Computer Graphics Ch3-4-6 October PDF
Document Details
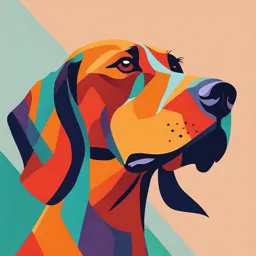
Uploaded by DependableGuitar7751
Cairo University Computer Science
F. S. Hill, S. M. Kelley
Tags
Summary
This document is a chapter on computer graphics, focusing on topics such as Windows, Viewports and Clippings, Creating Useful Drawing Tools, and Turtle Graphics. It seems to be a set of slides or notes, and not a formal exam paper.
Full Transcript
Computer Graphics Chapter Three Slides prepared by the author Computer Graphics using OpenGL (3rd Edition) 15/12/2024 By: F....
Computer Graphics Chapter Three Slides prepared by the author Computer Graphics using OpenGL (3rd Edition) 15/12/2024 By: F. S. Hill, S. M. Kelley 1 1 This Week Windows, Viewports and Clippings Creating Useful Drawing Tools Turtle Graphics 15/12/2024 Computer Graphics 2 2 1 Windows and Viewports Previously we looked at an OpenGL window where x and y were plotted as positive pixel values. However, we may not be interested in keeping track of pixels like this. We may prefer to plot points in the coordinates in which they are given. This means handling both positive and negative values. 15/12/2024 3 3 Windows and Viewports We need to distinguish between the world, world coordinates, world windows, the screen window and the viewport. It’s like this…. 15/12/2024 4 4 2 Windows and Viewports The World (what you can see, the real world) The World Window (the bit we want to capture) Screen Screen Window Viewport 15/12/2024 5 5 Windows and Viewports The world window is a rectangle. The viewport is a rectangle. Both are not necessarily the same size or have the same aspect ratio. Coordinates need to be stretched, shrunk and moved to make them fit. 15/12/2024 6 6 3 Windows and Viewports World Window Example Viewports 15/12/2024 7 7 Windows and Viewports World Window Viewport This is called Mapping (0,0) (100,0) (0,0) (100,0) 15/12/2024 8 8 4 Windows and Viewports Recall from the last lecture: ▪ x’ = Ax + B ▪ y’ = Cy + D This is exactly how mapping is achieved!! 15/12/2024 9 9 Windows and Viewports Mapping involves scaling and translation (moving). Both the world window and viewport can be any aligned rectangle. Usually the viewport is set to take up the entire screen window. 15/12/2024 10 10 5 12/15/2024 11 11 12/15/2024 12 12 6 Mapping from the Window to the Viewport The Figure shows a world window and a viewport in more detail. The world window is described by its left, top, right, and bottom borders as W.l, W.t, W.r, and W.b The viewport is described likewise in the coordinate system of the screen ' opened at some place on the screen) by V.l, V.t, V.r, and V.b 12/15/2024 Computer Graphics 13 CS510737 13 Windows and Viewports W.t = Window top V.t = Viewport top W.l = Window left V.l = Viewport left W.b = Window bottom V.b = Viewport bottom W.r = Window right V.r = Viewport right 15/12/2024 14 14 7 Windows and Viewports xmin, ymin, xmax, ymax = W.l, W.b, W.r, W.t SCREENWIDTH = V.r – V.l SCREENHEIGHT = V.t – V.b 15/12/2024 15 15 We derive a mapping or transformation, called the window-to-viewport mapping. This mapping is based on a formula that produces a point (sx, sy) in the screen window coordinates for any given point (x, y) in the world. sx = Ax + C, sy = By + D C A 12/15/2024 16 16 8 Windows and Viewports The same holds for y: sy − V.b y − W.b = V.t − V.b W.t − W.b or by rearranging D B V.t − V.b V.t − W.b sy = y + V.b − W.b W.t − W.b W.t − W.b 15/12/2024 17 17 Using the formulas in Equation 3.3, we obtain A = 180, C = 40, B = 240, and D = 60. Thus, for this example, the window-to-viewport mapping is sx = 180x + 40; sy = 240y + 60. 12/15/2024 18 18 9 PRACTICE EXERCISE Find values of A,B, C, and D for the case of a world window of (-10.0,10.0, -6.0,6.0) and a viewport of (0,600,0,400). What about the -ve values ?? 12/15/2024 19 19 Windows and Viewports Example World Window 400 Viewport (10,6) (-10,-6) 0 0 600 What are A, B, C & D ?? 15/12/2024 20 20 10 Windows and Viewports Do you need to perform these calculations each time you draw something with OpenGL?? 15/12/2024 21 21 Windows and Viewports No OpenGL does all the hard work for you. But it is important that you understand what is going on….. 