Python Programming Lecture 2 PDF
Document Details
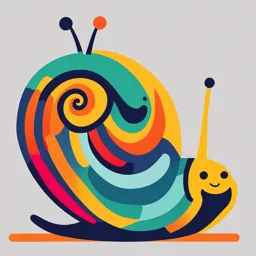
Uploaded by SpontaneousPersonification
Tags
Summary
This document is a lecture on Python programming, focusing on variables, data types, and casting. It provides definitions, examples, and outputs for various Python commands related to these topics.
Full Transcript
Programming by Python Lecture 2 1. Python variable creation and deletion 2.Data Types and Casting in python Quotations in python Python accepts single ('), double (") and triple (''' or """) quotes to denote string literals. Single and Double quotes ar...
Programming by Python Lecture 2 1. Python variable creation and deletion 2.Data Types and Casting in python Quotations in python Python accepts single ('), double (") and triple (''' or """) quotes to denote string literals. Single and Double quotes are primarily used for short strings. Single quote can contain double quotes inside without escaping, e.g., 'This is a "single-quoted" string.' Double quotes can contain single quotes inside without escaping, e.g., "This is a 'double-quoted' string." The triple quotes are used to span the string across multiple lines. Example: word = 'word' print (word) sentence = "This is a sentence." print (sentence) paragraph = """This is a paragraph. It is made up of multiple lines and sentences.""‚ print (paragraph) Creating Python variables A Python variable is created automatically when you assign a value to it. The equal sign (=) is used to assign values to variables. Variable declaration : Variable name = Value Multiple assignment is allowed in python Combined assignments is allowed in python by allocating comma separated variable names on left, and comma separated values on the right of = operator Example: x = 100 # Creates an integer variable Y = 1000.0 # Creates a floating point variable name = ‚Noha Sakr‚ # Creates a string variable a=b=c=10 # Multiple variable assignments a, b, c = 10,20,”Yusef” # combined assignments Printing Python variables Using print command Example: print (x) print (y) print (name) print (x, y, name) Deleting Python Variables The reference to a number object can be deleted by using the del statement. Example: x= 100 print (x) del x print (x) 100 Traceback (most recent call last): File "main.py", line 7, in print (x) NameError: name ‘x' is not defined Type of variable Data type of a Python variable can be retrieved using the python built-in function type() Example: x = "Zara" y = 10 z = 10.10 print(type(x)) print(type(y)) print(type(z)) Output Python Data types var1 = 1 # int data type var2 = True # bool data type var3 = 10.023 # float data type var4 = 10+3j # complex data type Sequence Data Types 1. String : No arithmetic operations, only slicing and concatenation Subsets of strings can be taken using the slice operator ([ ] and [:] ) with indexes starting from 0 in the beginning and -1 at the end. The plus (+) sign is the string concatenation operator. The asterisk (*) is the repetition operator in Python. str = 'Hello World!' print (str) # Prints complete string print (str) # Prints first character of the string print (str[2:5]) # Prints characters starting from 3rd to 5th print (str[2:]) # Prints string starting from 3rd character print (str * 2) # Prints string two times print (str + "TEST") # Prints concatenated string Hello World! H llo llo World! Hello World! Hello World! Hello World!TEST Sequence Data Types 2. List The most versatile compound data types. Contains items separated by commas and enclosed within square brackets ([]). Contains different data type X = [2024, ‚Yusef", 3.0, 5+6j, 1.23E-4] Type([2024, ‚Yusef", 3.0, 5+6j, 1.23E-4]) list = [ 'a', 786 , 2.23, ‘b', 70.2 ] tinylist = [1, ‘c'] print (list) # Prints complete list print (list) # Prints first element of the list print (list[1:3]) # Prints elements starting from 2nd till 3rd print (list[2:]) # Prints elements starting from 3rd