Introduction to Python PDF
Document Details
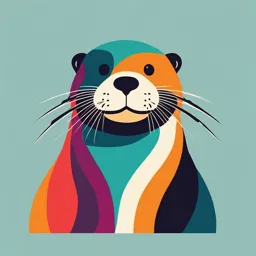
Uploaded by StylizedFibonacci4990
Tags
Summary
This document provides an introductory overview of the Python programming language. It covers fundamental concepts like data types, variables, operators, and programming basics. The document is organized into chapters and sections, making it informative and easy to follow. The document also covers characteristics and features.
Full Transcript
CHAPTER-1 INTRODUTION TO PYTHON 1.1 Introduction: General-purpose Object Oriented Programming language. High-level language Developed in late 1980 by Guido van Rossum at National Research Institute for Mathematics and Computer Science in the Nethe...
CHAPTER-1 INTRODUTION TO PYTHON 1.1 Introduction: General-purpose Object Oriented Programming language. High-level language Developed in late 1980 by Guido van Rossum at National Research Institute for Mathematics and Computer Science in the Netherlands. It is derived from programming languages such as ABC, Modula 3, small talk, Algol- 68. It is Open Source Scripting language. It is Case-sensitive language (Difference between uppercase and lowercase letters). One of the official languages at Google. 1.2 Characteristics of Python: Interpreted: Python source code is compiled to byte code as a.pyc file, and this byte code can be interpreted by the interpreter. Interactive Object Oriented Programming Language Easy & Simple Portable Scalable: Provides improved structure for supporting large programs. Integrated Expressive Language 1.3 Python Interpreter: Names of some Python interpreters are: PyCharm Python IDLE The Python Bundle pyGUI Sublime Text etc. There are two modes to use the python interpreter: i. Interactive Mode ii. Script Mode Page 2 CHAPTER-2 PYTHON FUNDAMENTALS 2.1 Python Character Set : It is a set of valid characters that a language recognize. Letters: A-Z, a-z Digits : 0-9 Special Symbols Whitespace 2.2 TOKENS Token: Smallest individual unit in a program is known as token. There are five types of token in python: 1. Keyword 2. Identifier 3. Literal 4. Operators 5. Punctuators 1. Keyword: Reserved words in the library of a language. There are 33 keywords in python. False class finally is return break None continue for lambda try except True def from nonlocal while in and del global not with raise as elif if or yield assert else import pass All the keywords are in lowercase except 03 keywords (True, False, None). Page 5 2. Identifier: The name given by the user to the entities like variable name, class-name, function-name etc. Rules for identifiers: It can be a combination of letters in lowercase (a to z) or uppercase (A to Z) or digits (0 to 9) or an underscore. It cannot start with a digit. Keywords cannot be used as an identifier. We cannot use special symbols like !, @, #, $, %, + etc. in identifier. _ (underscore) can be used in identifier. Commas or blank spaces are not allowed within an identifier. 3. Literal: Literals are the constant value. Literals can be defined as a data that is given in a variable or constant. Literal String Literal Numeric Boolean Special Collections int float complex True False None A. Numeric literals: Numeric Literals are immutable. Eg. 5, 6.7, 6+9j Page 6 B. String literals: String literals can be formed by enclosing a text in the quotes. We can use both single as well as double quotes for a String. Eg: "Aman" , '12345' Escape sequence characters: \\ Backslash \’ Single quote \” Double quote \a ASCII Bell \b Backspace \f ASCII Formfeed \n New line charater \t Horizontal tab C. Boolean literal: A Boolean literal can have any of the two values: True or False. D. Special literals: Python contains one special literal i.e. None. None is used to specify to that field that is not created. It is also used for end of lists in Python. E. Literal Collections: Collections such as tuples, lists and Dictionary are used in Python. Page 7 2.4 Basic terms of a Python Programs: A. Blocks and Indentation B. Statements C. Expressions D. Comments A. Blocks and Indentation: Python provides no braces to indicate blocks of code for class and function definition or flow control. Maximum line length should be maximum 79 characters. Blocks of code are denoted by line indentation, which is rigidly enforced. The number of spaces in the indentation is variable, but all statements within the block must be indented the same amount. for example – if True: print(“True”) else: print(“False”) B. Statements A line which has the instructions or expressions. C. Expressions: A legal combination of symbols and values that produce a result. Generally it produces a value. D. Comments: Comments are not executed. Comments explain a program and make a program understandable and readable. All characters after the # and up to the end of the physical line are part of the comment and the Python interpreter ignores them. Page 8 There are two types of comments in python: i. Single line comment ii. Multi-line comment i. Single line comment: This type of comments start in a line and when a line ends, it is automatically ends. Single line comment starts with # symbol. Example: if a>b: # Relational operator compare two values ii. Multi-Line comment: Multiline comments can be written in more than one lines. Triple quoted ‘ ’ ’ or “ ” ”) multi-line comments may be used in python. It is also known as docstring. Example: ‘’’ This program will calculate the average of 