C Programming Notes PDF
Document Details
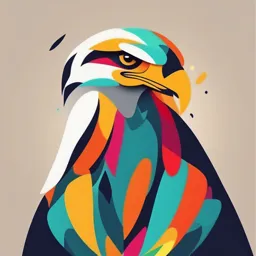
Uploaded by ReformedConcreteArt2722
MIT Junior College
Tags
Summary
These notes provide a summary of C programming concepts. The document outlines various programming structures, comparisons between interpreters and compilers, and examples of algorithms in C, focusing on topics such as data types, operators, and control structures.
Full Transcript
# C Programming - C programming language was developed at AT&T's Bell Laboratories of USA in 1972 by Dennis Ritchie. - Initially, C language was developed to be used in UNIX operating systems. - C programming language is a middle-level because it has both a relatively faster programming efficiency a...
# C Programming - C programming language was developed at AT&T's Bell Laboratories of USA in 1972 by Dennis Ritchie. - Initially, C language was developed to be used in UNIX operating systems. - C programming language is a middle-level because it has both a relatively faster programming efficiency and relatively good machine efficiency i.e., faster execution. ## Structures of C - Structure means to break a program into parts or blocks so that it may be easy to understand. We break the program into parts using functions, data-types, syntax, etc. ## Interpreter VS Compiler - A high language is one that is understandable by humans and is called as Source Code. - However, a computer does not understand high-level language, It only knows binary or machine code. - Both compilers and interpreters use to convert high-level language into machine code or binary code. | Interpreter | Compiler | | ------------------------------------------- | ----------------------------------------------------------------------------- | | Translates program one statement at a time. | Scans the entire program and translates it as 'a whole' into machine code. | | Takes less amount of time analyze the source code. The overall execution time is slower than compiler. | Takes a large amount of time to analyze the source code. Overall execution time is faster than interpreters. | | No object code is generated, hence are memory efficient. | Generates object code, which further requires linking, hence requires more memory. | | Programming languages like JavaScript, Python, Ruby use interpreters. | Programming languages like C, C++, Java use compilers. | ## The form of C programming - A C program is divided into six sections. - Documentation, Link, Definition, Global declaration, Main() function, Sub programs. - While the main function section is compulsory, the rest are optional in the structure of C program. - Every C program will have one or more functions and there is one mandatory function which is called main(). - This function is prefixed with keyword int. ## Library and Linking - A library is a collection of pre-compiled object files that can be linked to programs. - Library are typically stored as special archive with extension 'a'. - Linking refers to the creation of a single executable file from multiple object files. It is common that the linker will complain about undefined functions. ## To write the first C program ``` #include <stdio.h> int main() { printf("Hello World"); return 0; } ``` - `#include <stdio.h>` includes the standard input output library functions. The `printf()` function is defined in `stdio.h`. - The `main` function is the entry point of every program in C language. Here it is `int main()` - `printf()` function is used to print data on the console. It just displays the message. - **To compile C program- Ctrl + F9** - **To run C program- Alt + F9** # Algorithm - Algorithm is a simple English statement which provide step by step process for the given problem /program/question. - For example, an algorithm to find if given number is even or odd. 1. Start. 2. Accept a number. 3. Divide number by 2 and find remainder. 4. Check if remainder is zero or not. 5. If remainder is zero (i.e. n % 2 == 0) print given number is even. 6. Else print given number is odd. 7. Stop. ## Q.i. Write an algorithm to print addition of two numbers. 1. Start. 2. Accept two numbers. 3. Perform operation nl + n2. 4. Print result. 5. Stop. ## π. Write an algorithm to find area of circle. 1. Start. 2. Accept radius value. 3. Find the area using formula `3.14 x radius^2`. 4. Print result. 5. Stop. ## 1. Write an algorithm to find net salary (Net salary = Basic + ta + da) (ta = 20 % of basic, da = 40 % of basic) 1. Start. 2. Accept value of basic salary. 3. Calculate ta using formula, `ta = 20 x basic / 100`. 4. Calculate da using formula, `da = 40 x basic / 100`. 5. Add values of ta and da into basic. 6. Print result. 7. Stop. ## iv. Write an algorithm to find area of a rectangle. 