Design Patterns in Web Application Development PDF
Document Details
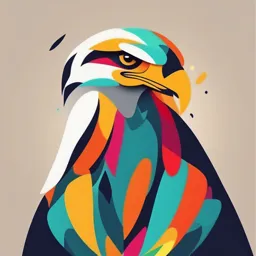
Uploaded by NiceQuantum
Technical University of Mombasa
Tags
Summary
This document is an introduction to design patterns in web application development. It explores various design patterns, their benefits, and how they improve scalability and maintainability. The document emphasizes the importance of design patterns for web development, with examples like MVC and others.
Full Transcript
Module 2: Title Design Patterns in Web Application Development 1 Design Patterns in Web Application Development Enhancing Scalability, Maintainability, and Security 2 Module 2:Overview Course Overview: Th...
Module 2: Title Design Patterns in Web Application Development 1 Design Patterns in Web Application Development Enhancing Scalability, Maintainability, and Security 2 Module 2:Overview Course Overview: This module introduces common software design patterns used in web development to ensure scalability, maintainability, and reusability of code security. 3 Learning Outcomes By the end of this module, the students should be able to: Identify common design patterns in web application development. Implement the MVC pattern in a web application. Evaluate the benefits of using design patterns in terms of scalability and maintenance. Adopt best practices in applying design patterns to web projects 4 Importance of Design Patterns in Web Development Help streamline and organize code. Improve efficiency, maintainability, and scalability. Provide proven solutions to common problems. Promote code reusability and clean architecture 5 Overview of Common Design Patterns Model-View-Controller (MVC) Factory Pattern Singleton Pattern Observer Pattern Decorator Pattern And more... Note: Each pattern offers a unique benefit to web development. 6 Model-View-Controller (MVC) Heading: MVC Pattern Model: Manages data and business logic. View: Handles display and user interaction. Controller: Manages user input, updates model and view. Image/Diagram: Simple MVC architecture 7 Factory Pattern Heading: Factory Pattern Provides an interface for creating objects without specifying the exact class. Used when systems require flexible object creation. Example: Object creation in polymorphic systems. Diagram: Factory method flow. 8 Singleton Pattern Heading: Singleton Pattern Ensures a class has only one instance. Global access point for services like logging or database connections. Use Case: Database connections in web apps. Visual: Singleton pattern diagram. 9 Observer Pattern Heading: Observer Pattern Defines a one-to-many dependency. When one object changes state, dependents are notified automatically. Use Case: Event-driven architectures (e.g., notifications, real-time data). Image: Observer pattern structure 10 Decorator Pattern Heading: Decorator Pattern Dynamically adds behavior to objects without modifying their original code. Used to enhance functionality in a flexible way. Example: Adding middleware in frameworks like Express.js. Visual: Decorator pattern in action 11 Repository Pattern Heading: Repository Pattern Abstracts data access layer to simplify database interactions. Decouples business logic from data access logic. Example: Used in ORM frameworks (e.g., Eloquent in Laravel). Diagram: Repository pattern flow. 12 Other Patterns Heading: Additional Design Patterns Service Locator Pattern Front Controller Pattern Proxy Pattern Command Pattern Strategy Pattern Facade Pattern Adapter Pattern 13 Other Patterns Heading: Additional Design Patterns Service Locator Pattern Front Controller Pattern Proxy Pattern Command Pattern Strategy Pattern Facade Pattern Adapter Pattern 14 Conclusion Heading: Summary of Design Patterns in Web Applications Improve scalability, maintainability, and security. Foster cleaner, reusable, and more flexible code. Essential in modern web development frameworks. Closing Remark: Choose the right pattern based on application needs 15 Implementing the MVC Pattern in a Web Application Using Laravel o A Simple Blog Application Example 16 Introduction to MVC in Laravel MVC stands for Model-View-Controller. It is a design pattern that separates an application into three main components: Model: Handles the data and business logic. View: Manages the user interface and presentation. Controller: Connects the Model and the View, handling user input and interactions. 