ARM Assembly Language Programming PDF
Document Details
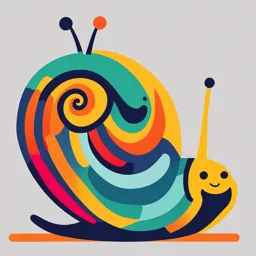
Uploaded by IlluminatingYtterbium
Tags
Summary
This document provides a detailed overview of ARM assembly language programming, focusing on data transfer instructions and addressing modes. It explains how data is transferred between registers and memory in ARM architecture. The document also includes examples and exercises.
Full Transcript
CHAPTER 3.2: ARM ASSEMBLY LANGUAGE PROGRAMMING BERN 2423: MICROPROCESSOR TECHNOLOGY DATA TRANSFER INSTRUCTIONS LOAD INSTRUCTION STORE INSTRUCTION ADDRESSING MODES 1 THANK YOU! THANK YOU! C...
CHAPTER 3.2: ARM ASSEMBLY LANGUAGE PROGRAMMING BERN 2423: MICROPROCESSOR TECHNOLOGY DATA TRANSFER INSTRUCTIONS LOAD INSTRUCTION STORE INSTRUCTION ADDRESSING MODES 1 THANK YOU! THANK YOU! Credits: All the information & knowledge shared in this course are in due thanks to all the lecturers & individuals that had taught this course – Microprocessor Technology – for it is their imparted wisdom that had spread the knowledge to all of us. Thank you! Also special thanks to various resources over the Internet, widely shared by fellow netizens, YouTubers, THANK YOU! in fact everyone on Earth! 2 CONTENT OUTLINE Introduction Load and Store Instructions Addressing Modes Base Register Addressing Mode Base Displacement Addressing Mode Offset Pre-indexed Post-indexed Conclusion 3 Exercises INTRODUCTION The ARM is a Load/Store Architecture: – Only load and store instructions can access memory – Does not support memory to memory data processing operations – Must move data values into registers before using them Data transfer instructions transfer data between registers and memory: Memory to register LDR/STR Register to memory 4 HOW CAN WE OPERATE THE DATA INSIDE MEMORY? In ARM architecture, data must be placed in the register before performing basic operations. You can’t perform operation directly to memory. Data Register Data Register Data Data Memory Memory Memory to memory data processing is not allowed because it can slow down the 5 memory access. Therefore, all data operation needs to be done in register before it can be transfer back to memory. LOAD AND STORE – THE INSTRUCTIONS The ARM has three sets of instructions which interact with main memory that are: Single register data transfer (LDR/STR) Block data transfer (LDM/STM) Single Data Swap (SWP) Load – take a single value from memory and write it to general-purpose register (MEMORY REGISTER) Store – read a value from a general-purpose register and store it to memory (REGISTER MEMORY) Memory system must support all access sizes Syntax: LDR{}{} Rd, STR{}{} Rd, Signed or not? Byte? Destination Addressing Halfword? register mode Word? 6 Example: LDR r0, [r1, #12] Load and Store (cont.) Most often used Load/Store instructions 7 COMPONENTS OF ANYCOMPUTER 8 DATATRANSFER: MEMORY TO REGISTER (LDR) To transfer a word of data, we need to specify two things: Register: r0 - r12, r13 - stack pointer, r14 - link register, r15 – PC Memory Address: How to access the memory? Think of memory as a single one-dimensional array, so we can address it simply by supplying a pointer to the memory address. Besides pointer, we also need to identify the base register where it will point to the beginning of the memory array. Base Register A register which contains a pointer to memory, example: [r0] Specifies the memory address pointed to by the value in r0 This is called register indirect addressing. 