Introduction To Hardware PDF
Document Details
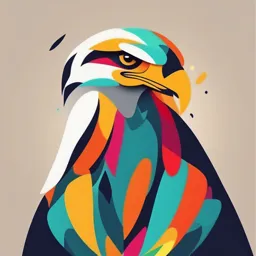
Uploaded by AchievableSugilite151
Tags
Related
- Computer Organization and Design RISC-V Edition PDF
- Computer Architecture Lecture Notes PDF
- Computer Organization and Architecture Lecture Notes PDF
- Chapter 1: Introduction to Computers and Programming PDF
- Computer Programming (01CE1101) Unit 1 Introduction PDF
- Computer and Programming Principles Chapter 2 PDF (2020/2021)
Summary
This document provides an introduction to computer hardware and its working principles. It also includes information on programming logic, algorithms, and flowcharts, as well as an overview of programming languages like C and C++.
Full Transcript
1 ME 171 : Computer Programming Language 3.00 Credit Hours Course Teacher: Dr. Md. Mamun, Dr. S. M. Shavik ❑ Introduction to computer hardware and its working principle; ❑ Programming logic, algorithms, and flowcharts. ❑ Introduction to structured programming; ❑ Overview of C and C++ programming l...
1 ME 171 : Computer Programming Language 3.00 Credit Hours Course Teacher: Dr. Md. Mamun, Dr. S. M. Shavik ❑ Introduction to computer hardware and its working principle; ❑ Programming logic, algorithms, and flowcharts. ❑ Introduction to structured programming; ❑ Overview of C and C++ programming languages: C and C++ fundamentals – data types and expressions; Operators; Libraries and keywords; ❑ Statements; Control statements; Input and output systems. ❑ Pointers; ❑ Arrays; ❑ Functions; ❑ Strings; ❑ File Input/Output ❑ Structure and Union ❑ Object Oriented programming; ❑ Introduction to advanced programming (Python) 2 Textbooks: 1. Teach Yourself C – Herbert Schildt, Osborne 2. Computer Fundamentals – Pradeep K. Sinha, BPB Publications [ Any suitable book on C programming ] ❖ সবার জন্য C কম্পিউটার প্রাগ্রাম্প িং লযািংগুয়েজ – প্ াোঃ কা রুজ্জা ান্ ম্পন্টন্, জ্ঞান্য়কাষ রকাশন্ী ❖ The C Programming Language – Brian. W. Kernighan and Dennis M. Ritchie, Pearson Education Softwares: ✓Codeblocks (used in the sessional) [ Any suitable compiler for C programming such as DevCPP, Visual C++, Turbo C++, Borland C++, Geany, GNU GCC, Cygwin, Xcode, etc.] 3 What is a Computer ? A computer is an electronic device, which can input, process (according to instructions provided), and output data. Programs (Instructions) Data Data input Processing output A computer is a machine that stores data, interact with devices, and execute programs (provides computing capabilities to its users). A computer is an electronic device that stores, retrieves, and processes data, and can be programmed with instructions. A computer is composed of hardware and software, and can exist in a variety of sizes and configuration, e.g., Mainframe computer as large as an entire building, Supercomputer, Single Board Computer (SBC) such as Raspberry Pi, Smartphone, Tablets, PC on a Stick, etc. Computers 4 Supercomputer (Speed) Mainframe Computer (Concurrency) Workstation (Specialized) [Purpose-built, Desktop Computer (General-purpose) i.e., gaming workstation, simulation workstation, animation workstation] 5 Computers Mac Mini All-in-one Computer Intel NUC Raspberry PI Raspberry PI zero Laptop Computer Stick Computer 6 Computers Surface Pro iPad Pro Galaxy S9 iPhone XS Max Mainframe vs Supercomputers 7 Mainframe Computers are the professional level commercial computers that work as a server and are used by the large organizations where data is processed in large quantity and is (concurrently) accessed by hundreds and thousands of users at a time through consoles or terminals (displays and keyboards/keypads). Supercomputers are the fastest computer of all that possess the ability to do billions of calculations per second and can process data 1000 times faster than the ordinary computers such as common personal computers. Mainframe computers are designed to deal with thousands of users accessing the system at a time. These are used in large business organizations such as banks, government database stations, military organizations, health organizations, e-commerce, and e-business, etc. Supercomputers are designed to be more quick and efficient in data processing. These are used in space centers such as NASA, weather forecasting stations, scientific laboratories, nuclear power stations, etc. For example, a modern high-end desktop computer can perform on average100 Gigaflops whereas a modern supercomputer can perform on average 100 Petaflops. Micro-controllers and System-on-Chip 8 A microcontroller (or MCU for Micro-Controller Unit) is a ‘small computer on a single chip (integrated circuit)’. A microcontroller contains one or more CPUs (processor cores) along with