CSIT-112 Final Study Guide PDF
Document Details
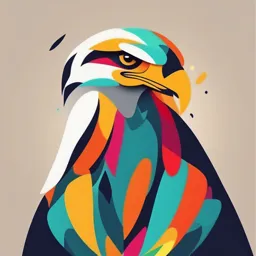
Uploaded by UnselfishNarrative
Kkottongnae University
Tags
Summary
This document is a study guide for a computer science course, focusing on topics such as object-oriented concepts, recursion, and exception handling. The guide includes questions related to each topic, which can potentially be used for a final exam. Includes important concepts like inheritance, polymorphism, and exception propagation.
Full Transcript
CSIT-112 Final Study Guide - Remember that this final will be fully comprehensive of the entire course. A bulk of the final will be based on the second half of the course. But please fill it out and familiarize yourself with all concepts...
CSIT-112 Final Study Guide - Remember that this final will be fully comprehensive of the entire course. A bulk of the final will be based on the second half of the course. But please fill it out and familiarize yourself with all concepts in this guide. Lecture 1: What is inheritance? A fundamental object-oriented design technique used to create and organize reuseable classes. The original class can be referred to in three different ways, what are they? Parent, Super, Base The derived class can be referred to in two different ways, what are they? Child, Subclass What type of relationship does proper inheritance create? Is-A Lecture 2 When we say inheritance is transitive, what does that mean? It is derived from the orgianl class meaning that the subclass can inherit properties of the top class. (If that makes sense) What is an abstract method? A method that is declared without use. Method header with no body/ What is an abstract class? It represents general concepts that derived classes have in common Lecture 4 What does polymorphism allow us to do? Allows us to define one interface to have multiple implementations. What can happen to a method if it is called through a polymorphic reference? It can change Lecture 5 What is sorting? The process of arranging a list of items in a particular order. What is the strategy we use for selection sort? a. Find the smallest value b. Switch it with the value in the first position c. Find the next smallest value in the list d. Switch it with the value in the second position e. Repeat untill al values are in their proper places. Lecture 6. What does the compareTo method do? Compare two inputs against each other then return a value a. What does it return if input1 < input2 - b. What does it return if input1==input2 0 c. What does it return if input1>input2 + What is the strategy for insertion sort? Lecture 7 What is a search pool? A group of items Lecture 8 What is an exception? Represents problems or unusual situations that may occur in a program (not a “error”) List scenarios that may cause an exception to be thrown Dividing by 0, Unable to read file, Array index that is out of bounds, math errors, ect. What is the call stack trace? Shows the line on which the exception occurred and the method call trail that leads to the attempt. What does each line of the call stack trace represent? Like what is the data it gives us? The Method, file, and line number. Explain the purpose of a try-catch statement? It allows a block of code to be tested for errors as its being executed at the same time as it defines a block of code if an error occurs in the try block. Lecture 9 What is exception propagation? An exception is first thrown from the top of the call stack and if its not caught, it drops down to the call stack to the previous method. What is the throwable class? What reserved word does it give us access to? throw Lecture 10 What are the three standard I/O streams? System.out/in/err What is system.in, system.out, and system.err? a. System Out: represents the console window b. System.in: represent the keyboard input, just like a scanner. c. System.err: represents the console window Lecture 11 In general, recursion is the process of what? The process of defining something in terms of itself We continue to break the problem down into smaller subproblems until we reach what? The base case What is a base case? The condition that terminates the recursive processing, allowing the active recursion methods to begin returning to their point of invocation. What are the four recursive problems we have gone over? Make sure you know how to write them out logically and produce the proper output Refer to my notes What happens if we do not have a base case? Infinite recursion, almost like a loop. When working with recursion, each call to the method creates a new environment in which to work, what does that mean? Explain It breaks down the problem smaller and smaller in its own fashion, later combining once getting its output. What is the difference between direct recursion and indirect recursion? Explain both Direct recursion invokes itself while, Indirect recursion reqires all the same care as direct recursion. Practice solving some recursive problems, here are two examples Lecture 12 What is a collection? An object that serves as a repo for other objects What are some collections you can think of that would add, remove, or manage elements? Add, remove, pop, push, etc. What is stable sorting? When numbers/elements with the same value appear in the output array in some order as they do in the input array. What is unstable sorting? The order of numbers/elements is not preserved during the sorting process and may appear in a different order in the output array. (Quick but not accurate) What is an abstract data type? What are the attributes of an abstract data type? A collection of data and the particular operations that are allowed on that data A static data structure has a fixed what? Fixed size Based on question 37, why can this be an issue? If we ever need to update the size of the array What is a dynamic data structure? Grows and shrinks when needed What do we use to link objects in dynamic data structures? References/ Pointer What is a reference (pointer)? Use to create links between objects What type of data structures can we make with references? List 3 Trees, Linked Lists, and Graphs Lecture 13 What is a linked list? A data structure in which objects are arranged in a linear order Nodes in a singly linked list are composed of what? What about a doubly linked list? Nodes are composed of data that hold the next pointer, head, tail. Each element of a list is an object with? An attribute key that stores the value a. A pointer next that points to its successor b. A point Prev that points to its predecessor Understand how the three linked list functions work What is the main benefit that we gain with linked lists compared to arrays? Lecture 14 What is a stack? A linear dynamic data structure that supports two operations (insertion and deletion) What is the policy of a stack? LIFO (Last in First Out) How are stacks represented? Draw one, which direction does it grow? (View Notes) What are the two functions to build/maintain a stack? Push and Pop What does top do? Top (Peek): Allows us to retrieve the top item without removing it What is a queue? A linear dynamic data structure in a form of a list that adds items only to the rear of the list and removes from the front/ What is the policy for a queue? FIFO (First in First Out) What are the insertion and deletion operations for a queue? Enqueue and Dequeue What does the head do? What does the tail do? a. Head: Keeps track of the index of the first element b. Tail: Keeps track of the index of the next location where it will be inserted Be able to explain Enqueue and Dequeue Lecture 15 What are two forms of non-linear data structures? Trees and Graphs What is a tree? Trees: U already know what a tree is What are all the attributes of a tree? Nodes, Edges, Children, Parent, Key, Root Please know how to fill in this tree with the appropriate attributes What is a binary tree? A tree in which each node can have no more than two child nodes What is a graph? A non-linear structure that doesn’t involve a root Typically what are the two types of graphs? Directed (Arrows) Undirected (Just a Line) Explain a undirected graph vs a directed graph Refer to Question 64. Know how to convert a graph to an adjacency matrix / or an adjacency matrix to a graph Lecture 16 What is a map? Explain why they are efficient. Are maps one way or two way? a. Map: A collection that establishes a relationship between keys and values Make an example of a map Student ID’s and Names What are two ways to implement a map? TreeMap and HashMap Explain a treemap, draw an example (View Notes) Explain a hashmap, draw an example (View Notes) What is a key and what is a value? Zip Code (Key): 07442 / Town (Value) Pompton Lakes What is the method to add a new entry to a map?.put What is the method to look up a value using a key?.get What can the var keyword be used for? To simplify complex variable declarations What is a lambda function? Why are they useful? It is a short block of code which takes in parameters then returns a value. They are useful when processing values stored in collections (Lists, Trees, Maps…) What is the form of a lambda expression? Parameters -> body What are the limitations of a lambda expression? a. They must return a value b. They cannot contain variables c. They cannot contain assignments d. They cannot contain statements such as IF or FOR What can a lambda expression be applied to? What is a stream? They can be applied to a stream. Stream: A sequence of elements on which you performtasks, where the elements are gotten from a collection.