9 DATATRANSFER:MEMORY TO REGISTER (LDR) Load Instruction Syntax: Data flow LDR r1, [r0] ; Load r1 with contents of memory location pointed to by contents of r0. where 1) operation name (LDR) 2) register that will receive value (r1) 3) register containing pointer to memory/base register [r0] ARM Instruction Name: LDR (meaning Load Register, so 32-bits or one Word is loaded at a time) 1 r0 contains the memory address that it points in 2 3 the memory 0x12345678 0x12345678 0x00000200 0x00000200 r1 r0 Base register 0x00000204 The value contained in memory location 0x00000200 is loaded in r1 0x00000208 0x0000020C 10 DATATRANSFER:REGISTERTO MEMORY (STR) Store Instruction Syntax: Data flow STR r0,[r1] ; Store contents of r0 to location pointed to by contents of r1 where 1) operation name (STR) 2) register that will transfer value (r0) 3) register containing pointer to memory/base register [r1] 1 3 Base register 0x5 r1 0x00000200 0x00000200 0x00000204 0x00000208 2 0x0000020C r0 contains value 0x5 Write the value 0x5 contained in r0 to 11 r0 0x5 memory location pointed to by r1 LOAD AND STORE(CONT.) 12 LOAD LDR Example: Consider the instruction, LDR r9, [r0] ; load a Word into r9 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: before LOAD r0 0×00006000 Address Memory 0×6000 0×EE r9 0×12345678 0×6001 0×FF after LOAD 0×6002 0×80 r0 0×6003 0×A7 13 r9 LOAD LDR Example: Consider the instruction, LDR r9, [r0] ; load a Word into r9 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: before LOAD r0 0×00006000 Address Memory 0×6000 0×EE r9 0×12345678 0×6001 0×FF after LOAD 0×6002 0×80 r0 0×00006000 0×6003 0×A7 14 r9 0×A780FFEE LOAD (CONT.) LDRB Example: Consider the instruction, LDRB r9, [r0] ; load a Byte into r9 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: before LOAD r0 0×00006000 Address Memory 0×6000 0×EE r9 0×12345678 0×6001 0×FF after LOAD 0×6002 0×80 r0 0×6003 0×A7 15 r9 LOAD (CONT.) LDRB Example: Consider the instruction, LDRB r9, [r0] ; load a Byte into r9 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: before LOAD r0 0×00006000 Address Memory 0×6000 0×EE r9 0×12345678 0×6001 0×FF after LOAD 0×6002 0×80 r0 0×00006000 0×6003 0×A7 16 r9 0×000000EE LOAD (CONT.) LDRH Example: Consider the instruction LDRH r9, [r0] ; load a Halfword into r9 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: Address Memory r9 before LOAD 0×12345678 0×6000 0×EE r9 after LOAD 0×6001 0×FF 0×6002 0×80 17 0×6003 0×A7 LOAD (CONT.) LDRH Example: Consider the instruction LDRH r9, [r0] ; load a Halfword into r9 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: Address Memory r9 before LOAD 0×12345678 0×6000 0×EE r9 after LOAD 0×6001 0×FF 0×0000FFEE 0×6002 0×80 18 0×6003 0×A7 LOAD (CONT.) LDRSB Example: Consider the instruction, LDRSB r9, [r0] ; load Signed-Byte into r9 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: before LOAD r0 0×00006000 Address Memory 0×6000 0×EE r9 0×12345678 0×6001 0×8C after LOAD 0×6002 0×80 0×A7 19 r0 0×6003 r9 LOAD (CONT.) LDRSB Example: Consider the instruction, LDRSB r9, [r0] ; load Signed-Byte into r9 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: before LOAD r0 0×00006000 Address Memory 0×6000 0×EE r9 0×12345678 0×6001 0×8C after LOAD 0×6002 0×80 0×A7 20 r0 0×00006000 0×6003 r9 0×FFFFFFEE LOAD (CONT.) LDRSH Example: Consider the instruction, LDRSH r9, [r0] ; load Signed-Halfword into r9 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: before LOAD r0 0×00006000 Address Memory 0×6000 0×EE r9 0×12345678 0×6001 0×8C 0×6002 0×80 after LOAD r0 0×6003 0×A7 21 r9 LOAD (CONT.) LDRSH Example: Consider the instruction, LDRSH r9, [r0] ; load Signed-Halfword into r9 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: before LOAD r0 0×00006000 Address Memory 0×6000 0×EE r9 0×12345678 0×6001 0×8C 0×6002 0×80 after LOAD r0 0×00006000 0×6003 0×A7 22 r9 0×FFFF8CEE STORE