What is displayed after running this program?
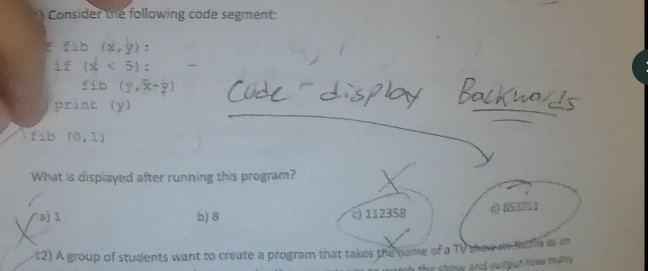
Understand the Problem
The question is asking for the output of a given code segment, specifically what value is displayed after running the program. This requires an understanding of the code's logic and recursion.
Answer
$8$, $5$, $3$, $2$, $1$
Answer for screen readers
The values displayed after running the program are $8$, $5$, $3$, $2$, $1$.
Steps to Solve
-
Initial Function Call The function
fib
is called with parameters $x = 0$ and $y = 1$. -
First Condition Check The first condition checks if $x < 5$. Since $0 < 5$ is true, we proceed to the recursive call.
-
First Recursive Call Invoke
fib(y, x + y)
which translates tofib(1, 0 + 1)
, resulting infib(1, 1)
. -
Next Condition Check Now, with $x = 1$ and $y = 1$, we check again if $x < 5$. Since $1 < 5$ is true, make another recursive call.
-
Second Recursive Call Invoke
fib(1, 1 + 1)
, resulting infib(1, 2)
. -
Next Condition Check With $x = 1$ and $y = 2$, check if $x < 5$. $1 < 5$ is still true, make another call.
-
Third Recursive Call Invoke
fib(2, 1 + 2)
, resulting infib(2, 3)
. -
Next Condition Check With $x = 2$ and $y = 3$, check if $x < 5$. Since $2 < 5$ is true, make another call.
-
Fourth Recursive Call Invoke
fib(3, 2 + 3)
, resulting infib(3, 5)
. -
Next Condition Check With $x = 3$ and $y = 5$, check if $x < 5$. Since $3 < 5$ is true, make another call.
-
Fifth Recursive Call Invoke
fib(4, 3 + 5)
, resulting infib(4, 8)
. -
Next Condition Check With $x = 4$ and $y = 8$, check if $x < 5$. $4 < 5$ is true, call again.
-
Sixth Recursive Call Invoke
fib(5, 4 + 8)
, resulting infib(5, 12)
. -
Final Condition Check Now, with $x = 5$, check if $x < 5$. Since $5 < 5$ is false, the recursion ends.
-
Print Execution Begins We now begin to return from the recursion and print the value of $y$:
- From
fib(5, 12)
nothing is printed yet. - From
fib(4, 8)
: print $8$. - From
fib(3, 5)
: print $5$. - From
fib(2, 3)
: print $3$. - From
fib(1, 2)
: print $2$. - From
fib(0, 1)
: print $1$.
Thus, the output will be printed in reverse order.
The values displayed after running the program are $8$, $5$, $3$, $2$, $1$.
More Information
This program demonstrates a recursive function that calculates Fibonacci-like pairs. It illustrates how recursion unwinds and how each recursive call waits to print its output until the base case is reached.
Tips
- Overlooking Base Cases: Forgetting that the recursive function stops when $x$ is not less than 5 can lead to infinite recursion.
- Assuming Immediate Outputs: People might expect outputs to display in the order calls are made, but in recursion, outputs can appear in reverse order due to how the stack unwinds.
AI-generated content may contain errors. Please verify critical information