10 values. First find the sum of 10 values and divide the sum by number of values ‘’’ Page 9 Multiple Statements on a Single Line: The semicolon ( ; ) allows multiple statements on the single line given that neither statement starts a new code block. Example:- x=5; print(“Value =” x) 2.5 Variable/Label in Python: Definition: Named location that refers to a value and whose value can be used and processed during program execution. Variables in python do not have fixed locations. The location they refer to changes every time their values change. Creating a variable: A variable is created the moment you first assign a value to it. Example: x=5 y = “hello” Variables do not need to be declared with any particular type and can even change type after they have been set. It is known as dynamic Typing. x = 4 # x is of type int x = "python" # x is now of type str print(x) Rules for Python variables: A variable name must start with a letter or the underscore character A variable name cannot start with a number A variable name can only contain alpha-numeric characters and underscore (A-z, 0-9, and _ ) Variable names are case-sensitive (age, Age and AGE are three different variables) Page 10 Python allows assign a single value to multiple variables. Example: x=y=z=5 You can also assign multiple values to multiple variables. For example − x , y , z = 4, 5, “python” 4 is assigned to x, 5 is assigned to y and string “python” assigned to variable z respectively. x=12 y=14 x,y=y,x print(x,y) Now the result will be 14 12 Lvalue and Rvalue: An expression has two values. Lvalue and Rvalue. Lvalue: the LHS part of the expression Rvalue: the RHS part of the expression Python first evaluates the RHS expression and then assigns to LHS. Example: p, q, r= 5, 10, 7 q, r, p = p+1, q+2, r-1 print (p,q,r) Now the result will be: 6 6 12 Note: Expressions separated with commas are evaluated from left to right and assigned in same order. Page 11 int( ) - constructs an integer number from an integer literal, a float literal or a string literal. Example: x = int(1) # x will be 1 y = int(2.8) # y will be 2 z = int("3") # z will be 3 float( ) - constructs a float number from an integer literal, a float literal or a string literal. Example: x = float(1) # x will be 1.0 y = float(2.8) # y will be 2.8 z = float("3") # z will be 3.0 w = float("4.2") # w will be 4.2 str( ) - constructs a string from a wide variety of data types, including strings, integer literals and float literals. Example: x = str("s1") # x will be 's1' y = str(2) # y will be '2' z = str(3.0) # z will be '3.0' Reading a number from a user: x= int (input(“Enter an integer number”)) 2.8 OUTPUT using print( ) statement: Syntax: print(object, sep=, end=) Page 13 object : It can be one or multiple objects separated by comma. sep : sep argument specifies the separator character or string. It separate the objects/items. By default sep argument adds space in between the items when printing. end : It determines the end character that will be printed at the end of print line. By default it has newline character( ‘\n’ ). Example: x=10 y=20 z=30 print(x,y,z, sep=’@’, end= ‘ ‘) Output: 10@20@30 Page 14 CHAPTER-3 DATA HANDLING 3.1 Data Types in Python: Python has Two data types – 1. Primitive Data Type (Numbers, String) 2. Collection Data Type (List, Tuple, Set, Dictionary) Data Types Primitive Collection Data Type Data Type Number String List Tuple Set Dictionary int float complex 1. Primitive Data Types: a. Numbers: Number data types store numeric values. There are three numeric types in Python: int float complex Example: w=1 # int y = 2.8 # float z = 1j # complex Page 15 integer : There are two types of integers in python: int Boolean int: int or integer, is a whole number, positive or negative, without decimals. Example: x=1 y = 35656222554887711 z = -3255522 Boolean: It has two values: True and False. True has the value 1 and False has the value 0. Example: >>>bool(0) False >>>bool(1) True >>>bool(‘ ‘) False >>>bool(-34) True >>>bool(34) True float : float or "floating point number" is a number, positive or negative, containing one or more decimals. Float can also be scientific numbers with an "e" to indicate the power of 10. Example: x = 1.10 y = 1.0 z = -35.59 a = 35e3 b = 12E4 c = -87.7e100 Page 16 Real and imaginary part of a number can be accessed through the attributes real and imag. b. String: Sequence of characters represented in the quotation marks. Python allows for either pairs of single or double quotes. Example: 'hello' is the same as "hello". Python does not have a character data type, a single character is simply a string with a length of 1. The python string store Unicode characters. Each character in a string has its own index. String is immutable data type means it can never change its value in place. 2. Collection Data Type: List Tuple Set Dictionary Page 17 3.2 MUTABLE & IMMUTABLE Data Type: Mutable Data Type: These are changeable. In the same memory address, new value can be stored. Example: List, Set, Dictionary Immutable Data Type: These are unchangeable. In the same memory address new value cannot be stored. Example: integer, float, Boolean, string and tuple. 