Python Chapter Five: Function PDF
Document Details
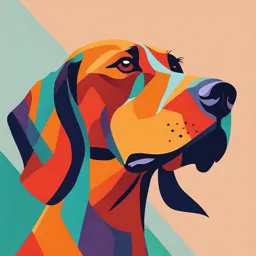
Uploaded by NobleKrypton
Jordan University of Science and Technology
Fatima M.AbuHjeela
Tags
Summary
This document is a chapter on functions in Python. It covers topics like introducing functions, their syntax, using local variables, passing arguments, global variables and constants and working with various python functions.
Full Transcript
Chapter Five: Function ❑ By: Fatima M.AbuHjeela Cyber Security Department 1 By : Fatima M. AbuHjeela Topics ❑Introduction to Functions ❑Defining and Calling a Void Function ❑Designing a Program to Use...
Chapter Five: Function ❑ By: Fatima M.AbuHjeela Cyber Security Department 1 By : Fatima M. AbuHjeela Topics ❑Introduction to Functions ❑Defining and Calling a Void Function ❑Designing a Program to Use Functions ❑Local Variables ❑Passing Arguments to Functions ❑Global Variables and Global Constants ❑Value-Returning Functions: Generating Random Numbers ❑Writing Your Own Value-Returning Functions ❑The math Module ❑Storing Functions in Modules 2 By: Fatima M.AbuHjeela Introduction to Functions ❑Function: group of statements within a program that perform as specific task ▪ Usually one task of a large program ▪ Functions can be executed in order to perform overall program task. ▪ Known as divide and conquer approach ❑Modularized program: ▪ program where in each task within the program is in its own function 3 By: Fatima M.AbuHjeela 4 By: Fatima M.AbuHjeela Benefits of Modularizing a Program with Functions ❑The benefits of using functions include: ▪ Simpler code ▪ Code reuse : write the code once and call it multiple times ▪ Better testing and debugging :Can test and debug each function individually ▪ Faster development ▪ Easier facilitation of teamwork: Different team members can write different functions 5 By: Fatima M.AbuHjeela Types of Functions in Python ❑Built-in Functions ❑ User-defined Functions ▪These are functions that Python ▪ These are functions created by provides by default, which are always ▪ users to perform specific tasks. available to use. Examples include: ▪ You define them using the def keyword. o print() o len() o type() o int(), str(), float(), etc. ❑ Lambda (Anonymous) Functions ❑ Higher-order Functions ▪ These are small, anonymous functions ▪ Functions that either take other created with the lambda keyword. functions as arguments, return a They can only contain a single function, or both. Examples include expression. map(), filter(), and reduce(). 6 By : Fatima M. AbuHjeela Defining and Calling a Function ❑Functions are given names ❑Function naming rules: ▪ Cannot use key words as a function name ▪ Cannot contain spaces ▪ First character must be a letter or underscore ▪ All other characters must be a letter, number or underscore ▪ Uppercase and lowercase characters are distinct 7 By: Fatima M.AbuHjeela Defining and Calling a Function ❑Function name should be descriptive of the task carried out by the function, often includes a verb ❑Function definition: specifies what function does 8 By: Fatima M.AbuHjeela Void Functions and Value-Returning Functions ❑ void function: Simply executes the statements it contains and then terminates. ❑ value-returning function: ▪ Executes the statements it contains, and then it returns a value back to the statement that called it. ▪ The input, int, and float functions are examples of value- returning functions. 9 By: Fatima M.AbuHjeela Defining and Calling a Function ❑Function header: first line of function Includes keyword def and function name, followed by parentheses and colon ❑Block: set of statements that belong together as a group ❑Call a function to execute it ▪ When a function is called: ◦ Interpreter jumps to the function and executes statements in the block ◦ Interpreter jumps back to part of program that called the function (Known as function return). ❑main function: called when the program starts ◦ Calls other functions when they are needed ◦ Defines the mainline logic of the program 10 By: Fatima M.AbuHjeela Indentation in Python ❑Each block must be indented ▪ Lines in block must begin with the same number of spaces ▪ Use tabs or spaces to indent lines in a block, but not both as this can confuse the Python interpreter ▪ IDLE automatically indents the lines in a block ▪ Blank lines that appear in a block are ignored 11 By: Fatima M.AbuHjeela Local Variables ❑Local variable: variable that is assigned a value inside a function ▪ Belongs to the function in which it was created ▪ Only statements inside that function can access it, error will occur if another function tries to access the variable ❑Scope: the part of a program in which a variable may be accessed ▪ For local variable: function in which created ❑Local variable cannot be accessed by statements inside its function which precede its creation ❑Different functions may have local variables with the same name ▪ Each function does not see the other function’s local variables, so