STR Example: Consider the instruction, STR r9, [r0] ; Store Word from r9 to location pointed to by contents of r0 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: before STORE before STORE after STORE r0 0×00006000 Address Memory Address Memory r9 0×12345678 0×6000 0×BE 0×6000 0×6001 0×BA 0×6001 after store 0×6002 0×ED 0×6002 r0 0×6003 0×FE 0×6003 r9 23 STORE (CONT.) STR Example: Consider the instruction, STR r9, [r0] ; Store Word from r9 to location pointed to by contents of r0 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: before STORE before STORE after STORE r0 0×00006000 Address Memory Address Memory r9 0×12345678 0×6000 0×BE 0×6000 0×78 0×6001 0×BA 0×6001 0×56 after store 0×6002 0×ED 0×6002 0×34 r0 0×00006000 0×6003 0×FE 0×6003 0×12 r9 0×12345678 24 STORE (CONT.) STRB Example: Consider the instruction, STRB r9, [r0] ; Store Byte from r9 to location pointed to by the contents of r0 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: before STORE before STORE after STORE r0 0×00006000 Address Memory Address Memory r9 0×12345678 0×6000 0×BE 0×6000 0×6001 0×BA 0×6001 after STORE 0×6002 0×ED 0×6002 r0 0×6003 0×FE 0×6003 25 r9 STORE (CONT.) STRB Example: Consider the instruction, STRB r9, [r0] ; Store Byte from r9 to location pointed to by the contents of r0 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: before STORE before STORE after STORE r0 0×00006000 Address Memory Address Memory r9 0×12345678 0×6000 0×BE 0×6000 0×78 0×6001 0×BA 0×6001 0×BA after STORE 0×6002 0×ED 0×6002 0×ED r0 0×00006000 0×6003 0×FE 0×6003 0×FE 26 r9 0×12345678 STORE (CONT.) STRH Example: Consider the instruction, STRH r9, [r0] ; Store Halfword from r9 to location pointed to by contents of r0 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: Before STORE Before STORE After STORE r0 0×00006000 Address Memory Address Memory r9 0×12345678 0×6000 0×BE 0×6000 0×6001 0×BA 0×6001 after STORE 0×6002 0×ED 0×6002 r0 0×6003 0×FE 0×6003 27 r9 STORE (CONT.) STRH Example: Consider the instruction, STRH r9, [r0] ; Store Halfword from r9 to location pointed to by contents of r0 Assuming the address in register r0 is 0x6000, before and after the instruction is executed, the data appear as follows: Before STORE Before STORE After STORE r0 0×00006000 Address Memory Address Memory r9 0×12345678 0×6000 0×BE 0×6000 0×78 0×6001 0×BA 0×6001 0×56 after STORE 0×6002 0×ED 0×6002 0×ED r0 0×00006000 0×6003 0×FE 0×6003 0×FE 28 r9 0×12345678 ADDRESSING MODES There are many ways in ARM to specify the address; these are called addressing modes. Two basic classification: 1. Base register Addressing E.g: LDR r0,[r1] Known as register indirect addressing mode Register holds the 32-bits memory address Also called as the base address 2. Base Displacement Addressing mode Offset (immediate, register, scaled register) Pre-indexed (immediate, register, scaled register) Post-indexed (immediate, register, scaled register) An effective address is calculated as: Effective address = < Base address + base displacement > 29 *Altogether there are 10 different addressing modes ADDRESSING MODES (CONT.) Offset: can take any values between -4095 and +4095, 12 bits (212) 1. Immediate offset, [Rn, #±imm] Address accessed is imm more/less than the address found in Rn. Rn does not change. Example: ldr r2, [r1, #12] ; r2:= mem32[r1+12] 1210 = C16 before LOAD Address Memory r1 0×00006000 Base address 0×00006000 0×000000BE r2 0×12345678 0×00006004 0×000000BA 0×00006008 0×000000ED after LOAD Effective address 0×0000600C 0×000000FE r1 30 r2 ADDRESSING MODES (CONT.) Offset: can take any values between -4095 and +4095, 12 bits (212) 1. Immediate offset, [Rn, #±imm] Address accessed