C Programming Pointers PDF
Document Details
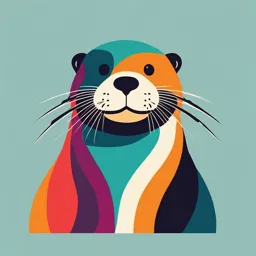
Uploaded by LuxuryAbundance
Algonquin College
ALGONQUIN COLLEGE
Tags
Summary
This document provides an introduction to pointers in C programming. It covers memory allocation functions like malloc, calloc, realloc, and free. It discusses the concept of pointers, how they store addresses, and their use in modifying variable values and passing large data structures.
Full Transcript
CST8234 – C Programming Pointers Learning Outcomes ❖ Memory Allocation - Functions ❖ Understanding Pointers ❖ Pointer Arithmetic ❖ Function Parameter Passing ❖ Multidimensional Arrays 2 Memory Allocation Functions (1/4) The standard library pro...
CST8234 – C Programming Pointers Learning Outcomes ❖ Memory Allocation - Functions ❖ Understanding Pointers ❖ Pointer Arithmetic ❖ Function Parameter Passing ❖ Multidimensional Arrays 2 Memory Allocation Functions (1/4) The standard library provides several functions for this purpose. ❑ malloc(): Allocates a specified number of bytes of memory and returns a pointer to the beginning of the block. #include void *malloc(size_t size); Parameters: size – the number of bytes of allocate Returns: A pointer to the allocated memory, or NULL if the allocation fails. int *arr = (int *)malloc(5 * sizeof(int)); if (arr == NULL) { } 3 Memory Allocation Functions (2/4) ❑ calloc(): Allocates a specified number of bytes of memory and returns a pointer to the beginning of the block. It also initializes all memory elements to a value ‘zero’. #include void *calloc(size_t num, size_t size); Parameters: num - number of elements to allocate and size – size of each element Returns: A pointer to the allocated memory, or NULL if the allocation fails. int *arr = (int *)calloc(5, sizeof(int)); if (arr == NULL) { } 4 Memory Allocation Functions (3/4) ❑ realloc(): Changes the size of previously allocated memory block. It can also be used to extend or reduce the size of the memory block. #include void *realloc(void *ptr, size_t size); Parameters: ptr - pointer to the previously allocated memory block and size – new size in bytes Returns: A pointer to the newly allocated memory, which may be the same as ptr, or NULL if the reallocation fails. arr = (int *)realloc(arr, 10 * sizeof(int)); if (arr == NULL) { } 5 Memory Allocation Functions (4/4) ❑ free(): Deallocates memory that was previously allocated. It is important to free any dynamically allocated memory to avoid memory leaks. #include void free(void *ptr); Parameters: ptr - pointer to the memory to be freed. Returns: Nothing. arr = (int *)realloc(arr, 10 * sizeof(int)); if (arr == NULL) { } 6 Pointers ❑ C pointers are a powerful feature that allows you to directly manipulate memory addresses. ❑ Definition: A pointer is a variable that stores the address of another variable. The type of pointer corresponds to the type of variable it points to (e.g., char*, int*) ❑ Declaration: To declare a pointer, you use the asterisk (*). int *ptr; ❑ Initialization: You can initialize a pointer to point to a variable: int var = 5; int *ptr = &var; ❑ Dereferencing: You can access the value stored at the address the pointer points to by dereferencing it with the asterisk: int value = *ptr; 7 Pointers int value = 5; int* address = &value; printf("the value is %d\n", value);