DS SEM-1, UNIT-1 PDF
Document Details
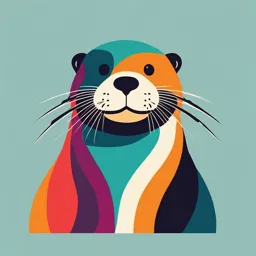
Uploaded by SofterSasquatch
Osmania University
Tags
Summary
This document is a unit on problem-solving and Python programming. It covers basic programming concepts, functions, data structures, and object-oriented programming. It provides examples and pseudo code, along with algorithm explanations. Suitable for introductory computer science courses.
Full Transcript
# Problem Solving and Python Programming ## Unit 01 ### Introduction to Computing and Problem Solving - Fundamentals of Computing - Computing devices - Identification of computational problems - Pseudo code and flowcharts - Instructions - Algorithms - Building blocks of al...
# Problem Solving and Python Programming ## Unit 01 ### Introduction to Computing and Problem Solving - Fundamentals of Computing - Computing devices - Identification of computational problems - Pseudo code and flowcharts - Instructions - Algorithms - Building blocks of algorithms ### Introduction to Python Programming - Python interpreter and Interactive Mode - Variables and identifiers - Arithmetic operators - Values and types - Statements - Reading input - Print output - Type conversions - The type() function and is operator - Dynamic and strongly typed language - Control flow statements - **The if** - **The if...else** - The if--- elif else decision control statements - Nested if statement - The while loop - The for loop - The continue and break statement ## Unit 02 ### Functions - Built-in functions - Commonly used modules. - Function definition and calling the function - The return statement and void function - Scope and lifetime of variables... - Default paramenters. - Keyword arguments. - \*args and \*\*kwargs. - Command line arguments. ### Strings - Creating and storing Strings - Basic string operations. - Accessing characters in string by Index Number - String slicing and joining. - For string methods. - Formatting strings. ## Unit 03 ### Lists - List operations - List slices. - List methods - List loop - Mutability - Aliasing - Cloning lists - List parameters - Tuples - Tuple assignment - Tuple as a return value... - Dictionaries: - Operations and methods - Advanced list processing - List comprehension - Illustrative programs - Selection sort, insertion sort, mergesort, histogram ### Files and exception -. Text files - Reading and writing files - Format operator. - Command line arguments -. Errors and exceptions - Handling exceptions. -. Modules - Packages - Illustrative programs - Word count, copy file. ## Unit 04 ### Object-Oriented Programming: - Classes and objects - Creating classes in Python - Creating objects in Python - The constructor method - Classes with multiple objects. - Class attributes versus data attributes - Encapsulation - Inheritance - the polymorphism ### Functional Programming: - Lambda - Iterators - Generators - List comprehensions. ## Unit 01 Continued ### Introduction to computing and problem solving - Fundamentals of computing - Computing: Computing refers to the use of computers and software to perform tasks, process information, and solve problems. - Computing devices: Computing devices are used to compute or calculate. For example: ATM machine, digital clock, digital washing machine. - Identification of computational components: Identifying computational components involves recognizing tasks or challenges that can be addressed or solved using computational components. - There are four types of computational components: 1) **Decision:** 2) **Search** 3) **Counting** 4) **Optimization** ### Decision problems - A decision problem is a type of computational problem that requires yes or no answers. It focuses on determining whether a given input satisfies a specific property or condition. ### Search problems - A search problem aims to find one or more solutions from a set of possible solutions. These problems often require exploring the space of potential solutions systematically to locate the one that satisfies certain conditions. ### Counting problems - A counting problem aims to determine the number of possible outcomes or solutions for a given scenario. These problems often require counting arrangements, combinations, or permutations based on certain criteria. ### Optimization problems: - An optimization problem seeks to find the best possible solution among a set of feasible solutions. The goal is usually to maximize or minimize an objective function, subject to constraints or conditions. ### Algorithms - An algorithm is a step-by-step procedure to solve a problem. - **Ex:** - **Step 1:** Start - **Step 2:** Read a, b. - **Step 3:** c = a + b. - **Step 4:** Print c. - **Step 5:** Stop. ### Flow chart - A flowchart is a graphical representation of an algorithm. --- ## Flow Chart Symbols | Symbol | Name | Purpose | | :-------- | :--------------- | :-------------------------------------------------------------------------------------------------------- | | ⚪ | Ellipse/oval | Start/stop | | ▭ | Parallelogram | Input/Output | | □ | Rectangle | Calculate/compute | | ♢ | Diamond | Decision | | → | Arrow | Flow | ## Example: Algorithm and flow chart for add, sub, multi, div. **Algorithm:** - **Step 1:** Start. - **Step 2:** Read a, b. - **Step 3:** c = a + b. - **Step 4:** d = a - b. - **Step 5:** e = a \* b. - **Step 6:** f = a/b. - **Step 7:** Print c. - **Step 8:** Print d. - **Step 9:** Print e. - **Step 10:** Print f. - **Step 11:** Stop. **Flow Chart:** ``` start ↓ read a, b ↓ c = a + b ↓ d = a - b ↓ e = a * b ↓ f = a / b ↓ print c ↓ print d ↓ print e ↓ print f ↓ stop ``` ## Pseudo Code - Pseudo code is simply an implementation of an algorithm in the form of annotations and informative text writing plan English. - It has no syntax like any of the programming languages and those can't be compiled (or) interpreted by the computer. - Pseudo code is independent of any programming language. - Each step must be understandable. - Each step set of instructions are written from top to bottom. ## Pseudo Code Keywords: | Keyword | Description | | :----------- | :------------------------------------------------------------------------------------------------------------- | | START | BEGIN | | INPUT | READ, OBTAIN, GET, INPUT | | COMPUTE | CALCULATE, ADD, SUBTR, INITIALIZE, COMPUTE | | INITIALIZE | SET, INITIALIZE | | ADD ONE | INCREMENT | | STOP | END | ## Advantages of Pseudo Code - It can be read and understood easily. - It can be done easily on a word processor. - It can be modified easily. - It occupies less space. - It will not run over many pages - Converting a pseudo code to a program is simple. ## Disadvantages of Pseudo Code - It is not visible. - We don't get a picture of the design. - There is no standardized style of form. - For beginners, it is more difficult to follow the logic (or) write pseudo code. ## Example: Pseudo Code to add two numbers. ``` BEGIN SET C = 0 READ A, B ADD C = A + B PRINT C END ``` ## Instructions - Instructions refer to the individual lines of code that make up a program. These lines of code are written in the python programming language, and are executed sequentially, one after the other, by the python interpreter. - Each instruction performs a specific operation or task. ## Instruction Types - Python: 1. **Variable Assignment:** Assigning a value to a variable using the assignment operator "=" - Ex: X = 5 2. **Print statement:** Outputting information to the console using the print statement. - Ex: print("Hello world!") 3. **Conditional statement:** Making decisions based on conditions using "if", "elif", and "else". - Ex: ```python if x > 0: print("positive") elif x == 0: print("zero") else: print("negative") ``` 4. **Loops:** Repeating a set of instruction using "for" and "while" loops . - Ex: ```python for i in range(5): print(i) ``` 5. **Function calls:** Invoking functions to perform specific tasks - Ex: result = add_numbers(3, 4) 6. **Exception Handling:** Dealing with errors and exceptional cases using "try", "except", "finally". - Ex: ```python try: result = x / y except ZeroDivisionError: print("Cannot divide by zero.") ``` ## Building Blocks of Algorithms in Python 1. **Variables and data types:** Variables are used to store and represent the data. Python supports various data types such as Integers, Float, Strings, list, and more. 2. **Operators:** Symbols used to perform operations on variables and values. 3. **Control structures:** These structures control the flow of execution in a program. - **Conditionals (if, elif, else):** Used for decision-making based on certain conditions. - **Loops (for, while):** Allow for iteration over a sequence of elements or repeated execution of a block of code. 4. **Functions:** Blocks of reusable code that perform a specific task. Functions take inputs (parameters), perform operations, and produce outputs. 5. **Data Structures:** - **Lists:** Collection of ordered data types - **Sets:** Collection of unordered data types - **Dictionaries:** Collection of key-value pairs 6. **Recursion:** A technique where a function calls itself, often employed in solving problems with repeated subtasks. 7. **Error Handling:** Using "try-except" blocks to handle exceptions and ensure robust code by gracefully managing unexpected situations. 