4.5. Web forms 49 4.5.1. Processing of the information returned by a web form 51 4.5.2. Generating web forms in PHP 56 4.5.2.1. HTML tags and how to process them in php 56 4.5.2.2. Uploading files to the web server 83 1. Characteristics of web programming You probably already know exactly what a web page is, and you may even know the steps that take place so that when you visit a website by entering its address in your browser, the page is downloaded to your computer and can be displayed. However, this procedure, which may seem simple, is sometimes not so simple. It all depends on how the page was made. When a web page is downloaded to your computer, its content defines what should be displayed on the screen. This content is programmed in a mark-up language, made up of tags, which can be HTML or XHTML. The tags that make up the page indicate the purpose of each of the parts that make it up. Thus, within these languages there are tags to indicate that a text is a heading, that it is part of a table, or that it is simply a paragraph of text. In addition, if the page is well structured, the information that tells the browser how to style each part of the page will be stored in another file, a style sheet or CSS. The style sheet is indicated on the web page and the browser downloads it along with the page. In it we can find, for example, styles that indicate that the header should be in Arial font and in red, or that paragraphs should be aligned to the left. These two files are downloaded to your computer from a web server in response to a request. The process is as shown in the figure below. The steps are as follows: 1. Your computer requests a page with a.htm,.html or.xhtml extension from a web server. 2. The server looks for that page in a store of pages (each page is usually a file). 3. If the server finds that page, it retrieves it. 4. Finally, it sends it to the browser so that it can display its content. This is a typical example of client-server communication. The client is the one that makes the request and initiates the communication, and the server is the one that receives the request and answers it. In our case, the browser is the web client. 1.1. Static and dynamic web pages The pages you saw in the example above are called static web pages. These pages are stored in their final form, as they were created, and their content does not change. They are useful for displaying a particular piece of information, and will display that same information each time they are loaded. The only way they can change is if a programmer modifies them and updates their content. As opposed to static web pages, as you might imagine, there are dynamic web pages. These pages, as their name suggests, are characterised because their content changes depending on several variables, such as the browser you are using, the user you are logged in with, or the actions you have performed previously. Within dynamic web pages, it is very important to distinguish two types: Those that include code that is executed by the browser. In these pages the executable code, usually in JavaScript language, is included within the HTML (or XHTML) and is downloaded along with the page. When the browser displays the page on screen, it executes the accompanying code. This code can incorporate multiple functionalities that can range from displaying animations to completely changing the appearance and content of the page. In this module we are not going to look at JavaScript, except where it relates to server-side web programming. As you know, there are many pages on the Internet that do not have.htm,.html or.xhtml extensions. Many of these pages have extensions such as.php,.asp,.jsp,.cgi or.aspx. In these, the content that is downloaded to the browser is similar to that of a static web page: HTML (or XHTML). What changes is the way in which this content is obtained. Contrary to what we have seen so far, these pages are not stored on the server; more specifically, the content that is stored is not the same content that is then sent to the browser. The HTML of these pages is formed as a result of the execution of a programme, and that execution takes place on the web server (although not necessarily by that same server). The scheme of operation of a dynamic web page is as follows: 1. The web client (browser) on your computer requests a web page from a web server. 2. The server looks for that page and retrieves it. 3. In the case of a dynamic web page, i.e. its content must be executed to obtain the HTML to be returned, the web server contacts the module responsible for executing the code and sends it to you. 4. As part of the execution process, it may be necessary to obtain information from some repository, such as querying records stored in a database. 5. The result of the execution will be a page in HTML format, similar to any other non-dynamic web page. 6. The web server sends the result obtained to the browser, which processes it and displays it on the screen. This procedure takes place all the time when we consult web pages. For example, when you check your email on GMail, HotMail, Yahoo or any other webmail service, the first thing you have to do is enter your username and password. Next, the server will usually show you a screen with your inbox, which displays the messages received in your account. This screen is a clear example of a dynamic web page. Obviously, the browser does not send the same page to all users, but generates it dynamically depending on the user who connects. To generate it, it runs a programme that obtains your user data (your contacts, the list of messages received) and with them composes the web page that you receive from the web server. Although using dynamic web pages may seem like the best way to build a website, it is not always the best option. It is undoubtedly the most powerful and flexible, but static web pages also have some advantages: You don't need to know how to code to create a site that uses only static web pages. You would simply need to know HTML/XHTML and CSS, and even this would not be indispensable: you could use a web design programme to generate them. The distinguishing feature of static web pages is that their content never changes, and this can also be an advantage in some cases. This is the case, for example, when you want to store a link to a specific content of the website: if the page is dynamic, when you revisit it using the link, its content may vary from how it was before. Or when you want to submit a site you have created to a search engine such as Google. For Google to display a website in its search results, it first has to index its content. In other words, a programme goes through the pages of the site looking at its content and classifying it. If the pages are generated dynamically, some or all of their content may not be visible to the search engine and therefore will not be indexed. This would never happen on a site using static web pages. As you know, in order for a web server to be able to process a dynamic web page, it needs to execute a programme. This execution is performed by a particular module, which may be integrated into the server or be independent. In addition, it may be necessary to query a database as part of the execution of the program. In other words, the execution of a dynamic web page requires a number of server-side resources. These resources must be installed and maintained. Static web pages only need a web server that communicates with your browser to send it to you. And in fact, to view a static page stored on your computer, you don't even need a web server. They are files that can be stored on a storage medium such as an optical disk or a USB stick and opened from there directly with a web browser. But if you decide to make a website using static pages, be aware that they have limitations. The most important disadvantage has already been mentioned above: updating your content must be done manually by editing the page stored on the web server. This involves maintenance that can be prohibitively expensive for websites with a large amount of content. The first web pages that were created on the Internet were static pages. These web sitess composed of static pages are considered the first generation. The second generation of the web came about thanks to dynamic web pages. Based on the dynamic web, other technologies have emerged that have made the Internet evolve into what we know today. Think about these examples and select the best option, static or dynamic pages: A personal CV A list of personal clothing for sale A personal collection of model kits A website of a greengrocer's shop 1.2. Web applications Web applications use dynamic web pages to create applications that run on a web server and are displayed in a browser. You can find web applications to perform multiple tasks. One of the first to appear were the ones you saw earlier, email clients, which