PSPD III Programming in C PDF
Document Details
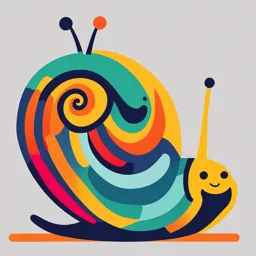
Uploaded by CatchyArtInformel
MIT World Peace University
Tags
Summary
This document explains C programming concepts and elements, including preprocessor directives, data types, operators, and control structures. It includes examples of C programs, and a breakdown of the C language.
Full Transcript
Problem Solving & Program Design 3.0 # Education with values Department of Polytechnic & Skill Development 1 Ove...
Problem Solving & Program Design 3.0 # Education with values Department of Polytechnic & Skill Development 1 Overview of C Course Outcome: Use basic ‘C’ data concepts to write algorithm steps and flowchart for given problem statements. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 2 C Language Elements The C program in has two parts: preprocessor directives and the main function. The preprocessor directives are commands that give instructions to the C preprocessor, whose job it is to modify the text of a C program before it is compiled. A preprocessor directive begins with a number symbol (#) as its first nonblank character. Preprocessor directive is a C program line beginning with # that provides an instruction to the preprocessor. Preprocessor is a system program that modifies a C program prior to its compilation. Library is a collection of useful functions and symbols that may be accessed by a program. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 3 3G of Procedural Programming Languages Line No 1 // Test Program 2 Comments 2 // Addition program. 3 #include Lines 1 and 2 4 5 // function main begins program execution begin with //, indicating that these 6 int main( ) 7 { two lines are comments. You insert 8 int integer1; // first number to be entered by user 9 int integer2; // second number to be entered by user comments to document programs 10 11 printf( "Enter first integer\n" ); // prompt and improve program readability. 12 scanf( "%d", &integer1 ); // read an integer 13 Comments do not cause the 14 printf( "Enter second integer\n" ); // prompt 15 scanf( "%d", &integer2 ); // read an integer computer to perform actions when 16 17 18 int sum; // variable in which sum will be stored sum = integer1 + integer2; // assign total to sum you execute programs—they’re 19 20 printf( "Sum is %d\n", sum ); // print sum simply ignored. 21 } // end function main # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 4 3G of Procedural Programming Languages Line No 1 // Test Program 2 Line 3 tells the preprocessor to 2 // Addition program. 3 #include include the contents of the standard 4 5 // function main begins program execution input/output header (). This #include Preprocessor Directive is a file containing information the Line 3 compiler uses to ensure that you #include is a C correctly use standard input/output preprocessor directive. library functions such as The preprocessor handles lines printf, scanf,getch etc. beginning with # before Line 4 is left blank to improve compilation. readability. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 5 3G of Procedural Programming Languages Header files stdlib.h stdint.h assert.h string.h stdio.h complex.h tgmath.h time.h ctype.h errno.h wchar.h inttypes.h fenv.h wctype.h iso646.h float.h limits.h locale.h signal.h math.h stdarg.h setjmp.h stdbool.h stddef.h # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 6 3G of Procedural Programming Languages Line No 1 // Test Program 2 C programs consist of functions, one 2 // Addition program. 3 #include of which must be main. Every 4 5 // function main begins program execution program begins executing at the 6 int main ( ) function main. Before every function The main Function write a comment (as in line 5) stating Line 6 the function’s purpose. int main( ) { Functions return information. The is a part of every C program. The keyword int to the left of main parentheses after main indicate that indicates that main “returns” an main is a program building block integer (whole number) value. called a function. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 7 3G of Procedural Programming Languages Line No 8 9 These definitions specify that int integer1; // first number to be entered by user int integer2; // second number to be entered by user 10 integer1 and integer2 have type int. This means they’ll hold Line 8 & Line 9 are definitions whole-number integer values, such as int integer1 = 0; 7, –11, 0 and 31914. int integer2 = 0; Line 7 & Line 21 The names integer1 and integer2 are The left brace (line 7) begins main's variables—locations in mem- body and the corresponding right ory where the program can store brace (line 21) ends it. values for later use. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 8 Declaration A declaration declares a (variable) name. When we declare a variable, we specify its type and variable’s name. When we declare a variable, the compiler reserves memory for our variable. This occupied space is called an object or data object in memory. These data objects are accessed by names we call variables. We need to declare a variable before we can use it. To declare a variable, we put the type name before the variable name and end the entire statement with a semicolon (;). The declaration pseudo-code looks like: type name variable name; # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 9 Types char – holds character values ( 1 Byte ) 1,2,a,b etc int – holds whole numbers (4 Bytes) 1,2 etc float – holds floating-point values of single precision ( 4 Bytes) Float can hold upto 6 decimal digits double – holds floating-point values of double precision ( 8 Bytes ) Double can hold upto 15 decimal digits # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 10 3G of Procedural Programming Languages 10 11 printf( "Enter first integer\n" ); // prompt 12 scanf( "%d", &integer1 ); // read an integer 13 14 printf( "Enter second integer\n" ); // prompt Line 11 & Line 12 printf("Enter first integer: "); // prompt displays "Enter first integer: ". This message is called a prompt because it tells the user to take a specific action. scanf("%d", &integer1); // read an integer uses scanf to obtain a value from the user. The function reads from the standard input, which is usually the keyboard. