High-Level Programming I - Program Development Process PDF
Document Details
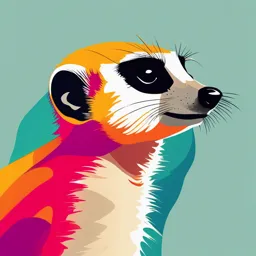
Uploaded by JubilantVulture4763
Prasanna Ghali
Tags
Summary
This presentation covers the program development process, including problem-solving, and implementation phases, along with algorithms for calculating x^y. It provides examples and details about each step of the process.
Full Transcript
HIGH-LEVEL PROGRAMMING I Program Development Process by Prasanna Ghali Outline 2 Program development process Six steps of problem-solving phase Implementation phase Program Development Process Problem-solving phase 3 Problem Steps 1-2: Analyze Problem Steps 3-5: Devise Algorithm St...
HIGH-LEVEL PROGRAMMING I Program Development Process by Prasanna Ghali Outline 2 Program development process Six steps of problem-solving phase Implementation phase Program Development Process Problem-solving phase 3 Problem Steps 1-2: Analyze Problem Steps 3-5: Devise Algorithm Step 6: Trace Algorithm Incorrect algorithm Incorrect implementation Step 3: Debug Incorrect program Step 2: Test Step 1: Code Correct program Implementation phase Working Program Problem-Solving Phase 4 1. 2. 3. 4. 5. 6. Understand problem clearly Describe inputs and outputs Work problem by hand for simple data set Decompose solution into step-by-step details Generalize steps into algorithm Test algorithm with broader variety of data Algorithm for 𝑦 𝑥 (1/8) 5 Step 1: Understand problem clearly 𝑥 = 2, 𝑦 = 3, then 23 = 8 4 If 𝑥 = 3, 𝑦 = 4, then 3 = 81 If Step 2: Describe input and output clearly Inputs are integer values, output is integer value 𝒙 𝒚 𝒙𝒚 Algorithm for 𝑦 𝑥 (2/8) 6 Step 3: Work the problem by hand for simple data set Set 𝑥 = 3, 𝑦 = 4 Multiply 3 by 3 You get 9 Multiply You get 27 Multiply You 4 3 3 by 9 3 by 27 get 81 is 81 Algorithm for 𝑦 𝑥 (3/8) 7 Step 4: Decompose solution into step-by-step details Each step must be precise Nothing is left to guesswork Algorithm for 𝑦 𝑥 (4/8) 8 Step 5: Generalize steps into algorithm – you’ll need to see underlying pattern to solve problem Requires two activities: Replace particular values used in each step with mathematical expressions of parameters Find repetition in terms of parameters Algorithm for 𝑦 𝑥 (5/8) 9 Replace particular values used in each step with mathematical expressions of parameters Set 𝑥 = 𝑛 = 3, 𝑦 = 4 Multiply 𝑥 by 𝑛 = 3 You get 𝑛 = 9 Multiply You get 𝑛 = 27 Multiply You 𝑥 𝑦 𝑥 by 𝑛 = 9 𝑥 by 𝑛 = 27 get 𝑛 = 81 is 𝑛 Algorithm for 𝑦 𝑥 10 Find repetition Set 𝑛 = 𝑥 = 3, 𝑦 = 4 𝑛 = 𝑚𝑢𝑙𝑡𝑖𝑝𝑙𝑦 𝑥 𝑏𝑦 𝑛 𝑛 = 𝑚𝑢𝑙𝑡𝑖𝑝𝑙𝑦 𝑥 𝑏𝑦 𝑛 𝑛 = 𝑚𝑢𝑙𝑡𝑖𝑝𝑙𝑦 𝑥 𝑏𝑦 𝑛 𝑦 𝑛 = 𝑥 is 𝑟𝑒𝑠𝑢𝑙𝑡 (6/8) Algorithm for 𝑦 𝑥 (7/8) 11 Generalize steps into algorithm Set 𝑛 = 𝑥, 𝑖 = 1 while (𝑖 < 𝑦) 𝑛 = 𝑛 ∗ 𝑥 𝑖 = 𝑖 + 1 endwhile 𝑛 is answer Algorithm for 𝑦 𝑥 (8/8) 12 Test algorithm with broader variety of data Implementation Phase 13 Code the algorithm Test code Debug code in case testing step generates incorrect results Coding the Algorithm 14 #include <stdio.h> int main(void) { int x, y, i, n; printf("Enter two integers: "); scanf("%d %d", &x, &y); n = x; i = 1; while(i < y) { n = n * x; i += 1; } printf("%d raised to power of %d is: %d\n", x, y, n); return 0; } Coding the Algorithm – Even better 15 #include <stdio.h> int exponent(int x, int y); int main(void) { printf("Enter base and power: "); int base, power; scanf("%d %d", &base, &power); int result = exponent(base, power); printf("%d ^ %d is: %d\n", base, power, result); return 0; } int exponent(int x, int y) { int n = x, i = 1; while (i < y) { n = n * x; i = i + 1; } return n; } Testing the Code 16 Whitebox vs. Blackbox testing Unit test requires calling function or program and verifying that results are correct If testing step generates incorrect results, debug code Note: There are 2 major bugs in previous program!!!