Programming quiz 2.pdf
Document Details
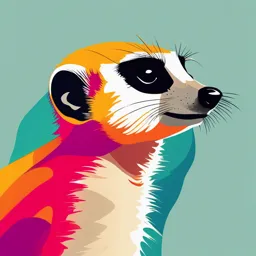
Uploaded by ChampionTulip3548
Tags
Full Transcript
Summary ( Strings and Text Files) A string is a sequence of zero or more characters. The len function returns the number of characters in its string argument. Each character occupies a position in the string. The positions range from 0 to the length of the string minus 1. A str...
Summary ( Strings and Text Files) A string is a sequence of zero or more characters. The len function returns the number of characters in its string argument. Each character occupies a position in the string. The positions range from 0 to the length of the string minus 1. A string is an immutable data structure. Its contents can be accessed, but its structure cannot be modified. The subscript operator [] can be used to access a character at a given position in a string. The operand or index inside the subscript operator must be an integer expression whose value is less than the string’s length. A negative index can be used to access a character at or near the end of the string, starting with −1. A subscript or index operator can also be used for slicing—to fetch a substring from a string. When the subscript has the form [:] , the substring contains the characters from the start position to the end of the string. When the form is [:] , the positions range from the first one to end - 1. When the form is [:] , the positions range from start to end - 1. Basically: Slicing Var[3:4] Everything after 3 (including 3) and everything before 4 (excluding 4) The in operator is used to detect the presence or absence of a substring in a string. Its usage is in. A method is an operation that is used with an object. A method can expect arguments and return a value. The string type includes many useful methods for use with string objects. A text file is a software object that allows a program to transfer data to and from permanent storage on disk, CDs, or flash memory. A file object is used to open a connection to a text file for input or output. The file method write is used to output a string to a text file. The file method read inputs the entire contents of a text file as a single string. The file method readline inputs a line of text from a text file as a string. The for loop treats an input file as a sequence of lines. On each pass through the loop, the loop’s variable is bound to a line of text read from the file. Method and Object Examples 1. String Object Object: A string (e.g., "Hello, World!"). Methods: ○.upper(): Converts the string to uppercase. ○.lower(): Converts the string to lowercase. ○.strip(): Removes whitespace from the beginning and end. Example: text = " Hello, World! " print(text.upper()) # Output: " HELLO, WORLD! " print(text.strip()) # Output: "Hello, World!" 2. List Object Object: A list (e.g., [1, 2, 3, 4, 5]). Methods: ○.append(): Adds an element to the end of the list. ○.remove(): Removes the first occurrence of a specified value. ○.sort(): Sorts the list in ascending order. numbers = [3, 1, 4, 2] numbers.append(5) # Adds 5 to the end numbers.sort() # Sorts the list print(numbers) # Output: [1, 2, 3, 4, 5] Mutable = changeable Immutable - not changeable Review Questions For Questions 1, 2, 3, 4, 5, and 6, assume that the variable data refers to the string "No way!". Data = “No way!” 1. The expression len(data) evaluates to 7 2. The expression data evaluates to ‘O’ 3. The expression data[-1] evaluates to ! 4. The expression data[3:6] evaluates to Way 5. The expression data.replace("No", "Yes") evaluates to Yes way! 6. The expression data.find("way!") evaluates to 3 7. A Caesar cipher locates the coded text of a plaintext character a given distance to the left or to the right in the sequence of characters 8. The binary number 111 represents the decimal integer 7 Solution: (1x2^2)+(1x2^1)+(1x2^0) 4+2+1=7 (The binary number 111 equals 4 + 2 + 1, which is 7 in decimal.) 9. Which of the following binary numbers represents the decimal integer value 8? Solution: Divide everything by 2 then combine the remainders 8/2 = 4 remainder 0 4/2 = 2 remainder 0 2/2 = 1 remainder 0 ½ = 0 remainder 1 (The binary number 1000 equals 8 in decimal.) 10. Which file method is used to read the entire contents of a file in a single operation? read Binary numbers are a fundamental concept in computing and digital electronics, representing values using only two symbols: 0 and 1. Here’s a simple breakdown of how binary numbers work: 1. Base System Binary is Base 2: The binary system is a base-2 numeral system. This means each digit in a binary number represents a power of 2, as opposed to the decimal system, which is base 10. 2. Place Values Each digit (bit) in a binary number has a place value that is a power of 2, starting from the rightmost bit: ○ The rightmost bit represents 202^020 (which is 1). ○ The next bit to the left represents 212^121 (which is 2). ○ The next bit to the left represents 222^222 (which is 4). ○ The next bit to the left represents 232^323 (which is 8). ○ And so on. 3. Example of Binary Representation For example, consider the binary number 1011: 1×2^3=81 \times 2^3 = 81×23=8 (the leftmost bit) 0×2^2=00 \times 2^2 = 00×22=0 1×2^1=21 \times 2^1 = 21×21=2 1×2^0=11 \times 2^0 = 11×20=1 So, adding these together: 8+0+2+1=118 + 0 + 2 + 1 = 118+0+2+1=11 Thus, the binary number 1011 is equivalent to the decimal number 11. 