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 11 3G of Procedural Programming Languages Line 11 & Line 12 The “f ” in scanf stands for “formatted.” This scanf has two arguments—"%d“ and &integer1. The "%d" is the format control string. It indicates the type of data the user should enter. The %d conversion specification specifies that the data should be an integer—the d stands for “decimal integer”. A % character begins each conversion specification. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 12 3G of Procedural Programming Languages Line 11 & Line 12 scanf ’s second argument begins with an ampersand (&) followed by the variable name. The & is the address operator and, when combined with the variable name, tells scanf the location (or address) in memory of the variable integer1. scanf then stores the value the user enters at that memory location # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 13 3G of Procedural Programming Languages Line 11 & Line 12 When line 11 executes, the computer waits for the user to enter a value for integer1. The user types an integer, then presses the Enter key (or Return key) to send the number to the computer. The computer then places the number (or value) in integer1. Any subsequent references to integer1 in the program use this same value. Functions printf and scanf facilitate interaction between the user and the computer. This interaction resembles a dialogue and is often called interactive computing. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 14 3G of Procedural Programming Languages 14 printf( "Enter second integer\n" ); // prompt 15 scanf( "%d", &integer2 ); // read an integer 16 17 int sum; // variable in which sum will be stored 18 calculates the total of variables sum = integer1 + integer2; // assign total to sum Defining the sum Variable integer1 and integer2, then assigns Lines 17 the result to variable sum using the defines the int variable sum before assignment operator (=). we use sum in line 18. The statement is read as, “sum gets Assignment Statement the value of the expression integer1 Line 18 + integer2.” sum = integer1 + integer2; // assign Most calculations are performed in total to sum assignments. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 15 3G of Procedural Programming Languages Binary Operators The = operator and the + operator are binary operator & each has two operands. The + operator’s operands are integer1 and integer2. The = operator’s operands are sum and the value of the expression integer1 + integer2. Place spaces on either side of a binary operator to make the operator stand out and make the program more readable # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 16 3G of Procedural Programming Languages 17 int sum; // variable in which sum will be stored 18 sum = integer1 + integer2; // assign total to sum 19 20 printf( "Sum is %d\n", sum ); // print sum 21 } // end function main %d is a placeholder for an integer. Printing with a Format Control String The sum is the value to insert in Line 20 place of %d. printf("Sum is %d\n", sum); // print The conversion specification for an sum integer (%d) is the same in both contains some literal characters to printf and scanf. display ("Sum is ") and the conversion specification %d # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 17 Operators Arithmetic Operators # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 18 Operators Integer Division and Remainder Operator Integer division (that is, dividing one integer by another) yields an integer result, so 7 / 4 evaluates to 1, and 17 / 5 evaluates to 3. The integer-only remainder operator, %, yields the remainder after integer division, so 7 % 4 yields 3 and 17 % 5 yields 2. An attempt to divide by zero usually is undefined on computer systems. If 34 chocolates are distributed equally between 6 students, how many chocolates each student gets & how many chocolates are left ? # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 19 Operators Decision Making: Equality and Relational Operators Executable statements either perform actions like calculations, input and output or make decisions. For example, a program might determine whether a person’s grade on an exam is greater than or equal to 50, so it can decide whether to print the message “Congratulations! You passed.” A condition is an expression that can be true (that is, the condition is met) or false (that is, the condition isn’t met). If … Then statement # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 20 Operators Equality and Relational Operators # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 21 Operators Logical Operators To test multiple conditions in the process of making a decision, we had to perform these tests in separate statements or in nested if or if…else statements. C provides logical operators that may be used to form more complex conditions by combining simple conditions. The logical operators are && (logical AND), || (logical OR) and ! (logical NOT, which is also called logical negation). # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 22 Operators Logical AND (&&) Operator Suppose we wish to ensure that two conditions are both true before we choose a certain path of execution,We can use the logical operator && if (gender == 1 && age >= 65) { printf(“Senior Female”); } This if statement contains two simple conditions.The condition gender== 1 might, for example, determine whether a person is a female. The condition age >= 65 determines whether a person is a senior citizen. If both of the simple conditions are true, output is shown as Senior Female. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 23 Operators Logical OR (||) Operator Suppose we wish to ensure at some point in a program that either or both of two conditions are true, we use the || operator. if (semesterAverage >= 90 || finalExam >= 90) { printf("Student grade is A"); } This statement contains two simple conditions. The condition semesterAverage >= 90 & the condition finalExam >= 90 determines whether the student deserves an “A” because of entire semester and an outstanding performance on the final exam respectively. If either of statement is true student will get A grade. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 24 Operators Logical Negation (!) Operator The ! (logical negation) operator is used to to “reverse” the meaning of a condition. The logical negation operator has a single condition as an operand. You use it when you’re interested in choosing a path of execution if the operand condition is false, such as in the following program segment: if (grade != sentinelValue) { printf("The next grade is %f\n", grade); } # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 25 Operators # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 26 Operators Various Operators & If Statement 1 // PSPD 3 2 // Using if statements, relational 3 // operators, and equality operators. 4 #include 5 6 // function main begins program execution 7 int main(void) { 8 printf("Enter two integers, and I will tell you\n"); 9 printf("the relationships they satisfy: "); # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 27 Operators Various Operators & If Statement 10 11 int number1; // first number to be read from user 12 int number2; // second number to be read from user 13 14 scanf("%d %d", &number1, &number2); // read two integers 15 16 if (number1 == number2) { 17 printf("%d is equal to %d\n", number1, number2); 18 } // end if # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 28 Operators Various Operators & If Statement 19 20 if (number1 != number2) { 21 printf("%d is not equal to %d\n", number1, number2); 22 } // end if 23 24 if (number1 < number2) { 25 printf("%d is less than %d\n", number1, number2); 26 } // end if 27 # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 29 Operators Various Operators & If Statement 28 if (number1 > number2) { 29 printf("%d is greater than %d\n", number1, number2); 30 } // end if 31 32 if (number1 = number2) { 37 printf("%d is greater than or equal to %d\n", number1, number2); 38 } // end if 39 } // end function main # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 31 Token Tokens are the basic lexical building blocks of source code. Tokens are one or more symbols understood by the compiler that help it interpret the program code. Characters are combined into tokens according to the rules of the programming language. The compiler checks the tokens so that they can be formed into legal strings according to the syntax of the language. There are five classes of tokens: identifiers, reserved words, operators, separators, and constants # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 32 Token Identifier Identifier or name is a sequence of characters created by the programmer to identify or name a specific object. An identifier is used for any variable, function, data definition, labels. In C language, an identifier is a combination of alphanumeric characters int var1, var2; float Avg; function sum(); # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 33 Token Identifier Rules: Identifiers are case-sensitive, so uppercase and lowercase letters are considered different characters. The first character of an identifier must be a nondigit, such as an underscore or an uppercase or lowercase letter. Commas and blank spaces are not permitted when naming an identifier. Keywords in C cannot be used as identifiers. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 34 Token Keywords Keywords are the vocabulary of C. Because they are special to C, one cannot use them for variable names. There are 32 words defined as keywords in C. These have predefined uses and cannot be used for any other purpose in a C program. They are used by the compiler to compile the program. They are always written in lower- case letters. These are explicitly reserved words that have a strict meaning as individual tokens to the compiler. They cannot be redefined or used in other contexts. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 35 Token Keywords Use of variable names with the same name as any of the keywords will cause a compiler error. # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 36 Token Constant The constants in C are the read-only variables whose values cannot be modified once they are declared in the C program. The type of constant can be an integer constant, a floating pointer constant, a string constant, or a character constant. In C language, the const keyword is used to define the constants. Syntax to define constant: const data_type var_name = value; const int a = 3.75 # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 37 Token Separator These are tokens used to separate other tokens. Two common kinds of separators are indicators of an end of an instruction and separators used for grouping. ; { } # Education with values Department of Computer Engineering | School of Polytechnic & Skill Development 38 Token Separator Consider the following piece of code if(x