1. Start. 2. Accept values of sides of a rectangle. 3. Calculate area using formula `length x width`. 4. Print result. 5. Stop. ## v. Write an algorithm to find area of a triangle. 1. Start. 2. Accept values of base and height of triangle. 3. Calculate area using formula, `area = 1/2 x b x h`. 4. Print result. 5. Stop. ## vi. Write an algorithm to find the area of square. 1. Start. 2. Accept value of side of a square. 3. Multiply the value by itself to get the area of square. 4. Print result. 5. Stop. ## vii. Write an algorithm to find square of a number. 1. Start. 2. Accept a number. 3. Multiply the number by itself. 4. Print result. 5. Stop. # Flow-Chart - It is a pictorial or diagrammatic representation of an algorithm. - Symbols used in Flow chart. 1. **start/stop symbol** 2. **input/output symbol** 3. **processing symbol** 4. **decision symbol** 5. **flowline symbol** 6. **connector symbol** ## Q.i. Draw a flowchart to print addition of two integer numbers. - **Algorithm:** 1. Accept two numbers nl, n2. 2. Perform operation `nl + n2`. 3. Print result. - **Flowchart:** ``` start ↓ Read "num1","num2" ↓ sum = num1 + num2 ↓ Print sum ↓ end ``` ## (ii) Draw a flowchart to print given number is odd or even. - **Flowchart:** ``` start ↓ Accept "nl" ↓ if n%2==0 ↓ Yes ↓ Print Even ↓ Stop No ↓ Print Odd ↓ Stop ``` ## (iii) Draw a flowchart to print if given number is prime or not. - **Algorithm:** 1. Start. 2. Read number n 3. Check the number is not divisible by 2 to n/2 - 1 4. If (iii) is true print given number is prime. else print given number is not prime. 5. Stop. - **Flowchart:** ``` Start ↓ Read "n", i = 2 ↓ if i == n ↓ Yes ↓ Print prime No ↓ if n / i == 0 ↓ Yes ↓ Print not a prime No ↓ i = i + 1 ↓ Stop ``` ## (iv) Draw a flowchart to print print largest number among given two number. - **Algorithm:** 1. Start. 2. Accept two number `nl, n2`. 3. Check if `nl > n2`, print `nl` is largest. else, check `n1 == n2`, print `n1` and `n2` are equal. else, print `n2` is largest. 4. Stop. - **Flowchart:** ``` Start ↓ Accept two numbers "nl", "n2" ↓ if nl > n2 ↓ Yes ↓ Print "nl is largest" ↓ Stop No ↓ if "n1 == n2 ↓ Yes ↓ Print "n1 and n2 equal ↓ No ↓ Print "n2 is largest ↓ Stop ``` ## v. Draw a flowchart to find largest number among three numbers. - **Flowchart:** ``` Start ↓ Input A, B, C ↓ If A > B ↓ Yes ↓ If A > C ↓ Yes ↓ Print "A is greater" ↓ Stop No ↓ If B > C ↓ Yes ↓ Print "B is greater" ↓ Stop No ↓ Print "C is greater" ↓ Stop ``` # Variable - Variable is a name of memory location. Its value can be changed. It is used to store data/information. - It is non-static. - A variable is character, i.e., nothing but information (alphabet, digit, symbol). ## Syntax: `<datatype> <variable1>, <variable2>,…;` ## Key Word: - A reserved word we cannot use for our purpose, i.e., cannot use as a variable. - Those keywords are: - break - case - char - const - continue - default - unsigned - do - double - else - enum - float - for - void - goto - if - int - long - return - short - while - signed - sizeof - static - struct - switch - typedef - …etc. - For example, while declaring variable, we can’t use `int char;` because `char` is datatype keyword. ## Constants - A constant is a value that cannot be changed in a program. - **Primary Constants:** - Integer, float, character are called as primary constants. - **Secondary Constants:** - array, structure, pointers, enum, etc. are called as secondary constants. ## Character Set - A character denotes any alphabet, digit or special symbol used to represent information. - Valid character set in C: - **Alphabets:** - A, B, C, …, Y, Z, a, b, c, …, y, z - **Digits:** - 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 - **Special Symbols:** - `* ! @ # $ % ^ & * ( ) - = + < > { } [ ] ; : " ? / ` ## Datatypes - **Basic datatypes:** Primary / Primitive datatypes - **Derived datatypes:** Secondary (union, structure, array, etc.) - **Enumerative datatypes:** Enums - **Void datatypes:** empty value / Nothing ## Integer Datatype - Integers are whole numbers, excluding decimal values. - Integer datatype occupies 2 bytes (32 bit) and 4 byte (64 bit size) of memory to store an integer value. - The range of 2 byte memory can hold any value between -32768 and 32767. - The range of 4 byte memory can hold any value between -2147483648 to 2147483647. - **Example:** ``` int age = 10; ``` - In this example, the variable named `age` would be defined as an integer and assigned the value of 10. ## Character Datatype - Character datatype stores character in fixed length field. Character can be a number, letter, symbol. - The size of both signed and unsigned character is `1 byte`. - **Example:** ``` char ch = 'a'; ``` - Here `ch` is