allow you to check incoming email messages and send your own using a browser. Today there are web applications for a multitude of tasks such as word processing, task management, or image editing and storage. These applications have certain advantages and disadvantages compared to traditional applications that run on the machine's own operating system. Name some of them and justify why you think they are web applications. Advantages of web applications: It is not necessary to install them on the computers where they are to be used. They are installed and run only on one computer, on the server, and this is enough for them to be used simultaneously from many computers. As they are only installed on one computer, it is very easy to manage them (make backup copies of your data, correct errors, update them). They can be used on all systems that have a web browser, regardless of their characteristics (a powerful computer is not necessary) or their operating system. They can be used from any place where there is a connection to the server. In many cases this makes it possible to access the applications from non-conventional systems, such as mobile phones. See the following link: The network is the computer Disadvantages of web applications: The user interface of web applications is the page displayed in the browser. This restricts the interface features to those of a web page. We depend on a connection to the server in order to use them. If the connection fails, we cannot access the web application. The information displayed in the browser must be transmitted from the server. This makes certain types of applications unsuitable for implementation as a web application (e.g. applications that handle multimedia content, such as video editing applications). Today, many web applications take advantage of the benefits of dynamic page generation. The vast majority of their content is stored in a database. Applications such as Drupal, Joomla! and many others offer two distinct parts: An external or front-end part, which is the set of pages seen by the vast majority of users who use them (external users). An internal part or back-end, which is another set of dynamic pages used by the people who produce the content and those who administer the web application (internal users) to create content, organise it, decide the external appearance, etc. 1.3. Execution of code on the server and on the client. As we have seen, when your browser requests a web page from a web server, it is possible that before sending it to you, it has to run, by itself or by delegation, some program to obtain it. That program is the one that generates, in part or in whole, the web page that arrives on your computer. In these cases, the code is executed in the web server environment. In addition, when a web page arrives in your browser, it is also possible that it includes some program or fragments of code that must be executed. This code, usually in JavaScript language, will be executed in your browser and, in addition to being able to modify the content of the page, it can also carry out actions such as animating text or objects on the page or checking the data you enter in a form. These two technologies complement each other. Thus, to return to the example of webmail, the programme that is responsible for obtaining your messages and their content from a database is executed in the server environment, while your browser executes, for example, the code responsible for warning you when you want to send a message and you have forgotten to put a text in the subject line. This division is so because the code that runs in the web client (in your browser) does not have, or rather traditionally did not have, access to the data stored on the server. In other words, when you wanted to read a new email in your browser, the Javascript code running in the browser could not obtain the content of that email from the database. The solution was to create a new page on the server with the requested information and send it back to the browser. However, for some years now there has been a web development technique known as AJAX, which allows us to create programmes in which the JavaScript code that is executed in the browser can communicate with an Internet server to obtain information with which, for example, to modify the current web page. In our example, when you click with the mouse on an email you want to read, the page can contain Javascript code that detects the action and, at that moment, consult the text contained in that same email via the Internet and display it on the same page, modifying its structure if necessary. In other words, without leaving a page, you can modify its content based on the information stored on an Internet server. In this module you will learn how to create web applications that run on the server side. Another module in this same cycle, Web Development in a Client Environment, teaches the characteristics of programming code that runs in the web browser. Many of today's web applications use these two technologies: code execution on the server and on the client. Thus, code that runs on the server generates web pages that already include code intended for execution in the browser. Towards the end of this module you will see the techniques used to program applications that incorporate this functionality. Useful links: How PHP Works (1) How PHP Works (2) Think about which of these actions are executed on the server or on the client: Select an item from a drop-down list Click on a button and a new window opens. Mouse over a black and white image to make it turn color or change its size. Display a list of products Calculate and display a mathematical operation 2. Technologies for server-side web programming. When you program an application, you use a programming language. For example, you use the Java language to create applications that run on different operating systems. When programming each application, you use certain tools such as a development environment or code libraries. In addition, once you have finished developing it, that application will need certain components to run, such as a Java virtual machine. In this block you will learn about the different technologies that can be used to program applications that run on a web server, and how they relate to each other. You will see the advantages and disadvantages of using each one, and which programming languages you will need to learn in order to use them. The main components you need to have in order to run web applications on a server are the following: A web server to receive requests from web clients (usually browsers) and send them the page they request (once it has been generated, since we are talking about dynamic web pages). The web server must know the procedure to follow to generate the web page: which module will be in charge of executing the code and how to communicate with it. The module in charge of executing the code or programme and generating the resulting web page. This module must be integrated in some way with the web server, and will depend on the language and technology used to program the web application. A database application, which will normally also be a server. This module is not strictly necessary but in practice it is used in all web applications that use large amounts of data to store them. The programming language you will use to develop the applications. In addition to the components to be used, it is also important to decide how you are going to organise the application code. Many of the architectures used in web application programming help you to structure the application code in layers or tiers. The reason for layering the design of an application is so that the logical functions of the application can be separated, so that it is possible to run each one on a different server (if necessary). In an application you can distinguish, in a general way, presentation functions (which is in charge of formatting the data to present it to the end user), logic (which uses the data to execute a process and obtain a result), persistence (which keeps the data stored in an organised way) and access (which obtains and inserts data into the storage space). Each layer can take care of one or more of the above functions. For example, in 3-layer applications we can find: A client layer, which is where you will program everything related to the user interface, that is, the visible part of the application with which the user will interact. An intermediate layer where you will have to program the functionality of your application. A data access layer, which will be responsible for storing the application's information in a database and retrieving it when necessary. 