memory and programmable input/output (I/O) peripherals. Program memory flash or OTP ROM is also often included on chip, as well as a small amount of RAM. Microcontrollers are designed for embedded applications, in contrast to the microprocessors used in personal computers for general-purpose applications. They are used in automations of devices, such as automobile engine control systems, implantable medical devices, office machines, appliances, power tools, toys and other embedded systems. By reducing the size and cost, MCUs make it economical to digitally control devices and processes. A System on a Chip (SoC) contains most of the main components of a general- purpose computer on its die, so it matches the compact size of an MCU. But unlike an MCU, the technical capacities and capabilities of different components of an SoC are almost comparable to a general-purpose CPU-based computer. They are used in smartphones, tablets, mini-computers, laptops and even desktop computers. 9 CPU Architecture (Neumann Architecture – Computer) An instruction "Read a data byte from memory and store it in the accumulator" is executed as follows: - Cycle 1 - Read Instruction Cycle 2 - Read Data out of RAM and put into Accumulator 10 CPU Architecture (Harvard Architecture – MCU and SoC) The same instruction (as shown under Neumann Architecture) would be executed as follows: Cycle 1 / - Complete previous instruction & - Read the "Move Data to Accumulator" instruction Cycle 2 / - Execute "Move Data to Accumulator" instruction & - Read next instruction Hence each instruction is effectively executed in one instruction cycle. Thus, due to parallelism, Harvard architecture executes more instructions in a given time compared to Neumann Architecture. 11 Why is a Computer? Note that, a computer is a synergistic system: CPU – Central Processing Unit, the brain/heart of a computer system. CPU Human Synergy Computer Speed Intelligence System AI – Artificial Intelligence, ML – Machine Learning/Deep Learning Through Artificial Neural Network (ANN); – These are in essence extremely ‘Sophisticated’ Programming by Human for Machines. AI systems may precisely be called ‘Expert systems’ such as IBM’s Deep Blue (Chess expert), Apple’s Siri, Microsoft’s Cortana, Google’s Google Assistant, Amazon’s Alexa, Samsung’s BixBy, etc. (Personal Digital Voice Assistants). NPU – Neural Processing Unit; APU – AI Processing Unit DPU – Data Processing Unit; TPU – Tensor Processing Unit 12 Computer Programming In traditional programming, every single smallest step has to be given to the CPU in the form of instructions to directly operate on the input data and output the processed data. In machine learning programming, the computer is given instructions to learn patterns/rules from a bunch of input dataset (instead of direct operation on the dataset) to output the inference or make a prediction over new data based on the already learned pattern or rules. A programming language is a formal constructed language designed to communicate these instructions to the computer. Some popular computer programming languages are Fortran, C, C++, Python, Java, HTML, PHP, Javascript, Perl, Ruby, Matlab, etc. 13 Computer Programming Like human languages, all computer programming languages dictates their own set of alphabets (characters), words (keywords), syntax (grammar), semantics (meaning). However, the most important component of programming, i.e. , logical step by step ‘instructions’ (simply “LOGIC” or more prominent term “ALGORITHM”) to get something done by the CPU remains the same for a particular problem irrespective of the language of instruction. The last component of programming is “Data Structure”, which dictates the method of manipulating the program ‘data’, i.e., storage/retrieval/modification of data (types) in the memory. 14 Computer Programming Therefore, learning computer programming for the first time using a particular language means learning its alphabets, keywords, syntax, semantics plus “algorithm” (the single most significant aspect) and “data structure”. Once a person develops skills / expertise in any particular computer language, (s)he can easily switch to another language (only by learning its alphabets, keywords, syntax, semantics). For a particular problem, the same “algorithm” can be used in all the languages. 15 Major Components of a Computer System A computer system consists of two sub-systems: hardware and software. Hardware is the electronic and mechanical parts of a computer system. Software is the data and the computer programs of a computer system. 