SIN 3011 / SIM 3025 Scientific Computing PDF
Document Details
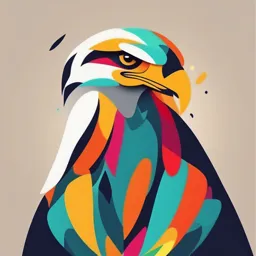
Uploaded by GraciousTellurium9246
Universiti Malaya
Dr. Noor Fadiya Mohd Noor
Tags
Related
- SIN 3011 / SIM 3025 Scientific Computing Chapter 1 PDF
- Anaconda, Pandas, and NumPy PDF
- NumPy Arrays & Vectors in Python (University of Petra) PDF
- Introduction to NumPy PDF
- Software Engineering II: Vorlesung Kapitel 3 (Wintersemester 2024/25) PDF
- MBB110 Data Analysis for Molecular Biology & Biochemistry Lecture 3 (Spring 2025) PDF
Summary
This document contains lecture material on arrays and strings. It explains concepts like data manipulation, array index out of bounds, searching, sorting, input/output, and more within the context of scientific computing.
Full Transcript
SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing SIN 3011 / SIM 3025 Chapter 3: Array a...
SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing SIN 3011 / SIM 3025 Chapter 3: Array and String Objectives In this chapter, you will learn/review: - Arrays and the data manipulation - “Array index out of bounds” - Restrictions on array processing - Searching and sorting in array - Parallel array - Multidimensional array - Inputting and outputting arrays - Array passing as a parameter to a function - C-string and string Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 2 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 1 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 1.0 Introduction Simple data type: Variables of these types can store only one value at a time. Structured data type: A data type in which each data item is a collection of other data items. Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 3 2.0 Array Array: A collection of a fixed number of components, all of the same data type. In one-dimensional array, components are arranged in a list form. Syntax for declaring a one-dimensional array: intExp: any constant expression that evaluates to a positive integer Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 4 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 2 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 2.1 Syntax General syntax: indexExp: called the index – An expression with a nonnegative integer value. Value of the index is the position of the item in the array. []: array subscripting operator – Array index always starts at 0 Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 5 2.2 Base Address of an Array Base address of an array: Address (memory location) of the first array component. Example: – If list is a one-dimensional array, its base address is the address of list. When an array is passed as a parameter, the base address of the actual array is passed to the formal parameter Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 6 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 3 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 2.3 Integral Data Type and Array Indices C++ allows any integral type to be used as an array index – Improves code readability Example: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 7 3.0 Array Processing Operations on an array: – Declaration – Initialization – Data input into an array – Data output from an array – Others such as finding the largest/smallest element etc. Each operation requires ability to step through elements of the array – Easily accomplished by a loop Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 8 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 4 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 4.0 Declaration and Initialization Given the declaration: int list; //array of size 100 int i; Use a for loop to access array elements: for (i = 0; i < 100; i++) //Line 1 cin >> list[i]; //Line 2 Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 9 4.1 Other Ways to Declare Arrays Examples: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 10 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 5 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 4.2 Array Initialization During Declaration Arrays can be initialized during declaration – Values are placed between curly braces – Size determined by the number of initial values in the braces Example: double sales[] = {12.25, 32.50, 16.90, 23, 45.68}; Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 11 4.3 Partial Initialization of Arrays During Declaration The statement: int list = {0}; – Declares an array of 10 components and initializes all of them to zero. The statement: int list = {8, 5, 12}; – Declares an array of 10 components and initializes list to 8, list to 5, list to 12 – All other components are initialized to 0. Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 12 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 6 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 5.0 Accessing Array Components Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 13 Accessing Array Components (cont’d.) Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 14 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 7 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 6.0 Array Index Out of Bounds Index of an array is in bounds if the index is >=0 and = 1) Declaration syntax: To access a component: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 22 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 11 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 11.1 Two-dimensional Array Two-dimensional array: collection of a fixed number of components (of the same type) arranged in two dimensions – Sometimes called matrices or tables Declaration syntax: This image cannot currently be display ed. – intExp1 and intExp2 are expressions with positive integer values specifying the number of rows and columns in the array Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 23 11.2 Declaring 2D Array Using Enum Enumeration types can be used for array indices: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 24 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 12 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 11.3 Declaring 2D Array Using Typedef Can use typedef to define a two-dimensional array data type. To declare an array of 20 rows and 