no confusion 12 By: Fatima M.AbuHjeela Passing Arguments to Functions ❑Argument: piece of data that is sent into a function ▪ Function can use argument in calculations ▪ When calling the function, the argument is placed in parentheses following the function name 13 By: Fatima M.AbuHjeela ❑ Function arguments can be classified into several types based on how they are used and passed to functions Positional Arguments: These are the most common arguments, passed to a function in the same order as defined in the function signature. The number of arguments in the call should match the function definition Keyword Arguments Argument Types: These arguments are specified by the parameter name, allowing you to pass arguments in any order. They make code more readable and allow flexibility with argument order Default Arguments Default arguments allow parameters to have a default value if no argument is passed in the function call. They must be defined at the end of the parameter list in the function definition Variable-Length Arguments (*args) *args allows a function to accept an arbitrary number of positional arguments, which are collected into a tuple. Useful when you don’t know the number of arguments in advance. 14 By : Fatima M. AbuHjeela Passing Arguments to Functions ❑Positional Arguments: variable that is assigned the value of an argument when the function is called ▪ The parameter and the argument reference the same value ▪ General format: def function_name(parameter): ▪ Scope of a parameter: the function in which the parameter is used 15 By: Fatima M.AbuHjeela Passing Multiple Arguments ❑Python allows writing a function that accepts multiple arguments ▪ Parameter list replaces single parameter ▪ Parameter list items separated by comma ▪ Arguments are passed by position to corresponding parameters ▪ First parameter receives value of first argument, second parameter receives value of second argument, etc. 16 By: Fatima M.AbuHjeela Making Changes to Parameters ❑Changes made to a parameter value within the function do not affect the argument ▪ Known as pass by value ▪ Provides a way for unidirectional communication between one function and another function ▪ Calling function can communicate with called function 17 By: Fatima M.AbuHjeela Default Arguments ❑Default argument: argument that specifies which parameter the value should be passed to ▪ Position when calling function is irrelevant ▪ General Format: function_name(parameter=value) ▪ Positional arguments must appear first 18 By: Fatima M.AbuHjeela Keyword Arguments ❑These arguments are specified by the parameter name, allowing you to pass arguments in any order. ❑They make code more readable and allow flexibility with argument order ❑You can also mix positional and keyword arguments, but positional arguments must come first: 19 By : Fatima M. AbuHjeela Arbitary Arguments ❑Arbitrary Arguments, *args: ▪ *args allows a function to accept an arbitrary number of positional arguments, which are collected into a tuple. ▪ Useful when you don’t know the number of arguments in advance. 20 By: Fatima M.AbuHjeela Global Variables and Global Constants ❑Global variable: created by assignment statement written outside all the functions ▪ Can be accessed by any statement in the program file, including from within a function ▪ If a function needs to assign a value to the global variable, the global variable must be redeclared within the function ▪ General format: global variable_name ❑Reasons to avoid using global variables: ▪ Global variables making debugging difficult Many locations in the code could be causing a wrong variable value ▪ Functions that use global variables are usually dependent on those variables Makes function hard to transfer to another program ▪ Global variables make a program hard to understand 21 By: Fatima M.AbuHjeela Global Constants ❑Global constant: global name that references a value that cannot be changed ▪ Permissible to use global constants in a program ▪ To simulate global constant in Python, create global variable and do not re- declare it within functions 22 By: Fatima M.AbuHjeela Global Examples 23 By : Fatima M. AbuHjeela Standard Library Functions and the import Statement ❑Standard library: library of pre-written functions that comes with Python ▪ Library functions perform tasks that programmers commonly need ▪ Example: print, input, range ▪ Viewed by programmers as a “black box” ❑Some library functions built into Python interpreter ❑Modules: files that stores functions of the standard library ▪ Help organize library functions not built into the interpreter ▪ Copied to computer when you install Python ❑To call a function stored in a module, need to write an import statement ▪ Written at the top of the program ▪ Format: import module_name 24 By: Fatima M.AbuHjeela Generating Random Numbers ❑Random number are useful in a lot of programming tasks ❑random module: includes library functions for working with random numbers ❑Dot notation: notation for