4. Counting in Binary Counting in binary is similar to counting in decimal, but you only use the digits 0 and 1: ○ Decimal: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 ○ Binary: 0, 1, 10 (2), 11 (3), 100 (4), 101 (5), 110 (6), 111 (7), 1000 (8),... 5. Converting Binary to Decimal To convert a binary number to decimal, you can use the place value method as described above, or you can multiply each bit by its corresponding power of 2 and sum the results. 6. Converting Decimal to Binary To convert a decimal number to binary: 1. Divide the number by 2. 2. Record the remainder (0 or 1). 3. Continue dividing the quotient by 2 until it reaches 0. 4. The binary number is the remainders read in reverse order. Example: Convert 13 to binary: 13 divided by 2 is 6, remainder 1. 6 divided by 2 is 3, remainder 0. 3 divided by 2 is 1, remainder 1. 1 divided by 2 is 0, remainder 1. Reading the remainders from bottom to top gives 1101. In a binary number, the position of each digit (bit) is determined by its distance from the rightmost side of the number. Here’s how you can identify the positions: Counting Positions 1. Start from the Right: The rightmost digit is considered to be in position 0. 2. Move Left: Each digit to the left increases the position by 1. Strings String Descriptio Exampl Outpu Method n e t str.lower() Converts all "Hello".lower() hello characters to lowercase. str.upper Converts all "Hello".upper() HELLO () characters to uppercase. str.strip Removes " Hello ".strip() Hello () leading and trailing whitespace. str.split Splits a "a,b,c".split(",") ['a', 'b', () string into a 'c'] list based on a delimiter. str.join Joins a list of ",".join(['a', 'b', 'c']) a,b,c () strings into a single string. str.replace Replaces "Hello".replace("e", "a") Hallo () a substring with another substring. str.find Returns the index of "Hello".find("e") 1 () the first occurrence of a substring. str.count() Returns the "Hello".count("l") 2 number of occurrences of a substring. str.count(s substring: The “Hello Hello Hello” 3 ubstring, substring you want my_string.count("Hello", 0, start, end) to count in the 30) print(count_range) string. start (optional): The index at which to start searching in the string (inclusive). end (optional): The index at which to stop searching in the string (exclusive) str.startsw Checks if a string "Hello".startswith("He") Tru ith() starts with a e specified prefix. str.endswi Checks if a string "Hello".endswith("lo") Tru th() ends with a e specified suffix. Text Files Python provides built-in functions for creating, writing, and reading files. Two types of files can be handled in Python, normal text files and binary files (written in binary language, 0s, and 1s). Text files: In this type of file, Each line of text is terminated with a special character called EOL (End of Line), which is the new line character (‘\n’) in Python by default. Binary files: In this type of file, there is no terminator for a line, and the data is stored after converting it into machine-understandable binary language. 6 access modes in Python: Read Only (‘r’): Open text file for reading. The handle is positioned at the beginning of the file. If the file does not exist, raises the I/O error. This is also the default mode in which a file is opened. Read and Write (‘r+’): Open the file for reading and writing. The handle is positioned at the beginning of the file. Raises I/O error if the file does not exist. Write Only (‘w’): Open the file for writing. For the existing files, the data is truncated and over-written. The handle is positioned at the beginning of the file. Creates the file if the file does not exist. Write and Read (‘w+’): Open the file for reading and writing. For an existing file, data is truncated and over-written. The handle is positioned at the beginning of the file. Append Only (‘a’): Open the file for writing. The file is created if it does not exist. The handle is positioned at the end of the file. The data being written will be inserted at the end, after the existing data. Append and Read (‘a+’): Open the file for reading and writing. The file is created if it does not exist. The handle is positioned at the end of the file. The data being written will be inserted at the end, after the existing data. Access Mode Behavior if a file does not exist Reading Modes r, r+ Raises a FileNotFoundError. Cannot proceed without the file. Writing modes w,w+ If the file does not exist, a new file is created. If it does exist, the existing file is truncated, erasing its content. Appending modes a,a+ If the file does not exist, a new file is created. If it does exist, the existing file is opened for appending, allowing new content to be added without erasing existing content. Opening a Text File in Python It is done using the open() function. No module is required to be imported for this function. File_object = open(r"File_Name","Access_Mode") Note: The r is placed before the filename to prevent the characters in the filename string to be treated as special characters. Closing a Text File in Python close() function closes the file and frees the memory space acquired by that file file1 = open("MyFile.txt","a") file1.close() Writing to a Python Text File Using write() write(): Inserts the string str1 in a single line in the text file. File_object.write(str1) Reading From a File Using