variable and value assigned to it is 'a'. ## Floating Datatype - Float is a floating point number which has a decimal number place. It is used when more precision is needed. - **For example:** ``` float pi = 3.14; ``` - Here `pi` is variable of type `float` and assigned value is `3.14`. ## Operators and Expressions - Operators are one of the features in C which has symbols that can be used to perform mathematical, relational, bitwise, conditional, or logical manipulations. - The C programming language has a lot of built-in operators to perform various tasks as per need of the program. ## (1) Arithmetic Operators: - Arithmetic operators are used to perform common mathematical operations. | Operator | Name | Description | | -------- | -------------- | ---------------------------------------------------------------------------------------------------------- | | + | Addition | Adds together two values | | - | Subtraction | Subtract one value from another | | * | Multiplication | Multiplies two values. | | / | Division | Divides one value by another | | % | Modulus | Returns the division remainder. | | ++ | Increment | Increases the value of variable by 1 | | -- | Decrement | Decreases the value of variable by 1 | ## (2) Relational Operators (Comparison Operators) - It checks the relationship between two operands. - If the given relation is true, it will return 1 and if the relation is false, then it will return 0. | Operator | Name | Description | | -------- | ------------------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------ | | == | Equal to | Check if the values are equal. | | != | Not equal | Check if the values are not equal. | | > | Greater than | Check if the first value is greater than second. | | < | Less than | Check if the first value is less than second. | | >= | Greater than or equal to | Check if the first value is greater than or equals to second value. | | <= | less than or equal to | Check if the first value is less than or equals to second value. | - The return value of comparison is either one `1` or `0`, which means true (1) or false (0) These values are known as Boolean values. ## (3) Logical Operators - Logical operators are used to test more than one condition in a program. - These logical operators are: | Operator | Name | Description | | -------- | -------- | ------------------------------------------------------------------------------------------- | | && | AND | Check if all conditions are true. | | || | OR | Check if one of the conditions is true. | | ! | NOT | Reverse the result. (return 0 if result is 1) | ## (4) Increment / Decrement Operator ### (i) Increment: - Increment operators are the unary operators used to increment or add 1 to the operand value. - The increment operand is denoted by the double plus symbol `++`. - If we use the increment operator as a prefix, it is called pre-increment. - The pre-increment operator is used to increase the original value of operand by 1 before assigning it to the expression. - **Syntax:** ``` x = ++A; ``` - The value of operand `A` is increased by `1` and then new value is assigned to variable. - If we use the increment operator as postfix, it is called as post-increment. - The post-increment operator is used to increase the original value by `1` after assigning it to the expression. - **Syntax:** ``` x = A++; ``` - The value of operand `A` is assigned to the variable. After that, the value `A` is incremented by `1`. ### (ii) Decrement - Decrement operators are unary operator used to decrease the original value of operand by `1`. - The decrement operator is denoted by double minus symbol `--`. - If we use decrement operator as a prefix, it is called pre-decrement. - The pre-decrement operator is used to assign decrease the operand value by `1` before assigning it to the mathematical expression. - (Simply, new value is assigned to variable). - **Syntax:** ``` B = --A; ``` - The value of operand `A` is decreased by `1`, and then the new value is assigned to variable `B`. - If we use decrement operator as a postfix, it is called post-decrement. - The post-decrement operator is used to decrease the value of operand by `1` after assigning it to the expression. - (Simply, it assign the value, then decrease the operand.) - **Syntax:** ``` B = A--; ``` - In the above example, the value of operand `A` is assigned to the variable `B`, and then the value of `A` is decreased by `1`. ## (5) Bitwise Operator - Bitwise operators are the operators which work on bits and perform the bit by bit operation. - Mathematical operators like addition, subtraction, multiplication, division, etc. are converted to bit level which makes processing faster and easier to implement and compilation of a program.