Lecture08.pdf
Document Details
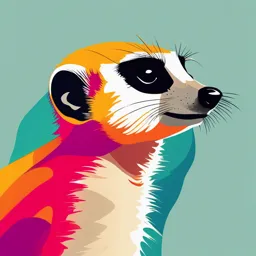
Uploaded by SmoothestSunstone
Tags
Full Transcript
Assembly Language Programming IV: shift, struct, recursion 1 Homework • Reading – PAL, pp 216-227 2 Using C Structs in Assembly Code • How do we access a C structure such as: #define NAMELEN 20 struct teststruct { int x; int y; char name[NAMELEN]; }t; t.x = 2; t.y = 5; strncpy(t.name, “wilson”...
Assembly Language Programming IV: shift, struct, recursion 1 Homework • Reading – PAL, pp 216-227 2 Using C Structs in Assembly Code • How do we access a C structure such as: #define NAMELEN 20 struct teststruct { int x; int y; char name[NAMELEN]; }t; t.x = 2; t.y = 5; strncpy(t.name, “wilson”, NAMELEN); trystruct(&t); /* pass to asm via pointer*/ 3 Using C Structs in Assembly Code • Assembly code would look like: Movl Movl Movl Movb 4(%esp),%edx (%edx),%eax 4(%edx),%ebx 8(%edx),%cl # # # # ptr to t: edx=0x0200e0 x itself: eax = 2 y itself: ebx = 5 1st string char: cl = ‘w’ struct teststruct t.x Stack ... %esp Return %eip 0xfffff4 0xfffff8 t.y ‘w’ ‘i’ ‘l’ ‘s’ ‘o’ ‘n’ ‘\0’ 0x0200e0 0x0200e4 0x0200e8 . . . 0x0200e0 0xfffffc 4 Using C Structs in Assembly Code • However, we would normally have a pointer to string: #define NAMELEN 20 char array [NAMELEN]; struct teststruct { int x; int y; char *name; }t; t.x = 2; t.y = 5; t.name = array; strncpy(array, “wilson”, NAMELEN); trystruct(&t); /* pass to asm via pointer*/ 5 Using C Structs in Assembly Code • Assembly code would look like: movl 4(%esp),%edx # ptr to t: edx = 0x0200e0 movl movl movl movb (%edx),%eax 4(%edx),%ebx 8(%edx),%edx (%edx),%cl # # # # x itself: eax = 2 y itself: ebx = 5 ptr to string: edx = 0x20158 first string char: cl = ‘w’ struct teststruct t.x Stack ... %esp Return %eip 0xfffff4 0xfffff8 t.y t.name 0x0200e0 0x0200e4 0x0200e8 . . . &t 0xfffffc ‘w’ ‘i’ ‘l’ ‘s’ ‘o’ ‘n’ ‘\0’ 0x020158 . . . 6 Introduction to Shift Instructions • We can shift the bits in a byte, word, or long word by a variable number of positions • These are the machine level instructions used to implement the C language operators << and >> – SAL / SHL are the left shift instructions for signed or unsigned data. SAL and SHL performs the same operations – SAR is the right shift instruction for signed data (arithmetic right shift) – SHR is the right shift instruction for unsigned data 7 (logical right shift) Introduction to Shift Instructions • The SAL / SHL Instruction (Signed / Unsigned) CF 0 • The SAR Instruction (Signed or Arithmetic shift) CF • The SHR Instruction (Unsigned or logical shift) 0 CF 8 Introduction to Shift Instructions • The target of the shifting can be a register or memory location (byte, word, or long word) • The count for the number of bits to shift can be specified with immediate data (constant) or the %cl register (variable) • Examples: sall $4, %eax sarb %cl, label # logical left shift of %eax by 4 bits # arithmetic right shift of memory byte # by a variable value stored in the %cl 9 Introduction to Shift Instructions • Multiplication by 2N can be done via left shift sall $4, %eax # %eax times 24 • Can combine left shifts and addition • Division by 2N can be done via right shift sarb %cl, label # memory byte / 2%cl • Can combine right shifts and subtraction 10 Introduction to Multiply and Divide • Unsigned Multiply and Divide – mul – div • Signed Multiply and Divide – imul – idiv • We won’t do much with these because of the complexity involved - especially for divide 11 Introduction to Multiply and Divide • Multiply always operates with %al, %ax, or %eax • Result needs more bits than either operand • Syntax: mulb %bl %ax %al * %bl mulw %bx %dx, %ax %ax * %bx mull %ebx %edx, %eax %eax * %ebx 12 Introduction to Multiply and Divide • Register Pictures (Byte) %bl * %ax (Word) %bx * %dx, %ax 13 Example – For/While Loop and mul • C code for n = 5! (done as a for loop) unsigned int i, n; n = 1; for (i = 1; i <= 5; i++) n *= i; • C code for n = 5! (done as a while loop) unsigned int i, n; n = i = 1; while (i <= 5) n *= i++; 14 Example – For/While Loop and mul • Assembly code for n = 5! (byte * byte = word) loop: exit: movb movb cmpb ja mulb incb jmp $1, %bl %bl, %al $5, %bl exit %bl %bl loop # # # # # # # # i = 1 n = i = 1 while (%bl <= 5) %bl > 5 now %ax = %al * %bl incr %bl and loop 5! in %ax • Note: No difference between for and while in assy 15 Example – For/While Loop and mul • Assembly code for n = 5! (word * word = long) loop: movw $1, %bx movw %bx, %ax cmpw $5, %bx ja exit mulw %bx incw %bx jmp loop exit: # # # # # # # # # i = 1 n = i = 1 while (%bx <= 5) %bx > 5 now %ax = %ax * %bx %dx = 0 now incr %bx and loop 5! in %eax 16 Recursive Factorial • Main program to call recursive factorial function .text pushl call addl ret $5 factorial $4, %esp 17 Recursive Factorial factorial: movl cmpl jna decl pushl call addl movw mulw return: ret .end # works up to 16 bit results 4(%esp), %eax $1, %eax return %eax %eax factorial $4, %esp 4(%esp), %bx %bx # 16 lsbs go to %ax # ignore msbs in %dx 18 Recursive Factorial stack frames Stack Operations (while calling) Decr & 5th Call Decr & 4th Call Decr & 3rd Call Decr & 2nd Call %esp at main 1st Call %eip value %eip value %eip value %eip value %eip value (fact) 1 (fact) 2 (fact) 3 (fact) 4 (main) 5 arg ==1 so Multiply & 1st Return 2nd Return %eax = 1 %eax = 2 Multiply & Multiply & 3rd Return 4th Return %eax = 6 %eax = 24 Multiply & 5th Return %eax = 120 Stack Operations (while returning)