C++ Mathematical Functions, Strings, and File I/O PDF
Document Details
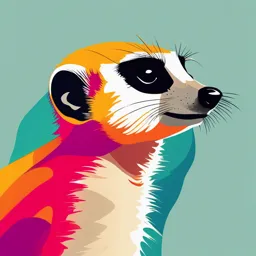
Uploaded by SupportingIvory
Douglas College
Tags
Related
Summary
This document is a set of C++ lecture notes on mathematical functions, characters, strings, and file input/output. It provides explanations and examples of various functions, including trigonometric, exponent, and string-related functions. The notes discuss reading data from and writing data to files. The notes may be for an undergraduate-level programming course and are likely from a university lecture or tutorial setting.
Full Transcript
Mathematical Functions, Characters, Strings, File I/O Chapter 4 Instructor: Link Course: CMPT 1109 2 Learning Outcomes ▸ Use Mathematical Functions ▸ Perform numeric operators on characters ▸ Convert int to chars and vis verse. ▸ Use numeric operators with chars. ▸...
Mathematical Functions, Characters, Strings, File I/O Chapter 4 Instructor: Link Course: CMPT 1109 2 Learning Outcomes ▸ Use Mathematical Functions ▸ Perform numeric operators on characters ▸ Convert int to chars and vis verse. ▸ Use numeric operators with chars. ▸ Generate a random number or a random character ▸ Apply character functions ▸ Program using string type ▸ Format the output ▸ Apply text file as input or output resource 3 Mathematical functions - Trigonometric ▸ Note: functions in gray color table are not required to remember, but we should know they exist and how to use them(search online when you need them) Function Description sin(radians) Returns the trigonometric sine of an angle in radians. cos(radians) Returns the trigonometric cosine of an angle in radians tan(radians) Returns the trigonometric tangent of an angle in radians. asin(a) Returns the angle in radians for the inverse of sine. acos(a) Returns the angle in radians for the inverse of cosine. atan(a) Returns the angle in radians for the inverse of tangent. 4 Trigonometric - Example Function Description sin(radians) Returns the trigonometric sine of an angle in radians. cos(radians) Returns the trigonometric cosine of an angle in radians tan(radians) Returns the trigonometric tangent of an angle in radians. asin(a) Returns the angle in radians for the inverse of sine. acos(a) Returns the angle in radians for the inverse of cosine. atan(a) Returns the angle in radians for the inverse of tangent. ▸ sin(PI / 6) returns 0.866 ▸ sin (270 * PI / 180) returns -1.0 ▸ atan(1.0) returns 0.785398 (same as PI/4) 5 Mathematical functions - Exponent ▸ Note: All functions from page 3 to 5 are from cmath header library. Function Description exp(x) Returns e raised to power of x (ex). log(x) Returns the natural logarithm of x (ln(x) = loge(x)). log10(x) Returns the base 10 logarithm of x (log10(x)). sqrt(x) Returns the square root of x( 𝑥) for x >=0. ceil(x) x is rounded up to its nearest integer. This integer is returned as a double value. floor(x) x is rounded down to its nearest integer. This integer is returned as a double value. pow(a, b) Returns a raised to the power of b (ab) 6 Mathematical functions – min, max ,abs Function Description min(x, y) Returns the minimum among x and y. (x and y should be same type) max(x, y) Returns the maximum among x and y. (x and y should be same type) abs(x) Returns the absolute value of x. ▸ max(2.5, 4.0) returns 4 ▸ max(2.5, 4) is compile-error ▸ abs(-2.1) returns 2.1 Note: all three are from library header cstdlib 7 Character Data Type and Operations Review: ▸ char type variable stores a single character. ▸ character literal is enclosed in single quotation marks: ▹ char digit9 = '9'; ▹ char letterZ = 'Z'; ▸ ASCII (American Standard Code for Information Interchange) is used by C++ to representing all available characters in 1 byte. 8 Escape sequence ▸ Some characters either cannot be typed on your keyboard or mean something special to C++ ▹ Either way, they cannot just be used in a print statement directly What happens to the statement... std:: cout > num1 >> num2 >> num3; input.close(); cout