8. **File Handling (I / O):** Reading from and writing to files for data I/P/O/P 9. **Libraries and modules:** Taking advantage of existing code through libraries for various functionalities, such as math operations, data manipulation and more. 10. **OOP (Object Oriented Programming):** Concepts like classes and objects for organizing code in a modular and reusable way. ## Chapter 02: Introduction to Python ### Features of Python: - Simple and easy to learn: Python is a very simple and easy language to learn, especially, for beginners. It has a few key words, a simple structure, and a clearly defined syntax. - Freeware and open source: Python is a copy-righted program that can be freely downloaded, installed, used, and shared. It is an open-source software, allowing people to customize the source code of Python in order to develop new flavors of Python. - Platform independent: Python is a platform-independent programming language that allows it to run on any platform, such as Windows, Linux, Mac OS, Solaris, and more. - Portability: It can run on a wide variety of hardware platforms with the same interface. - Dynamically typed language: Python allows variables to be used without explicit declaration of the data type. Python dynamically allocates the data type for a variable during execution of a statement. - Interpreted: Python allows you to run a program without any compilation. It is an interpreted language, allowing it to perform the operations in two modes: - **Interactive mode:** It is used to produce immediate results. - **Script mode:** It is used to run write and run the saved programs. - Both procedure and object-oriented: Python is both procedural and object-oriented. It allows you to write programs containing functions, classes (supporting all the object-oriented features), or a combination. - Extensible: It allows the user to add lower-level modules to the Python interpreter. It allows the use of other programming languages' existing code in Python. - Embedded: Python is compatible with other languages, allowing you to use Python programs in any other language. It can be easily integrated with C, C++, Java, LOM, etc. - Extensive library: Python contains a rich set of libraries to develop applications quickly. The library is portable and eases cross-platform compatibility in UNIX, Windows, and MAC OS systems. - GUI programming: Python supports GUI applications, which can be created and ported to many systems. It supports windows MFC, Macintosh, UNIX, etc.. The Microsoft Foundation Class library can be found online. ### Identifiers - Identifiers are the names that are used to identify various programming elements, such as variables, functions, classes, etc. ### Identifier Naming Rules - Identifiers can contain letters A to Z, a to z, digits 0 to 9, and the underscore (_). - The underscore is a special symbol. - Identifiers can begin with a letter or underscore, but not a digit. - **Ex:** abc, abc\_123, \-abc123 are all valid. 123abc is not valid. - Identifiers cannot have any blank spaces or special characters. - **Ex:** basic\_salary and amount are valid. basic salary is not valid. - Identifiers can differentiate between lower and upper case letters. - **Ex:** total, TOTAL are valid. - Keywords cannot be used as identifiers. - **Ex:** x, y, and z are valid but not if, else, while, try, etc. - An identifier can be of any length. - **Ex:** total, name, rollNumber, etc. ### Variables - A variable is a temporary storage location that holds a value. It can be changed during the execution of a program. ### Keywords - Keywords are the reserved words which have a specialized meaning explained to the compiler (or) interpreter. - Python supports 35 keywords. - **Ex**: if, else, elif, def, and, or, not, in, class, True, False, etc. ### Operators in Python - Operators are symbols used to perform mathematical (or) logical operations. - An **operand** is the value that acts upon the operators. ### Types of Operators in Python - Python supports the following types of operators. - **Arithmetic Operators:** Used for basic arithmetic operations. - Ex: +, -, \*, /, %, //, \*\* - **Comparison (Relation) Operators:** Used for comparing values. - Ex: ==, !=, >, <, >=, <= - **Logical Operators:** Used for combining logical expressions. - Ex: and, or, not - **Assignment Operators:** Used to assign values to variables. - Ex: =, +=, -= , \*=, /=, \%=, //=, \*\*= - **Bitwise Operators:** Used to perform bitwise operations. - Ex: &, |, ^, ~, <<, >> - **Identity Operators:** Used to check if two variables or objects refer to the same memory location. - Ex: is, is not - **Membership Operators:** Used to check if a specific value exists in a sequence. - Ex: in, not in ### Arithmetic Operators: - Arithmetic operators are used to perform various arithmetic operations. - **Symbol:** | **Operator Name** | **Description** | - :-------------------| :---------------------- | :------------------------------------------------------------------- | - + | Addition | Adds the values on either side of the operator. | - - | Subtraction | Subtracts the right side value from the left side value. | - \* | Multiplication | Multiplies the values on either side of the operator. | - / | Division | Divides the left side value by the right side value. | - % | Modulus | Returns the remainder after dividing the left side value by the right side value. | - \*\* | Exponent | Calculates the exponential power. | - // | Floor division | Returns the quotient of the division, ignoring the