read() read(): Returns the read bytes in form of a string. Reads n bytes, if no n specified, reads the entire file. File_object.read([n]) The os module in Python provides a way to interact with the operating system and perform operating system-level tasks. It gives access to functionalities that are dependent on the operating system, such as file and directory manipulation, environment variables, and process management. Here’s a breakdown of some of the most common functionalities of the os module, along with simple examples: 1. File and Directory Management os.getcwd(): Returns the current working directory. Example: import os print(os.getcwd()) # Outputs the current working directory path os.mkdir('new_folder') # Creates a directory named 'new_folder' os.rmdir('new_folder') # Removes the directory named 'new_folder' os.remove('file.txt') # Deletes the file named 'file.txt' os.rename('old_name.txt', 'new_name.txt') # Renames a file Summary (Lists and Dictionaries) A list is a sequence of zero or more elements. The elements can be of any type. The len function returns the number of elements in its list argument. Each element occupies a position in the list. The positions range from 0 to the length of the list minus 1. Lists can be manipulated with many of the operators used with strings, such as the subscript/index, concatenation, comparison, and in operators. Slicing a list returns a sublist. The list is a mutable data structure. An element can be replaced with a new element, added to the list, or removed from the list. Replacement uses the subscript operator. The list type includes several methods for insertion and removal of elements. The method index returns the position of a target element in a list. If the element is not in the list, an error is raised. The elements of a list can be arranged in ascending order by calling the sort method. Mutator methods are called to change the state of an object. These methods usually return the value None. This value is automatically returned by any function or method that does not have a return statement. Assignment of one variable to another variable causes both variables to refer to the same data object. When two or more variables refer to the same data object, they are aliases. When that data value is a mutable object such as a list, side effects can occur. A side effect is an unexpected change to the contents of a data object. To prevent side effects, avoid aliasing by assigning a copy of the original data object to the new variable. alias Variable1 = variable2 A tuple is quite similar to a list, but it has an immutable structure. A function definition consists of a header and a body. The header contains the function’s name and a parenthesized list of argument names. The body consists of a set of statements. The return statement returns a value from a function definition. The number and positions of arguments in a function call must match the number and positions of required parameters specified in the function’s definition. A dictionary associates a set of keys with values. Dictionaries organize data by content rather than position. The subscript/index operator is used to add a new key/value pair to a dictionary or to replace a value associated with an existing key. The dict type includes methods to access and remove data in a dictionary. Summary: Access Data: get(key, default) Remove Data: pop(key, default), popitem(), clear() Modify Data: update(other_dict), or assign directly with [] The for loop can traverse the keys of a dictionary. The methods keys and values return access to a dictionary’s keys and values, respectively. Bottom-up testing of a program begins by testing its lower-level functions and then testing the functions that depend on those lower-level functions. Top-down testing begins by testing the program’s main function and then testing the functions on which the main function depends. These lower-level functions are initially defined to return their names. Review Questions For Questions 1, 2, 3, 4, 5, and 6, assume that the variable data refers to the list [10, 20, 30]. 1. The expression data evaluates to a. 10 b. 20 c. 30 d. 0 2. The expression data[1:3] evaluates to a. [10, 20, 30] b. [20, 30] c. [10, 30] d. 3. The expression data.index(20) evaluates to a. 1 b. 2 c. True d. False 4. The expression data + [40, 50] evaluates to a. [10, 60, 80] b. [10, 20, 30, 40, 50] c. [50, 70] d. 5. After the statement data = 5, data evaluates to a. [5, 20, 30] b. [10, 5, 30] c. 5 d. [10, 20, 5] 6. After the statement data.insert(1, 15) , the original data evaluates to a. [15, 10, 20, 30] b. [10, 15, 30] c. [10, 15, 20, 30] d. [10, 20, 30, 15] For Questions 7, 8, and 9, assume that the variable info refers to the dictionary {"name":"Sandy", "age":17}. 7. The expression list(info.keys()) evaluates to a. ("name", "age") b. ["name", "age"] c. ("Sandy", 17) d. (17, "Sandy") 8. The expression info.get("hobbies", None) evaluates to a. "knitting" b. None c. 1000 d. 2000 9. The method to remove an entry from a dictionary is named a. delete b. pop c. remove d. insert 10. Which of the following are immutable data structures? a. dictionaries and lists b. strings and tuples c. integers and floats d. menus and buttons Dictionaries Keys-values A tuple in Python is an immutable sequence of elements, meaning that once a tuple is created, you cannot change, add, or remove elements from it. Tuples are often used to group related data together and are similar to lists, but with a key difference—tuples cannot be modified after creation. Key Characteristics of Tuples: 1. Ordered: The elements in a tuple have a defined order and can be accessed by index (like a list). 