15/12/2024 22 22 11 Windows and Viewports Each time you call for a vertex to be drawn (e.g. glVertex2f() etc..) the coordinates of the point are passed through a set of transformations that map world coordinates into viewport coordinates. 15/12/2024 23 23 Windows and Viewports First set the world window coordinates with: void glOrtho2D(GLDouble left, GLDouble right, GLDouble bottom, GLDouble top); Then set the viewport with: void glViewport(GLint xmin, GLint ymin, GLint width, GLint height); 15/12/2024 24 24 12 Doing It in OpenGL The world window is set by the function gluOrtho2D(),andthe viewport is set by the function gIViewport ().These functions have the prototypes void gluOrtho2D(GLdouble left, GLdouble right, GLdouble bottom, GLdouble top); which sets the window to have a lower left corner of (left, bottom) and an upper right corner of (right, top), and void gIViewport(GLint x, GLint y, GLint width, GLint height); which sets the viewport to have a lower left corner of (x, y) and an upper right corner of (x + width, y + height). By default, the viewport is the entire screen window: If W and H are the width and height of the screen window, respectively, the default viewport has lower left corner at (0, 0) and upper right corner at (W, H). 12/15/2024 25 25 Because OpenGL uses matrices to set up all its transformations, gluOrtho2D() must be preceded by two "setup" functions: gIMatrixMode (GL_PROJECTION) gILoadIdentity() // sets the window gIMatrixMode(GL_PROJECTION); glLoadIdentity(); gluOrtho2D(0.0, 2.0, 0.0, 1.0); // sets the viewport gIViewport(40, 60, 360, 240); 12/15/2024 26 26 13 In Figures 2.10 and 2.17, the programs used the following instructions: 1. in main(): glutInitWindowSize(640,480); // set screen window size This instruction sets the size of the screen window to 640 by 480. The default viewport was used, since no gIViewport () command was issued; the default viewport is the entire screen window. 2. in mylnit () : glMatrixMode(GL_PROJECTION); gILoadIdentity(); gluOrtho2D(0.0, 640.0, 0.0, 480.0); 12/15/2024 27 27 Windows and Viewports Example World Window 400 Viewport (10,6) (-10,-6) 0 0 600 // sets the window gIMatrixMode(GL_PROJECTION); glLoadIdentity(); gluOrtho2D(?, ?, ?, ?); gluOrtho2D(-10, 10, -6, 6); // sets the viewport gIViewport(?, ?, ?, ?); gIViewport(0, 0, 600, 400); 15/12/2024 28 28 14 Windows and Viewports void myInit(void) { glClearColor(1.0,1.0,1.0,0.0); glColor3f(0,0,0); glClear(GL_COLOR_BUFFER_BIT); glMatrixMode(GL_PROJECTION); glLoadIdentity(); //set the viewing coordinates gluOrtho2D(-10.0, 10.0, -6.0, 6.0); glViewport(0,0,600,400); } 15/12/2024 29 29 Handy Functions //--------------- setWindow --------------------- void setWindow(GLdouble left, Gldouble right, GLdouble bottom, GLdouble top) { glMatrixMode(GL_PROJECTION); glLoadIdentity(); gluOrtho2D(left, right, bottom, top); } //---------------- setViewport ------------------ void setViewport(GLdouble left, Gldouble right, GLdouble bottom, GLdouble top) { glViewport(left, bottom, right – left, top - bottom); } 15/12/2024 30 30 15 How OpenGl draws a point glPointSize(10.0); glBegin(GL_POINTS); glVertex2i(-10,-6); glVertex2i(0,0); glVertex2i(10,6); glEnd(); *NOTE: Vertex are given in World Coordinates and OpenGL maps them to the Viewport Coordinates. 15/12/2024 31 31 Windows and Viewports Plotting a function revisted.. //set the viewing coordinates setWindow(xmin, xmax, ymin, ymax); setViewport(0,640,0,480); glBegin(GL_POINTS); for(GLdouble x = xmin; x < xmax; x+=0.005 ) { glVertex2d(x, pow(2.7183, -x)*cos(2*3.14*x)); } glEnd(); -example322.cpp 15/12/2024 32 32 16 sinc(x) = [sin(PI *x)] / PI * x void myDisplay(void) // plot the sine function, using world coordinates { setWindow(-5.0, 5.0, -0.3, 1.0); // set the window setViewport(0, 640, 0, 480); // set the viewport glBegin(GL_LINE_STRIP); for(GLfloat x = -4.0; x < 4.0; x += 0.1) // draw the plot glVertex2f(x, sin(3.14159 * x) / (3.14159 * x)); glEnd(); glFlush(); } 12/15/2024 33 33 Setting the Window and Viewport Automatically Setting of the Window Often, the programmer does not know where the object of interest lies, or how big it is, in world coordinates. Like the dinosaur earlier, the object might be stored in a file, or it might be generated procedurally by some algorithm whose details are not known. In either case, it is convenient to let the application determine a good window to use. 12/15/2024 34 34 17 [A] Setting the Window To set the window to an appropriate size, you need to predetermine the size of the coordinates to be captured. Then set the window to the minimum and maximum extent of these coordinates. 