decimal part. | ### Relational Operators - Relational operators compare the values of two operands and return either true or false: - **Operator Name:** | **Symbol** | **Description** | - :---------------------- | :--------- | :--------------------------------------------------------------------------------------------------------------------- | - **Double Equal:** | == | Returns True if both operands are equal. Otherwise, returns False. | - **Not equal to:** | != | Returns True if both operands are not equal. Otherwise, returns False. | - **Greater than:** | > | Returns True if the left operand is greater than the right operand. Otherwise, returns False. | - **Less than:** | < | Returns True if the left operand is less than the right operand. Otherwise, returns False. | - **Greater than or equal to:** | >= | Returns True if the left operand is greater than or equal to the right operand. Otherwise, returns False. | - **Less than or equal to:** | <= | Returns True if the left operand is less than or equal to the right operand. Otherwise, returns False. | ### Logical Operators - Logical operators combine logical expressions and produce either True or False values. - **Symbol** | **Operator Name** | **Description** | - :----------| :---------------------- | :---------------------------------------------------------------------------------------------------- | - **And:** | **Logical and** | Returns True only if both operands are True. Otherwise, they return False. | - **Or:** | **Logical or** | Returns True if at least one of the operands is True. Otherwise, it returns False. | - **Not:** | **Logical not** | Returns True if the operand is False. Otherwise, it returns False. | ### Assignment Operators - Assignment operators store a value in a variable. - **Operator Name:** | **Symbol** | **Description** | - :---------------------- | :--------- | :---------------------------------------------------------------------------------------------------------------------------------------------------------------------- | - **Equal:** | = | Assigns the value of the right-side operand to the left-side operand. | - **Add AND:** | += | Adds the right side operand's value to the left side operand's value and assigns the result to the left side operand. | - **Subtract AND:** | -= | Subtracts the right side operand's value from the left side operand's value and assigns the result to the left side operand. | - **Multiply AND:** | \*= | Multiplies the right side operand's value with the left side operand's value and assigns the result to the left side operand. | - **Divison AND:** | /= | Divides the left side operand value by the right side operand value. and assigns the result to the left side operand. | - **Modulus AND:** | \%= | Returns (calculates) the remainder value after dividing the left side operand's value by the right side operand's value and assigns the result to the left side operand. | - **Exponent AND:**| \*\*= | Calculates the exponential power of the right side operand using the left side operand's value and assigns the result to the left side operand. | - **Floor Divison AND:** | //= | Returns the quotient value after dividing the left side operand's value by the right side operand's value, ignoring the decimal part. | ### Bitwise Operators - Bitwise operators manipulate with bits and perform bit by bit operations. - **Symbol:** | **Operator Name** | **Description** | - :----------| :---------------------- | :----------------------------------------------------------------------------------------------------------------------- | - **AND:** | **Bitwise AND** | If both corresponding bits are 1, it returns 1; otherwise, it returns 0. | - **OR:** | **Bitwise OR** | If either of the corresponding bits is 1, it returns 1; otherwise, it returns 0. | - **XOR:** | **Bitwise XOR** | If both corresponding bits are different (one is 0 and the other is 1), it returns 1; otherwise, it returns 0. | - **Left Shift Operator (<<):** Shifts the bits towards the left by a specified number of positions. - **Righ Shift Operator (>>):** Shifts the bits towards the right by a specified number of positions. - **One's complement (~):** Converts the bits - 0's as 1, 1's as 0's. ### Membership Operators - Membership operators check if a specific value exists in a sequence. - **Operator Name:** | **Symbol** | **Description** | - :---------------------- | :--------- | :------------------------------------------------------------------------------------------------------------------------- | - **In:** | **in** | It returns **True** if the value on the left side of the operator is found in the sequence on the right side. Otherwise, it returns **False.** | - **Not in:** | **not in** | It returns **True** if the value on the left side of the operator is not found in the sequence on the right side. Otherwise, it returns **False.