2. Immutable: Once created, you cannot change the values inside the tuple (unlike lists, which are mutable). 3. Can contain different types: A tuple can hold elements of different data types (e.g., strings, integers, floats, etc.). 4. Uses parentheses: Tuples are defined using parentheses (). # Creating a tuple person = ("Alice", 30, "Engineer") # Accessing elements by index print(person) # Output: Alice print(person) # Output: 30 print(person) # Output: Engineer Function def add(a, b): # Header with two parameters return a + b # Body that returns the sum of a and b result = add(3, 4) # Calling the function and storing the result print(result) # Output: 7 Arguments are the values you provide to a function when you call it. Types of Arguments 1. Positional Arguments: ○ These are the most common arguments. They are assigned to function parameters based on their position in the call. ○ Example: def add(a, b): # a and b are parameters return a + b result = add(3, 5) # 3 and 5 are arguments print(result) # Output: 8 Practice Quiz 1. The main function usually expects no arguments and returns no value. True The main function in Python CAN be defined to expect arguments and return a value, but it usually does not. 2. The index of the first item in a list is 1. False 3. A difference between functions and methods is that methods cannot return values. False 4. A module behaves like a function, but has a slightly different syntax. False Module example - import math, import random, import os Syntax: A module is imported using the import statement, while functions are defined using the def keyword. A module does not act like a function; rather, it is a container for functions and other code. 5. An Identity function usually tests its argument for the presence or absence of some property. False An identity function is a function that always returns the same value that was used as its argument. In other words, the output of the function is the same as input. Example: def identity(x): return x # Example usage result = identity(5) print(result) # Output: 5 6. In scripts that include the definitions of several cooperating functions, it is often useful to define a special function named init that serves as the entry point for the script. False While it can be useful to define a special function (like init or main) as an entry point in scripts, it is not a requirement, especially in Python. Scripts can run without such a dedicated entry function, making the statement misleading. While it's common practice to have an entry point in a script (often a main function), the function is typically named main, not init. The main function is where execution starts in many programming languages, including Python, and it can call other functions as needed. The name init is more commonly associated with initialization functions in some programming paradigms but is not standard as an entry point for scripts. if __name__ == "__main__": main() 7. When used with strings, the left operand of Python's in operator is a target substring and the right operand is the string to be searched. True In the context of the in operator in Python, the substring is the left operand when it appears before the in keyword. substring = "keep" # left operand string = "You keep me running around." # right operand if substring in string: print("Found!") If left_operand in right_operand: In programming, an operand is a value or variable on which an operator acts. The terms left operand and right operand refer to the positions of the operands in a binary operation, which is an operation that involves two operands. Left Operand: The left operand is the operand that appears to the left of the operator in an expression. For example, in the expression A + B, A is the left operand. Right Operand: 8. The right operand is the operand that appears to the right of the operator in an expression. 9. In the same expression A + B, B is the right operand. 10. The median of a list of values is the value that occurs most frequently. False The median of a list of values is the middle value when the values are sorted in order. 11. In a dictionary, a -> separates a key and its value. False In a dictionary, a colon : (not an arrow ->) separates a key from its value. Example: my_dict = { 'key1': 'value1', 'key2': 'value2', } 12. When using the open function to open a file for output (i.e., using 'w' as the mode string), if the file does not exist, Python raises an error. False When you use the open function in Python with the mode 'w' (write mode) to open a file: If the file does not exist, Python will create a new file. If the file does exist, it will be truncated (emptied) before writing to it. 13. All data output to or input from a text file must be strings. True When working with text files in Python, all data input from or output to the file must be in string format. Even if you are writing or reading numbers or other data types, you need to convert them to or from strings. 