Programming in Python for Business Analytics Lecture Notes PDF
Document Details
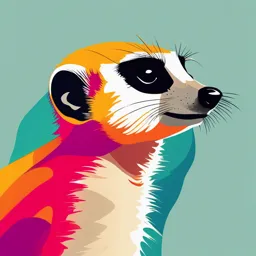
Uploaded by AdventurousJasper8246
The University of Manchester, Alliance Manchester Business School
Dr. Xian Yang
Tags
Related
- Introduction to Object-Oriented Programming (OOP) PDF
- Programming in Business Analytics Lecture 5 Notes PDF
- Programming for Business Analytics - Lecture 5 Notes PDF
- Starting Out with Python - Classes and Object-Oriented Programming PDF
- Network Programming Control Flow Functions OOP in Python PDF
- Digital Skills & Python Programming - Chapter 7 PDF
Summary
These lecture notes provide a basic introduction to programming in Python, focusing on Object-Oriented Programming (OOP) concepts and related ideas such as functions, modules, and exceptions for business analytics. They are suitable for undergraduate-level computer science students, or self-learners interested in acquiring foundational Python skills.
Full Transcript
Programming in for Business Analytics (BMAN73701) Lecture 4 – Object-Oriented Programming (OOP) Dr. Xian Yang [email protected] Functions Modules Exceptions 2 Functions Pre-defined...
Programming in for Business Analytics (BMAN73701) Lecture 4 – Object-Oriented Programming (OOP) Dr. Xian Yang [email protected] Functions Modules Exceptions 2 Functions Pre-defined functions vs user-defined functions def function_name(arguments): indented statement block Functions can have also zero arguments, e.g. or more than one argument, e.g. 3 Functions return statement allows your function to return a value (otherwise it returns the special value None) Once a value from a function is returned, the function stops being executed immediately You must define a function first before calling it Functions can have any number of arguments An argument can be of any type (even a function) 4 Local vs global variables Function arguments and variables created inside a function cannot be referenced outside of the function’s definition 5 Local vs global variables Python assumes variables are local, if not otherwise declared When you define variables inside a function definition, they are local to this function by default (even if the name is the same) Global variables are generally bad practice and should be avoided. In most cases where you are tempted to use a global variable, it is better to utilize a parameter for getting a value into a function or return a value to get it out. in function, var 6 in function, test 7 4 6 Recursion Example: Compute the sum of items in a list iteratively (i.e. using a loop) Example: Compute the sum of items in a list using a recursive function Recursion: An algorithmic technique where a function, in order to accomplish a task, calls itself with some part of the task. 7 Modules Pieces of code (.py files) that someone else has written to do a common task, e.g. mathematical operations Using modules: Add import module_name at the top of your code Use module_name.var to access functions and values with the name var in the module Option to import only certain functions/variables from a module and rename them 8 Exception handling To handle exceptions, use try/except statement try statement contains code that may throw an exception, except statement defines what to do if a particular exception is thrown except statement can have more than one except clause (at most one except clause will be executed) 9 Exception handling Use a finally statement to ensure some code runs regardless if an error occurs or not, and what type the error is 10 pass Statement (new material) The pass statement does nothing It is used when a statement is required syntactically but you do not want any command or code to execute Also useful in places where your code will eventually go, but has not been written yet 11 Object-oriented programming (OOP) Introduction to OOP The __init__ method and self keyword Different attribute and method types Important principles in OOP: Encapsulation, polymorphism, inheritance 12 Object-oriented programming (OOP) An object-oriented program (OOP) is based on classes and a collection of interacting objects, which can receive messages, process data, and send messages to other objects Nice introduction to OOP https://www.python-course.eu/object_oriented_programming.php 13 Object-oriented programming (OOP) Object in Python: Everything in Python (e.g. an integer, list, function, etc) is an object and can be assigned to a variable. Identity: object’s address in memory (check with id()) Type: determines operations that the object supports and also defines the possible values for objects of that type (e.g. int, list, etc) (check with type()) Value (compare with ==) 14 Functions as objects 15 Object-oriented programming (OOP) Class: A structure for defining a type of object. So, a dict is a class, a list is a class etc. They are useful for grouping together data, and for having variables behave a particular, repeatable way. A class is a mechanism Python gives us to create new user- defined types (i.e. attributes and methods) from Python code. Instance: An object which is created from a particular class. e.g., ls = [1,2,3] 16 Example: Class & Instance “A class definition can be compared to the recipe to bake a cake. The main difference between a recipe (class) and a cake (an instance) is obvious. A cake can be eaten when it is baked, but you can't eat a recipe. A class contains attributes and methods. If you bake a cake you need ingredients and instructions to bake the cake.” https://www.python- course.eu/object_orient ed_programming.php 17 Example code Working example: A class Car