Lecture 3.2_ Conditional Execution.pdf
Document Details
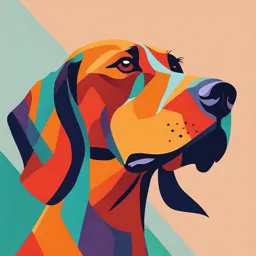
Uploaded by GenerousChrysoprase
La Trobe University
Tags
Related
- 3-4.pdf
- Unit 1 Python Basics, Control Structures, and Other Features PDF
- Python Programming Assignment PDF
- Programming in Python for Business Analytics (BMAN73701) Lecture Notes PDF
- SDU University CSS 115: Python Programming Fundamentals Fall 2024 Lecture 4 PDF
- Computer Science Class XI Past Paper PDF 2023-24
Full Transcript
Lecture 3.2 Conditional Execution Lecture Overview 1. Control Flow 2. Selection Statements 3. Conditional Execution Pitfalls 2 1. Control Flow 3 Control Flow ◎ Control flow describes the order in which the statements making up a program are executed. ◎ So far all of the Python code we've lo...
Lecture 3.2 Conditional Execution Lecture Overview 1. Control Flow 2. Selection Statements 3. Conditional Execution Pitfalls 2 1. Control Flow 3 Control Flow ◎ Control flow describes the order in which the statements making up a program are executed. ◎ So far all of the Python code we've looked at has had sequential control flow. ◎ However, there are a few different control structures which can result in different kinds of control flow. 4 Control Flow: Sequence (or sequential) ◎ The most basic control structure is a sequence of statements. ◎ Represented by a series of process/input/output elements in a flowchart. ◎ Statements in a sequence are executed in order of appearance. Statement 1 ◎ There is only one possible path for control flow. Statement 2 Statement 3 5 Control Flow: Sequence (or sequential) Example Program: Temperature Converter Start Input number Multiply by 9 Divide by 5 Add 32 Output number # Input tc = input('Enter Celsius: ') x = float(tc) # Processing x = x * 9 # Multiply by 9 x = x / 5 # Divide by 5 x = x + 32 # Add 32 # Output tf = str(x) print('Fahrenheit: ' + tf) Stop 6 Control Flow: Selection ◎ In a selection control structure, a condition determines which statements are executed. ◎ A selection introduces multiple paths for control flow to take, from which one will be selected. ◎ Represented by a decision element in a flowchart. yes no Condition Statement 1 Statement 2 ◎ This allows the program to make a decision. 7 Check Your Understanding Q. Does the flowchart show a sequence or selection control structure? Start Input number Multiply by 20 Divide by 4 Add 10 Output number Stop 8 Check Your Understanding Q. Does the flowchart show a sequence or selection control structure? A. Sequence. There is only one possible path for control flow to take. Start Input number Multiply by 20 Divide by 4 Add 10 Output number Stop 9 Visualising Control Flow ◎ Drawing the control flow over a flowchart can be helpful when reasoning about a program's logic. ◎ When drawn in this way, control flow is a single path from the start element to the stop element. ○ The control flow never splits. ○ When a decision element is encountered, one branch is selected. 10 Control Flow Example: Selection Start 1. Begin at the start element. 2. The first condition determines which path the control flow will take. 3. The condition is not met, so the “no” path is taken. 4. The second condition is met, so the “yes” path is taken. 5. The control flow continues to the stop element. Let age = 78 age < 12? yes no Display “Child” age > 65? no yes Display “Senior” Display “Done.” Stop 11 Check Your Understanding Q. What is the correct control flow for this flowchart? Start Let x = 10 x >= 8? yes no Display “Sad” Display “Happy” Stop 12 Check Your Understanding Q. What is the correct control flow for this flowchart? A. See image. ◎ Control flow can't split. ◎ The condition x >= 8 is met, so the "yes" branch is taken. Start Let x = 10 x >= 8? yes no Display “Sad” Display “Happy” Stop 13 2. Selection Statements 14 Selection Statements ◎ Selection statements are used to define selection control structures. ◎ When coding in Python, indentation MUST be used to group statements under a particular selection statement. 15 Selection Statements ◎ Python has three main selection statements: ○ if statements, which begin a selection control structure by defining a sequence of statements to execute if a condition is met. ○ elif and else statements, which optionally define alternative code paths (or "branches") in the selection control structure. 16 Simple Conditional Execution (if) if Condition: Statement ◎ If the condition is truthy: ○ Execute the statement(s) in the if block. yes Condition no Statement ◎ If the condition is falsy: ○ Do nothing. 