16 Hardware vs. Software Hardware – Physical Components (CPU, Mainboard, RAM, HDD, Keyboard, Monitor, etc.) Software – Logical Instructions (OS, User programs, User Data, etc.) Firmware – Logical Instructions imprinted on physical Components (BIOS, UEFI, etc. installed in Mainboard) Basic Input Output System (BIOS) or Universal Extensible Firmware Interface (UEFI) provides the start-up (‘booting’) instructions which the CPU executes immediately after the computer is powered on. Without instructions, the CPU cannot even start the computer!!! 17 Computer Hardware Main Hardware Components of a Processing Unit: o CPU – Central Processing Unit (Processor) o Main Memory (RAM – Random Access Memory ) o MainBoard (Motherboard) o Power Supply Unit (PSU) o Secondary Memory /Auxiliary Storage [Hard Disk (Mechanical/SSD), Flash Disk (eMMC, SD), Optical Disk (DVD) and/or other Storage Devices] 18 Modern CPUs RAM Modules 19 Mainboard / Motherboard Switching Mode Power Supply (SMPS) 20 Processing Unit of a Modern Desktop Computer System 21 Central Processing Unit (CPU) CPU is the ‘brain’ plus ‘heart’ of the computer system. It renders the whole computing system live and useful. It does the fundamental computing within the system. It directly or indirectly controls all the other components. A single-core of a CPU consists of 3 units: CPU 1. The Arithmetic and Logic Unit (ALU). 2. The Control Unit (CU). 3. Registers (Memory). When a computer is switched on, the CPU continuously goes through a process called fetch-decode-execute cycle: The Control Unit fetches the current instruction from memory, decodes it and instructs the ALU (Arithmetic Logic Unit) to execute the instruction. The execution of an instruction may generate further data fetches from memory The result of executing an instruction is stored in either a register (CPU’s own memory) or RAM 22 Multi-Core CPU A multi-core CPU consists of a multiple of these 3-unit-core in a single chip so that multiple fetch-decode-execute (multi-threading) can take place making computation faster and efficient. 23 CPU Thus CPU continuously transfers data to and from the RAM. Instructions/Data transfer to and from the CPU are done in units called word (16 bit). If a CPU can handle a maximum word-size of 32 bits (Dword-double word), the CPU is called 32-bit CPU. If a CPU can handle a maximum word-size of 64 bits (Qword-quad word), the CPU is called 64-bit CPU. In addition to the registers, a CPU also has cache memories which are used to store the instructions that are used by the CPU repeatedly or the instructions in the pipeline (next executable instructions). Usually, the higher the amount of the cache memory, the better the CPU performs. But cache memories are very expensive. 24 CPU Instruction Set Architecture There are two types of CPU Instruction Set Architecture* (ISA) in wide use today: CISC (Complex Instruction Set Computer) – Intel x86, AMD64, x86_64, Intel Xeon, Atom, etc. are CISC CPUs. These CPUs support many instructions (sophisticated ones as well) but are power hungry. These are used in regular Desktop, Laptop, Netbook computers for their huge existing user-base, system frameworks and software libraries. RISC (Reduced Instruction Set Computer) – ARM (v5, v6, v7) CPUs are RISC ones. They support fewer instructions (often simpler ones) and are power saver. So they are used in smart phones, tablet computers, etc. [*‘CPU architecture’ means physical and logical design-base, i.e., number and layout of physical transistor circuits and logical instruction sets implemented in the CPU.] Motherboard 25 Mainboard is a sophisticated PCB (Printed Circuit Board). It mainly provides the required circuitry to connect different devices with the CPU, for example, RAM to the CPU. It contains electrical wires plus some special-purpose IC chips (chipsets) to facilitate the connectivity of different devices with the CPU. Mainboards also contain the startup instructions (BIOS ROM chip). The CPU is connected to RAM and I/O devices by the ‘bus’. When more than two devices can communicate (as a network) using the same set of connected wires (without the need for dedicated one-to-one connection between all devices) then this connectivity is termed as ‘bus topology’. Bus topology is implemented by a set of wires plus dedicated ICs which follow some communication protocols (a set of agreed-upon rules). For example, Universal Serial Bus (USB) is used to connect keyboard/mouse, pen drives, etc. to the CPU, SATA bus is used to connect hard disk drives, DVD drives to the CPU, etc. Other buses are SCSI, RS-232, PCI-E, Firewire, Thunderbolt, etc. Some buses used in MCUs are SPI, I2C, JTAG, etc. 26 Basic Computer System Main Hardware Components + Main Software Components: OS - Operating System [User Software