** | ### Identity Operators - Identity operators check if two variables or objects refer to the same memory location - they have the same identity, rather than just the same value. - **Operator Name:** | **Symbol** | **Description** | - :---------------------- | :--------- | :-------------------------------------------------------------------------------------------------------------------- | - **Is:** | **is** | Returns True if both operands refer to the same object. Otherwise, returns False. | - **Is not:** | **is not** | Returns True if both operands refer to different objects. Otherwise, returns False. | ### Type Conversion: - Type conversion is the process of converting the value of one data type to another data type. - Python supports two types of type conversion: - **Implicit conversion:** Python automatically converts one data type to another data type without needing any user involvement. Implicit conversion is automatically performed by the Python interpreter. Python aims to avoid the loss of data during implicit conversion. - **Ex:** ```python x = 10 y = 20.5 z = x + y print(type(z)) ``` - In the above example, x is of type int, which is automatically converted to float by the Python interpreter. - **Explicit conversion:** Explicit conversion requires the user to explicitly convert the data type of an object to the required data type. This type conversion is also called type casting. It explicitly converts the data type of an object. This type conversion is sometimes called type casting or type coercion. - **Syntax:** *required data type* (*expression*) - **Ex:** - `int(10.5)` converts the float 10.5 to an integer 10 - `str(10.5)` converts the float 10.5 to a string '10.5' - **These are served built-in functions to perform conversions from one data type to another data type.** - **These functions return a new object representing the converted value.** ### Type Conversion Functions: - **int():** Used to convert float, numbers, strings, and booleans to integer type. - **Ex:** - `int(10.5)` converts the float 10.5 to an integer 10 - `int("10")` converts the numerical string '10' to an integer 10 - `int(True)` converts the boolean True to an integer 1 - `int("10.5")` results in ValueError - `int("hai")` results in ValueError - **float():** Used to convert int, float, string, and booleans to float type. - **Ex:** - `float(10)` converts the integer 10 to the float 10.0 - `float(True)` converts the boolean True to the float 1.0 - `float("10.5")` converts the string "10.5" to the float 10.5 - `float("hai")` results in ValueError - **bool():** Used to convert int, float, string, complex to bool type. - **Ex**: - `bool(10)` converts the integer 10 to a boolean True - `bool(0)` converts the integer 0 to a boolean False - `bool(10.5)` converts the float 10.5 to a boolean True - `bool("hai")` converts the string 'hai' to a boolean True. - **str():** Used to convert the given type to string type. - **Ex:** - `str(10)` converts the integer 10 into a string "10" - `str(10.5)` converts the float 10.5 to a string "10.5" - `str(True)` converts the boolean True to a string "True" - `str(10+2j)` converts the complex number 10+2j to a string "10+2j" - **list():** Used to convert a sequence type to a list. - **Ex:** - `list((10, 20, 30, 40))` converts the tuple (10, 20, 30, 40) to list [10, 20, 30, 40] - **tuple():** Used to convert a sequence type to a tuple. - **Ex:** - `tuple([10, 20, 30, 40])` converts the list [10, 20, 30, 40] to tuple (10, 20, 30, 40) - **set():** Used to convert a sequenced type to a set. - **Ex:** - `set([10, 20, 30, 40, 10])` converts the list [10, 20, 30, 40, 10] to a set {10, 20, 30, 40}. - **frozenset():** Used to convert a sequence type to a frozen set. - **Ex:** - `frozenset([10, 20, 30, 40])` converts the list [10, 20, 30, 40] to a frozenset {10, 20, 30, 40} ### The type() function - The type() function returns the type of the object in the argument. - **Ex:** - `a = ("BSC", "Honours")` - `b = ["BSC", "Honours"]` - `c = {"BSC": 1, "Honours": 2}` - `d = ("BSC Honours")` - `e =(10, 2, 3)` - `print(type(a))` outputs `<class 'tuple'>` - `print(type(b))` outputs `<class 'list'>` - `print(type(c))` outputs `<class 'dict'>` - `print(type(d))` outputs `<class 'str'>` - `print(type(e))` outputs `<class 'float'>` ### The "is" Operator - The "is" operator checks if two objects refer to the same memory location. This means they have the same identity, rather than just the same value. - It returns **True** if the two variables or objects have the same identity. Otherwise, it returns **False.