3.3 Basic Operators in Python: i. Arithmetic Operators ii. Relational Operator iii. Logical Operators iv. Bitwise operators v. Assignment Operators vi. Other Special Operators o Identity Operators o Membership operators i. Arithmetic Operators: To perform mathematical operations. RESULT OPERATOR NAME SYNTAX (X=14, Y=4) + Addition x+y 18 _ Subtraction x–y 10 * Multiplication x*y 56 / Division (float) x/y 3.5 // Division (floor) x // y 3 % Modulus x%y 2 ** Exponent x**y 38416 Page 18 Example: >>>x= -5 >>>x**2 >>> -25 ii. Relational Operators: Relational operators compare the values. It either returns True or False according to the condition. RESULT OPERATOR NAME SYNTAX (IF X=16, Y=42) False > Greater than x>y True < Less than x= Greater than or equal to x >= y True b: print(“a is greater”) else: print(“b is greater”) 3. elif statement: It is short form of else-if statement. If the previous conditions were not true, then do this condition". It is also known as nested if statement. Example: a=input(“Enter first number”) b=input("Enter Second Number:") if a>b: Page 27 print("a is greater") elif a==b: print("both numbers are equal") else: print("b is greater") 4.2 LOOPS in PYTHON Loop: Execute a set of statements repeatedly until a particular condition is satisfied. There are two types of loops in python: 1. while loop 2. for loop while Loops in loop Python for loop Page 28 1. while loop: With the while loop we can execute a set of statements as long as a condition is true. It requires to define an indexing variable. Example: To print table of number 2 i=2 while i>>math.sqrt(49) 7.0 2 ceil( ) Returns the upper integer >>>math.ceil(81.3) 82 3 floor( ) Returns the lower integer >>>math.floor(81.3) 81 4 pow( ) Calculate the power of a number >>>math.pow(2,3) 8.0 5 fabs( ) Returns the absolute value of a number >>>math.fabs(-5.6) 5.6 6 exp( ) Returns the e raised to the power i.e. e3 >>>math.exp(3) 20.085536923187668 b. Function in random module: random module has a function randint( ). randint( ) function generates the random integer values including start and end values. Syntax: randint(start, end) It has two parameters. Both parameters must have integer values. Example: import random n=random.randint(3,7) *The value of n will be 3 to 7. 3. USER DEFINED FUNCTIONS: The syntax to define a function is: def function-name ( parameters) : #statement(s) Page 38 Where: Keyword def marks the start of function header. A function name to uniquely identify it. Function naming follows the same rules of writing identifiers in Python. Parameters (arguments) through which we pass values to a function. They are optional. A colon (:) to mark the end of function header. One or more valid python statements that make up the function body. Statements must have same indentation level. An optional return statement to return a value from the function. Example: def display(name): print("Hello " + name + " How are you?") 5.3 Function Parameters: A functions has two types of parameters: 1. Formal Parameter: Formal parameters are written in the function prototype and function header of the definition. Formal parameters are local variables which are assigned values from the arguments when the function is called. 2. Actual Parameter: When a function is called, the values that are passed in the call are called actual parameters. At the time of the call each actual parameter is assigned to the corresponding formal parameter in the function definition. Example : def ADD(x, y): #Defining a function and x and y are formal parameters z=x+y print("Sum = ", z) a=float(input("Enter first number: " )) b=float(input("Enter second number: " )) ADD(a,b) #Calling the function by passing actual parameters In the above example, x and y are formal parameters. a and b are actual parameters. Page 39 5.4 Calling the function: Once we have defined a function, we can call it from another function, program or even the Python prompt. To call a function we simply type the function name with appropriate parameters. Syntax: function-name(parameter) Example: ADD(10,20) OUTPUT: Sum = 30.0 How function works? def functionName(parameter): ….. … ….. … ….. … ….. … functionName(parameter) ….. … ….. … The return statement: The return statement is used to exit a function and go back to the place from where it was called. There are two types of functions according to return statement: a. Function returning some value (non-void function) b. Function not returning any value (void function) Page 40 a. Function returning some value (non-void function) : Syntax: return expression/value Example-1: Function returning one value def my_function(x): return 5 * x Example-2 Function returning multiple values: def sum(a,b,c): return a+5, b+4, c+7 S=sum(2,3,4) # S will store the returned values as a tuple print(S) OUTPUT: (7, 7, 11) Example-3: Storing the returned values separately: def sum(a,b,c): return a+5, b+4, c+7 s1, s2, s3=sum(2, 3, 4) # storing the values separately print(s1, s2, s3) OUTPUT: 7 7 11 b. Function not returning any value (void function) : The function that performs some operationsbut does not return any value, called void function. def message(): print("Hello") m=message() print(m) Page 41 5.7 lambda Function: lambda keyword, is used to create anonymous function which doesn’t have any name. While normal functions are defined using the def keyword, in Python anonymous functions are defined using the lambda keyword. Syntax: lambda arguments: expression Lambda functions can have any number of arguments but only one expression. The expression is evaluated and returned. Lambda functions can be used wherever function objects are required. Example: value = lambda x: x * 4 print(value(6)) Output: 24 Page 44