Programs / Packages] + One Input device + One Output device [Keyboard/Mouse + Monitor/Printer] 27 Computing Platform (Environment) The computing platform or environment consists of any existing suitable combinations the main hardware component, i.e., CPU plus the main software component, i.e., OS (Operating System). Intel/AMD x86 CPU + Windows OS (32 bit) Intel x86_64/AMD64 CPU + Windows OS (64 bit) Intel CPU + Linux Intel CPU + Mac OS X ARM CPU + Linux ARM CPU + Android ARM CPU + iOS ARM CPU + iPadOS ARM CPU + Chrome OS Intel/AMD x86 CPU + Chrome OS ARM CPU + Windows on ARM ARM CPU + Mac OS X 28 Computing Platform (Environment) The basic knowledge of a suitable platform is necessary for a programmer. Because all that a CPU understands are patterns of 1’s and 0’s. i.e., Machine language (or Object Code) Any program written in any language (called “Source Code”) must be compiled into Machine language of a particular CPU type (CISC / RISC) for the CPU to understand and execute accordingly. Note that, Different CPU understand different machine languages (bit patterns). Different OSs provide different ready-to-be-used machine code (called “API routines/Library”) that are used by the programmer in their own program. API routines don’t match among OSs. [Emulator: QEMU / Simulator(Semi-Emulator): Hypervisor, UMT] 29 Primary Memory (RAM) Primary memory is divided into a number of memory cells (bits) or bytes. A bit (binary digit) is the smallest storage unit within a computer. It is a tiny electrical circuit that can be in one of two states: A voltage high represented by the symbol 1 A voltage low represented by the symbol 0 Any system of symbols can be represented by bit or byte patterns. A byte is a chunk of 8 bits. OSs keep track of the RAM on byte count. Each RAM byte must have a unique unsigned integer address, i.e., no two RAM bytes can have the same address. Byte addresses are automatically assigned by the OS. 30 16-byte RAM – Logical State byte-1 byte-2 byte-3 byte-4 Address 0000 0001 0010 0011 Value garbage garbage garbage garbage byte-5 byte-6 byte-7 byte-8 Address 0100 0101 0110 0111 Value garbage garbage garbage garbage byte-9 byte-10 byte-11 byte-12 Address 1000 1001 1010 1011 Value garbage garbage garbage garbage byte-13 byte-14 byte-15 byte-16 Address 1100 1101 1110 1111 Value garbage garbage garbage garbage 31 Primary Memory A 4-bit CPU/OS can only keep track of 24 =16 byte of RAM. A 16-bit CPU/OS can only keep track of 216 =65,536 byte (64 KB) of RAM. A 32-bit CPU/OS can only keep track of 232 =4,294,967,296 ≈ 4 billion (giga) addresses of RAM bytes, i.e., 4 GB of RAM Storages such as HDDs are divided into 512-byte sectors with unique addresses. Since only 4 billion addresses are available, A 32-bit CPU/OS can only address 4 𝐺𝑖𝑔𝑎 𝑠𝑒𝑐𝑡𝑜𝑟𝑠 × 512 𝑏𝑦𝑡𝑒𝑠 = 2 𝑇𝐵 partition of HDD. A 32-bit file system e.g., FAT32 stores each byte of a file in a new byte address in the disc; So, it can address 4 billion bytes = 4 GB of max file-size. This 4 GB RAM limit are avoided by 64-bit CPUs and 64-bit OSs. A 64-bit CPU/OS can keep track of 264 =18,446,744,073,709,551,616 byte (18 EB, i.e., 18 Giga GB) of RAM and 9 ZB (9 Giga TB) partition of HDD. In order to avail the 64-bit computing advantages, one must have both 64-bit CPU plus 64-bit OS which can run both 64-bit software and 32-bit software. 32-bit CPU cannot run 64-bit OS and 64-bit Software. 64-bit CPU can run 32-bit OS but 32-bit OS cannot run 64-bit Software. 32 Address Primary Memory Value UNIT SYMBOL POWER Number of OF 2 bytes Byte 0 1 2 Kilobyte KB 10 1,024 2 Megabyte MB 20 1,048,576 2 Gigabyte GB 30 1,073,741,824 2 Terabyte TB 40 1,099,511,627,776 2 33 Primary Memory RAM is the main working area of the CPU. Each RAM byte has got two very important properties for the programmers to understand clearly. One is its address (location), another is its value (content). All that a CPU does is reading value(s) from the pointed (addressed) RAM byte(s), (process it internally using its registers) or writing value(s) to the pointed (addressed) RAM byte(s) Each bit of information (programming instructions/data) for processing by the CPU is fetched from the RAM or stored back to the RAM after/or during processing. Not only that, every devices communicates to the CPU via its reserved area inside RAM (allocated by the OS). 34 Primary Memory Since RAM is volatile, (i.e., it loses all the instructions/data once the power is off), secondary persistent storage devices such as hard disk, usb flash drives, etc. are used to store the programming instructions/data permanently. However, for any processing to be done by the CPU, all the programming instructions/data must be loaded into the RAM byte(s). Usually in programming, the values contained into the RAM bytes are of general interest to the programmer. However in order to point (instruct) the CPU to manipulate the value(s) of the desired RAM byte(s), its address is to be mentioned by the programmer. 