is imm more/less than the address found in Rn. Rn does not change. Example: ldr r2, [r1, #12] ; r2:= mem32[r1+12] 1210 = C16 before LOAD Address Memory r1 0×00006000 Base address 0×00006000 0×000000BE r2 0×12345678 0×00006004 0×000000BA 0×00006008 0×000000ED after LOAD Effective address 0×0000600C 0×000000FE r1 0×00006000 31 r2 0×000000FE r1 value will not be changed by immediate offset ADDRESSING MODES (CONT.) Example: Immediate offset for STR STR r0, [r1, #-8] ; Store Word from r0 to location pointed to by contents of [r1, #-8] before STORE before STORE after STORE r1 0×00006000 Address Memory Address Memory r0 0×12345678 0×00005FF8 0×000000BE Effective address 0×00005FF8 0×00005FFC 0×000000BA -8 0×00005FFC after STORE 0×00006000 0×000000ED Base address 0×00006000 r1 0×00006004 0×000000FE 0×00006004 r0 32 ADDRESSING MODES (CONT.) Example: Immediate offset for STR STR r0, [r1, #-8] ; Store Word from r0 to location pointed to by contents of [r1, #-8] before STORE before STORE after STORE r1 0×00006000 Address Memory Address Memory r0 0×12345678 0×00005FF8 0×000000BE Effective address 0×00005FF8 0×12345678 0×00005FFC 0×000000BA -8 0×00005FFC 0×000000BA after STORE 0×00006000 0×000000ED Base address 0×00006000 0×000000ED r1 0×00006000 0×00006004 0×000000FE 0×00006004 0×000000FE r0 0×12345678 r1 value will not be changed by immediate offset 33 ADDRESSING MODES (CONT.) 2. Register offset, [Rn, ±Rm] Address accessed is the value in Rn ± the value in Rm. Rn and Rm do not change values. This is just shorthand for [Rn, ±Rm, LSL #0]. before LOAD Address Memory r0 0×00006000 Base address 0×00006000 0×000000BE r1 0×0000000C 0×00006004 0×000000BA 0×00006008 0×000000ED r2 0×12345678 Effective address 0×0000600C 0×000000FE after LOAD r0 r1 34 r2 ADDRESSING MODES (CONT.) 2. Register offset, [Rn, ±Rm] Address accessed is the value in Rn ± the value in Rm. Rn and Rm do not change values. This is just shorthand for [Rn, ±Rm, LSL #0]. before LOAD Address Memory r0 0×00006000 Base address 0×00006000 0×000000BE r1 0×0000000C 0×00006004 0×000000BA 0×00006008 0×000000ED r2 0×12345678 Effective address 0×0000600C 0×000000FE after LOAD r0 0×00006000 r0 and r1 do not change r1 0×0000000C values 35 r2 0×000000FE r2 is updated with the value contained in the effective address ADDRESSING MODES (CONT.) Example: STR r2, [r0, r1] ; Store word from r2 to location pointed to by contents of [r0, r1] before STORE before STORE after STORE r0 0×00006000 Address Memory Address Memory 0×00006000 0×000000BE Base address 0×00006000 r1 0×0000000C 0×00006004 0×000000BA 0×00006004 r2 0×12345678 0×00006008 0×000000ED 0×00006008 0×0000600C 0×000000FE Effective address 0×0000600C after STORE r0 r1 r2 36 ADDRESSING MODES (CONT.) Example: STR r2, [r0, r1] ; Store word from r2 to location pointed to by contents of [r0, r1] before STORE before STORE after STORE r0 0×00006000 Address Memory Address Memory 0×00006000 0×000000BE Base address 0×00006000 0×000000BE r1 0×0000000C 0×00006004 0×000000BA 0×00006004 0×000000BA r2 0×12345678 0×00006008 0×000000ED 0×00006008 0×000000ED 0×0000600C 0×000000FE Effective address 0×0000600C 0×12345678 after STORE r0 0×00006000 r1 0×0000000C r0, r1 and r2 do not change values r2 0×12345678 37 ADDRESSING MODES (CONT.) 3. Scaled register offset, [Rn, ±Rm, Shift] Address accessed is the value in Rn ± the value in Rm shifted as specified. Rn and Rm do not change values. LSL: Logical Shift Left Shifts left by the specified amount (multiplies by powers of two) e.g. LSL #5 = multiply by 32 LSR: Logical Shift Right Shifts right by the specified amount (divides by powers of two) e.g. LSR #5 = divide by 32 ASR: Arithmetic Shift Right (same as