Introduction to Computer Programming PDF
Document Details
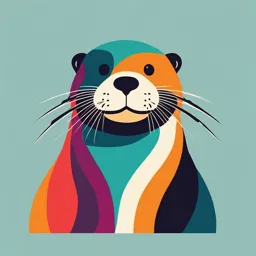
Uploaded by ClearFable
Tags
Summary
This document provides a fundamental introduction to computer programming concepts, including hardware, software, programming languages, and the steps involved in program development. It also details the purpose and components of computer algorithms and their visualization via flowcharting. Furthermore, a basic introduction to Python programming is provided.
Full Transcript
# Introduction to Computer Programming ## What is a Computer? * A tool to manipulate data to produce useful information * Has hardware and software. * Hardware: Tangible parts such as keyboard, mouse, etc. * Software: Non-tangible parts that are installed in a computer to command the hardwa...
# Introduction to Computer Programming ## What is a Computer? * A tool to manipulate data to produce useful information * Has hardware and software. * Hardware: Tangible parts such as keyboard, mouse, etc. * Software: Non-tangible parts that are installed in a computer to command the hardware to function and to help the users accomplish their task. * System software: Windows 10, Android OS, IOS * Utility software: Windows Defender, Norton Anti-Virus etc. * Application software: MS Word, Information System, POS etc. ## What is a Computer program? * A collection of instructions or statements that tell the computer what to do. * It is written or programmed using a programming language. * "Without computer programs, computers are useless" ## What is a Programming language? * Programs used by programmers to create programs or software. * Allows you to: * Design the Graphical User Interface (GUI) of the program * Add instructions (codes) * A programming language will translate the human-readable code (English) into a machine-readable language (binary) and compile it. ## What is Programming? * Designing or creating a set of instructions to ask the computer to carry out certain jobs. * The computer does what the program tells it to do. * The process of planning a sequence of steps for a computer to follow. * "A good programmer is a good planner" ## What is a program? * A set of steps that guides computer programmers through a program. * A systematic way of developing quality software ## The development of a program * **Analyze the problem:** * Identify the problem specifications and define each program input, output, and processing components. * **Design the program:** * Plan the solution to the problem: come up with logical sequence of precise steps that solve the problem using tools such as: * Algorithm * Pseudocode * Decision tables * Structures charts * Flowchart * **Code the program:** * Translate the algorithm into specific programming language. * Programmers must follow the language rules. * Violation of any rules causes errors, and these must be eliminated before going to the next step. * Coding is the technical word for writing the program. * **Test and debug the program:** * After the removal of syntax errors, the program will execute. * Testing is the process of finding errors in a program. * Debugging is the process of correcting those errors. ## 2 Types of Errors * **Syntax errors:** Occur when the code violated the syntax or grammar of the programming language. * **Logical errors:** A mistake that the programmer made while designing a solution to a problem. * Syntax and logical errors are collectively known as bugs. * **Document the Program** * Allows another person or the programmer to understand what the programs does and how to use the program at a later date. * Can include a detailed description of the program. * **Deploying and Maintaining:** * The program is deployed (installed) at the user's site and is kept under watch until the user gives their approval. * Even after the software is completed, it needs to be maintained and evaluated regularly. * The programming team fixes any errors in the software. ## Is Programming Hard? No. Unless you don't like it. ## Why do they say it's hard? * Programming needs: * The Right Mindset * The Right Determination ## Programming Algorithm * Set of instructions/rules to be followed by a computer program, which should contain an Input, Process, and an Output, depending on the problem to be solved. ## IPO Model * **Input:** Press call on mobile phone. * **Process:** Phone connects to network * **Output:** Phone connects with your friend. ## Variables * **Math:** A letter that represents any number. * **Programming:** A letter/word that serves as temporary storage. ## Programming Problem * Create a program that will display the sum of 2 numbers inputted by the user. ## Pseudocode: * Let numOne = 0, numTwo = 0, and sum = 0 * Input numOne and numTwo * sum = numOne + num Two * Output sum ## Programming Problem * Create a program that will display "Even or Odd" depending on the number inputted by the user. ## Pseudocode: * Let num = 0 * Input num * If num is an Even number, output "Even". * If num is an Odd number, output "Odd". ## Activity 1: Pseudocode ## Example * **Algorithm:** * Initialize the 3 variables, 2 for the addends and 1 for the sum. * Let the user input the addends. * Perform the addition between the addends and assign it to the sum. * Display the sum * **Pseudocode:** A method that allows a programmer to represent the algorithm in a more programming related way. It is also called false codes because it tend to look like a programming language but can still be understood by a person that has little understanding in programming. # Introduction to Python ## Python Language * Developed by Guido van Rossum * Easiest language to understand. * Indentation is important. * Very