35 Primary Memory This becomes clumsy for the programmers to remember the addresses of the RAM bytes to manipulate their values. So All the programming languages provide symbolic names for pointing to the RAM byte(s) called ‘identifiers’. An ‘identifier’ points to the address of a particular RAM byte. A very common example of an identifier is a variable used in a program. A (named) variable is linked to a particular RAM byte by the OS (as designated by the compiler) during execution of the program. A programmer can easily mention the variable name and manipulate that particular RAM byte by the CPU. Identifiers other than variables do exist. Examples are, (named) constants, functions, labels, etc. (They point to RAM bytes) Since a variable is a symbolic link to RAM byte’s address, it also has got the same two important properties: (i) address of that RAM byte and (ii) value of that RAM byte. 36 Software Software is the programs and data that a computer uses. Programs are lists of instructions for the processor Data can be any information that a program needs: character data, numerical data, image data, audio data, etc. Both programs and data are saved in computer memory in the same way. Computer software is divided into two main categories: 1. Systems software 2. Applications software System software manages computer resources and makes computers easy to use. Examples are Operating Systems, Antivirus softwares, Disk utility programs, Networking softwares, etc. An applications software enables a computer to be used to do a particular task. 37 Operating Systems The most important systems program is the operating system. It is a group of programs that coordinates the operation of all the hardware and software components of the computer system. It is responsible for starting application programs running and finding the resources that they need. Examples of operating systems are: Windows 11/10, Windows 7/XP, Linux, Unix, Mac OS X, Android, iOS, iPadOS, Chrome OS, etc. 38 Computer Software Hardware Abstraction Layer (HAL) Types of software 39 Hardware Abstraction Layer (HAL) The core component of an OS is its ‘kernel’. Kernel consists of hardware device drivers which control the hardware at their lowest level. OSs also provide a set of API libraries/routines/framework to the user at a higher level to use those hardware in an efficient way, thus increasing the productivity of the programmer. Through kernel and APIs, OSs implement Hardware Abstraction Layer (HAL) to facilitate the usage of computer by the user/programmer. HAL removes the hardships to control the hardware at their lowest levels. HAL also prevents direct access to the hardware by the user/programmer. With the evolution of the OSs, the HAL is growing thicker* and creating more restrictions* for the user to directly access the hardware but providing more and more API libraries/routines/framework to increase productivity. [ *Android ‘rooting’ or iOS ‘jailbreaking’ refers to the circumvention (like creating a ‘hole’ through the HAL layer) of these hardware as well as software restrictions. Rooting or jailbreaking allows the programmer to install 3rd-party software and make direct access to different hardware components of the smartphones and tablets. ] 40 Software Application Programs Systems Programs Word processors Operating System Game programs Networking System Spreadsheets Internet Security System Database programs Data Backup System Graphics programs Online Payment Gateway System Programming Language IDEs Email Server Web browsers Web Server Open-source software are the software with source code. It’s about the code which anyone can inspect and modify. It emphasizes collaboration and transparency. Examples: Linux: An open-source operating system kernel and Firefox: An open-source web browser. Open-source software are usually free. Free software are software that are free to use and distribute. It’s about the price. Examples: GNU Emacs: A free text editor and LibreOffice: A free office-suite. Free software are not essentially open-source. 