LSR) ASR #5 = divide by 32 38 ADDRESSING MODES (CONT.) Example: LDR r0, [r1, r2, LSL #2] before LOAD Address Memory r0 0×12345678 Base address = 0×00006000 0×000000BE. r2×22 = C16×4 = 3016 r1 0×00006000.. r1 + 3016 = 0×00006030 r2 0×0000000C Effective address = 0×00006030 0×000000FE after LOAD r0 r1 r2 39 ADDRESSING MODES (CONT.) Example: LDR r0, [r1, r2, LSL #2] before LOAD Address Memory r0 0×12345678 Base address = 0×00006000 0×000000BE. r2×22 = C16×4 = 3016 r1 0×00006000.. r1 + 3016 = 0×00006030 r2 0×0000000C Effective address = 0×00006030 0×000000FE after LOAD r0 0×000000FE Copy the value hold by effective adress r1 0×00006000 The value of r1 does not change r2 0×0000000C 40 ADDRESSING MODES (CONT.) Example: STR r0, [r1, r2, LSL #2] ; Store word from r0 to location pointed to by contents of [r1, r2, LSL #2] before STORE before STORE after STORE r0 0×12345678 Address Memory Address Memory 0×00006000 0×000000BE Base address = 0×00006000 0×000000BE r1 0×00006000...... r2 0×0000000C 0×00006030 0×000000FE after STORE r0 r1 r2 41 ADDRESSING MODES (CONT.) Example: STR r0, [r1, r2, LSL #2] ; Store word from r0 to location pointed to by contents of [r1, r2, LSL #2] before STORE before STORE after STORE r0 0×12345678 Address Memory Address Memory 0×00006000 0×000000BE Base address = 0×00006000 0×000000BE r1 0×00006000...... r2 0×0000000C 0×00006030 0×000000FE Effective address = 0×00006030 0×12345678 after STORE r0 0×12345678 r0 remain the same r2×22 = C16×4 = 3016 r1 0×00006000 value of r1 does not change r1 + 3016 = 0×00006030 r2 0×0000000C 42 ADDRESSING MODES (CONT.) Pre-index addressing 4. Immediate pre-indexed, [Rn, #±imm]! Address accessed is as with immediate offset mode, but Rn's value updates to become the address accessed. 43 ADDRESSING MODES (CONT.) Example: LDR r0, [r1, #4]! before LOAD r0 0×00000000 Address Memory r1 0×00009000 Base address = 0×00009000 0×01010101 Effective address = 0×00009004 0×02020202 after LOAD r0 r1 44 ADDRESSING MODES (CONT.) Example: LDR r0, [r1, #4]! before LOAD r0 0×00000000 Address Memory r1 0×00009000 Base address = 0×00009000 0×01010101 Effective address = 0×00009004 0×02020202 after LOAD r0 0×02020202 r1 0×00009004 The base register is updated with the immediate offset 45 ADDRESSING MODES (CONT.) Example: STR r0, [r5, # -4]! ; Store word from r0 to location pointed to by contents of [r5, #-4]! before STORE before STORE after STORE r5 0×00006000 Address Memory Address Memory r0 0×12345678 0×00005FF8 0×000000BE 0×00005FF8 0×00005FFC 0×000000BA Effective address 0×00005FFC after STORE -4 0×00006000 0×000000ED Base address 0×00006000 r5 0×00006004 0×000000FE 0×00006004 r0 46 ADDRESSING MODES (CONT.) Example: STR r0, [r5, # -4]! ; Store word from r0 to location pointed to by contents of [r5, #-4]! before STORE before STORE after STORE r5 0×00006000 Address Memory Address Memory r0 0×12345678 0×00005FF8 0×000000BE 0×00005FF8 0×000000BE 0×00005FFC 0×000000BA Effective address 0×00005FFC 0×12345678 after STORE -4 0×00006000 0×000000ED Base address 0×00006000 0×000000ED r5 0×00005FFC 0×00006004 0×000000FE 0×00006004 0×000000FE r0 0×12345678 The base register is updated with the immediate offset 47 ADDRESSING MODES (CONT.) Pre-index addressing 5. Register pre-indexed, [Rn, ±Rm]! Address accessed is as with register offset mode, but Rn's value updates to become the address accessed. The optional “!” specifies writing the effective address back into Rn at the end of the instruction. Without it, Rn contains its original value after the instruction executes. This type of incrementing is useful in stepping through tables or lists, since 48 the base address is automatically updated for us. ADDRESSING