Python Mock Exam PDF
Document Details
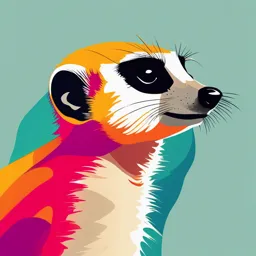
Uploaded by SharperEmpowerment5424
Tags
Summary
Mock exam questions for a Python programming course cover topics including the use of lists, variables, and functions in a programming context. The code samples contain instructions to practice for the programming questions.
Full Transcript
# Question 01 numbers = [] # Variables: to store user-entered numbers while : # TODO: keep this an infinite loop num = int(input("Enter a positive integer: ")) if num > 0: numbers.append(num) # Variables: storing the number in a list else: print("Please enter a posi...
# Question 01 numbers = [] # Variables: to store user-entered numbers while : # TODO: keep this an infinite loop num = int(input("Enter a positive integer: ")) if num > 0: numbers.append(num) # Variables: storing the number in a list else: print("Please enter a positive integer.") # TODO: write code to start a new iteration cont = input("Do you want to enter another number? (yes/no): ") # Standard input if cont.lower() != : # TODO: complete the condition break # TODO: write code to exit the while loop choice = input("Enter 'sum' to calculate sum, 'max' for maximum, 'min' for minimum: ") match choice: # handling user's choice case 'sum': = # TODO: initialize total for n in numbers: # For loop: iterating over numbers total += n # Arithmetic operator: summing numbers print(f"The sum is {total}") # Standard output case 'max': = # TODO: initialize maximum for n in numbers: # For loop: finding maximum if n maximum: # TODO: insert appropriate operator between n and maximum maximum = n print(f"The maximum number is {maximum}") # Standard output case 'min': = # TODO: initialize minimum for n in numbers: # For loop: finding minimum if n < minimum: # TODO: insert appropriate operator between n and minimum minimum = n print(f"The minimum number is {minimum}") # Standard output case : # TODO: complete the default case print("Invalid command") # Standard output #%% # Question 02 # TODO: Initialize list names with names: Alice, Bob, Charlie, and Diana names = grades = [85, 58, 92, 76] # TODO: create a list of tuples from the lists: names and grades student_data = # TODO: convert student_data into the two lists names_unzipped, grades_unzipped = # TODO: create a dictionary from student_data student_dict = # TODO: modify to allow 60 and above grades passed_students = {k: v for k, v in student_dict.items()} all_students_set = set(names) # Creating set from list passed_students_set = set(passed_students.keys()) # Creating set from dict keys # TODO: compute the set of failed students failed_students = messages = [f"Congratulations, {name}!" for name in passed_students] # List comprehension # TODO: complete the lambda function # TODO: complete the slice to pick top 3 students top_students = sorted(student_dict.items(), key=lambda x: , reverse=True)[:] try: student_data = 'Ann' # Attempt to modify tuple (Tuples are immutable) except TypeError: pass # Handle immutability exception del student_dict['Bob'] # Delete operation in dictionary # TODO: write down the indices on which 90 and 95 will be stored grades[:2] = [90, 95] # Assignment to slices in a list del names[-1] # Delete last item using slicing and del high_scorers = {name for name, grade in student_dict.items() if grade > 80} # Set comprehension # TODO: write down names of the high scorers #%% # Question 03 from abc import ABC, abstractmethod # TODO: correct args and kwargs to accept multiple positional and keyword arguments respectively def create_shapes(args, shape_type='Circle', kwargs): shapes = [] for dim in args: shape = globals()[shape_type](dim, **kwargs) shapes.append(shape) return shapes def compute_total_area(shapes): # Function definition total = 0 for shape in shapes: # TODO: call appropriate function to compute shape's area total += return total # TODO: make the Shape class abstract class Shape : count = 0 # TODO: make the area() to be overriden def area(self): pass # TODO: derive Circle from Shape class Circle : count = 0 def __init__(self, radius): self.radius = radius Circle.count += 1 Shape.count += 1 def area(self): return 3.14 * self.radius ** 2 class Rectangle(Shape): def __init__(self, width, height): self.width = width self.height = height # TODO: increment the class variable of Shape by 1 def area(self): return self.width * self.height class Square(Rectangle): def __init__(self, side): super().__init__(side, side) class ColorMixin: def __init__(self, color='Red'): self.color = color # TODO: derive ColoredSquare from Square, ColorMixin class ColoredSquare : def __init__(self, side, color='Red'): # TODO: call both the init methods with appropriate parameters Square.