Introduction To Object Oriented Programming PDF
Document Details
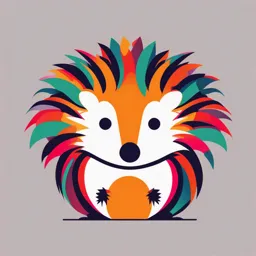
Uploaded by SimplestIridium168
Tags
Related
- OOP - JAVA Object-Oriented Programming PDF
- Topic 1.6 Running Program with Objects PDF
- CS0070 Object-Oriented Programming Module 1 PDF
- Object Oriented Programming (2nd Year 1st Sem) PDF
- Introduction to Java Programming and Data Structures (2019) by Y. Daniel Liang - PDF
- VILLASAN Object-Oriented Programming PDF
Summary
This document provides an introduction to object-oriented programming (OOP), specifically focusing on concepts within the Java programming language. It covers topics like classes, objects, methods, and instance variables. The document includes lecture notes and slide content. The document is likely supplementary material for an introductory computer science course.
Full Transcript
Classes and Objects Java™ How to Program, 10/e Late Objects Version © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 1 References & Reading The content is mainly selected (sometimes modified) from the...
Classes and Objects Java™ How to Program, 10/e Late Objects Version © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 1 References & Reading The content is mainly selected (sometimes modified) from the original slides provided by the authors of the textbook Readings ◦ Chapter 7: Introduction to Classes and Objects ◦ Chapter 8: Classes and Objects: A Deeper Look © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 2 Outline 7.1 Introduction to Object Oriented Technology 7.2 Primitive Types vs. Reference Types 7.3 Instance Variables, set Methods and get Methods 8.3 Controlling Access to Members 7.3 Default and Explicit Initialization for Instance Variables 7.4 Account Class: Initializing Objects with Constructors 8.6 Default and No-Argument Constructors 8.5 Time Class Case Study: Overloaded Constructors © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 3 1.5 Introduction to Object Technology Objects, or more precisely, the classes objects come from, are essentially reusable software components. ◦ There are date objects, time objects, audio objects, video objects, automobile objects, people objects, etc. ◦ Almost any noun can be reasonably represented as a software object in terms of attributes (e.g., name, color and size) and behaviors (e.g., calculating, moving and communicating). Software development groups can use a modular, object-oriented design-and- implementation approach to be much more productive than with earlier popular techniques like “structured programming”—object-oriented programs are often easier to understand, correct and modify. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 4 Methods and Classes In Java, we create a program unit called a class to house the set of methods that perform the class’s tasks. Performing a task in a program requires a method. The method houses the program statements that actually perform its tasks and hides these statements from its user Example of class: Account Example of methods: withdraw() and deposit() © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 5 Instantiation Just as someone has to build a car from its engineering drawings before you can actually drive a car, you must build an object of a class before a program can perform the tasks that the class’s methods define. An object is then referred to as an instance of its class. Example of instantiating an object: Account account1 = new Account(); account1 is an instance or object of the Account class. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 6 Reusability Just as a car’s engineering drawings can be reused many times to build many cars, you can reuse a class many times to build many objects. Reuse of existing classes when building new classes and programs saves time and effort. Reuse also helps you build more reliable and effective systems, because existing classes and components often have undergone extensive testing, debugging and performance tuning. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 7 Attributes and Instance Variables A car has attributes like : Color, its number of doors, the amount of gas in its tank, its current speed and its record of total miles driven (i.e., its odometer reading). The car’s attributes are represented as part of its design in its engineering diagrams. Every car maintains its own attributes. Each car knows how much gas is in its own gas tank, but not how much is in the tanks of other cars. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 8 Attributes and Instance Variables (Cont.) An object, has attributes that it carries along as it’s used in a program. An Account object has a balance attribute that represents the amount of money in the account. Each Account object knows the balance in the account it represents, but not the balances of the other accounts in the bank. Attributes are specified by the class’s instance variables. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 9 Encapsulation Classes (and their objects) encapsulate, i.e., encase, their attributes and methods. Objects may communicate with one another, but they’re normally not allowed to know how other objects are implemented—implementation details are hidden within the objects themselves. Information hiding, as we’ll see, is crucial to good software engineering. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 10 Inheritance A new class of objects can be created conveniently by inheritance—the new class (called the subclass) starts with the characteristics of an existing class (called the superclass), possibly customizing them and adding unique characteristics of its own. Example: an object of class “convertible” certainly is an object of the more general class “automobile”, but more specifically, the roof can be raised or lowered. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 11 Interfaces Interfaces are collections of related methods that typically enable you to tell objects what to do, but not how to do it This feature allows programmers to work similarly with different APIs since they implement the same interface(s). A class implements zero or more interfaces, each of which can have one or more methods, just as a car implements separate interfaces for basic driving functions, controlling the radio, controlling the heating and air conditioning systems, and the like. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 12 Object-Oriented Analysis and Design (OOAD) How will you create the code (i.e., the program instructions) for your programs? Follow a detailed analysis process for determining your project’s requirements (i.e., defining what the system is supposed to do) Develop a design that satisfies them (i.e., specifying how the system should do it). Carefully review the design (and have your design reviewed by other software professionals) before writing any code. Analyzing and designing your system from an object-oriented point of view is called an object-oriented-analysis-and-design (OOAD) process. Languages like Java are object oriented. Object-oriented programming (OOP) allows you to implement an object-oriented design as a working system. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 13 The UML (Unified Modeling Language) The Unified Modeling Language (UML) is the most widely used graphical scheme for modeling object-oriented systems. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 14 Primitive Types vs. Reference Types Java’s types are divided into primitive types and reference types. Primitive types: boolean, byte, char, short, int, long, float and double. Appendix D lists the eight primitive types in Java. All nonprimitive types (classes) are reference types. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 15 Primitive Types vs. Reference Types (cont.) © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 16 Primitive Types vs. Reference Types (cont.) A primitive-type variable can hold exactly one value of its declared type at a time. Programs use variables of reference types (normally called references) to store the addresses of objects in the computer’s memory. Such a variable is said to refer to an object in the program. To call an object’s methods, you need a reference to the object. If an object’s method requires additional data to perform its task, then you’d pass arguments in the method call. Primitive-type variables do not refer to objects, so such variables cannot be used to invoke methods. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 17 Instance Variables, set Methods and get Methods Each class you create becomes a new type that can be used to declare variables and create objects. You can declare new classes as needed. Declaring instance variables private is known as data hiding or information hiding. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 18 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 19 Class Declaration Each class declaration that begins with the access modifier public must be stored in a file that has the same name as the class and ends with the.java filename extension. Every class declaration starts with the keyword class followed by the class name. Class, method and variable names are identifiers. By convention all use camel case names. Class names begin with an uppercase letter, and method and variable names begin with a lowercase letter. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 20 Instance Variable name An object has attributes that are implemented as instance variables and carried with it throughout its lifetime. Instance variables exist before methods are called on an object, while the methods are executing and after the methods complete execution. A class normally contains one or more methods that manipulate the instance variables that belong to particular objects of the class. Instance variables are declared inside a class declaration but outside the bodies of the class’s method declarations. Each object (instance) of the class has its own copy of each of the class’s instance variables. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 21 Time1 class declaration maintains the time in 24-hour format // Fig. 8.1: Time1 class declaration maintains the time in 24-hour format. package pkg8_02; public class Time1 { private int hour; // 0 - 23 private int minute; // 0 - 59 private int second; // 0 - 59 // set a time value using universal time; throw an exception if the hour, minute or second is invalid public void setTime(int hour, int minute, int second) { // validate hour, minute and second if (hour < 0 || hour >= 24 || minute < 0 || minute >= 60 || second < 0 || second >= 60) { throw new IllegalArgumentException( "hour, minute and/or second was out of range"); } this.hour = hour; this.minute = minute; this.second = second; } // end method setTime public String toUniversalString() // convert to String in universal-time format (HH:MM:SS) { return String.format("%02d:%02d:%02d", hour, minute, second); } // end method toUniversalString public String toString() // convert to String in standard-time format (H:MM:SS AM or PM) { return String.format("%d:%02d:%02d %s", ((hour == 0 || hour == 12) ? 12: hour % 12), minute, second, (hour < 12 ? "AM" : "PM")); } } // end class Time1 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 22 Controlling Access: public and private Access Modifiers Most instance-variable declarations are preceded with the keyword private, which is an access modifier. Variables or methods declared with access modifier private are accessible only to methods of the class in which they are declared. Access modifiers public and private control access to a class’s variables and methods. Chapter 9 introduces access modifier protected. public methods present to the class’s clients a view of the services the class provides (the class’s public interface). Clients are not concerned with how the class accomplishes its tasks. Accordingly, the class’s private variables and private methods (i.e., its implementation details) are not accessible to its clients. Note: private class members are not accessible outside the class. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 23 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 24 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 25 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 26 Package Access If no access modifier is specified for a method or variable when it’s declared in a class, the method or variable is considered to have package access. In a program that uses multiple classes from the same package, these classes can access each other’s package-access members directly through references to objects of the appropriate classes, or in the case of static members through the class name. Package access is rarely used. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 27 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 28 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 29 7.2.1 Account Class with an Instance Variable, a set Method and a get Method (Cont.) Instance Method setName of Class Account In the preceding chapters (in CS140), you’ve declared only static methods in each class. A class’s non-static methods are known as instance methods. Method setName’s declaration indicates that setName receives parameter name of type String—which represents the name that will be passed to the method as an argument. If a method contains a local variable with the same name as an instance variable, that method’s body will refer to the local variable rather than the instance variable. In this case, the local variable is said to shadow the instance variable in the method’s body. The method’s body can use the keyword this to refer to the shadowed instance variable explicitly, as shown on the left side of the assignment in line 12. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 30 Account Class with an Instance Variable, a set Method and a get Method (Cont.) Recall that variables declared in the body of a particular method are local variables and can be used only in that method and that a method’s parameters also are local variables of the method. The Method setName’s body contains a single statement that assigns the value of the name parameter (a String) to the class’s name instance variable, thus storing the account name in the object. The Method getName returns a particular Account object’s name to the caller. The method has an empty parameter list, so it does not require additional information to perform its task. Driver Class AccountTest A class that creates an object of another class, then calls the object’s methods, is a driver class. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 31 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 32 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 33 Instantiating an Object—Keyword new and Constructors A class instance creation expression begins with keyword new and creates a new object. A constructor is similar to a method but is called implicitly by the new operator to initialize an object’s instance variables at the time the object is created. Calling Class Account’s getName & setName Methods To call a method of an object, follow the object name with a dot separator, the method name and a set of parentheses containing the method’s arguments. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 34 Compiling and Executing an App with Multiple Classes The javac command can compile multiple classes at once. Simply list the source-code filenames after the command with each filename separated by a space from the next. If the directory containing the app includes only one app’s files, you can compile all of its classes with the command javac *.java. The asterisk (*) in *.java indicates that all files in the current directory ending with the filename extension “.java” should be compiled. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 35 Account UML Class Diagram with an Instance Variable and set and get Methods We’ll often use UML class diagrams to summarize a class’s attributes and operations. UML diagrams help systems designers specify a system in a concise, graphical, programming-language-independent manner, before programmers implement the system in a specific programming language. Figure 7.3 presents a UML class diagram for class Account of Fig. 7.1. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 36 Account UML Class Diagram with an Instance Variable and set & get Methods (Cont.) Top Compartment In the UML, each class is modeled in a class diagram as a rectangle with three compartments. The top one contains the class’s name centered horizontally in boldface. Middle Compartment The middle compartment contains the class’s attributes (attribute name, followed by a colon and the type), which correspond to instance variables in Java. Private attributes are preceded by a minus sign (–) in the UML. Bottom Compartment The bottom compartment contains the class’s operations, which correspond to methods and constructors in Java. A plus sign (+) in front of the operation name indicates that the operation is a public one in the UML. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 37 Account UML Class Diagram with return types and parameters of Methods Return Types The UML indicates an operation’s return type by placing a colon and the return type after the parentheses following the operation name. UML class diagrams do not specify return types for operations that do not return values. Parameters The UML models a parameter of an operation by listing the parameter name, followed by a colon and the parameter type between the parentheses after the operation name © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 38 Additional Notes on Class AccountTest Notes on import Declarations Most classes you’ll use in Java programs must be imported explicitly. Classes that are compiled in the same directory are considered to be in the same package—known as the default package. Classes in the same package are implicitly imported into the source-code files of other classes in that package (no need to be explicitly imported). An import- declaration is not required if you always refer to a class with its fully qualified class name, which includes its package name and class name. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 39 Default and Explicit Initialization for Instance Variables Recall that local variables are not initialized by default. Primitive-type instance variables are initialized by default—instance variables of types byte, char, short, int, long, float and double are initialized to 0, and variables of type boolean are initialized to false. You can specify your own initial value for a primitive-type instance variable by assigning the variable a value in its declaration, as in private int numberOfStudents = 10; Reference-type instance variables (such as those of type String), if not explicitly initialized, are initialized by default to the value null—which represents a “reference to nothing.” © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 40 Account Class: Initializing Objects with Constructors Every class must have at least one constructor. If a class does not define constructors, the compiler creates a default constructor that takes no parameters Note: The default constructor initializes the instance variables to the initial values specified in their declarations or to their default values (zero for primitive numeric types, false for boolean values and null for references). Each declared class can optionally provide a constructor with (or without) parameters Constructors cannot return values. IF you declare a constructor for a class, THEN the compiler will not create a default constructor for that class. Notes: Local variables are not automatically initialized. Every instance variable has a default initial value—a value provided by Java when you do not specify the instance variable’s initial value. The default value for an instance variable of type String is null. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 41 Declaring an Account Constructor for Custom Object Initialization © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 42 Class AccountTest: Initializing Account Objects When They’re Created © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 43 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 44 A Constructor of Class Account’s UML Class Diagram The UML models constructors as operations To distinguish a constructor from a class’s operations, the UML places the word “constructor” between guillemets (« and ») before the constructor’s name. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 45 Default and No-Argument Constructors If your class declares constructors, the compiler will not create a default constructor. In this case, you must declare a no-argument constructor if default initialization is required. Like a default constructor, a no-argument constructor is invoked with empty parentheses. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 46 Time Class Case Study: Overloaded Constructors Class Time2 represents the time of day. private int instance variables hour, minute and second represent the time in universal-time format (24-hour clock format in which hours are in the range 0– 23, and minutes and seconds are each in the range 0–59). public methods setTime, toUniversalString and toString are called the public services or the public interface that the class provides to its clients. Overloaded constructors enable objects of a class to be initialized in different ways. To overload constructors, simply provide multiple constructor declarations with different signatures. Recall that the compiler differentiates signatures by the number of parameters, the types of the parameters and the order of the parameter types in each signature. Class Time2 (Fig. 8.5) contains five overloaded constructors that provide convenient ways to initialize objects. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 47 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 48 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 49 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 50 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 51 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 52 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 53 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 54 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 55 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 56 © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 57 Time Class Case Study: Overloaded Constructors (Cont.) A program can declare a so-called no-argument constructor that is invoked without arguments. Such a constructor simply initializes the object as specified in the constructor’s body. Using this in method-call syntax as the first statement in a constructor’s body invokes another constructor of the same class. Popular way to reuse initialization code provided by another of the class’s constructors rather than defining similar code in the no-argument constructor’s body. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 58 Notes Regarding Class Time2’s set and get Methods and Constructors Methods can access a class’s private data directly without calling the get methods. However, consider changing the representation of the time from three int values (requiring 12 bytes of memory) to a single int value representing the total number of seconds that have elapsed since midnight (requiring only four bytes of memory). ◦ If we made such a change, only the bodies of the methods that access the private data directly would need to change—in particular, the three-argument constructor, the setTime method and the individual set and get methods for the hour, minute and second. ◦ There would be no need to modify the bodies of methods toUniversalString or toString because they do not access the data directly. Designing the class in this manner reduces the likelihood of programming errors when altering the class’s implementation. © Copyright 1992-2015 by Pearson Education, Inc. All Rights Reserved. 59