MODES (CONT.) Example: LDR r2,[r0, r1]! before LOAD Address Memory r0 0×00006000 0×00006000 0×000000BE r1 0×0000000C 0×00006004 0×000000BA 0×00006008 0×000000ED r2 0×12345678 0×0000600C 0×000000FE after LOAD r0 r1 r2 49 ADDRESSING MODES (CONT.) Example: LDR r2,[r0, r1]! before LOAD Address Memory r0 0×00006000 Base address = 0×00006000 0×000000BE r1 0×0000000C 0×00006004 0×000000BA 0×00006008 0×000000ED r2 0×12345678 Effective address = 0×0000600C 0×000000FE after LOAD r0 0×0000600C The base register is updated with the register value r1 0×0000000C r2 0×000000FE r2 is updated with the value contained in the effective address 50 ADDRESSING MODES (CONT.) Example: STR r2,[r0, r1]! ; Store word from r0 to location pointed to by contents of [r0, r1]! before STORE before STORE after STORE r0 0×00006000 Address Memory Address Memory 0×00006000 0×000000BE 0×00006000 r1 0×0000000C 0×00006004 0×000000BA 0×00006004 r2 0×12345678 0×00006008 0×000000ED 0×00006008 0×0000600C 0×000000FE 0×0000600C after STORE r0 r1 r2 51 ADDRESSING MODES (CONT.) Example: STR r2,[r0, r1]! ; Store word from r0 to location pointed to by contents of [r0, r1]! before STORE before STORE after STORE r0 0×00006000 Address Memory Address Memory 0×00006000 0×000000BE Base address 0×00006000 0×000000BE r1 0×0000000C 0×00006004 0×000000BA 0×00006004 0×000000BA r2 0×12345678 0×00006008 0×000000ED 0×00006008 0×000000ED 0×0000600C 0×000000FE Effective address 0×0000600C 0×12345678 after STORE The content of base register r0 is r0 0×0000600C updated to become effective address r1 0×0000000C r1 and r2 do not r2 0×12345678 change values 52 ADDRESSING MODES (CONT.) 6. Scaled register pre-indexed, [Rn, ±Rm, shift]! Address accessed is as with scaled register offset mode, but Rn's value updates to become the address accessed. 53 ADDRESSING MODES (CONT.) Example: LDR r0, [r1, r2, LSL #2]! before LOAD r0 0×12345678 Address Memory Base address 0×00006000 r2×22 = C16×4 = 3016 r1 0×00006000.. r1 + 3016 = 0×00006030. r2 0×0000000C Effective address 0×00006030 after LOAD r0 r1 r2 54 ADDRESSING MODES (CONT.) Example: LDR r0, [r1, r2, LSL #2]! before LOAD r0 0×12345678 Address Memory Base address = 0×00006000 0×000000BE r2×22 = C16×4 = 3016 r1 0×00006000.. r1 + 3016 = 0×00006030. r2 0×0000000C Effective address = 0×00006030 0×000000FE after LOAD r0 0×000000FE Copy the value hold by effective adress r1 0×00006030 The value of r1 is updated with the effective address r2 0×0000000C 55 ADDRESSING MODES (CONT.) Example: STR r0, [r1, r2, LSL #2]! ; Store word from r0 to location pointed to by contents of [r1, r2, LSL#2]! Before store after STORE before STORE r0 0×12345678 Address Memory Address Memory 0×00006000 0×000000BE Base address 0×00006000 r1 0×00006000...... r2 0×0000000C 0×00006030 0×000000FE Effective address 0×00006030 after STORE r0 r2×22 = C16×4 = 3016 r1 r1 + 3016 = 0×00006030 r2 56 ADDRESSING MODES (CONT.) Example: STR r0, [r1, r2, LSL #2]! ; Store word from r0 to location pointed to by contents of [r1, r2, LSL#2]! before STORE before STORE after STORE r0 0×12345678 Address Memory Address Memory 0×00006000 0×000000BE Base address 0×00006000 0×000000BE r1 0×00006000...... r2 0×0000000C 0×00006030 0×000000FE Effective address 0×00006030 0×12345678 after STORE r0 0×12345678 r0 remain the same r2×22 = C16×4 = 3016 The value of r1 is updated with the r1 0×00006030 r1 + 3016 = 0×00006030 effective address r2 0×0000000C 57 ADDRESSING MODES (CONT.) Post-index addressing 7. Immediate post-indexed, [Rn], #±imm Address accessed is value found in Rn, and then Rn's value is increased / decreased by imm. This is called: post-indexed addressing - the base address is used without