Functions PDF
Document Details
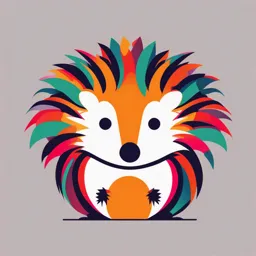
Uploaded by SurrealMarsh
Tags
Summary
This document explains functions in computer programming. It details the advantages of using functions, such as modularity, structured programming, debuggability, and reusability.
Full Transcript
FUNCTIONS INTRODUCTION The planning for large algorithms consists of first understanding the problem as a whole, second breaking it into simpler, understandable parts. We call each of these parts of a algorithm a module and the process of subdividing a...
FUNCTIONS INTRODUCTION The planning for large algorithms consists of first understanding the problem as a whole, second breaking it into simpler, understandable parts. We call each of these parts of a algorithm a module and the process of subdividing a problem into manageable parts top-down design. The principles of top-down design and structured algorithm dictate that a algorithm should be divided into a main module and its related modules. Each module is in turn divided into sub-modules until the resulting modules are intrinsic; that is, until they are implicitly understood without further division. Top-down design is usually done using a visual representation of the modules known as a structure chart. The structure chart shows the relation between each module and its sub-modules. The structure chart is read top-down, left-right. First we read Main Module. Main Module represents our entire set of code to solve the algorithm. INTRODUCTION Structure INTRODUCTION Moving down and left, we then read Module 1. On the same level with Module 1 are Module 2 and Module 3. The Main Module consists of three sub-modules. At this level, however we are dealing only with Module 1. Module 1 is further subdivided into three modules, Module 1a, Module 1b, and Module 1c. To write the algorithm for Module 1, we need to write for its three sub-modules. The Main Module is known as a calling module because it has sub-modules. Each of the sub-modules is known as a called module. But because Modules 1, 2, and 3 also have sub-modules, they are also calling modules; they are both called and calling modules. Communication between modules in a structure chart is allowed only through a calling module. INTRODUCTION If Module 1 needs to send data to Module 2, the data must be passed through the calling module, Main Module. No communication can take place directly between modules that do not have a calling- called relationship. How can Module 1a send data to Module 3b? Module 1a first sends data to Module 1, which in turn sends it to the Main Module, which passes it to Module 3, and then on to Module 3b. The technique used to pass data to a function is known as parameter passing. The parameters are contained in a list that is a definition of the data passed to the function by the caller. The list serves as a formal declaration of the data types and names. FUNCTIONS A function is a self contained program segment that carries out some specific well defined tasks. Advantages of functions Write the algorithm as collections of small functions to make the program modular Structured programming Algorithm easier to debug Easier modification Reusable in other algorithms FUNCTIONS Function Definition func_name(parameter list) { declarations statements } Function Header FUNCTIONS Function Prototypes If a function is not defined before it is used, it must be declared by specifying the return type and the types of the parameters. If all functions are defined before they are used, no prototypes are needed. In this case, main() is the last function of the algorithm. Scope Rules for Functions Variables defined within a function (including main) are local to this function and no other function has direct access to them. The only way to pass variables to function is as parameters. The only way to pass (a single) variable back to the calling function is via the return statement. FUNCTIONS PROTOTYPES Function Calls When a function is called, expressions in the parameter list are evaluated (in no particular order!) and results are transformed to the required type. Parameters are copied to local variables for the function and function body is executed when return is encountered, the function is terminated and the result (specified in the return statement) is passed to the calling function (for example main). Types of Functions User Defined Functions Standard Library Functions and Header Files USER DEFINED FUNCTIONS Every algorithm must have a main function to indicate where the algorithm has to begin its execution. While it is possible to code any algorithm utilizing only main function, it leads to a number of problems. The algorithm may become too large and complex and as a result task of debugging, testing and maintaining becomes difficult. If an algorithm is divided into functional parts, then each part may be independently coded and later combined into a single unit, these subprograms called “functions” are much easier to understand debug and test. There are times when some types of operation for calculation is repeated many times at many points through out a program. For instance, we might use the factorial of a number at several points in the program. In such situations, we may repeat the statements whenever they are needed. Another approach is to design a function that can be called and used whenever required. USER DEFINED FUNCTIONS A function is a self-contained block of code that performs a particular task. Once a function has been designed and packed it can be treated as a “black box” that takes some data from main algorithm and returns a value. The inner details of the algorithm are invisible to the rest of program. function-name ( argument list ) argument declaration; { local variable declarations; executable statement 1; executable statement 2; ---------- ---------- ---------- return (expression) ; } USER DEFINED FUNCTIONS The return statement is the mechanism for returning a value to the calling function. All functions by default returns int type data. We can force a function to return a particular type of data by using a type specifier in the header. A function can be called by simply using the function name in the statement. CATEGORIES OF FUNCTIONS A function depending on whether arguments are present or not and whether a value is returned or not may belong to Functions with No Arguments and No Return Value Functions with Arguments and No Return Value Functions with No Arguments but a Return Value Functions with Arguments and a Return Value CATEGORIES OF FUNCTIONS Functions with No Arguments and No Return Value When a function has no arguments, it does not receive any data from calling function. In effect, there is no data transfer between calling function and called function. CATEGORIES OF FUNCTIONS Functions with Arguments and No Return Value The function takes argument but does not return value. The actual (sent through main) and formal(declared in header section) should match in number, type and order. In case actual arguments are more than formal arguments, extra actual arguments are discarded. On other hand unmatched formal arguments are initialized to some garbage values. CATEGORIES OF FUNCTIONS Functions with No Arguments but a Return Value These functions do not take any arguments but return a value. They may rely on other factors within the function to produce a result, which is then returned. CATEGORIES OF FUNCTIONS Functions with Arguments and a Return Value These functions take arguments and return a value. They use the input parameters to perform a calculation or process and return the result to the caller. PARAMETER PASSING TECHNIQUES Call by Value The process of passing the actual value of variables is known as Call by Value. PARAMETER PASSING TECHNIQUES Call by Reference The process of calling a function to pass the addresses of variables is known as Call by Reference. The function which is called by reference can change the value of the variable used in the call. THANK YOU