12/15/2024 35 35 [A] Setting the Window The program needs to pass over the data twice: 1. Execute the drawing routine, but don’t do any drawing. 2. Execute the drawing routine again, but this time draw! 12/15/2024 36 36 18 [A] Setting the Window GLdouble ytemp; for(GLdouble x = xmin+0.005; x < xmax; x+=0.005 ) { ytemp = pow(2.7183, -x)*cos(2*3.14*x); if (ytemp > ymax) ymax = ytemp; if (ytemp < ymin) Pass 1 to calculate ymin = ytemp; suitable ymin and } ymax setWindow(xmin, xmax, ymin, ymax); Set the window boundaries glBegin(GL_POINTS); for(GLdouble x = xmin; x < xmax; x+=0.005 ) { Pass 2 glVertex2d(x, pow(2.7183, -x)*cos(2*3.14*x)); } glEnd(); 12/15/2024 37 37 [B] Setting the Viewport To draw an undistorted version of the data in a viewport, you need to ensure the viewport and the window have the same aspect ratio. i.e. Wwindow Wviewport = H window H viewport 12/15/2024 38 38 19 [B] Setting the Viewport Recall, if the aspect ratio of a rectangle is less than 1, the rectangle is taller than wide. E.g. W/H = 3/5 If the aspect ratio is greater than 1, the rectangle is wider than tall. E.g. W/H = 5/3 12/15/2024 39 39 Automatic Setting of the Viewport to Preserve the Aspect Ratio You need to specify a viewport that has the same aspect ratio as the world window to avoid distortion version of a figure and will fit in the screen window. Suppose the aspect ratio of the world window is known to be R and the screen window has width W and height H. There are two distinct situations: The world window may have a larger aspect ratio than the screen window (R > W/H), or it may have a smaller aspect ratio (R < W/H). 12/15/2024 40 40 20 The two situations are shown in Figure 3.16. We consider each in turn. Case (a): R > W/H. the viewport to match aspect ratio R will extend fully across the screen window, therefore, the viewport will have width W and height W/R, so it is set with the following command setViewport(0, W, 0, W/R); Case (b): R < W/H. the viewport to match aspect ratio R will reach from the top to the bottom of the screen window. At its largest, the viewport will have height H, but width HR, so it is set with the command setViewport(0, H * R, 0, H); 12/15/2024 41 41 Set the Viewport a) R > W/H (where R is the aspect ratio of the world window) If the world window is ‘flatter’ than the screen window, there will be unused space above and/or below. The width of the world window will be mapped to the entire width of the screen window. setViewport(0,W,0,W/R); 12/15/2024 42 42 21 Set the Viewport b) R < W/H (where R is the aspect ratio of the world window) If the world window is ‘taller’ than the screen window, there will be unused space on the sides. The height of the world window will be mapped to the entire height of the screen window. setViewport(0,H*R,0,H); 12/15/2024 43 43 EXAMPLE 3.2.7 A tall window Suppose the window has aspect ratio R = 1.6 and the screen window has H = 200 and W = 360, and hence, W/H = 1.8. Therefore, Case (b) applies, setViewport(0, H * R, 0, H); and the viewport is set to have a height of H = 200 pixels and a width of = H * R = 320 pixels. EXAMPLE 3.2.8 A short window Suppose R = 2 and the screen window is the same as in the previous example. Then Case (a) applies, setViewport(0, W, 0, W/R); and the viewport is set to have a height of W/R = 180 pixels and a width of W = 360 pixels. 12/15/2024 44 44 22 Resizing the Screen Window In a windows-based system the user can resize the screen window at run time. This action generates a resize event that the system can respond to. // specifies the function myReshape called on an resize event glutReshapeFunc(myReshape); void myReshape(GLsizei W, GLsizei H); When this function is executed, the system automatically passes it the new width and height of the screen window, which the function can then use in its calculations. (GLsizei is a 32-bit integer; see Figure 2.7.) This works the same as for the glutMouseFunc and glutKeyboardFunc … If you don’t run a glViewport after a window resize, the glViewport defaults to the window coordinates. 