element print (tinylist * 2) # Prints list two times print (list + tinylist) # Prints concatenated lists [ 'a', 786 , 2.23, ‘b', 70.2 ] a [786 , 2.23] [2.23, ‘b', 70.2 ] [1,’c’,1,’c'] ['a', 786 , 2.23, ‘b', 70.2,1,c ] Sequence Data Types 3. Tuple A tuple consists of a number of values separated by commas. Unlike lists, tuples are enclosed within parentheses (...) Each item in the tuple has an index referring to its position starting from 0. Tuples are immutable and can be seen of as read-only lists. tuple = ( 'a', 7 , 2.2, ‘b') list = [ 'a', 7 , 2.3, 'j'] tuple = 1000 # Invalid syntax with tuple list = 1000 # Valid syntax with list Dictionary Data types Consists of key:value pairs. kind of hash table type. Can be any Python type, but mostly numbers or strings. Separated by comma and put inside curly brackets {}. semicolon':' symbol is put between key and value to establish mapping between them Example: dict = {} dict['one'] = "This is noha" dict = "This is Yusef‚ dict2 = {'name': 'noha','mob':123, 'dept': 'computer science'} print (dict['one']) print (dict) print (dict2) print (dict2.keys()) print (dict2.values()) This is noha This is yusef {'name': 'noha', 'mob': 123, 'dept': 'computer science'} ['name', 'mob', 'dept'] ['noha', 123, 'computer science'] Set Data Type A set in Python is not indexed or ordered collection such as string, list or tuple. An object cannot appear more than once in a set. Comma separated items in a set are put inside curly brackets or braces {}. Example: student_id = {1, 2, 3, 4, 5} print('Student ID:', student_id) vowels = {'a', 'e', 'i', 'o', 'u'} print('Vowel Letters:', vowels) mixed_set = {'Hello', 1, -2, 'Bye'} print('Set of mixed data types:', mixed_set) Boolean Data type one of built-in data types which represents one of the two values either True or False. bool() function evaluates the value of any expression and returns True or False based on the expression. Example: a = True print(a) print(type(a)) True Boolean Data type Example: a = 2 b = 4 print(bool(a==b)) print(a==b) a = None print(bool(a)) a = () print(bool(a)) a = 0.0 print(bool(a)) a = 10 print(bool(a)) False False False False False True Data Types Casting There are two types of type conversion in Python. 1. Implicit Conversion : automatic type conversion 1.1 Implicit int to float : When any arithmetic operation on int and float operands is done. 1.2 Implicit bool to int and float Boolean object is first upgraded to int and then to float True is equal to 1, and False is equal to 0. a = 10 b = 10.2 C = a+b print (c) # output 20.2 a=True b=10.5 c= a+b print (c) # output 11.5 Data Types Casting 2. Explicit Conversion : manual type conversion by calling Python's built-in functions int(), float() and str() to perform the explicit conversions such as string to integer. 1. int () Example 1 : int (float) a = int(10.5) Print ( a) # Output 10 a = int(2*3.14) Print (a) # Output 6 print (type(a)) Example 2 : int (bool) a=int(True) Print( a) # Output 1 Print(type(a) ) # Output Data Types Casting Example 3 : int (numerical value) a = int("100") print (a) # Output 100 Print( type(a) ) # Output a = ("10"+"01") a = int("10"+"01") Print (a) # Output 1001 Example 4: int(float value or string) a = int(‚20.5") a = int("Hello World") # Output ValueError Data Types Casting Example 5 : binary => decimal a = int("110011", 2) Print (a) # Output 51 Example 6: octal => decimal a = int("20", 8) Print(a ) # Output 16 Example7: Hexadecimal => decimal a = int("2A9", 16) print (a ) # Output 681 Data Types Casting 2. Float a = float(100) Print (a) # Output 100.0 a = float("9.99") print (a) # Output 9.99 a = float("1,234.50") # ValueError 3. Str a = str(10) Print (a) # Output '10' a = str(11.10) print (a) # Output '11.1’' Sequence type casting A string and tuple can be converted into a list object by using the list() function c="Hello" Obj = list(c) Print (Obj ) # Output ['H', 'e', 'l', 'l', 'o'] Thank You..