10 columns: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 25 11.4 2D Array Initialization During Declaration Two-dimensional arrays can be initialized when they are declared: – Elements of each row are enclosed within braces and separated by commas – All rows are enclosed within braces – For number arrays, unspecified elements are set to 0. Example: double sales={12.25,32.50,16.90,45.68}; Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 26 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 13 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 11.5 2D Array Initialization Examples: – To initialize row number 4 (fifth row) to 0: – To initialize the entire matrix to 0: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 27 2D Array Initialization (cont’d.) Examples: – To input into row number 4 (fifth row): – To input data into each component of matrix: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 28 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 14 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 11.6 Processing 2D Arrays Ways to process a two-dimensional array: – Process entire array – Row processing: process a single row at a time – Column processing: process a single column at a time Each row and each column of a two-dimensional array is a one-dimensional array – To process, use algorithms similar to processing one- dimensional arrays Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 29 11.7 Accessing 2D Array Components Accessing components in a two-dimensional array: – Where indexExp1 and indexExp2 are expressions with positive integer values, and specify the row and column positions Example: sales = 25.75; Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 30 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 15 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing Accessing 2D Array Components (cont’d.) Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 31 12.0 Outputting A 2D Array Use a nested loop to output the components of a two dimensional array: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 32 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 16 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing Sum by Row Example: – To find the sum of row number 4: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 33 Sum by Column Example: – To find the sum of each individual column: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 34 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 17 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing Largest Element in Each Row and Each Column Example: – To find the largest element in each row: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 35 13.0 Array as Function Parameter Arrays are passed by reference only Do not use symbol & when declaring an array as a formal parameter. Size of the array is usually omitted – If provided, it is ignored by the compiler Example: Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 36 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 18 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 13.1 Passing 2D Array as Function Parameter Two-dimensional arrays are passed by reference as parameters to a function – Base address is passed to formal parameter Two-dimensional arrays are stored in row order. When declaring a two-dimensional array as a formal parameter, can omit size of first dimension, but not the second. Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 37 13.2 Constant Array as Formal Parameter Can prevent a function from changing the actual parameter when passed by reference – Use const in the declaration of the formal parameter Example: This image cannot currently be display ed. Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 38 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 19 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 14.0 C-Strings (Character Arrays) Character array: An array whose components are of type char. C-strings are null-terminated ('\0‘) character arrays. Example: – 'A' is the character A – "A" is the C-string A – "A" represents two characters, 'A' and '\0‘ Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 39 C-Strings (Character Arrays) (cont’d.) Example: char name; Since C-strings are null terminated and name has 16 components, the largest string it can store has 15 characters. If you store a string whose length is less than the array size, the last components are unused. Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 40 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 20 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing C-Strings (Character Arrays) (cont’d.) Size of an array can be omitted if the array is initialized during declaration. Example: char name[] = "John"; – Declares an array of length 5 and stores the C-string "John" in it Useful string manipulation functions – strcpy, strcmp, and strlen Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 41 14.1 String Comparison C-strings are compared character by character using the collating sequence of the system – Use the function strcmp If using the ASCII character set: – "Air" < "Boat" – "Air" < "An" – "Bill" < "Billy" – "Hello" < "hello" Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 42 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 21 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 14.2 Reading and Writing Strings Most rules for arrays also apply to C-strings (which are character arrays). Aggregate operations, such as assignment and comparison, are not allowed on arrays. C++ does allow aggregate operations for the input and output of C-strings Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 43 14.3 String Input Example: cin >> name; – Stores the next input C-string into name To read strings with blanks, use get function: cin.get(str, m+1); – Stores the next m characters into str but the newline character is not stored in str – If input string has fewer than m characters, reading stops at the newline character Edited by Associate Professor Dr. Noor Fadiya Mohd Noor, ISM 44 Edited by Assoc. Prof. Dr. Noor Fadiya Mohd Noor 22 SIN 3011 / SIM 3025: 10/17/2024 Scientific Computing 14.4 String Output Example: cout