2.1. Architectures and platforms. The first choice you will make before you start programming a web application is the architecture you are going to use. Nowadays, you can choose between: Java EE (Enterprise Edition), formerly also known as J2EE. It is a platform oriented to the programming of applications in Java language. It can work with different database managers, and includes several libraries and specifications for the development of applications in a modular way. It is supported by large companies such as Sun and Oracle, which maintain Java, and IBM. It is a good solution for the development of medium to large applications. One of its main advantages is the multitude of existing libraries in this language and the large base of programmers who know it. Within this architecture there are different technologies such as JSP pages and servlets, both oriented towards the dynamic generation of web pages, or EJBs, components that normally provide the logic of the web application. AMP. It stands for Apache, MySQL and PHP/Perl/Python. The first two acronyms refer to the web server (Apache) and the database server (MySQL). The last one corresponds to the programming language used, which can be PHP, Perl or Python, PHP being the most used of the three. Depending on the operating system used for the server, the acronyms LAMP (for Linux), WAMP (for Windows) or MAMP (for Mac) are used. It is also possible to use other components, such as the PostgreSQL database manager instead of MySQL. All the components of this architecture are open source. It is a programming platform that allows the development of small or medium-sized applications with a simple learning curve. Its great advantage is the large community that supports it and the multitude of open source applications available. There are software packages that include a complete AMP platform in a single installation. Some do not even need to be installed, and even have versions for different operating systems such as Linux, Windows or Mac. One of the best known is XAMPP. CGI/Perl. It is the combination of two components: Perl, a powerful open source language originally created for server administration, and CGI, a standard to allow the web server to run generic programs, written in any language (you can also use for example C or Python), which return web pages (HTML) as a result of their execution. It is the most primitive of the architectures we compare here. The main drawback of this combination is that CGI is slow, although there are methods to speed it up. On the other hand, Perl is a very powerful language with a large user community and a lot of free code available. ASP.Net is the commercial architecture proposed by Microsoft for the development of applications. It is the part of the.Net platform intended for the generation of dynamic web pages. It comes from the evolution of Microsoft's previous technology, ASP. The programming language can be Visual Basic.Net or C#. The architecture uses Microsoft's web server, IIS, and can obtain information from various database managers including, of course, Microsoft SQL Server. One of the major advantages of the.Net architecture is that it includes everything needed for application development and deployment. For example, it has its own development environment, Visual Studio, although other options are available. The biggest disadvantage is that it is a proprietary commercial platform. 2.2. Selection of a web programming architecture. As you have seen, there are many decisions you have to make before you even start developing a web application. The architecture you will use, the programming language, the development environment, the database manager, the web server, even how you will structure your application. To make the right decision, you should consider the following points, among others: How big is the project? What programming languages do I know, and is it worth the effort to learn a new one? Will I use open source or proprietary tools? What is the cost of using commercial solutions? Will I be programming the application on my own or will I be part of a group of programmers? Do I have a web server or database manager available or can I freely decide to use the one I think I need? What type of licence will I apply to the application I develop? By studying the answers to these and other questions, you will be able to see which architectures are best suited to your application and which are not viable. 2.3. Integration with the web server As you know, the communication between a web client or browser and a web server is carried out thanks to the HTTP protocol. In the case of web applications, HTTP is the link between the user and the application itself. Any input of information by the user is transmitted via an HTTP request, and the result is delivered to the user in the form of an HTTP response. On the server side, these requests are processed by the web server (also called HTTP server). It is therefore up to the web server to decide how to process the requests it receives. Each of the architectures we have just seen has a way of integrating with the web server to execute the application code. The oldest technology is CGI. CGI is a standard protocol that exists on many platforms. It is implemented by the vast majority of web servers. It defines what the web server must do to delegate the generation of a web page to an external program. These external programs are known as CGI scripts, regardless of the language in which they are programmed (although they are usually programmed in scripting languages such as Perl). The main problem with CGI is that every time a CGI script is executed, the operating system must create a new process. This results in higher resource consumption and slower execution speed. There are some solutions that speed up the execution, such as FastCGI, and also other methods to execute scripts in a web server environment, for example the mod_perl module to execute scripts programmed in Perl in Apache. Although it is also possible to execute code in PHP language using CGI, PHP interpreters are not usually used with this method. Like mod_perl, there are other modules that can be installed on the Apache web server to run dynamic web pages. The mod_php module is the usual form used to run PHP scripts using AMP platforms, and its equivalent for the Python language is mod_python. The Java EE architecture is more complex. In order to run Java EE applications on a server we basically have two options: application servers, which implement all the technologies available in Java EE, and servlet containers, which support only part of the specification. Depending on the size of our application and the technologies it uses, we will have to install one solution or the other. There are several implementations of certified Java EE application servers. The two most widely used commercial solutions are IBM Websphere and BEA Weblogic. There are also open source solutions such as JBoss, Geronimo (from the Apache Foundation) or Glassfish. However, in most cases it is not necessary to use a complete application server, especially if we do not use EJB in our applications, but a servlet container will suffice. In this area, Tomcat, the reference implementation of a servlet container, which is also open source, stands out. Once the solution we have chosen is installed, we have to integrate it with the web server we use, so that it recognizes requests for servlets and JSP pages and redirects them. Another option is to use a single solution for static pages and dynamic pages. For example, the Tomcat servlet container includes its own HTTP server that can replace Apache. The ASP.Net architecture uses Microsoft's IIS server, which already integrates support in the form of modules for handling ASP and ASP.Net dynamic page requests. The web server administration utility includes functions for managing the web applications installed on the server. 