calling a function belonging to a module ❑Format: module_name.function_name() ❑Random number created by functions in random module are actually pseudo-random numbers ❑Seed value: initializes the formula that generates random numbers ▪ Need to use different seeds in order to get different series of random numbers ▪ By default uses system time for seed ▪ Can use random.seed() function to specify desired seed value 25 By: Fatima M.AbuHjeela Random Module Functions 26 By : Fatima M. AbuHjeela Generating Random Numbers 27 By: Fatima M.AbuHjeela Random Example 28 By : Fatima M. AbuHjeela The math Module ❑math module: part of standard library that contains functions that are useful for performing mathematical calculations ▪ Typically accept one or more values as arguments, perform mathematical operation, and return the result ▪ Use of module requires an import math statement ❑The math module defines variables pi and e, which are assigned the mathematical values for pi and e ❑Can be used in equations that require these values, to get more accurate results ❑Variables must also be called using the dot notation circle_area = math.pi * radius**2 29 By: Fatima M.AbuHjeela The math Module 30 By: Fatima M.AbuHjeela Returning Functions ❑To write a value-returning function, you write a simple function and add one or more return statements ▪ Format: return expression ▪ The value for expression will be returned to the part of the program that called the function ❑The expression in the return statement can be a complex expression, such as a sum of two variables or the result of another value- returning function ❑Use the returned value : Assign it to a variable or use as an argument in another function 31 By: Fatima M.AbuHjeela Returning Multiple Values ❑In Python, a function can return multiple values, Specified after the return statement separated by commas ▪ Format: return expression1,expression2, etc. ❑When you call such a function in an assignment statement, you need a separate variable on the left side of the = operator to receive each returned value 32 By: Fatima M.AbuHjeela Lambda Function ❑What is a Lambda Function? ▪ A lambda function is a small anonymous function in Python. ▪ It's defined using the lambda keyword, rather than the standard def keyword. ▪ Lambda functions can take any number of arguments but can only have one expression. ▪ The result of the expression is automatically returned. ❑Syntax of Lambda arguments: Input parameters, similar to regular function arguments. expression: The operation to be performed. It can be any valid Python expression. 33 By : Fatima M. AbuHjeela Lambda Function ❑Advantages of Lambda Functions ▪Concise: They allow you to write shorter, more readable code when the function is simple. ▪Anonymous: Lambda functions don't require a name (although they can be assigned to variables). ▪Functional Programming: Useful for functional programming techniques (e.g., with map(), filter(), reduce()). ❑Limitations of Lambda Functions ▪Single Expression: Lambda functions can only contain a single expression. No multiple statements or complex logic. ▪Readability: While lambda functions are concise, they can sometimes be less readable compared to standard function definitions. 34 By : Fatima M. AbuHjeela Lambda Example 35 By : Fatima M. AbuHjeela Nested Function ❑A nested function in Python refers to a function defined inside another function. ▪ The inner function (nested function) can access variables and parameters from the outer function, but the outer function cannot access the inner function directly unless it is returned or passed. 36 By : Fatima M. AbuHjeela Storing Functions in Modules In large, complex programs, it is important to keep code organized Modularization: grouping related functions in modules ◦ Makes program easier to understand, test, and maintain ◦ Make it easier to reuse code for multiple different programs ◦ Import the module containing the required function to each program that needs it 37 By: Fatima M.AbuHjeela Storing Functions in Modules ❑Module is a file that contains Python code ▪ Contains function definition but does not contain calls to the functions ▪ Importing programs will call the functions ❑Rules for module names: ▪ File name should end in.py ▪ Cannot be the same as a Python keyword ❑Import module using import statement 38 By: Fatima M.AbuHjeela Summary ▪ The advantages of using functions ▪ The syntax for defining and calling a function ▪ Methods for designing a program to use functions ▪ Use of local variables and their scope ▪ Syntax and limitations of passing arguments to functions ▪ Global variables, global constants, and their advantages and disadvantages ▪ Value-returning functions, including: oWriting value-returning functions oUsing value-returning functions oFunctions returning multiple values ▪ Using library functions and the import statement ▪ Modules, including: oThe random and math modules oGrouping your own functions in modules 39 By: Fatima M.AbuHjeela