Android App/Project Folder Structure PDF
Document Details
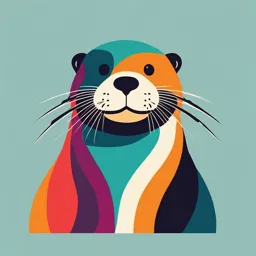
Uploaded by SelfDeterminationCarnelian6952
Tags
Summary
This document provides an overview of the components and structure required for Android application development using Android Studio. It includes explanations of Android application components and a walk-through of the project folder structure.
Full Transcript
Lec. #2: Anatomy of Android Application Mobile Applications Development 1 FIRST SEMESTER OF THE ACADEMIC YEAR 2021/2024 Android App. Components All the application components are defined in the android app description file (AndroidMainfest.xml) The basic core application components t...
Lec. #2: Anatomy of Android Application Mobile Applications Development 1 FIRST SEMESTER OF THE ACADEMIC YEAR 2021/2024 Android App. Components All the application components are defined in the android app description file (AndroidMainfest.xml) The basic core application components that can be used in Android application: ◦ Activities ◦ Intents ◦ Content Providers ◦ Broadcast Receivers ◦ Services 2 Activities Intents An activity represents a single screen with a In android, Intent is a messaging object which user interface (UI) and it will acts an entry is used to request an action from another point for the user’s to interact with app. component. For example, a contacts app that is having In android, intents are mainly used to multiple activities like showing a list of perform the following: contacts, add a new contact, and another Starting an Activity activity to search for the contacts. All these Starting a Service activities in the contact app are independent Delivering a Broadcast of each other but will work together to provide a better user experience. There are two types of intents available in android, those are Implicit Intents Explicit Intents 3 Services Broadcast Receivers Service is a component that keeps an app Broadcast Receiver is a component that will running in the background to perform long allow a system to deliver events to the app running operations based on our sending a low battery message to the app. requirements. The apps can also initiate broadcasts to let For Service, we don’t have any user interface other apps know that required data available and it will run the apps in background in a device to use it. play music in background when the user in different app. Generally, we use Intents to deliver broadcast events to other apps and Broadcast Receivers These are the three different types of use status bar notifications to let the user services: know that broadcast event occurs. Foreground Background Bound 4 Content Providers Content Providers are useful to exchange the data between the apps based on the requests. It can share the app data that stores in the file system, SQLite database, on the web or any other storage location that our app can access. By using it, other apps can query or modify the data of our app based on the permissions provided by content provider. For example, android provides a Content Provider (ContactsContract.Data) to manage contacts information, by using proper permissions any app can query the content provider to perform read and write operations on contacts information. 5 Additional Components There are additional components used to build the relationship between the previous components to implement our application logic: Component Description Fragments These are used to represent the portion of user interface in an activity Layouts These are used to define the user interface (UI) for an activity or app Views These are used to build a user interface for an app using UI elements like buttons, lists, etc. Resources To build an android app we required external elements like images, audio files, strings, dimens, etc. other than coding Manifest File It’s a configuration file (AndroidManifest.xml) for the application and it will contain the information about activities, intents, content providers, services, broadcast receivers, permissions, etc. 6 Android App / Project Folder Structure Once we setup android development environment using android studio and if we create a sample application using android studio, our project folder structure will be like as shown below. 7 Android App / Project Folder Structure The Android project structure on the disk might be differs from the above representation. To see the actual file structure of the project, select Project from the Project dropdown instead of Android. The android app project will contain different types of app modules, source code files, and resource files. We will explore all the folders and files in the android app. 8 Android App / Project Folder Structure Java Folder This folder will contain all the java source code (.java) files which we’ll create during the application development. res (Resources) Folder It’s an important folder that will contain all non-code resources, such as bitmap images, UI strings, XML layouts like as shown below. Drawable Folder (res/drawable) It will contain the different types of images as per the requirement of application. It’s a best practice to add all the images in a drawable folder other than app/launcher icons for the application development. 9 Android App / Project Folder Structure Layout Folder (res/layout) This folder will contain all XML layout files which we used to define the user interface of our application. Following is the structure of the layout folder in the android application. Mipmap Folder (res/mipmap) This folder will contain app / launcher icons that are used to show on the home screen. It will contain different density type of icons such as hdpi, mdpi, xhdpi, xxhdpi, xxxhdpi, to use different icons based on the size of the device. Values Folder (res/values) This folder will contain various XML files, such as strings, colors, style definitions and a static array of strings or integers. Following is the structure of the values folder in android application. 10 Android App / Project Folder Structure Gradle Scripts In android, Gradle means automated build system and by using this we can define a build configuration that applies to all modules in our application. In Gradle build.gradle (Project), and build.gradle (Module) files are useful to build configurations that apply to all our app modules or specific to one app module Android Layout File (activity_main.xml) The UI of our application will be designed in this file and it will contain Design and Text modes. It will exists in the layouts folder and the structure of activity_main.xml file in Design mode 11 AndroidManifest.xml 12 AndroidManifest.xml Every application must have an It declares which permissions the application must AndroidManifest.xml file (with precisely that have in order to access protected parts of the API name) in its root directory. and interact with other applications. It also declares the permissions that others are It presents essential information about the required to have in order to interact with the application to the Android system, information application's components. the system must have before it can run any of the It lists the Instrumentation classes that provide application's code. profiling and other information as the application is running. These declarations are present in the Among other things, the manifest does the manifest only while the application is being following: developed and tested; they're removed before the It names the Java package for the application. The application is published. package name serves as a unique identifier for the It declares the minimum level of the Android API application. that the application requires. It describes the components of the application. It lists the libraries that the application must be It determines which processes will host application linked against. components. 13 Reading* https://stuff.mit.edu/afs/sipb/project/android/docs/guide/topics/manifest/manifest-intro.html 14 Application Resources Resources are the additional files and static content that your code uses, such as bitmaps, layout definitions, user interface strings, animation instructions, and more. You should always externalize app resources such as images and strings from your code, so that you can maintain them independently. You should also provide alternative resources for specific device configurations, by grouping them in specially-named resource directories. At runtime, Android uses the appropriate resource based on the current configuration. For example, you might want to provide a different UI layout depending on the screen size or different strings depending on the language setting. Once you externalize your app resources, you can access them using resource IDs that are generated in your project's R class. This document shows you how to group your resources in your Android project and provide alternative resources for specific device configurations, and then access them from your app code or other XML files. 15 Application Resources You should place each type of resource in a specific subdirectory of your project's res/ directory. For example, here's the file hierarchy for a simple project: 16 Application Resources Resource directories supported inside project res/ directory. Directory Resource Type animator/ XML files that define property animations. anim/ XML files that define tween animations. color/ XML files that define a state list of colors. drawable/ Bitmap files (.png,.jpg,.gif) or XML files that are compiled into on of a drawable resource subtypes like shapes, and animation drawables mipmap/ Drawable files for different launcher icon densities. layout/ XML files that define a user interface layout. menu/ XML files that define app menus, such as an Options Menu, Context Menu, or Sub Menu raw/ Arbitrary files to save in their raw form. To open these resources with a raw InputStream, call Resources.openRawResource() with the resource ID, which is R.raw.filename. 17 Application Resources Directory Resource Type values/ XML files that contain simple values for example: arrays.xml for resource arrays colors.xml for color values dimens.xml for dimension values. strings.xml for string values. styles.xml for styles. xml/ Arbitrary XML files that can be read at runtime by calling Resources.getXML(). Various XML configuration files must be saved here, such as a searchable configuration. font/ Font files with extensions such as.ttf,.otf, or.ttc, or XML files that include a element. 18