Introduction Of C Programming PDF
Document Details
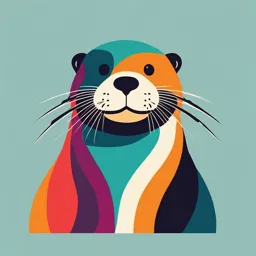
Uploaded by EnchantedJadeite1592
Amrita Vishwa Vidyapeetham
Tags
Summary
This document is an introduction to C programming, focusing on procedural programming concepts. It covers topics such as structured programming, operators, and data types in C. The document is well-structured and clearly explains the basics of the language, making it suitable for introductory-level readers.
Full Transcript
23CSE201 PROCEDURAL PROGRAMMING USING C STRUCTURED PROGRAMMING LANGUAGE Program is a set of instruction which is written in any language on which computer can understand. Structured programming facilitates program understanding and modification and has a top – down approach, where a system...
23CSE201 PROCEDURAL PROGRAMMING USING C STRUCTURED PROGRAMMING LANGUAGE Program is a set of instruction which is written in any language on which computer can understand. Structured programming facilitates program understanding and modification and has a top – down approach, where a system is divided into subsystems or into small logical modules. Also known as Modular Programming Language. ALGOL, PASCAL, C Programming are example of Structured Programming Language. C is Structured/ Procedure/ Modular Programming Language because C language is divided into small logical functions or structures. STRUCTURED PROGRAMMING LANGUAGE C / Computer programming is the process of designing and building an executable computer program for completing a specific computing task. C Programming is Middle Level and General – purpose programming language that is used for developing portable applications. C Programming is language developed in 1972 by “Dennis M. Ritchie” at the bell telephone company APPLICATIONS OF C PROGRAMMING Operating Systems System Programming Network Programming Game Development IoT (Internet of Things) Telecommunications POP VS OOP In procedural programming, data and functions are treated separately, while OOP promotes encapsulation by bundling data and methods together within classes. Procedural programming follows a top-down approach, whereas OOP promotes a bottom-up approach, where objects collaborate to achieve a task. OOP POP FEATURES Simple and clear Small in Size Structured Language (Top-Down Approach) Middle Level Language Portable Modularity Case Sensitive Easy Error Detection Building block for many other Programming Languages Powerful and Efficient Language (Robustness) Built- in Functions and Keywords BASIC STRUCTURE OF C PROGRAM BASIC STRUCTURE OF C PROGRAM 1.Documentation Section Consists of a Set of Comment Line Giving the Name of Program. 2. Link Section Provide instruction to compiler to link function from system. (#include) 3.Definition Section is used to defines all Symbolic Constants. (i.e. #define PI 3.14) 4.Global Declaration Section Used to declare variable globally. - This section also declares the entire user define function. 5.main () Function Section - Every C program must have one main() function. This Section contains two parts; - Declaration part declares all the variables used in the executable part. 6. Subprogram Section contains all the user defines function that are called in the main function. EXAMPLE OF C PROGRAM OR STANDARD DIRECTORIES IN C The C Standard Directories / Libraries provides many inbuilt functions, macros, string handling functions, math functions, input/output processing functions, memory management and many more services. is Mandatory Header file in C programming; is used to call printf( ) and scanf( ) functions. is optional; and it’s use to call getch( ) and clrscr( ) type of function. is used for all string related functions like; strlen(), strcpy(), strcat() etc.. CHARACTER SET IN C 1. Letters or Alphabets includes the uppercase (A,B,C….Z) and lowercase(a,b,c….z) alphabets. 2. Digits include(0,1,2….9) numbers. 3. Special Characters includes semi-colon(;), bracket { }, single quote(‘ ’), double quotes (“ ”), +, - *, /, %, , = etc. 4. White Spaces are used to separate the words or tokens like tab(\t) and newline(\n). TOKENS IN C Tokens are the smallest elements of a program, which are meaning full to the compiler. Example of Tokens; Keywords or data type like int, float Operators (i.e. +, -, *, /, %) Punctuation marks like Semicolon (;) bracket { } etc. Constants of a program are also considered as token. KEYWORDS IN C The keywords (Reserve Words) are special words used in C programming having specific meaning and the meaning of the words cannot be changed. [32 Keywords] IDENTIFIERS IN C Identifiers are used for naming of variables, functions, array etc. These are User Defined name which Consist of Alphabets, Number, Underscore. Identifier’s name should not be same