17 Example: Simple Conditional Execution Task definition Write a program which subtracts 40% tax from a person's income when their pre-tax income is above $100,000. Display the result. ◎ Input: pre-tax income. ◎ Output: post-tax income. ◎ If pre-tax income is greater than $100,000, then the processing steps are: ○ Calculate tax as 40% of the pre-tax income. ○ Subtract tax from the income. 18 Example: Simple Conditional Execution # File: tax1.py income = float(input('Enter income: $')) if income > 100000: tax = 0.4 * income income = income - tax print(income) ◎ Remember: indentation is important! ◎ If the condition evaluates to: ○ True, then the indented statements are executed. ○ False, then the indented statements are skipped. 19 Conditional Execution - Multiple if statements 20 Conditional Execution - Multiple if statement 21 Conditional Execution - Nested if statements 22 Alternative Execution (if-else) if Condition: Statement 1 else: Statement 2 ◎ If the condition is truthy: ○ Execute the statement(s) in the if block. ◎ If the condition is falsy: ○ Execute the statement(s) in the else block. yes Statement 1 Condition no Statement 2 23 Alternative Execution (if-else) 24 Alternative Execution (if-else) 25 Example: Alternative Execution # File: tax2.py income = float(input('Enter income: $')) if income > 100000: tax = 0.4 * income income = income - tax else: print('No tax applied.') print(income) ◎ Using alternative execution, we can extend our tax program to inform the user when no tax is applied. 26 Chained Conditionals (if-elif-else) if Condition 1: Statement 1 elif Condition 2: Statement 2 else: Statement 3 ◎ elif is a contraction of "else if". ◎ The else clause is optional. ◎ Multiple elif clauses are allowed. 27 Example: Chained Conditionals # File: tax3.py income = float(input('Enter income: $')) if income > 100000: tax = 0.4 * income income = income - tax elif income > 50000: tax = 0.3 * income income = income - tax print(income) ◎ Using chained conditionals, we can extend our original tax program to include a 30% tax bracket. 28 Chained Conditionals (if-elif-else) 29 Chained Conditionals (if-elif-else) 30 Chained Conditionals ◎ Chained conditionals introduce the possibility of having many different branches in the same selection control structure. ○ Only one of these branches will be executed. ◎ If multiple conditions could be satisfied, control flow will go through the branch which appears first. ○ In fact, once a branch is taken the rest of the control structure is skipped---the remaining conditions aren't even evaluated. 31 Check Your Understanding Q. Which print statement will never be executed, regardless of the input? x = float(input()) if x > 25: print('Big') elif x > 50: print('Huge') elif x > 5: print('Medium') else: print('Small') print('Done.') 32 Check Your Understanding Q. Which print statement will never be executed, regardless of the input? A. print('Huge'). Any value of x that could satisfy x > 50 must also satisfy x > 25. Since x > 25 is checked first, print('Huge') can never be executed. x = float(input()) if x > 25: print('Big') elif x > 50: print('Huge') elif x > 5: print('Medium') else: print('Small') print('Done.') 33 3. Conditional Execution Pitfalls 34 Mistake #1: Indentation ◎ Indentation is important---incorrect indentation is a common beginner mistake! ◎ You must use consistent indentation. ○ I strongly recommend using 4 spaces. ◎ Python uses indentation to group statements into blocks. 35 Indentation Rules ◎ Increase indentation after if, elif, and else statements. ◎ Maintain indentation for all statements grouped under the if, elif, or else statement. ◎ Reduce indentation to end the if, elif, or else block. ◎ Blank lines and comment-only lines ignore indentation rules. 36 Example: Incorrect Indentation >>> if x > 2: ... x = x / 2 ... else: ... x = x + 5 File "<stdin>", line 4 x = x + 5 ^ IndentationError: expected an indented block 37 Check Your Understanding Q. Will this Python script result in an error? If not, what is the expected output? 1 2 3 4 5 6 7 price = 90 if price > 100: print('Discount') price = price - 2 print(price) 38 Check Your Understanding Q. Will this Python script result in an error? If not, what is the expected output? A. No error, output 88. ◎ Line 4 is not executed (condition is not true). ◎ Lines 5 and 7 are executed, since they are not part of the conditional (indentation reduced). 