that defines a car via the attributes brand, color, age of a car, and number of seats. Each car should be able to make a sound (method). Instances of this class could be e.g. a 2-year old white Porsche and a 20- year old yellow Audi. 18 Classes are created using the keyword class Class attribute Constructor Instance attribute Instance method porsche and audi are two instances of the class Car. Working example: A class Car that defines a car via the attributes brand, color, age of a car, and number of seats. Each car should be able to make a sound (method). Instances of this class could be e.g. a 2-year old white Porsche and a 20-year old yellow Audi. 19 Object-oriented programming (OOP) Introduction to OOP The __init__ method and self keyword Different attribute and method types Important principles in OOP: Encapsulation, polymorphism, inheritance 20 __init__ and self The __init__ function is the constructor and is run when an instance of the class is created Like any function, the __init__ function takes arguments 21 The self keyword in Python is a reference to the current instance of the class. It is used to access attributes and other methods of the instance. 22 Object-oriented programming (OOP) Introduction to OOP The __init__ method and self keyword Different attribute and method types Important principles in OOP: Encapsulation, polymorphism, inheritance 23 Attributes We have some attributes that we might not know at the start But we write them here so we know all attributes an instance has We set them to None for now Instance attributes can be accessed via the instance only (instance.attribute_name). Class attributes can be accessed via the class (class.attribute_name) or the instance (instance.attribute_name). Two types of attributes Class attributes: Are shared by all instances of the class Instance attributes: Are specific to an instance of the class (and are of the form self.attribute_name) 24 If you modify the Class attribute through instance (e.g., porsche.num_tires = 3), this change only affects that instance. The attribute num_tires for the porsche object is now an instance variable, separate from the class attribute. If you modify the Class attribute through class (e.g., Car.num_tires =5), then this change affects the attribute at the class level. All instances that have not overridden this attribute (i.e., have not set their own instance-specific value for num_tires) will reflect this new value. 25 Quiz 1 (1) (2) (3) (4) What is the output of this code? a. b. c. d. (1) 4 4 4 (1) 4 4 4 (1) 4 4 4 (1) 4 4 4 (2) 4 3 4 (2) 3 3 4 (2) 4 3 4 (2) 3 3 4 (3) 6 3 4 (3) 6 3 6 (3) 6 3 6 (3) 6 3 4 (4) 6 (4) 6 (4) 6 (4) 6 26 Quiz 1 Ans: c. (1) (1) 4 4 4 (2) (2) 4 3 4 (3) (3) 6 3 6 (4) (4) 6 What is the output of this code? 27 Methods Three types of methods are available: Instance methods Class methods Static methods Instance methods are the most common type of method in a class. They are used to perform operations that involve the data of the instance of the class. These methods have access to the instance through self, which is always passed as the first argument in instance methods. These methods are accessed via instance.method_name() (e.g., porsche.roar()) We could instead call the instance method by using class.method(instance) (e.g., Car.roar(porsche)), but this is more writing so we use the other method. 28 Instance Methods 29 Class Methods Three types of methods are available: Instance methods Class methods Static methods Class methods are marked with a @classmethod decorator Instead of accepting a self parameter, class methods take a cls parameter that points to the class—and not the object instance— when the method is called. They can be called by both instance and class Because the class method only has access to this cls argument, it cannot modify object instance state. However, class methods can still modify class state that applies across all instances of the class 30 Class Methods Class method modifying a class state Class method used to create an object 31 Methods Three types of methods are available: Instance methods Class methods Static methods Static methods are with a @staticmethod decorator Can be called through both class and instance Takes neither a self nor a cls parameter (but of course it’s free to accept an arbitrary number of other parameters). Nothing more than a function that is defined inside a class that can neither modify object state nor class state Static methods are primarily a way to group functions which have some logical connection with a class 32 Methods 33 Quiz 2 Which of the function calls below will raise an error? 1) 2) 3) 4) 5) 6) 7) 8) 34 Quiz 2 Which of the function calls below will raise an error? 4) 35 Object-oriented programming (OOP) Introduction to OOP The __init__ method and self keyword Different attribute and method types Important principles in OOP: Encapsulation, polymorphism, inheritance 36 Encapsulation Encapsulation: Restriction of access to variables and methods (also called information hiding) Advantages: Prevent data from being modified by accident + Improve control of data flow in a program Python has three levels of restriction that control how data can be accessed and from where; variables and methods can be Public no underscore, e.g. a variable speed Private two underscores, e.g. a variable __speed Protected one underscore, e.g. a variable _speed 37 Public vs Private Three levels of restrictions are available for methods and variables: Public Private Protected Can be freely Private variables and modified and run methods can be from anywhere, accessed from within either inside or