and Keywords are not used as Identifiers. The C is Case Sensitive and hence the uppercase and lowercase letters are not treated as Same (for example, a and A are different) VARIABLES IN C Variables are a Placeholder which is used to store Data Temporary. C Variable is a named location in a memory where a program can update / manipulate the data. The value of variable may get change in program. Each Variable identifies by Unique Identifier. C Variable might be belonging to any of the data type like int, float, char etc. RULES FOR VARIABLE NAME - Only uppercase and lowercase letters, digits and underscore can be used. - Variable name always begins with letter or alphabet. - Only first 32 characters are significant. - Keywords can be not be used. - Variables name are case sensitive. The count and Count are two different. - The underscore character is used to separate the word in a variable. - White spaces are not allowed in variable name. - Special Characters are not allowed. DATATYPES IN C Datatype in a C refers to the type of a variable or used for declaring variable or Function. DECLARATION OF VARIABLE The variables are used to store the data values, before storing a value into the variable it must be declared. Syntax: Datatype Variable1, Variable2,........, Variable n; Here, Datatype is one of the valid Datatype of the C and followed by the list of variables Separated by commas. The Variable1,Variable2,.....Variable n are name of variable. The semi-colon(;) shows the end of the declaration statements. Example: int a; float num1; char c1,c2,c3; INITIALIZATION OF VARIABLE After declaring variable, they are assigned values or initialize using assignment operator(=). Syntax VariableName = Value; Example: max=10; a=2.5; It is also Possible to assign the value to the variable (initialize) at the & time of declaration with following format. Syntax: data_type variable_name=constant / value; Example: int length=5; EXAMPLE OF DATATYPE #include int main() { char c=’N’; int i=123; float f=10.23; double d=345.6789; printf(“%c”,c); printf(“%d”,i); printf(“%f”,f); printf(“%lf”,d); return 0; } ADDITION OF TWO NUMBER #include int main() { int a,b,sum; a=10; b=20; sum=a+b; printf(“Addition of a and b is %d”,sum); return 0; } DYNAMIC INITIALIZATION Dynamic Initialization of Variable refers to initializing the variables at run time. i.e; Value of the variable is provided during run time. In C we can take value from user with the use of scanf( ) function. Syntax: scanf(“FormatSpecifier”, &VariableName); Example: scanf(“%d”, &a); DYNAMIC INITIALIZATION Dynamic Initialization of Variable refers to initializing the variables at run time. i.e; Value of the variable is provided during run time. In C we can take value from user with the use of scanf( ) function. Syntax: scanf(“FormatSpecifier”, &VariableName); Example: scanf(“%d”, &a); ADDITION OF TWO NUMBER #include int main() { int a,b,sum; scanf(“%d %d”,&a,&b); sum=a+b; printf(“Addition of a and b is %d”,sum); return 0; } CONSTANTS IN C 1. Symbolic Constant 2. Constants with const keyword SYMBOLIC CONSTANT Constants variable values cannot be modified by the program once they are defined. and Constants may be belonging to any of the datatype. Constants refer to fixed values. They are also called literals. Symbolic constants may repeat several times in a program. The well-known constant pie is an example of symbolic constants. (Define in Definition Section) Syntax: #define Variable_name Constant_value Example: #define PIE 3.14 EXAMPLE OF SYMBOLIC CONSTANT #include #define PI 3.14 int main() { float r=7.5; float area=PI*r*r; printf(“Area of circle is: %f”,area); return 0; } CONST KEYWORD C provides another way to define the constants with keyword const. Syntax: const datatype ConstantVariableName = ConstantValue; Where, const is a keyword / reserved word. datatype is the type of constant variable. ConstantVariableName is a name of variable. ConstantValue denotes value of the variable. Example: const int max=5; const float pie=3.14; ENUM IN C Enumeration (or enum) is a user defined data type in C. It is mainly used to assign names to integral constants, the names make a program easy to read and maintain. The keyword ‘enum’ is used to declare new enumeration types in C and C++. SYNTAX: enum EnumName {constant1, constant2, constant3,....... }; Example: enum color{red,gren,blue,green}; enum week{mon,tue,wed,thu,fri,sat,sun}; enum month{jan,feb,mar,apr,...dec}; EXAMPLE OF ENUM #include enum week{Mon, Tue, Wed, Thur, Fri, Sat, Sun}; int main() { enum week day; day = Wed; printf("%d",day); return 0; } Mon Tue Wed Thur Fri Sat Sun 0 1 2 3 4 5 6 EXAMPLE OF ENUM #include enum year{Jan, Feb, Mar, Apr, May, Jun, Jul, Aug, Sep, Oct, Nov, Dec}; int main() { int i; for (i=Jan; i