1 2 3 4 5 6 7 price = 90 if price > 100: print('Discount') price = price - 2 print(price) 39 Mistake #2: Empty Blocks ◎ An if, elif, or else statement must be followed by at least one indented statement. ○ Comments do not count! Beware! The following program will result in an error: x = 5 if x > 10: # Do nothing... else: print('Small x') 40 The pass Keyword ◎ If you really want to do nothing in a block, use the pass keyword. x = 5 if x > 10: # Do nothing... pass else: print('Small x') ◎ In Python, pass is a statement which does nothing. 41 Mistake #3: Missing Variable Definitions ◎ Take care when creating variables in a conditional and using them afterwards. ◎ If you create (define) a variable in one path, but the control flow does not take that path, the variable will not be defined. ○ Trying to use that variable afterwards will result in an error. ◎ Tip: before using a variable, ensure that it is defined in all possible control flows leading to that point. 42 Example: Missing Variable Definitions Beware! The variable y is not defined, leading to an error. >>> x = 5 >>> if x > 10: ... y = 42 ... >>> print(x + y) Traceback (most recent call last): File "<stdin>", line 1, in <module> NameError: name 'y' is not defined 43 Example: Missing Variable Definitions ◎ Both of the solutions below ensure that y is always defined before it is used. x = 5 y = 0 if x > 10: y = 42 x = 5 if x > 10: y = 42 else: y = 0 print(x + y) print(x + y) 44 Nested Conditionals ◎ Complex decisions can be handled by nesting if statements. ◎ A nested if statement is an if statement contained within another if, elif, or else block. ◎ In Python, nesting is achieved through deeper levels of indentation. 45 Example: Nested Conditionals Task definition Write a program which prints whether an input number is "negative" or "positive". If the number is positive, also print out whether the number is "even" or "odd". 46 Example: Nested Conditionals Task definition Write a program which prints whether an input number is "negative" or "positive". If the number is positive, also print out whether the number is "even" or "odd". x = int(input()) if x < 0: print('negative') if x > 0: print('positive') if x % 2 == 0: print('even') else: print('odd') ◎ Then nested if/else statement (highlighted) is only encountered when x > 0 is true. 47 Things Can Get Confusing! ◎ Sometimes having a lot of chained and nested conditionals can get confusing. ◎ Using print statements is a good way of tracing control flow. ○ Simply place print statements at various points in the code. ○ When you don't need the print statements anymore, delete them (or comment them out). 48 Example: Tracing Control Flow print('[1]') income = 110000 if income > 50000: print('[2]') tax = 0.3 * income income = income - tax elif income > 100000: print('[3]') tax = 0.4 * income income = income - tax print('[4]') ◎ This program is not working as expected---not enough tax is being applied. ◎ We can add the highlighted print statements to trace control flow and help us debug the issue. print(income) 49 Example: Tracing Control Flow print('[1]') income = 110000 if income > 50000: print('[2]') tax = 0.3 * income income = income - tax elif income > 100000: print('[3]') tax = 0.4 * income income = income - tax print('[4]') Output: [1] [2] [4] 77000.0 ◎ We now know which path the control flow takes. print(income) ◎ This also reveals the source of our error: the conditions are checked in the wrong order. 50 Example: Tracing Control Flow income = 110000 if income > 100000: tax = 0.4 * income income = income - tax elif income > 50000: tax = 0.3 * income income = income - tax print(income) ◎ We can fix the problem by reordering the branches in the selection control structure. ◎ Now that the bug is fixed, we can remove the tracing print statements. 51 Summary 52 In This Lecture We... ◎ Learnt how basic control structures determine control flow. ◎ Expressed conditional execution in Python code using if-elif-else structures. ◎ Explored common pitfalls conditional execution. when dealing with 53 Next Lecture We Will... ◎ Learn how the iteration control structure can be used to execute the same code multiple times. 54 Thanks for your attention! The slides and lecture recording will be made available on LMS. The “Cordelia” presentation template by Jimena Catalina is licensed under CC BY 4.0. PPT Acknowledgement: Dr Aiden Nibali, CS&IT LTU. 55