12/15/2024 45 45 Making a Matched Viewport One common approach is to find a new viewport that both fits into the new screen window and has the same aspect ratio as the world window. Matching the aspect ratios of the viewport and world window in this way will prevent distortion in the new picture. Figure 3.17 shows a version of myReshape () that does the desired matching: 12/15/2024 46 46 23 void myReshape(GLsizei W, GLsizei H) { if(R > W/H) // use (global) window aspect ratio setViewport(0, W, 0, W/R); else setViewport(0, H * R, 0, H); } It finds the largest matching viewport (i.e., one that matches the aspect ratio R of the window) that will fit into the new screen window. The routine obtains the width and height of the new screen window through its arguments. The code is a simple embodiment of the result in Figure 3.16. 12/15/2024 47 47 Clipping Lines In this section, we describe a classic line-clipping algorithm, the Cohen-Sutherlan clipper, that computes which part (if any) of a line segment with endpoints p 1 and p2 lies inside the world window and then reports back the endpoints of that part. 12/15/2024 48 48 24 Figure 3.18 shows a typical situation covering some of the many possible actions of a clipper. The function clipSegment ( ) does one of four things to each line segment 1. If the entire line lies within the window (e.g., like segment CD), the function returns a value of 1. 2. If the entire line lies outside the window (e.g., like segment AB), the function it returns a value of 0. 3. If one endpoint is inside the window and one is outside (e.g., like segment ED),| the function clips the portion of the segment that lies outside the window and returns a value of 1. 4. If both endpoints are outside the window, but a portion of the segment passe( through it (e.g., like segment AE), the function clips both ends and returns value of 1. 12/15/2024 49 49 Left -- Above ---- Right --- Below 12/15/2024 50 50 25 12/15/2024 51 51 Testing for a Trivial Accept or Trivial Reject Trivial accept: Both code words are FFFF; Trivial reject: The code words have a T in the same position; both points are to the left of the window, or both are above, etc. How? Answer: Code1 AND Code2 != 0. First, endpoint pairs are checked for trivial acceptance. If the line cannot be trivially accepted, region checks are done. Now logically AND together the two region codes. A non-zero result means the line must be totally outside the window. Why? 12/15/2024 52 52 26 Chopping When There Is Neither Trivial Accept nor Trivial Reject The Cohen-Sutherland algorithm uses a divide-and-conquer strategy. If the segment can be neither trivially accepted nor rejected, it is broken into two parts at one of the window boundaries. One part lies outside the window and is discarded. The other part is potentially visible, so the entire process is repeated for this segment against another of the four window boundaries. 12/15/2024 53 53 do{ form the code words for p1 and p2 1- if (trivial accept) return 1; //Trivial accept: Both code words are FFFF; 2- if (trivial reject) return 0; // Code1 AND Code2 != 0 / line is partially inside 3- Choose an endpoint of the line that is outside the given rectangle; 4- Find the intersection point of the rectangular boundary (based on region code); 5- Replace endpoint with the intersection point and update the region code; }while(1); Thus, after at most four iterations, trivial acceptance or rejection is assured. 12/15/2024 54 54 27 A situation that requires all four clips is shown in Figure 3.24. The 1st clip changes p1 to A 2nd finds A still outside and bellow and so changes A to C 3rd alters p2 to B Last changes p2 to D 12/15/2024 55 55 12/15/2024 56 56 28 PRACTICE EXERCISE 3.3.1 Hand simulation of clipSegment () Go through the clipping routine by hand for the case of a window given by {left, right, bottom, top} = (30, 220, 50, 240) and the following line segments: 1. p1 = (40,140),p2 = (100,200); 2. p2 = (10, 270), p1 = (300, 0); 3. p1 = (20,10),p2 = (20,200); 4. p1 = (0, 0), p2 = (250,250); In each case, determine the endpoints of the clipped segment, and for a visual check, sketch the situation on graph paper. 12/15/2024 57 57 29