its own class outside of the class The value of a private Public variables variable cannot and methods don't be modified from use underscores, e.g. outside of a class a variable speed Private variables / methods begin with two underscores, e.g. __speed 38 Public vs Private 39 Public vs Private 40 Protected Three levels of restrictions are available for methods and variables: Public Private Protected Can be freely Private variables and Not often used modified and run methods can be Should only be from anywhere, accessed from within accessed by its own either inside or its own class class and any classes outside of the class The value of a private derived from it (like Public variables variable cannot private) and methods don't be modified from But can be accessed use underscores, e.g. outside of a class outside of the class a variable speed Private variables / (like public) methods begin with Protected variables two underscores, e.g. / methods begin with __speed one underscore, e.g. _speed 41 Public, Private, Protected 42 Get and set methods for public and private variables Data encapsulation via methods doesn't necessarily mean that the data is hidden. You might be capable of accessing and seeing the data anyway, but using the methods is recommended 43 Inheritance The transfer of the characteristics of a class to other classes that are derived from it. In other words, inheritance provides a way of sharing functionality between classes. The class containing the shared functionalities (here the class Animal) is called superclass A class that inherits from another class is called a subclass To inherit a class from another class, put the name of superclass in parenthesis after the class name 44 Inheritance If a class inherits from another with the same attributes or methods, it overrides them 45 Inheritance Inheritance can also be indirect: one class inherits from another, and that class can inherit from a third class However, circular inheritance is not possible 46 Polymorphism Polymorphism: based on the greek words Poly (many) and morphism (forms) Polymorphic behaviour allows you to specify common methods in an “abstract” level, and implement them in particular instances Inheritance is one of the means to achieve polymorphism 47 Example Write a program defining a class Person. To create an instance of this class, you will have to provide the first and last name of the person. The class Person should also have the functionality (a method called Name()) to return a string with the name of the person in the format “first_name last_name”. The program should have another class, Employee, which can do all the things that the Person class do. However, to construct an instance of this class, you will have to provide the first and last name of the employee as well as the staff number. The class Employee should also have the functionality to return a string with the first and last name of the employee alongside the staff number in the format “first_name last_name, staff_number” (use the method Name() from the Person class to obtain the first and last name). 48 Example 49 11/2/24, 3:02 PM Review Test Submission: Self-check: Object-oriented... BMAN73701 Programming in Python for Business Analytics 2024-25 1st Semester Course Content Week 2, Lecture 2 (Xian Yang) - Object-oriented programming with Python Review Test Submission: Self-check: Object-oriented programming Review Test Submission: Self-check: Object-oriented programming User Melissa Hidayat Course BMAN73701 Programming in Python for Business Analytics 2024-25 1st Semester Test Self-check: Object-oriented programming Started 02/11/24 15:00 Submitted 02/11/24 15:01 Status Completed Attempt Score 30 out of 30 points Time Elapsed 1 minute Self-Test Student answers and score are not visible to the instructor. Results All Answers, Submitted Answers, Correct Answers Displayed Question 1 10 out of 10 points What type of object is a method? Selected Answer: Function Answers: Integer Class Function NoneType Question 2 10 out of 10 points What is the result of this code? https://online.manchester.ac.uk/webapps/assessment/review/review.jsp?attempt_id=_24173004_1&course_id=_83513_1&content_id=_16225329… 1/3 11/2/24, 3:02 PM Review Test Submission: Self-check: Object-oriented... Selected Answer: Bottom Correct Answer: Evaluation Method Correct Answer Case Sensitivity Exact Match Bottom Case Sensitive Question 3 10 out of 10 points Fill in the blanks to create a class and its contructors, taking one argument and assigning it to the “name” attribute. Then create an object of the class. [var1] Student: def [var2](self, name): self[var3] = name test = Student(“Bob”[var4] Specified Answer for: var1 class Specified Answer for: var2 __init__ Specified Answer for: var3.name Specified Answer for: var4 ) Correct Answers for: var1 Evaluation Method Correct Answer Case Sensitivity Exact Match class Case Sensitive Correct Answers for: var2 Evaluation Method Correct Answer Case Sensitivity Exact Match __init__ Case Sensitive Correct Answers for: var3 Evaluation Method Correct Answer Case Sensitivity Exact Match.name Case Sensitive Correct Answers for: var4 Evaluation Method Correct Answer Case Sensitivity Exact Match ) Case Sensitive https://online.manchester.ac.uk/webapps/assessment/review/review.jsp?attempt_id=_24173004_1&course_id=_83513_1&content_id=_16225329… 2/3 11/2/24, 3:02 PM Review Test Submission: Self-check: Object-oriented... Saturday, 2 November 2024 15:02:05 o'clock GMT ← OK https://online.manchester.ac.uk/webapps/assessment/review/review.jsp?attempt_id=_24173004_1&course_id=_83513_1&content_id=_16225329… 3/3