** - **Ex:** - `x = 5` - `y = 5` - `print(x is y)` outputs **True** - `x = "hello"` - `y = "hello"` - `print(x is y)` outputs **True** - `x = [1, 2, 3]` - `y = [1, 2, 3]` - `print(x is y)` outputs **False** ### Dynamically Typed and Strongly Typed Languages - **Dynamically Typed:** A dynamically typed language doesn't know about the type of a variable until the code runs. The declaration of the variable has no use. The language stores the value at some memory location, binds the variable name to the memory container, and makes the contents of the container accessible through the variable. The data type doesn't matter, as the code will get to know the type of the value at runtime. -**Ex:** - `x = 6` - `print(type(x))` outputs `<class 'int'>` - `y = "hello"` - `print(type(y)) ` outputs `<class 'str'>` - **Strongly Typed:** If a language is strongly typed, it follows some important rules. When it is compiled or interpreted, it keeps track of all of the variables and constants to make sure that the data types are assigned correctly. In Python, variables are not explicitly declared with the type. However, the interpreter implicitly keeps track of all the types of the variables, hence the language is considered to be strongly typed. - **Ex:** - `var1 = 10` - `var2 = 20.20` - `var3 = 'variable'` - `print(type(var1))` outputs `<class 'int'>` - `print(type(var2))` outputs `<class 'float'>` - `print(type(var3))` outputs `<class 'str'>` ## Control Statements: - **Control Statements** are used to alter the flow of execution in a program. They provide a mechanism to control which parts of the code are executed and in what order. - **Conditional Statements** - **if statement:** The most simple decision making statement in Python. It is used to evaluate the given condition: If the condition is true, a block of statements within the if block will be executed. If the condition is false, the block of statements will be skipped. - **Syntax:** ```python if (condition): statement1 statement2 ... ``` - **Ex:** ```python age = 18 if (age >= 18): print("Eligible for voting") print("I am always there.") ``` - **if-else Statement:** This statement allows you to provide alternative code execution paths, depending on whether the condition is true or false. If the condition is true, the first block of code will execute; if the condition is false, the second block of code will execute. - **Syntax:** ```python if (condition): statement1 statement2 else: statement3 statement4 ``` - **Ex:** ```python age = 18 if (age >= 18): print("Eligible for voting") else: print("Not eligible for voting.") ``` - **if-elif Ladder:** This is a multi-way decision structure. The `if-elif` ladder evaluates conditions one by one. If the first condition is true, its corresponding block is executed, and the other conditions are skipped. If the first condition is false, the next `elif` condition is evaluated and so on. If none of the conditions in the ladder are true, the `else` block, if present, is executed. - **Syntax:** ```python if condition1: statement1 elif condition2: statement2 elif condition3: statement3 else: statement4 ``` - **Ex:** ```python avg = 85 if (avg >= 85): grade = 'A' elif (avg >= 75): grade = 'B' elif (avg >= 60): grade = 'C' elif (avg >= 50): grade = 'D' else: grade = 'E' print(grade) ``` - **Another Syntax:** - `[when true] if (condition) else [when false]` - **Ex:** - `a,b = 10,20` - `max = 0 if (a>b) else b` - **Nested if Statement:** A nested if statement contains an `if` statement within another `if` statement. It is used to evaluate complex conditions. - **Syntax** ```python if (condition1): if (condition2): statement1 else: statement2 else: statement3 ``` - **Ex:** ```python a, b, c = 10, 20, 30 if (a > b): if (a > c): print("a is greatest") else: print("c is greatest") else: if (b > c): print("b is greatest") else: print("c is greatest") ``` - **Looping Statements** - **while Loop:** The `while` loop continues to execute a block of code as long as the given condition is True. -**Syntax:** ```python while (condition): statement1 statement2 ... ``` - **Ex:** ```python i = 1 while (i <= 10): print(i) i += 1 ``` - The output will be the numbers from 1 to 10 - **for Loop:** The `for` loop in Python is used to iterate over a sequence (or) other iterable objects. - **Syntax:** ```python for val in sequence: statement1 statement2 ... ``` - **Here "val" is the variable that takes the value of the item inside the sequence on each iteration.** - **Ex:** ```python numbers = [10, 20, 30, 40, 50] for item in numbers: print(item) ``` - The output will be the values in the list - 10, 20, 30, 40, 50 - **Ex:** - `for i in range(10):` This will go through integers from 0 to 9. - `for i in range(10, 20):` This will go through integers from 10 to 19. - `for i in range(1, 10, 2):` This will go through integers from 1 to 10 with a step value of 2. - **Iterating over a sequence is known as traversal.** ## Transfer Statements - Transfer statements alter the flow of execution within a loop. - **Break, Continue, Pass** - **break Statement**: The `break` statement is used to terminate the current loop iteration immediately. This statement causes the loop to stop executing and jump out of the loop completely. The code after the loop continues execution normally. - **Ex:** ```python marks = [80, 60, 70, 150 ,85, 90] sum = 0 for val in marks: if (val