17 Model - Defining Data Structure Models represent data and interact with the database. Laravel models are created using the php artisan make:model command. Example model: Post for a blog post. Fields: title, content, and timestamps. · Code Snippet: 18 Database Migration Use migrations to create and modify database tables. Example: Create posts table with fields id, title, content, and timestamps. Migration file is created using php artisan make:migration. · Code Snippet: 19 View - Displaying Data Creating the View with Blade Templates Views are responsible for displaying data to the user. Laravel uses Blade templating engine to create views. Example: A form to create a new blog post and a page to display posts. · Example HTML: 20 Controller - Handling Requests Creating the PostController Controllers handle the logic of the application. Example: PostController manages CRUD operations (Create, Read, Update, Delete) for blog posts. Functions: index() to list posts. create() to display the form. store() to save the new post to the database. · Code Snippet: 21 Routing - Connecting URLs to Controllers Defining Routes for Blog Posts Routes connect user requests (URLs) to specific controller actions. Example routes: GET /posts → Displays all posts. GET /posts/create → Displays the post creation form. POST /posts → Submits a new post. · Code Snippet: 22 Final Application Workflow Application Workflow 1. User requests the post creation form → GET /posts/create. 2. User submits the form → POST /posts. 3. Controller validates the data and saves it to the database. 4. User is redirected to the post listing page → GET /posts. 23 Conclusion Key Takeaways Laravel’s MVC pattern ensures a clean separation of concerns. Model: Handles data. View: Displays user interface. Controller: Manages logic and user interaction. By using migrations, Blade templates, and controllers, we can build scalable and maintainable web applications. 24 Benefits of Using Design Patterns: Scalability and Maintenance How design patterns improve scalability and streamline maintenance in web development. 25 Introduction to Design Patterns Title: What are Design Patterns? Design patterns are proven solutions to common software design problems. Help developers write scalable, maintainable, and reusable code. Commonly used in web application frameworks like MVC, Factory, Singleton, etc. 26 Overview of Scalability & Maintenance Scalability and Maintenance in Web Applications Scalability: The ability to handle increased workloads by adding resources. Maintenance: Keeping the software functional, easy to update, and bug-free over time. Design patterns support both scalability and maintainability 27 Benefits of Design Patterns for Scalability Design Patterns Boost Scalability Modular and Reusable Code: Enables individual components to be scaled independently. Efficient Resource Management: Singleton, Proxy patterns control object creation, reduce memory overhead. Distributed Systems: Observer, Factory patterns decouple components, promoting distributed architecture. Example: The MVC pattern helps separate concerns, making it easier to add new features or improve performance as an app grows. 28 Benefits of Design Patterns for Maintenance Design Patterns Simplify Maintenance Improved Code Readability: Clear structure makes it easy for developers to understand and modify. Reduced Code Duplication: Reuse common functionality, e.g., using the Decorator pattern for adding features. Ease of Refactoring: Adapter, Facade patterns enable easy updates or extensions. Example: Repository pattern abstracts data logic, allowing easy updates to database operations without rewriting core application logic 29 Case Study 1 – MVC for Scalability Case Study: MVC in Action Example: A large e-commerce website using the MVC pattern. Scalability: Views (front-end) scaled independently from Models (back-end). Outcome: Easier to maintain and improve user experience without affecting backend logic. 30 Slide 7: Case Study 2 – Singleton for Maintenance Case Study: Singleton for Database Management Example: A web application using Singleton for database connections. Maintenance: Single database instance ensures consistency and reduces memory usage. Outcome: Easier to maintain database connections and troubleshoot issues without creating multiple instances. 31 Conclusion – Summary of Benefits Summary of Benefits Scalability: Modular, decoupled code makes it easy to scale applications as they grow. Maintenance: Consistent structure, reusability, and better resource management simplify ongoing development. Long-term Success: Design patterns contribute to both improved performance and easier updates. 32 Choosing the Right Pattern Assessing Project Needs: Identify the problem or requirement. Match with appropriate design patterns. Avoiding Overuse: Apply patterns based on necessity, not trends. 33 Best Practices in Applying Design Patterns Understand the Patterns: Study patterns and their use cases. Follow Good Design Principles: Simplicity, encapsulation, and flexibility. Maintain Code Quality: Adhere to SOLID principles. Document the patterns used. 34 Educating and Training Team Training: Workshops and resources. Promoting Best Practices: Encouraging consistent use of design patterns 35 Educating and Training Team Training: Workshops and resources. Promoting Best Practices: Encouraging consistent use of design patterns 36 The end Thank you 9/29/2024 37