3. Languages One of the most notable differences between one web programming language and another is the way they are executed on the web server.You must distinguish three main groups: Scripting languages (scripting). These are those in which the programs are executed directly from their original source code.They are usually stored in a plain text file. When the web server needs to execute code programmed in a scripting language, it passes the request to an interpreter, which processes the program lines and generates a web page as a result. Of the languages you studied earlier, Perl, Python, PHP and ASP (the precursor to ASP.Net) belong to this group. Languages compiled to native code. These are those in which the source code is translated into binary code, dependent on the processor, before being executed. The web server stores the programs in their binary mode, which it executes directly when invoked. The main method for running binary programs from a web server is CGI. Using CGI we can make the web server execute code programmed in any general purpose language such as C. Languages compiled to intermediate code.These are languages in which the original source code is translated into an intermediate code, independent of the processor, before being executed.This is the way in which applications programmed in Java, for example, are executed, and what makes them run on several different platforms. In web programming, the languages of the Java EE (servlets and JSP pages) and ASP.Net architectures operate in this way. In the ASP.Net platform and in many Java EE implementations, a JIT compilation procedure is used. This term refers to the way intermediate code is converted to binary code for execution by the processor. To speed up execution, the compiler can translate all or part of the intermediate code to native code when a program is invoked. The native code obtained is usually stored to be used again when needed. Each of these ways of code execution by the web server has its advantages and disadvantages: Scripting languages have the advantage that it is not necessary to translate the original source code to be executed, which increases their portability. If any modifications need to be made to a program, they can be done on the fly.On the other hand, the interpretation process offers worse performance than the other alternatives. Languages compiled to native code have the fastest execution speed, but have problems in terms of integration with the web server. They are general-purpose programs that are not intended to run in a web server environment. For example, processes are not reused to handle multiple requests: for each request made to the web server, a new process must be executed.In addition, programs are not portable between different platforms. Languages compiled to intermediate code offer a balance between the two previous options. Their performance is very good and they can be ported between different platforms where there is an implementation of the architecture (such as a servlet container or a Java EE application server). 3.1. Embedded code in markup language When the web began to evolve from static to dynamic web pages, one of the first technologies used was code execution using CGI. CGI scripts are standard programs, which are executed by the operating system, but output the HTML code of a web page. Therefore, CGI scripts must contain, mixed within their code, statements responsible for generating the web page. For example, if you want to generate a web page using CGI from a statement script in Linux, you have to do something like the following Hello World example: File: /var/www/cgi-bin/hello.sh #!/bin/bash echo "Content-type: text/html" echo "" echo '' echo '' echo '' echo 'Hello World' echo '' echo '' echo 'Hello World' echo '' echo '' exit 0 A Perl example #!/usr/bin/perl print "Content-type: text/html\n\n"; print. The code is executed by an execution environment with which the web server is integrated (usually using Apache with the mod_php module). The configuration of both the Apache web server and PHP is done through configuration files. The Apache one is httpd.conf, and the PHP one is php.ini. This file, php.ini, can be found in different locations. The phpinfo() function you ran earlier tells you, among many other things, where the php.ini file is stored on your computer. In our case it is in /etc/php5/apache2/php.ini. Depending on how PHP is integrated with Apache, the changes you make to its configuration file will be applied at one time or another. If, as in our case, we use mod_php to run PHP as an Apache module, the PHP configuration options will be applied every time Apache is restarted. So don't forget to do it every time you make changes to php.ini. For example: sudo /etc/init.d/apache2 restart or sudo service apache2 restart. Some of the most commonly used directives contained in the php.ini file are: short_open_tag. Indicates whether short delimiters can be used in PHP. It is preferable not to use them, because it can cause problems if we use pages with XML. To prohibit the use of these delimiters with PHP, set this directive to Off. max_execution_time. Allows you to set the maximum number of seconds that the execution of a PHP script can last. Prevents the server from crashing if an error occurs in a script. error_reporting. Specifies what kind of errors will be displayed in case they occur. For example, if you make error_reporting = E_ALL, it will show you all types of errors. If you do not want it to show warnings but other types of errors, you can do error_reporting = E_ALL & ~E_NOTICE. file_uploads. Indicates whether or not files can be uploaded to the server via HTTP. upload_max_filesize. In case files can be uploaded over HTTP, you can specify the maximum allowed limit for the size of each file. For example, upload_max_filesize = 1M. We will see other directives throughout the module as we need them. You should know A complete list of directives that can be used in php.ini is included in the PHP documentation. https://www.php.net/manual/en/ini.list.php 4. PHP language features As you write code in PHP, it will be useful to insert some comments in the code to help you review it when you need it. In a web page, comments to the HTML go between the delimiters. Within the PHP code, there are three ways to put comments: One-line comments using //. These are C-style comments. When a line begins with // symbols, the whole line is considered to contain a comment, until the next line. One-line comments using #. They are similar to the previous ones, but using Linux scripting syntax. Multi-line comments. Also the same as in the C language. When the. Remember that when you add comments to PHP code, they will not appear in any case in the web page that is sent to the browser (just the opposite of what happens with comments to HTML tags). 4.1. PHP language elements 4.1.1. Variables and data types in PHP As in all programming languages, in PHP you can create variables to store values. Variables in PHP must always begin with a $ sign. Variable names must begin with letters or the _ character, and can also contain numbers. However, unlike in many other languages, in PHP you do not need to declare a variable or specify a specific type (integer, string,...). To start using a variable, simply assign it a value using the = operator. $my_variable = 7; Depending on the value assigned to it, the variable is given a data type, which may change if its contents change. That is, the type of the variable is decided by the context in which it is used. // When assigned the value 7, the variable is of type "integer". $my_variable = 7; // If we change the content $my_variable = "seven"; // The variable can change type // In this case it becomes of type "string". The simple data types in PHP are: boolean. Their possible values are true and false. Also, any integer is considered true, except for 0 which is false. integer. Any number without decimal places. They can be represented in decimal, octal (starting with a 0), or hexadecimal (starting with 0x) format. real (float). Any number with decimals. They can also be represented in scientific notation. string. Sets of characters delimited by single or double quotation marks. null. A special data type, used to indicate that the variable has no value. Examples: $my_boolean = false; $my_integer = 0x2A; $my_float = 7.3e-1; $my_string = “text"; $mi_variable = null; If you perform an operation with variables of different types, both are first converted to a common type. For example, if you add an integer with a real, the integer is converted to real before the addition is performed: $my_integer = 3; $my_real = 2.3; $result = $my_integer + $my_actual; // The variable $result is of type real. These type conversions, which in the example above are performed automatically, can also be performed by force: $my_integer = 3; $my_actual = 2.3; $result = $my_whole + (int) $my_actual; // The variable $my_actual is converted to an integer (value 2) before being added. // The variable $result is of type integer (value 5). You should know The PHP documentation specifies the possible type conversions and the results obtained with each one: https://www.php.net/manual/en/language.types.type-juggling.php#language.types.typecast ing 4.1.2. Scope of variable use In PHP you can use variables anywhere in a program. If such a variable does not already exist, the first time it is used, space is reserved for it. At that point, depending on where in the code it appears, it is decided from which parts of the program the variable can be used. This is called variable visibility. If the variable first appears within a function, it is said that the variable is local to the function. If an assignment appears outside the function, it will be considered a distinct variable. For example: $a = 1; function test() { $b = $a; // Therefore, the variable $a used in the previous assignment is a new variable // which has no value assigned to it (its value is null). } If in the above function you wanted to use the external $a variable, you