14. The index of the last item in a list is the length of the list. False 15. Lists of integers can be built using the range function. True 16. A data structure is a compound unit that consists of several smaller pieces of data. True Examples of Data Structures: List: A collection of ordered items, e.g., [1, 2, 3, 4]. Dictionary: A collection of key-value pairs, e.g., {"name": "Alice", "age": 25}. Tuple: An ordered, immutable collection of items, e.g., (1, 2, 3). Set: A collection of unique items, e.g., {1, 2, 3}. 17. After input is finished, another call to read returns an empty string to indicate that the end of the file has been reached. True When you read from a file in Python, you can use methods like read(), readline(), or readlines(). After reading all the content in a file, if you make another call to the read() method, it will return an empty string (''). This indicates that there is no more data to read, signifying that you've reached the end of the file (EOF). 18. Although a list's elements are always ordered by position, it is possible to impose a natural ordering on them as well. True.sort 19. To prevent aliasing, a new object can be created and the contents of the original can be copied to it. True Aliasing occurs when two variables reference the same object in memory. Changes made to one variable will affect the other because they point to the same object. To prevent this, you can create a new object and copy the data from the original object into it. This way, the new object will have its own memory space and will not be affected by changes made to the original object. 20. Many applications now use data mangling to protect information transmitted on networks. False In summary, while Python allows for various methods of protecting data, "data mangling" as understood in the context of class attributes is not related to network data protection. True data protection involves techniques like encryption and secure transmission protocols, which are the accepted practices in Python for safeguarding data. 21. The string method isalphabetic returns True if the string contains only letters or False otherwise. False the method name is slightly incorrect. The correct method name in Python is isalpha. 22. When replacing an element in a list, the subscript/index is used to reference the target of the assignment statement, which is not the list but an element's position within it. True In programming, when you replace an element in a list, you use an index (or subscript) to specify the position of the element you want to change. This index refers to the location of the element within the list, not the list itself. 23. Each item in a list has a unique index that specifies its position. True 24. Using a text editor such as Notepad or TextEdit, you can create, view, and save data in a text file. True 25. When using the open function to open a file for input (i.e., using 'r' as the mode string), if the file does not exist, Python raises an error. True 26. A Python dictionary is written as a sequence of key/value pairs separated by commas. These pairs are sometimes called indexes. False these are called key-value pairs 27. The remove file system function is included in the os.path module. False The remove function is not included in the os.path module; instead, it is part of the os module itself. 28. A Caesar cipher uses a plaintext character to compute two or more encrypted characters, and each encrypted character is computed using two or more plaintext characters. False 29. A function can be defined in a Python shell, but it is more convenient to define it in an IDLE window, where it can be saved to a file. True 30. If pop is used with just one argument and this key is absent from the dictionary, Python raises an error. True In Python, when using the pop() method with just one argument (the key), if that key is not present in the dictionary, Python will raise a KeyError. my_dict = {"a": 1, "b": 2} # Trying to pop a key that exists value = my_dict.pop("a") # value will be 1 print(my_dict) # Output: {'b': 2} # Trying to pop a key that does not exist value = my_dict.pop("c") # Raises KeyError: 'c' 31. The string is a mutable data structure. False 32. A method is always called with a given data value called an object, which is placed before the method name in the call. True 33. Anytime you foresee using a list whose structure will not change, you can, and should, use a tuple instead. True Aspect Tuple List Mutability Immutable Mutable (can change (cannot change elements) elements) Performance Faster (since it's Slightly slower (because it's fixed in size) flexible) Use Case Use when data Use when you need to won’t change modify data Safety Safer (prevents More flexible, but prone to accidental changes changes) Examples Coordinates, To-do lists, dynamic data, fixed settings, collections constants In short, if the structure or data won’t change, using a tuple is more efficient and safer than using a list. 34. The list method ascending mutates a list by arranging its elements in ascending order. False In Python, the method to sort a list in ascending order is sort(), and it mutates the original list. Example: my_list = [5, 3, 8, 1] my_list.sort() # This sorts the list in ascending order print(my_list) # Output: [1, 3, 5, 8] 35. You add a new key/value pair to a dictionary by using the subscript operator []. True 36. The decimal number system is also called the base ten number system. True Decimal System: Base ten (10). It uses ten digits: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9. Binary System: Base two (2). It uses only two digits: 0 and 1. 