an offset as the transfer address, after which it is auto-indexed:(r1=r1+4) 58 ADDRESSING MODES (CONT.) Example: LDR r2, [r1], #4 ; loads r2 with the contents of the address pointed to by r1 and then increments r1 by 4 before LOAD Address Memory r2 0×FEEDBABE Base address 0×8000 0×AB r1 0×00008000 0×8001 0×CD 0×8002 0×EF 0×8003 0×00 after LOAD r2 r1 59 ADDRESSING MODES (CONT.) Example: LDR r2, [r1], #4 ; loads r2 with the contents of the address pointed to by r1 and then increments r1 by 4 before LOAD Address Memory r2 0×FEEDBABE Base address 0×8000 0×AB r1 0×00008000 0×8001 0×CD 0×8002 0×EF 0×8003 0×00 after LOAD Content of r2 is updated with the r2 0×00EFCDAB word contained in the base register r1 0×00008004 After loading the value of r1(base 60 register) into r2, only then you can update the base register according to the immediate value ADDRESSING MODES (CONT.) Example: STR r3, [r8], #4 ;store word from location r3 to the address pointed by r8 and then increment r8 by 4 before STORE after STORE before STORE Address Memory Address Memory r3 0×FEEDBABE Base address = 0×8000 0×AB Base address 0×8000 r8 0×00008000 0×8001 0×CD 0×8001 0×8002 0×EF 0×8002 0×8003 0×00 0×8003 after STORE r3 r8 61 ADDRESSING MODES (CONT.) Example: STR r3, [r8], #4 ;store word from location r3 to the address pointed by r8 and then increment r8 by 4 before STORE after STORE before STORE Address Memory Address Memory r3 0×FEEDBABE Base address = 0×8000 0×AB Base address = 0×8000 0×BE r8 0×00008000 0×8001 0×CD 0×8001 0×BA 0×8002 0×EF 0×8002 0×ED 0×8003 0×00 0×8003 0×FE after STORE r3 0×FEEDBABE After storing the value of r3 into r8 (base register), only then you can r8 0×00008004 update the base register according 62 to the immediate value ADDRESSING MODES (CONT.) 8. Register post-indexed, [Rn], ±Rm Address accessed is value found in Rn, and then Rn's value is increased / decreased by Rm. 63 ADDRESSING MODES (CONT.) Example LDR r0, [r1], r2 before LOAD r0 0×12345678 Address Memory Base address 0×00006000 0×000000BE r1 0×00006000 0×00006004 0×000000BA r2 0×0000000C 0×00006008 0×000000ED 0×0000600C 0×000000FE after LOAD r0 r1 r2 64 ADDRESSING MODES (CONT.) Example LDR r0, [r1], r2 before LOAD r0 0×12345678 Address Memory Base address 0×00006000 0×000000BE r1 0×00006000 0×00006004 0×000000BA r2 0×0000000C 0×00006008 0×000000ED 0×0000600C 0×000000FE after LOAD r0 0×000000BE Load the word hold by the base address r1 0×0000600C The value of r1 is increased by r2 r2 0×0000000C 65 ADDRESSING MODES (CONT.) Example: STR r0, [r1], r2 ; store word from location r0 to the address pointed by r1 and then increment r1 by r2 before STORE After STORE before STORE r0 0×12345678 Address Memory Address Memory 0×00006000 0×000000BE Base address 0×00006000 r1 0×00006000 0×00006004 0×000000BA 0×00006004 r2 0×0000000C 0×00006008 0×000000ED 0×00006008 0×0000600C 0×000000FE 0×0000600C after STORE r0 r1 r2 66 ADDRESSING MODES (CONT.) Example: STR r0, [r1], r2 ; store word from location r0 to the address pointed by r1 and then increment r1 by r2 before STORE After STORE before STORE r0 0×12345678 Address Memory Address Memory 0×00006000 0×000000BE Base address 0×00006000 0×12345678 r1 0×00006000 0×00006004 0×000000BA 0×00006004 0×000000BA r2 0×0000000C 0×00006008 0×000000ED 0×00006008 0×000000ED 0×0000600C 0×000000FE 0×0000600C 0×000000FE after STORE r0 0×12345678 r0 remain the same r1 0×0000600C The value of r1 is increased by r2 r2 0×0000000C 67 ADDRESSING MODES (CONT.) 9. Scaled register post-indexed, [Rn],±Rm, shift Address accessed is the value found in Rn, and then Rn's value is increased / decreased by Rm shifted according to shift. This is just shorthand for [Rn], ±Rm, LSL #0 68 ADDRESSING MODES (CONT.) Example: LDR r0, [r1], r2, LSL #2 before LOAD r0 0×12345678 Address Memory Base address 0×00006000 0×000000BE r2×22 = C16×4 = 3016 r1 0×00006000... r1 + 3016 = 0×00006030 r2 0×0000000C Effective address after LOAD r0 r1 r2 69 ADDRESSING MODES (CONT.) Example: LDR r0, [r1], r2, LSL #2 before LOAD r0 0×12345678 Address Memory Base address 0×00006000 0×000000BE r2×22 = C16×4 = 3016 r1 0×00006000... r1 + 3016 = 0×00006030 r2 0×0000000C Effective address 0×00006030 0×000000FE after LOAD r0 0×000000BE Copy the value hold by base adress r1 r1 0×00006030 The value of r1 is updated with the effective address r2 0×0000000C 70 ADDRESSING MODES (CONT.) Example: STR r0, [r1], r2, LSL #2 before STORE before STORE after STORE r0 0×12345678 Address Memory Address Memory 0×00006000 0×000000BE Base address 0×00006000 r1 0×00006000...... r2 0×0000000C 0×00006030 0×000000FE Effective address0×00006030 after STORE r0 r2×22 = C16×4 = 3016 r1 r1 + 3016 = 0×00006030 r2 71 ADDRESSING MODES (CONT.) Example: STR r0, [r1], r2, LSL #2 before STORE before STORE after STORE r0 0×12345678 Address Memory Address Memory 0×00006000 0×000000BE Base address 0×00006000 0×12345678 r1 0×00006000...... r2 0×0000000C 0×00006030 0×000000FE Effective address0×00006030 0×000000FE after STORE r0 0×12345678 r0 remain the same r2×22 = C16×4 = 3016 The value of r1 is updated with the r1 0×00006030 r1 + 3016 = 0×00006030 effective address r2 0×0000000C 72 CONCLUSION: COMPARISON OF ADDRESSING MODES / INDEXING ARM Addressing Modes Indexing ARM Assembly Code Effective Base Mode Address Register Offset LDR r0, [r1, r2] r1 + r2 Unchanged Pre-index LDR r0, [r1, r2]! r1 + r2 r1 = r1 + r2 Post-index LDR r0, [r1], r2 r1 r1 = r1 + r2 73 EXAMPLES OF PRE-AND POST- INDEXEDADDRESSING 74 EXERCISES 1. Describe the contents of register r9 after the following instructions completed, assuming that the memory contains values shown below. Register r0 contains 0x30, and the memory system is little-endian. Address Memory 0×30 0×06 0×31 0×FC 0×32 0×03 0×33 0×FF a. LDRSB r9, [ r 0 ] b. LDR r9, [ r 0 ] c. LDRSH r9, [ r 0 ] 75 d. LDRB r9, [ r 0 ] EXERCISES (CONT.) 2. Assume register r4 contains 0×6000. What would the register contain after executing the following instructions? a. STR r6, [r4, # 1 2 ] b. STRB r7, [r4], # 4 c. LDRH r5, [r4], # 8 d. LDR r12, [r4, #12]! 3. Assuming you have a little-endian memory system, what would register r9 contains after executing the following instructions? Register r6 holds the value 0×ABCDEFGH and register r3 holds 0×6000. STR r6, [ r 3 ] LDRB r9, [ r 3 ] 76 EXERCISES (CONT.) 1. Copy LDR r1, =TABLE1 ; TABLE1 = 0x0002 0000 2. LDR r2, =TABLE2 ; TABLE2 = 0x0004 0000 3. LDR r0, [r1] ; load first value …. 4. STR r0, [r2] ; and store it in TABLE2 5. LDR r0, [r1, #4] ; load second value 6. STR r0, [r2, #4] ; and store it (all in hexadecimal) After r0 r1 r2 0002 0000- 0002 0004- 0004 0000- 0004 0004- Line 0002 0003 0002 0007 0000 4003 0004 0007 1 0000 0000 0002 0000 0000 0000 1357 2468 A123 B246 0 0 2 3 4 5 6 77 50 r1,r2 will NOT be changed by pre-indexed addressing instructions EXERCISES (CONT.) 1.Copy LDR r1, =TABLE1 ; TABLE1 = 0x0002 0000 2. LDR r2, =TABLE2 ; TABLE2 = 0x0004 0000 3. LDR r0, [r1] ; load first value …. 4. STR r0, [r2] ; and store it in TABLE2 5. LDR r0, [r1, #4]! ; load second value, r1 will change 6. STR r0, [r2, #4]! ; and store it, r2 will change too After r0 r1 r2 0002 0000- 0002 0004- 0004 0000- 0004 0004- Line 0002 0003 0002 0007 0004 0003 0004 0007 1 0000 0000 0002 0000 0000 0000 1357 2468 A123 B246 0 0 2 3 4 5 6 78 r1,r2 will be changed by pre-indexed addressing instructions 51 (all in hexadecimal)