could do so by using the global word. This way you tell PHP not to create a new local variable, but to use the existing one. $a = 1; function test(){ global $a; $b = $a; // In this case $b is assigned the value 1 } Local variables to a function disappear when the function ends and their value is lost. If you want to keep the value of a local variable between function calls, you must declare the variable as static using the word static. function counter() { static $a=0; $a++; // Each time the function is executed, the value of $a is incremented. } Static variables must be initialised in the same statement in which they are declared as static. This way, they are initialised only the first time the function is called. You should know More examples of variable scopes can be found in the PHP documentation. https://www.php.net/manual/en/language.variables.scope.php 4.1.3. Special PHP variables In a previous paragraph you learned what global variables are and how they are used. PHP includes a few predefined internal variables that can be used from any scope, so they are called superglobal variables. You don't even need to use global to access them. Each of these variables is an array containing a set of values (we will see later in this unit how arrays can be used). The superglobal variables available in PHP are the following: $_SERVER. Contains information about the web server and execution environment. Among the information provided by this variable, we have: Main values of the variable $_SERVER Value Content $_SERVER['PHP_SELF'] script that is currently running. $_SERVER['SERVER_ADDR'] IP address of the web server. $_SERVER['SERVER_NAME'] name of the web server. $_SERVER['DOCUMENT_ROOT'] root directory under which the current script is running. $_SERVER['REMOTE_ADDR'] IP address from which the user is viewing the page. $_SERVER['REQUEST_METHOD'] method used to access the page ('GET', 'HEAD', 'POST' or 'PUT') You should know All the information provided by $_SERVER can be found in the PHP documentation: https://www.php.net/manual/en/reserved.variables.server.php $_GET, $_POST and $_COOKIE contain the variables passed to the current script using respectively the GET (parameters in the URL), HTTP POST and HTTP Cookies methods. $_REQUEST joins the contents of the three previous arrays, $_GET, $_POST and $_COOKIE, into one. $_ENV contains any variables that may have been passed to PHP from the running environment. $_FILES contains any files that may have been uploaded to the server using the POST method. $_SESSION contains the session variables available for the current script. We will be working with these variables in later units. You should know It is useful to have at hand the information about these superglobal variables available in the PHP manual. https://www.php.net/manual/en/language.variables.superglobals.php 4.1.4. Expressions and operators As in many other languages, expressions are used in PHP to perform actions within a program. All expressions must contain at least one operand and one operator. For example: $my_variable = 7; $a = $b + $c; $value++; $x += 5; The operators you can use in PHP are similar to those in many other languages such as Java. They include operators for: Perform arithmetic operations: negation, addition, subtraction, multiplication, division and modulo. These include pre and post increment and decrement operators, ++ and --. These operators increment or decrement the value of the operand to which they are applied. When used in conjunction with an assignment expression, they modify the operand before or after the assignment depending on its position (before or after) with respect to the operand. For example: $a = 5; $b = ++$a; // Before one is added to $a (it becomes a value of 6) // and then it is assigned to $b (which also ends up with value 6). $a = 5; $b = $a++; // First $b is assigned the value of $a (5). // and then add one to $a (it becomes a value of 6). Performing assignments. In addition to the = operator, there are operators with which to perform operations and assignments in a single step (+=, -=,...). Compare operands. In addition to those that we can find in other languages (>, >=,...), in PHP we have two operators to check equality (==, ===) and three to check difference (, != and !==). The operators and != are equivalent. They compare the values of the operands. The === operator returns true only if the operands are of the same type and also have the same value. The !== operator returns true if the values of the operands are different or if the operands are not of the same type. For example: $x = 0; // The expression $x == false is true (returns true). // However, $x === false is not true (it returns false) because $x is an integer, not a boolean. Compare boolean expressions. Treat operands as boolean variables (true or false). There are operators to perform logical AND (and or && operators), logical OR (or or or || operators), non-logical (! operator) and exclusive logical OR (xor operator). Perform operations on the bits that make up an integer: invert them, shift them, etc. You should know In the PHP documentation, all the operators available in the language and how they are used are explained in detail: https://www.php.net/manual/en/language.operators.php 4.1.5. HTML code generation There are several ways to include content in the web page from the result of executing PHP code. The simplest way is to use echo, which returns nothing (void), and generates as output the text of the parameters it receives. void echo (string $arg1,...); Another possibility is print, which works in a similar way. The most important difference between print and echo is that print can only receive one parameter and always returns 1. int print (string $arg); Both print and echo are not really functions, so you are not required to use parentheses when using them. For example, the code in the following document can still be done using echo. Using the print function in PHP: Desarrollo Web printf is another option to generate output from PHP. It can take several parameters, the first of which is always a string indicating the format to be applied. That string must contain a conversion specifier for each of the other parameters passed to the function, and in the same order as they appear in the parameter list. For example: Each conversion specifier is preceded by the % character and consists of the following parts: sign (optional). Indicates whether negative numbers are signed (default) or positive numbers are signed (indicated by a + sign). padding (optional). Indicates which character will be used to adjust the size of a string. The options are the 0 character or the space character (space is used by default). alignment (optional). Indicates which type of alignment will be used to generate the output: right-justified (default) or left-justified (indicated by the - character). width (optional). Indicates the minimum number of output characters for a given parameter. precision (optional). Indicates the number of decimal digits to be displayed for a real number. It is written as a digit preceded by a full stop. type (mandatory). Indicates how the value of the corresponding parameter is to be treated. In the following table you can see a list of all type specifiers. Type specifiers for the printf and sprintf functions. Specifier Meaning B argument is treated as an integer and presented as a binary number. c the argument is treated as an integer, and displayed as the character with that ASCII value. d the argument is treated as an integer and displayed as a decimal number. u the argument is treated as an integer and displayed as an unsigned decimal number. o the argument is treated as an integer and presented as an octal number. x the argument is treated as an integer and presented as a hexadecimal number (with lower case). X argument is treated as an integer and presented as a hexadecimal number (with capital letters). f the argument is treated as a double and presented as a floating point number (taking locality into account). F the argument is treated as a double and presented as a floating point number (regardless of locale). e the argument is presented in scientific notation, using lower case e (for example, 1.2e+3). E the argument is presented in scientific notation, using capital e (e.g. 1.2E+3). g the shortest form between %f and %e is used. G the shortest form between %f and %E is used. s the argument is treated as a string and presented as such. % the % character is displayed. No argument is needed. For example, when executing the following line, the output is the signed PI number with only two decimal places. printf("The number PI is %+.2f", 3.1416); // +3.14 There is a function similar to printf but instead of generating an output with the obtained string, it allows saving it in a variable: sprintf. $txt_pi = sprintf("The number PI is %+.2f", 3.1416); 4.1.6. Text