PPS Important Questions for Mid-1 PDF
Document Details
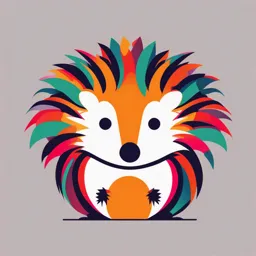
Uploaded by HealthfulSerpentine4576
PPS
Tags
Summary
This PDF file contains a set of important questions related to C programming, suitable for a mid-term assessment. The questions cover various topics, including computer languages, number systems, operators, datatypes, control statements, arrays, and matrix operations. This document is likely to be beneficial for students preparing for their mid-term exams.
Full Transcript
## C Language Programming Problems 1. **Computer Languages and Number Systems** * Explain different types of computer languages. * Explain different types of number systems with examples. 2. **Operators in C language** * List and explain different types of operators in the C language. 3....
## C Language Programming Problems 1. **Computer Languages and Number Systems** * Explain different types of computer languages. * Explain different types of number systems with examples. 2. **Operators in C language** * List and explain different types of operators in the C language. 3. **Datatypes in C language** * What is datatype? * Explain different datatypes in the C language, including name, size, range, and format specifier. 4. **Sum of Individual Digits and Syntax** * Write a C program to find the sum of individual digits. * Write the syntax for the following with examples: * `if`, `while`, and `do-while`. 5. **Factorial and Switch Statements** * Write a C program to print the factorial of a given number. * Write the syntax for the following with examples: * `switch`, `nested if`. 6. **Finding the Biggest of Three Numbers** * Write an algorithm, draw the flowchart, and write a C program to find the biggest of three numbers. 7. **Reverse of a Given Number and Differences between `while` and `do-while`** * Write a C program to print the reverse of a given number. * Write any five differences between the `while` and `do-while` loops. 8. **Fibonacci Series and Even or Odd Check** * Write a C program to generate the Fibonacci series between 0 and N, where N is supplied by the user. * Write a C program to check if a given number is even or odd. 9. **`goto`, `continue`, Checking Vowel** * Explain the `goto`, `continue` statements in C language with examples. * Write a C program to check if a given character is a vowel or not. 10. **Control Statements Explained** * Explain all control statements in C language with examples: * Selection statements * Looping statements * Jumping statements 11. **Arrays** * What is an array? * Write syntax with examples for 1D, 2D, and multidimensional arrays, including declaration and initialization. * Write a C program to find the largest and smallest element in the array. 12. **Matrix Operations** * Write a C program to perform addition of two matrices. * Write a C program to perform multiplication of two matrices.