37. Some applications extract portions of strings called characters. False When discussing strings in programming, characters are the individual units that make up the string (e.g., letters, digits, symbols). However, when you extract portions of strings, what you're actually extracting is substrings, not characters. A substring is a contiguous sequence of characters within a string. 38. The two digits in the base two number system are 0 and 1. True 39. The == operator returns True if the variables are aliases for the same object. Unfortunately, == returns False if the contents of two different objects are the same. False The == operator checks for equality of values (contents), and it will return True if two different objects have the same content. The is operator checks for identity (whether two variables point to the same object), and it will return True only if they are the same object. 40. A list is a sequence of data values called indexes. False A list is a sequence of data values, but the term used to describe the position of these data values within the list is indexes. The individual data values within a list are called elements, not indexes. Example: my_list = [10, 20, 30, 40] # Here, the elements are 10, 20, 30, and 40. # Their corresponding indexes are 0, 1, 2, and 3. 41. A list is immutable False 42. The absolute value of a digit is determined by raising the base of the system to the power specified by the position (baseposition). False Summary The absolute value of a digit itself is simply the digit's non-negative value. The overall value of a digit in a number is determined by multiplying it by the base raised to the power of its position. Positional Value (Overall Value): In a positional numeral system (like decimal or binary), each digit's value is determined by multiplying the digit by the base raised to the power of its position. For example, in the decimal number 345: 3×10^2+4×10^1+5×10^0=300+40+5=345 Here, the base (10) is raised to the power of the position (2 for the hundreds place, 1 for the tens place, 0 for the units place) and multiplied by the digit. 43. The print function strips the quotation marks from a string, but it does not alter the look of a list. True 44. Data can be output to a text file using a(n) output object. False 45. A lookup table is a type of dictionary. True 46. The definition of the main function and the other function definitions can appear in no particular order in the script, as long as main is called at the very end of the script. True 47. The file method write expects a single string as an argument. True 48. In Python, all data values are objects. True In Python, all data values, including integers, floats, strings, lists, dictionaries, and even functions, are treated as objects. This means that every value has an associated type and can be manipulated using methods and attributes. 49. The string method isnumeric returns True if the string contains only digits or False otherwise. False 50. The is operator has no way of distinguishing between object identity and structural equivalence. False The is operator in Python is specifically designed to check for object identity, meaning it determines whether two variables point to the same object in memory. It does not assess structural equivalence (the content of the objects) but solely checks if both references refer to the same object. # Two lists with the same content list1 = [1, 2, 3] list2 = [1, 2, 3] # Check if they are the same object print(list1 is list2) # Output: False (different objects in memory) # Check if they have the same content (structural equivalence) print(list1 == list2) # Output: True (content is the same) Summary The is operator checks for object identity (whether two variables refer to the same object). The == operator checks for structural equivalence (whether the values or contents of the objects are the same). 51. The exists file system function is included in the os.path module. True 52. A binary number is sometimes referred to as a string of bits or a bit string. True 1. What else is included in the os path module A Caesar cipher is a simple encryption technique used to encode messages by shifting the letters of the alphabet by a fixed number of places. It’s one of the earliest and most well-known methods of encryption. How It Works: 1. Shift: Choose a number to represent the shift (also known as the key). For example, a shift of 3 means that each letter in the plaintext will be replaced by the letter that is three places down the alphabet. 2. Encryption: ○ For example, with a shift of 3: A→D B→E C→F... X→A Y→B Z→C 3. Decryption: To decode the message, you would shift the letters back by the same number. Example: Plaintext: HELLO Shift: 3 Ciphertext: KHOOR Encryption Steps: H → K (H shifted 3 positions forward) E→H L→O L→O O→R Limitations: The Caesar cipher is easy to break, especially with frequency analysis (since it only has 25 possible keys for the English alphabet). Modern cryptography uses much more complex algorithms for secure communication. Summary: The Caesar cipher is a straightforward way to encrypt messages by shifting letters. It's mainly of historical interest today but serves as a useful introduction to the concepts of encryption and decryption.