strings In PHP, text strings can use either single quotes or double quotes. However, there is an important difference between using one or the other. When a variable is put inside double quotes, it is processed and replaced by its value. Thus, the example above about the use of print could also have been put in the following way: The variable $course is recognised inside the double quotes, and substituted with the value "DWES" before generating the output. If you had done the same thing using single quotes, no substitution would be made. In order for PHP to correctly distinguish the text that forms the string from the variable name, it is sometimes necessary to enclose it in braces. print "Module: ${module}" When single quotes are used, only two substitutions are made within the string: when the sequence of characters \' is encountered, a single quote is displayed in the output; and when the sequence \\ is encountered, a backslash is displayed in the output. These sequences are known as escape sequences. In strings that use double inverted commas, in addition to the sequence \\, some other sequences can be used, but not the sequence \'. In this table you can see which escape sequences can be used, and what their result is. Escape sequences: Sequence Result \\ a backslash is displayed. \' a single quotation mark is displayed. \" a double quotation mark is displayed. \n a line feed (LF or 0x0A (10) in ASCII) is displayed. \r a carriage return (CR or 0x0D (13) in ASCII) is displayed. \t a horizontal tab (HT or 0x09 (9) in ASCII) is displayed. NOTE: HTML will convert the tab to a space unless it is placed between and tags. e.g.: print " test test"; \v a vertical tab (VT or 0x0B (11) in ASCII) is displayed. \f a page feed (FF or 0x0C (12) in ASCII) is displayed. \$ a dollar sign is displayed. In PHP you have two unique operators for working with strings. With the dot concatenation operator (.) you can join the two strings you pass as operands. The assignment and concatenation operator (.=) concatenates the left-hand side argument to the right-hand side string. In PHP you have another alternative to create strings: the heredoc syntax. It consists of putting the operator The result of the above code is: $a is 3.1416 and is of type string. $b is 3.1416 and is of type double If all you are interested in is whether a variable is defined and is not null, you can use the isset function. The unset function destroys the variable or variables passed as a parameter. Only the following types of values are allowed for constants: integer, float, string, boolean and null. You should know String-related functions https://www.php.net/manual/en/ref.strings.php In PHP there is no specific data type for working with dates and times. The date and time information is stored internally as an integer. However, within the PHP functions you have at your disposal a few functions to work with that type of data. One of the most useful is perhaps the date function, which allows you to get a text string from a date and time, in a format of your choice. The function takes two parameters, the format description and the integer identifying the date, and returns a formatted string. string date (string $format [, int $datetime]); The format must be composed using as a basis a series of characters from the following table. Date function: format characters for dates and times. Character Result d two-digit day of the month j day of the month with one or two digits (without leading zeros). z day of the year, starting with zero ( 0 = 1 January ). N day of the week ( 1 = Monday,..., 7 = Sunday ). w day of the week ( 0 = Sunday,..., 6 = Saturday ). l text of the day of the week ( Monday,..., Sunday ). D text of the day of the week, only three letters, in English ( Mon,..., Sun ). W number of the week of the year. m number of the month with two digits. n number of the month with one or two digits (without leading zeros). t number of days in the month. F text of the day of the month, in English ( January,..., December ). M text of the day of the month, only three letters, in English ( Jan,..., Dec ). Y number of the year. y last two digits of the year number. L 1 if the year is a leap year, 0 if it is not. h 12-hour format, always with two digits. H 24-hour format, always with two digits. g 12-hour format, with one or two digits (without leading zeros). G 24-hour format, with one or two digits (without leading zeros). i minutes, always with two digits. s seconds, always with two digits. u microseconds. a am or pm, in lower case. A AM or PM, in upper case. r whole date in RFC 2822 format. In addition, the second parameter is optional. If not specified, the current time will be used to create the string. Example: In order for the system to give you information about your date and time, you must tell it your timezone. You can do this with the date_default_timezone_set function. To set the timezone in Spain peninsular you must indicate: date_default_timezone_set('Europe/Madrid'); You should know All the functions for managing dates and times can be found in the PHP documentation https://www.php.net/manual/en/ref.datetime.php 4.2. Control structures In PHP, scripts are constructed on a statement basis. Using curly braces, you can group statements into sets, which behave as if they were a single statement. To define the flow of a program in PHP, as in most programming languages, there are statements for two types of control structures: conditional statements, which allow you to define the conditions under which a statement or block of statements should be executed; and loop statements, with which you can define whether a statement or set of statements is repeated or not, and under what conditions. In addition, in PHP you can also use (although it is not recommended) the goto statement, which allows you to jump directly to another point in the program that you indicate by means of a tag. 4.2.1. Conditionals The if statement allows you to define an expression to execute or not to execute the next statement or set of statements. If the expression evaluates to true, the statement is executed. If it evaluates to false, it will not be executed. When the result of the expression is false, you can use else to indicate a statement or set of statements to execute in that case. Another alternative to else is to use elseif and write a new expression that will start a new conditional. When, as in the example, the if, elseif or else statement acts on a single statement, it is not necessary to use braces. You will have to use braces to form a set of statements whenever you want the conditional to act on more than one statement. switch. The switch statement is similar to linking several if statements comparing the same variable with different values. Each value goes into a case statement. When a match is found, subsequent statements begin executing until the switch block ends, or until a break statement is found. If there is no match with the value of any case, the statements of the default block are executed, if any. Exercise 1 Make a web page that displays the current date in Spanish, including the day of the week, in a format similar to the following: "Miércoles, 14 de Octubre de 2015". 4.2.2. Loops while: Using while you can define a loop that runs as long as an expression is true. The expression is evaluated before starting each execution of the loop. do / while: This is a loop similar to the previous one, but the expression is evaluated at the end, thus ensuring that the statement or set of statements in the loop is executed at least once. for: These are the most complex loops in PHP. Like those of the C language, they are made up of three expressions: for (expr1; expr2; expr3) statement or set of statements; The first expression, expr1, is executed only once at the beginning of the loop. The second expression, expr2, is evaluated to see if the statement or set of statements should be executed or not. If the result is false, the loop terminates. If the result is true, the statements are executed and at the end the third expression, expr3, is executed and expr2 is evaluated again to decide whether or not to re-execute the loop. You can nest any of the above loops at various levels. You can also use the statements break, to exit the loop, and continue, to skip the execution of the remaining statements and return to expression checking respectively. 