short syntax. * No semicolon needed. ## Python Language * C#: Console.Write("Hello World"); * JAVA: System.out.print("Hello World"); * PYTHON: print("Hello World") ## IDE * Integrated Development Environment * An application that is used for the convenience of the user's coding experience on a certain programming language, which provides tools to ease labor. ## print() * Used to display something in the console. * Syntax: print("Hello World") ## Creating a Variable * Creating a variable and assigning a value. * We can assign a value to a variable at the time it is created. * Use the assignment operator '=' to assign a value to a variable. * The operand that is on the left side of the assignment operator is a variable name. * The operand that on the right side of the assignment operator is the variable's value. * variable_name = variable_value * **Example** * name = "John" string assignment * age = 25 integer assignment * salary = 25800.60 float assignment * print(name) # John * print(age) #25 * print(salary) #25800.6 ## Changing the Value of a Variable * Many programming languages are statically typed. * The variable is declared with a specific type, and during its lifetime, it must always have that type. * In Python, variables are dynamically typed. * A variable can be assigned to a value of one type, and then later it can be assigned a different value of a different type. * **Example:** * var = 10 * print(var) # 10 * print(type(var)) # <class 'int'> * var = 55 * print(var) # 55 * var = "Now I'm a string" * print(var) # Now I'm a string" * print(type(var)) # <class 'str'> * var = 35.69 * print(var) # 35.69 * print(type(var)) # <class 'float'> ## Variables * Used to store data for later use. * **Common Data Types:** * String: Texts * Integer: Positive and Negative Numbers (No decimal) * Float: Decimal numbers * Character: Single letter or symbol * Boolean: True/False ## Identifiers * The name of the variable which the user decides to choose. * Syntax: (identifier = value) * firstName = "Computer" * lastName = "Programming" ## Declaring Variables * C++ Syntax * int main() * string name"Computer Programming"; * int age = 24; * float average = 96.5; * char letterA = 'A'; * bool isReal = false; * return 0; * Java Syntax * public static void main(string[] args) { * string name = "Computer Programming"; * int age = 24; * float average - 95.5; * char letterA = 'A'; * bool isReal = false; ## print() * Used to display something in the console. * Syntax: print(variable) ## input() * Used to make the user input something in the console. * Syntax: * variable = input() * variable = input("Enter Something") ## Casting Variables * A technique used to convert a data type to another data type. * Syntax: * Convert Numbers to String: str(number) * Convert String to Numbers: * int(string) * float(string) ## Arithmetic Operators * Used to perform mathematical operations inside of a program. | Operator | Syntax | Result | |----------|--------|--------| | Subtraction | x - y | Difference | | Addition | x + y | Sum | | Multiplication | x * y | Product | | Division | x / y | Quotient | | Modulus | x % y | Remainder | | Floor Division | x // y | Quotient (Rounded Off) | | Exponent| x ** y | Power | ## Example * Create a program that will display the sum of 2 numbers inputted by the user. ## Example 2 * Create a program that will compute for the student's general average for four major exams, prelim, midterm, semi, and final. Display the student's name and general average. * Create a program that will display the average of 4 subjects inputted by the user. * Create a program that will compute the product of 4 numbers inputted by the user. * Create a program that will compute the gross pay of an employee. Input the rate per hour, number of hours worked, name, and position of the employee. Display the name, position, and gross pay. * Create a program that will display the average of 3 subjects inputted by the user. * Create a program that will compute the product of 5 numbers inputted by the user. * Create a program that will compute for the salary of an employee. Input the rate per day, number of days worked, name, age, and position of the employee. Display the name, position, age, gender, and salary. ## Thank You! # Conditional Statements * A statement that makes the program smarter, it makes the program decide on what todo in a certain condition * **Conditional statements:** * IF statement (1 condition) * IF-Else statement (2 condition) * IF ELIF-ELSE (3 or more conditions) * Nested conditional statements (condition after a condition) ## Indentation * It is used to indicate what statements are included inside the conditional statement. ## Conditional Operators * Used to compare values inside a conditional statement. | Operator | Description | Usage | |----------|-------------|--------| | == | Equals To | 2 == 2 - TRUE | | != | Not Equal To | 2 != 2 - FALSE | | > | Greater Than | 5 > 3 - TRUE | | < | Less Than | 5 < 5 - FALSE | | >= | Greater Than OR Equal | 5 >= 5 - TRUE | | <= | Less Than OR Equal | 4 <= 5 - TRUE | ## IF Statement * Used when dealing with one condition. * Syntax: * if (condition): * #Do something * **Example:** * if age >= 18: * print("Legal Age") ## If-Else Statement * Used when dealing with two conditions * Syntax: * if (condition): * #Do Something * else: * #Do Something * **Example:** * if age >= 18: * print("Legal Age") * else: * print('Minor') ## If-Elif-Else Statement * Used when dealing with three or more conditions. * Syntax:: * if (condition): * #Do Something * elif (condition): * #Do Something * else: * #Do Something * **Example:** * if age >= 18: * print('Legal Age') * elif age >= 13: * print('Teenager') * else: * print('Too Young') ## Nested Conditional Statement * Used when dealing with conditions inside a condition. * Syntax: * if (condition): * if (condition): * #Do something * else: * #Do something * **Example:** * if age >= 18: * if height >= 170: * print('Legal Age and Tall') * elif height >= 156: * print('Legal Age and Average') * else: * print("Legal Age and Short") ## Logical Operators * Used to include 2 or more conditions in one line. * **Logical Operators:** * AND - Both conditions must be true * OR - Either condition must be true ## Sample Problems * Make a program that will determine whether the difference of two numbers is positive and negative. Display the difference and remarks. * Make a program that will determine and display the largest number among 3 numbers being inputted. * Create a program that makes the user input 3 grades, so that the system could average it then check if the grade is: | Grade | Remarks | |----------|-------------| | >100 or <=50 | Invalid Grade | | 98-100 | With Highest Honor | | 95-97 | With High Honors | | 90-94 | With Honors| | 75-89 | Passed | | 74-51 | Failed | *Note: Print the necessary message for each condition* ## Algorithm and Flowchart ## Programming Algorithm * Set of instructions/rules to be followed by a computer program. Which should contain an Input, Process, and an Output. Depending on the problem to be solved. ## Flowchart * A method that allows a programmer to represent the Algorithm in a diagram or an illustration. * It represents the sequence of a programming algorithm by using standard graphic symbols that will represent the Input, Process, and the Output. ## Advantages and Limitations of Flowcharts * Flowcharts are language independent. They can be learned and applied without formal knowledge of a programming language * Flowcharts encourage the programmer to think about the problem in a structured manner and can be used to easily communicate the logic of the program * Flowcharts can be a valuable tool for documenting code for other programmers, but they do not represent a programming language * They often require a long sequence of interconnected symbols * They do not convey why a given set of operations is made ## Types of Flowcharts * **Program flowchart:** Describes graphically, in detail, the logical operations and steps within a program and the sequence in which these steps are to be executed for the transformation of data to produce the needed output. * **System flowchart:** A graphic representation of the procedures involved in converting data on input media to data in output form. It illustrates which data is used or produced at various points in a sequence of operations. System flowcharts portray the interaction among data, hardware, and personnel. ## Basic Symbols These symbols are the ones often used to create a diagram that represents an algorithm that a computer program must follow: | Symbol | Description | |-------------|------------------| | ELLIPSE | START or END | | PARALLELOGRAM | INPUT or OUTPUT | | RECTANGLE | PROCESS | | DIAMOND | DECISION | | CIRCLE | On-page connector | | | Off-page connector | ## Input Output Symbol *Represents an instruction to input or output an operation.* ## Processing Symbol *Represents a group of program instructions that perform a processing function of the program, such as to perform arithmetic operations, or to compare, sort, etc.* ## Decision Symbol *Denotes a point in the program where more than one path can be taken.* ## Off-Page Connector *Used to connect flowchart portions on different pages.* ## Terminal Symbol *Used to designate the beginning and the end of a program, or a point of interruption.* ## On-Page Connector *Non-processing symbol which is used to connect one part of a flowchart to another without drawing flowlines.* ## Flowlines *Used to show reading order or sequence in which flowchart symbols are to be read.* ## Notations Used in Flowcharting | Notation | Meaning | Notation | Meaning | | -------- | -------- | -------- | -------- | | + | Addition | = or EQ | Equal to | | - | Subtraction | > or GT | Greater than| | * | Multiplication | < or LT | Less than | | / | Division | != or NE | Not equal to | | ** | Exponentation | >= or GE | Greater than or Equal to | | () | Grouping | <= or LE | Less than or Equal to | | || | Logical or | Y | Yes | | && | Logical and | N | No | | blank | End of File | ## Programming Problem * Create a program that will display the sum of 2 numbers inputted by the user. ## Pseudocode: * Let numOne = 0, numTwo = 0, and sum = 0 * Input numOne and numTwo * sum = numOne + numTwo * Output sum ## Programming Problem * Create a program that will display “Even or Odd”, depending on the number inputted by the user. ## Pseudocode: * Let num = 0 * Input num * If num is an Even number, output “Even” * If num is an Odd number, output “Odd” ## Programming Problem * Draw a flowchart that will input the name and value for the grade of a student. Determine whether the grade is passed or failed (passing grade is 75%). Print the name, grade, and remarks of the students. * Draw a flowchart that will compute for the general average of the four major exams, using these weights: prelim = 20%, midterm = 20%, semi = 20%, final = 40%. Display the name and general average of the student. ## Exercises: Flowchart * Draw a flowchart that will display the average of 3 numbers inputted by the user. * Draw a flowchart that will display the product of 3 numbers inputted by the user. * Draw a flowchart that will compute for the gross pay of an employee. Input the rate per hour, number of hours worked, name and position of the employee. Display the name, position and gross pay. ## Thank You!