41 Computer Languages (Abstraction) All the existing Computer programming languages can be broadly categorized into three groups: Low Level Language (Machine Language) – Consists of patterns of 1’s and 0’s. No conversion required. Readily understood and executed by the CPU. But very difficult to program. Moreover, machine language of one CPU architecture (x86) is not understood by another CPU architecture (ARM). Computer language evolution Mid Level Language (Assembly Language or Symbolic Language) – Consists of mnemonics (symbolic names for bit patterns). Easier to program. Must be converted into machine language for the CPU to understand and execute the program. High level Language – Consists of human language like words and symbols. Much easier to program. Must be converted into machine language for the CPU to understand and execute the program. Examples are Fortran, C, C++, Java, Python, Matlab, etc. Computer Languages (Abstraction) 42 Computer Language Evolution (Chronology) Application Type (Usage): General-purpose (C, C++), Scientific (Matlab), Database (SQL), Scripting (Javascript, php), Artificial Intelligence/Machine Learing (Python), etc. Programming Style (Paradigm): Structured (Fortran, C), Procedural (C), Functional (Haskell), Object Oriented Programming (OOP- C++, Java, Python) Note: The only language understood by a computer is machine language. 43 Steps to Solve a Problem by Computer Programming Generally four steps are followed to solve a real-world computational problem by using a computer programming language: Step – 1: Problem Definition – General statement of the problem or the purpose of what is/are to be done with available inputs and desired outputs. Step – 2: Algorithm Development with appropriate Data Structure – Exploring the process/methodology to solve the problem and resolvingComputer the process/methodology language evolution into groups of single step sequential/logical instructions (called ‘psudocode’) executable by the CPU using appropriate data structure. Step – 3: Coding– Developing the “source code” of the solution using a suitable computer programming language. Step – 4: Compiling, Linking, Testing and Debugging the Code – Converting the source code into “machine/object code” and test run the code, check the output and errors and debug (correct) errors in the code. Then recompile, retest and debug if necessary. 44 Software Modules Required for Programming Four software modules are required to constitute a complete programming environment. Module – 1: Text Editor – A plain text editor is required to develop the source code. Examples – Notepad, Wordpad, Notepad++, WinEdit, TextEdit, vi, vim, emacs, etc. Module – 2: Compiler or Interpreter – A compiler/interpreter is required to convert the source code into machine code. Examples - gcc, g++, tcc, vcc, etc. Module – 3: Linker – A linker program links the API routines from the OS or other 3rd party library codes to the programmer’s object code. Examples – ld, link, lld, etc. Computer language evolution Module – 4: Debugger – A debugger checks for syntactical/logical errors in the code. Examples – gdb, windbg, lldb, etc. There are some software packages which provide all the four required software modules within a single user interface. These softwares are called IDE (Integrated Development Environment). Examples - Codeblocks, Dev-C++, Turbo C, MS Visual Studio, XCode, Eclipse, etc. Some online IDEs are also available where one can write code, compile and run the code within the browser. Examples are “https://www.onlinegdb.com/” for programming using C and “https://colab.research.google.com/” for using python. Compiler vs Interpreter 45 Compiler Interpreter A compiler converts the entire source code An interpreter converts one line of the source into machine code at once before runtime. code at a time during runtime. A compiler creates permanent machine code An interpreter does not create permanent (filename.o), so compiled program does not machine code. So, an interpreted program need the source code (filename.c) to run. needs its source code during each run. Compiled programs are platform dependent. Platform independent. Interpreters are in- Separate object files must be generated for built into most OSs and Web browsers, so no each platform from the source codes. So, separate interpreter is required. programmers must have compilers for those platforms. Computer language evolution Source code can be kept closed during Source code must be open for the program to distribution of the software. run. Compiled programs always run faster. So, Interpreted programs run slower. Since time critical programs such as games, platform independent, these programs are scientific simulation software, etc. are used in online (internet) programs facilitating distributed as compiled programs. the same programs to run in PCs, tablets, smartphones independent of their platforms. Languages that use compilers are Fortran, Languages that use interpreters are Java, C, C++, etc. Python, Matlab, JavaScript, php, etc.