4.3. Functions When you want to repeat the execution of a block of code, you can use a loop. Functions have a similar utility: they allow us to associate a label (the name of the function) with a block of code to be executed. In addition, by using functions we are helping to better structure the code. As you know, functions allow you to create local variables that will not be visible outside the function body. As a programmer you can take advantage of the large number of functions available in PHP. Of these, many are included in the PHP core and can be used directly. Many others are available as extensions, and can be incorporated into the language when needed. Several extensions are included with the PHP distribution. In order to use the functions of an extension, you have to make sure to enable it by using an extension directive in the php.ini file. Many other extensions are not included with PHP and must be downloaded before you can use them. To get extensions for the PHP language you can use PECL. PECL is a repository of PHP extensions. Included with PHP is a pecl command that you can use to easily install extensions: pecl install extension_name You should know More information about PECL can be found in the PHP manual. https://www.php.net/manual/en/install.pecl.php 4.3.1. Inclusion of external files As the programs you make grow, you will find that finding the information you are looking for within the code becomes more and more difficult. Sometimes it is useful to group certain groups of functions or blocks of code together, and put them in a separate file. You can then reference those files so that PHP includes their contents as part of the actual program. To incorporate content from an external file into your program, you have several possibilities: include: Evaluates the contents of the file you specify and includes it as part of the current file, at the same point where the call is made. The location of the file can be specified using an absolute path, but the most usual is with a relative path. In this case, the path specified in the include_path directive of the php.ini file is used as the basis. If it is not found in that location, it will also be searched in the current script directory, and in the execution directory. definitions.php: When you start evaluating the content of the external file, the PHP mode is automatically exited and its content is treated in principle as HTML tags. For this reason, it is necessary to mark the beginning of the PHP code that will contain our external file by using the delimiter since it can confuse the closing and then on return from the original file from where it was called not to reopen. It is also recommended that all text files are UTF-8 encoded and end with a blank line, the latter avoids confusion in GIT when they are modified. include_once: If you mistakenly include the same file more than once, you will usually get some kind of error (for example, when repeating a function definition). include_once works exactly like include, but only includes those files that have not yet been included. require: If the file you want to include is not found, include gives a warning and continues executing the script. The most important difference when using require is that in this case, when the file cannot be included, the execution of the script stops. require_once. This is a combination of the previous two. It ensures the inclusion of the specified file only once, and generates an error if it cannot be done. Many programmers use the double extension.inc.php for PHP language files that are intended to be included within other files, and are never to be executed by themselves. 4.3.2. Execution and creation of functions You know that to call a function, you just have to put its name and some parenthesis. To create your own functions, you must use the keyword function. In PHP you do not need to define a function before using it, except when it is conditionally defined as shown in the following example: When a function is defined in a conditional way its definitions must be processed before being called. Therefore, the function definition must be before any call. You should know Functions https://www.php.net/manual/en/language.functions.php 4.3.3. Arguments In the previous example you used a global variable in the function, which is not a good practice. It is always better to use arguments or parameters when making the call. Also, instead of displaying the result on the screen or storing the result in a global variable, functions can return a value using the return statement. When a function encounters a return statement, it terminates its processing and returns the value you specify. You can rewrite the above function as follows: The arguments are indicated in the function definition as a comma-separated list of variables. The type of each argument is not specified, just as it is not specified whether or not the function will return a value (if a function does not have a return statement, it returns null at the end of its processing). When defining the function, you can specify default values for the arguments, so that when you call the function you can not specify the value of an argument; in this case the default value is taken. There may be default values defined for several arguments, but in the function's argument list they must all be to the right of any non-default arguments. In the previous examples the arguments were passed by value. That is, any changes made inside the function to the argument values will not be reflected outside the function. If you want this to happen you must define the parameter so that its value is passed by reference, by adding the & symbol before its name. You should know When passing arguments by reference, there is no reference sign in a function call - only in the function definition. Function definitions alone are sufficient to correctly pass the argument by reference. As of PHP 5.3.0, you will get a warning saying that "call-time pass-by-reference" is deprecated when using & in foo(&$a);. As of PHP 5.4.0, call-time pass-by-reference has been removed, so using it will raise a fatal error. https://www.php.net/manual/en/language.references.pass.php Exercise 2 Earlier you did an exercise that showed the current date in English. For the same purpose (you can use the code already done), create a function that returns a string with the date in Spanish, and insert the function in an external file. Then create a PHP page that includes that file and uses the function to display the date obtained. 4.4. Compound data types A compound data type is one that allows you to store more than one value. In PHP you can use two compound data types: the array and the object. We will see objects later; let's start with arrays. An array is a data type that allows you to store multiple values. Each member of the array is stored in a position that is referenced using a key value. Keys can be numeric or associative. // numerical array $modules1 = array(0 => "Programming", 1 => "Databases",..., 9 => "Server-side web development"); // associative array $modules2 = array("PR" => "Programming", "DB" => "Databases",..., "DWES" => "Web development in server environment"); You should know In PHP there is the function print_r, which shows us all the contents of the array we pass it. It is very useful for debugging tasks. https://www.php.net/manual/en/function.print-r.php To refer to the elements stored in an array, you have to use the key value in square brackets: $modules1 $modules2 ["DWES"] The above arrays are vectors, that is, one-dimensional arrays. In PHP you can also create multi-dimensional arrays by storing another array in each of the elements of an array. // two-dimensional array $cycles = array( "DAW" => array ("PR" => "Programming", "DB" => "Databases",..., "DWES" => "Server-side web development"), "DAM" => array ("PR" => "Programming", "DB" => "Databases",..., "PMDM" => "Multimedia and Mobile Devices Programming") ); To refer to elements stored in a multidimensional array, you must indicate the keys for each of the dimensions: $cycles ["DAW"] ["DWES"] In PHP you do not need to specify the size of the array before creating it. You don't even need to indicate that a particular variable is an array. You can simply start assigning values to it: // numeric array $modules1 = "Programming"; $modules1 = "Databases";... $modules1 = "Web development in server environment"; // associative array $modules2 ["PR"] = "Programming"; $modules2 ["DB"] = "Databases";... $modules2 ["DB"] = "Databases";... $modules2 ["DWES"] = "Web development in server environment"; You don't even need to specify the key value. If you omit it, the array will be filled from the last existing numeric key, or from position 0 if none exists: $modules1 [ ] = "Programming"; $modules1 [ ] = "Databases";... $modules1 [ ] = "Web development in server environment"; You should know Arrays https://www.php.net/manual/en/book.array.php 4.4.1. Traversing arrays (I) Text strings can be treated as arrays in which one letter is stored in each position, with 0 being the index for the first letter, 1 for the second, etc. // text string module = "Server-side web development"; // $module == "a"; To go through the elements of an array, in PHP you can use a specific loop: foreach. It uses a temporary variable to assign at each iteration the value of each of the elements of the array. You can use it in two ways. Traversing only the elements: $modules = array("PR" => "Programming", "DB" => "Databases",..., "DWES" => "Server-side web development"); foreach ($modules as $module) { print "Module: ".