__init__( ) ColorMixin.__init__( ) shapes = create_shapes(2, 3, 4, shape_type='ColoredSquare', color='Blue') total_area = compute_total_area(shapes) print(f"Total area: {total_area}") print(f"Shape count: {Shape.count}, Circle count: {Circle.count}") # TODO: write down the values of Shape.count and Circle.count at this point for shape in shapes: # TODO: insert shape color and area in {} and {}, respectively print(f"Shape color: {}, Area: {}") #%% # Question 04 import numpy as np import pandas as pd data = np.genfromtxt('data.csv', delimiter=',') # Load data from CSV (NumPy array creation) col_means = np.nanmean(data, axis=0) # Aggregation function, operations on NaN # TODO: pass a boolean mask of data to where() inds = np.where( ) # Find indices of NaNs data[inds] = np.take(col_means, inds) # Replace NaNs with column means mean = data.mean(axis=0) # Aggregation function std = data.std(axis=0) # TODO: use vectorization to subtract mean from # each data element and divide it by std normalized = # Vectorization and broadcasting weights = np.linspace(1, 2, data.shape) # Create array using linspace # TODO: perform element-wise multiplication # between the normalized values and the weights weighted = # Element-wise multiplication, upcasting # TODO: what does -1 mean here? reshaped = weighted.reshape(-1, data.shape*2) # Array shape manipulation split1, split2 = np.array_split(reshaped, 2) # Splitting array into smaller ones # TODO: stack split1 and split2 vertically v_stacked = # TODO: stack split1 and split2 horizontally h_stacked = # TODO: apply the universal functin exp() to v_stacked and # store the result in exp_array exp_array = # TODO: create a shallow copy of exp_array shallow_copy = deep_copy = exp_array.copy() dims, shape = exp_array.ndim, exp_array.shape # Array attributes: ndim, shape size, dtype = exp_array.size, exp_array.dtype itemsize, data_buffer = exp_array.itemsize, exp_array.data # TODO: create a dataframe from NumPy array exp_array df = pd.DataFrame(exp_array) df['Sum'] = df.sum(axis=1) # Add new column (aggregation function) df.iloc[[1, 3], [0, 2]] = np.nan # Random modification to cells (introduce NaNs) df = df.dropna() # Handle missing data (drop rows with NaN) df['Category'] = pd.cut(df['Sum'], bins=3, labels=False) # Convert numerical to categorical data # TODO: drop rows of df where 'Category' is 0 df = df[ ] # Drop rows based on condition print(df.head()) # Viewing data: head() print(df.info()) # DataFrame info() df.to_csv('modified_data.csv', index=False) # Save DataFrame to CSV file #%% # Question 05 import numpy as np import scipy.integrate as integrate import scipy.interpolate as interp import scipy.ndimage as ndimage import matplotlib.pyplot as plt # Define quadratic function (SciPy integration of quadratic function) def f(x, a=1, b=0, c=0): return a * x**2 + b * x + c # TODO: store the returned value of integration in variable area # but disregard/ignore storing the error estimate = integrate.quad(f, 0, 10) x = np.linspace(0, 10, 100) # Generate data (NumPy array creation) y = f(x) # TODO: apply Gaussian filter to y, with sigma=2 y_smooth = # TODO: compute cubic spline interpolation for datasets x and y interp_func = interp.interp1d(x, y, kind='cubic') # Interpolation x_new = np.linspace(0, 10, 400) y_interp = interp_func(x_new) # TODO: create two vertically stacked sub-plots each of size (8, 6) fig, axs = plt. # Plot original data (first subplot) # TODO: place the positional arguments x and y appropriately axs.plot(label='Original', color='blue', linestyle='-', linewidth=2) # Styling line # TODO: place the positional arguments x and y appropriately axs.scatter(color='blue', marker='o', s=10) axs.set_title('Quadratic Function') # Title axs.set_xlabel('x Axis') axs.set_ylabel('y Axis') # TODO: show plot legend axs. # TODO: set plot grid axs. # TODO: axs.tick_params(axis='both', which='both', direction='inout') # Major and minor ticks # Plot smoothed and interpolated data (second subplot) axs.plot(x, y_smooth, label='Smoothed', color='red', linestyle='--') # Smoothed data axs.plot(x_new, y_interp, label='Interpolated', color='green', linestyle='-.') # Interpolated data axs.set_title('Smoothed and Interpolated Data') # Title axs.set_xlabel('x Axis') # x label axs.set_ylabel('y Axis') # y label # TODO: add additional y axis ax_twin = axs. ax_twin.plot(x, y, label='Original Twin', color='gray', alpha=0.3) ax_twin.set_ylabel('Twin y Axis') for ax in axs: ax.spines['top'].set_visible(False) # Spine styling ax.tick_params(axis='x', which='major', labelsize=8) # Major tick label size plt.tight_layout() plt.show()