$module. "" } Or by traversing the elements and their key values simultaneously: $modules = array("PR" => "Programming", "DB" => "Databases",..., "DWES" => "Web development in server environment"); foreach ($modules as $code=> $module) { print " The code of module ".$module." is ".$code."" } Exercise Make a PHP page that uses foreach to display all the values of the $_SERVER array in a table with two columns. The first column should contain the variable name, and the second column its value. 4.4.2. Traversing arrays (II) But in PHP there is also another way to traverse the values of an array. Each array maintains an internal pointer, which can be used for this purpose. Using specific functions, we can move forward, backward or initialise the pointer, as well as retrieve the values of the element (or of the key/element pair) to which the pointer points at any given moment. Some of these functions are: Functions for traversing arrays. (some are deprecated) Function Result reset Sets the internal pointer to the beginning of the array. next Moves the internal pointer one position forward. prev Moves the internal pointer back one position. end Moves the internal pointer to the end of the array. current Returns the element at the current position. key Returns the key of the current position. each Returns an array containing the key and the element of the current position. In addition, it advances the internal pointer by one position. The reset, next, prev and end functions, in addition to moving the internal pointer, return, like current, the value of the new element where it is positioned. If you move the pointer outside the limits of the array (for example, if you are already on the last element and you call next), any of them returns false. However, by checking this return value you will not be able to distinguish whether you have moved out of bounds, or whether you are in a valid array position that contains the value "false". The key function returns null if the internal pointer is outside the array bounds. each function returns an array with four elements. Elements 0 and 'key' store the value of the key at the current position of the internal pointer. Elements 1 and 'value' return the stored value. If the array's internal pointer has gone beyond the bounds of the array, the each function returns false, so you can use it to create a loop that traverses the array as follows: while ($module = each($modules)) { print "The module code ".$module." is ".$module."" } Exercise Make a PHP page that uses these functions to create a table like the one in the previous exercise. 4.4.3. Functions related to compound data types In addition to assigning values directly, the array function allows you to create an array with a single line of code, as we saw earlier. This function receives a set of parameters, and creates an array from the values passed to it. If no key value is given in the parameters, it creates a numeric array (with base 0). If no parameters are passed, it creates an empty array. $a = array(); // empty array $modules = array("Programming", "Databases",..., "Server-side web development"); // numeric array Once an array is defined, you can add new elements (by not defining the index, or by using a new index) and modify existing ones (using the index of the element to be modified). You can also remove elements from an array using the unset function. In the case of numeric arrays, removing an element means that the keys of the element will no longer be consecutive. unset ($modules ); // The first element becomes $modules == "Databases"; The array_values function takes an array as a parameter, and returns a new array with the same elements and consecutive numeric indices with base 0. To check if a variable is an array, use the is_array function. To get the number of elements contained in an array, you have the function count. If you want to search for a specific element within an array, you can use the in_array function. It receives as parameters the element to search for and the array variable to search in, and returns true if it found the element or false otherwise. $modules = array( "Programming", "Databases", "Server-side WEB Development"); $module = "Databases"; if (in_array($module, $modules)) printf "Exists module named ".$module; Another possibility is the array_search function, which receives the same parameters but returns the key corresponding to the element, or false if it does not find it. And if you want to search for a key in an array, you have the function array_key_exists, which returns true or false. You should know Actually in PHP there are many functions to manage arrays. A complete list can be found in the PHP online manual. https://www.php.net/manual/en/ref.array.php 4.5. Web forms The natural way to get user data from a browser to the web application is to use HTML forms. HTML forms are always enclosed within the tags. Within a form are the elements on which the user can act, mainly using the labels , , y. The action attribute of the FORM element indicates the page to which the form data will be sent. In our case it will be a PHP script. On the other hand, the method attribute specifies the method used to send the information. This attribute can have two values: get: with this method the form data is added to the URI using a question mark "?" as a separator. post: con este método los datos se incluyen en el cuerpo del formulario y se envían utilizando el protocolo HTTP. As we will see, data will be collected in different ways depending on how it is sent. You should know To avoid problems when programming in PHP, you must know the HTML language, specifically the details relating to the creation of web forms. https://www.programiz.com/html/form You have a description of HTML form elements in section 4.5 Exercise Create an HTML form to enter the name of the student and the module he/she is taking, choosing between "Web Development in Server Environment" and "Web Development in Client Environment". Send the result to the page "process.php", which will be in charge of processing the data. WEB form Name: Modules: Server Side WEB Development Client Side WEB Development Note that if in a web form you have to submit a variable in which it is possible to store more than one value, as is the case of the checkboxes in the example above (you can check several at once), you will have to put square brackets around the variable name to indicate that it is an array. The action attribute of the form can be left empty and has the same effect as , i.e. it is sent to the same page from which the form was called. In the old days (before HTML5) this was also done with action="#". Now, even if you don't put action, by default it also sends you to the same page from which the form was called. You should know Sending form data (https://developer.mozilla.org) https://developer.mozilla.org/en-US/docs/Learn/HTML/Forms/Sending_and_retrieving_for m_data 4.5.1. Processing of the information returned by a web form In the example above you created a form in an HTML page that collected data from the user and sent it to a PHP page for processing. Since you used the POST method, the data can be collected using the $_POST variable. If you simply wanted to display it on the screen, this could be the code for "process.php": Web Development If you had used the GET method instead, the code needed to process the data would be similar; you would simply need to change the $_POST variable to $_GET. Web Development In either case you could have used $_REQUEST replacing $_POST and $_GET respectively. Web Development Whenever possible, it is preferable to validate the data entered in the browser before sending it. To do this you should use Javascript code. If for some reason there is data that needs to be validated on the server, for example because you need to check that a user's data does not already exist in the database before entering it, it will be necessary to do this with PHP code in the page that appears in the action attribute of the form. In this case, one possibility to consider is to use the same page that displays the form as the destination for the data. If the data is correct after checking the data, it is forwarded to another page. If they are incorrect, you fill in the correct data in the form and indicate which data are incorrect and why. To do it this way, you have to check if the page receives data (you have to show it and not generate the form), or if it does not receive data (you have to show the form). This can be done by using the isset function with a variable that should be received (for example, by naming the submit button and checking it). The following example code shows how to do this. Web Development