Lecture 3.1_ Comparisons and Boolean Logic.pdf
Document Details
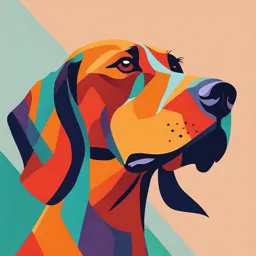
Uploaded by GenerousChrysoprase
La Trobe University
Tags
Related
- Python Game Coding Level 1 Learner Resource Lesson 3 PDF
- Fundamentals of Python: First Programs - Chapter 3 Control Statements PDF
- Boolean Logic and Circuits Slides PDF
- Python Book 3 Decision Structures and Boolean Logic PDF
- Boolean Expressions in Python PDF
- Lecture 3 - Programming 2 - 2024-2035 PDF
Full Transcript
Lecture 3.1 Comparisons and Boolean Logic We have covered in previous weeks (Week 1 and Week 2) ◎ ◎ ◎ ◎ ◎ ◎ Flowcharts How to install and run Python editor Program Development steps Input, Processing, and Output Variables, Statements, and Comments Expressions and Data Types Program Development...
Lecture 3.1 Comparisons and Boolean Logic We have covered in previous weeks (Week 1 and Week 2) ◎ ◎ ◎ ◎ ◎ ◎ Flowcharts How to install and run Python editor Program Development steps Input, Processing, and Output Variables, Statements, and Comments Expressions and Data Types Program Development steps ◎ A program can be used to solve complex problem ○ ○ Programs are a set of written actions in order to fulfil a need / solve a problem A programming language is the tool used to create a solution (Program) Design a flowchart Implement: Python print data Problem Task Definition if condition true print data Solution for x in range print x Call function ... 3 Python Input ◎ Take Input from User – direct or keyboard ◎ Take input from File – text, csv, binary, etc ◎ Take input from System – click, button, drop-down, mouse 4 Python Input ◎ Take Input from User – Direct >>> x = 10 # integer >>> y = 0.6 # float >>> z = “Hello” # string >>> x = 10 >>> y = 0.6 >>> z = x + y >>> x = “Hello” >>> y = “CSE4IP” >>> z = x + y # integer # float # string # string 5 Python Input ◎ Take Input from User – keyboard Python uses input() function to get input from the user - Keyboard Syntax: - input(“prompt”) - prompt : a string - a default message displayed before the input 6 Python Input ◎ Take Input from User – keyboard input(“prompt”) Example: take three different inputs from user. >>> x = input (“Enter a number: ”) Enter a number: 5 >>> y = input (“Enter a number: ”) Enter a number: 8.3 >>> z = input (“Enter a string: ”) Enter a string: Hello CSE4IP 7 Python Input Take Input from User – keyboard: input(prompt) Example: take three different inputs from user. >>> x = input (“Enter a number: ”) Enter a number: 5 >>> X ‘5’ >>> y = input (“Enter a number: ”) Enter a number: 8.3 >>> y ‘8.3’ >>> z = input (“Enter a string: ”) Enter a string: Hello CSE4IP >>> Z ‘Hello CSE4IP’ o o o o o We can see that all inputs are saved as string (‘’) We can use Explicit Type Conversion functions (covered in Lecture 2.2) to convert them into a proper data type. int() float() str() 8 Python Input Take Input from User – keyboard: input(prompt) 9 Python Output ◎ Display the output of a program to the standard output device - screen and console ◎ Save the output in a file – text, csv, binary, …, etc. 10 Python Output 11 Comparisons and Boolean Logic Topic 3 Intended Learning Outcomes ◎ By the end of week 3 you should be able to: ○ Write boolean expressions for questions with yes/no answers, and ○ Use selection control structures to specify different flows through a program. 13 Lecture Overview 1. Booleans 2. Comparison Operators 3. Logical Operators 14 1. Booleans 15 Review of Types ◎ We have encountered three data types so far: ○ str (string): A string of characters (i.e. text). ◉ e.g. ‘CSE4IP', "August". ○ int (integer): A whole number. ◉ e.g. 23, -1000 ○ float (floating point number): A number with a fractional part. ◉ e.g. 23.45, -5.0 16 How About Yes/No Values? ◎ How would we store the answer to a yes/no question? ○ e.g. Is the video paused? ◎ Could use strings: ○ is_paused = 'yes' ○ Quickly gets confusing. Does "YES" also mean yes? "Y"? "True"? ◎ Could use integers (0 means no, 1 means yes). ○ is_paused = 1 ○ Not very readable. 17 Introducing Booleans ◎ A boolean represents a binary value. ○ Yes/no, true/false, on/off. ◎ There are only two possible boolean values. ◎ Useful for representing answers to yes/no questions. ○ Did the user opt into the newsletter? ○ Is the car new? ○ Is the average test score greater than 50? 18 Visual Representations of Booleans 19 Booleans in Python ◎ In Python, booleans have the bool type. ◎ The two boolean literals in Python are True and False. ○ These are the only two values of type bool. >>> type(True) <class 'bool'> >>> type(False) <class 'bool'> ◎ For the previous example: ○ is_paused = True 20 Converting Values to Booleans ◎ Values of other types can be converted into booleans. ◎ A value that converts to True is said to be truthy. ◎ A value that converts to False is said to be falsy. 21 Truthy and Falsy: Numbers ◎ For numeric types: ○ Zero is falsy. ○ All other truthy. values are >>> bool(0) False >>> bool(-42) True >>> bool(0.00) False >>> bool(0.0001) True 22 Truthy and Falsy: Strings ◎ For strings: ○ The empty string is falsy. ○ All other values are truthy. ◎ The empty string is a string with no characters in it. >>> bool('hello') True >>> bool('') False >>> bool('False') True >>> bool(' ') True >>> bool("") False 23 Check Your Understanding Q. Is the string 'No' truthy or falsy? 24 Check Your Understanding Q. Is the string 'No' truthy or falsy? A. Truthy. The only falsy string is the empty string. Python does not attempt to read the contents of the string beyond checking if it is empty. 25 2. Comparison Operators 26 Boolean Expressions ◎ Recall that a numeric expression is an expression which evaluates to a number (e.g. 2 + 2). ◎ A boolean expression is an expression which evaluates to a boolean (i.e. True or False). ◎ A simple kind of boolean expression is comparing two values. For example: ○ Is one number greater than another? ○ Are two strings equal? 27 Comparison Operators ◎ Two values can be compared using a comparison operator. ◎ The result of a comparison will be True or False, depending on whether the condition is satisfied. ◎ Note that Python uses double equals (==) to check for equality. PYTHON MATHS CONDITION x == y x=y x is equal to y x != y x≠y x is not equal to y x > y x>y x is greater than y x < y x<y x is less than y x >= y x≥y x is greater than or equal to y x <= y x≤y x is less than or equal to y 28 Numeric Comparisons ◎ Numeric comparisons work as you would expect from mathematics. >>> x = 5 >>> x > 2 True >>> x > 5 False >>> x >= 5 True >>> y = 7.5 >>> x < y True >>> -50 > y False 29 String Comparisons ◎ String comparisons are based on lexicographical ordering. ○ This is similar to dictionary ordering. >>> word = 'apple’ ◎ However, all uppercase letters come before lowercase letters. >>> word < 'apple pie' True >>> word > 'banana' False >>> word == 'apple' True >>> word < 'Zucchini' False 30 Comparisons Across Types Beware! Avoid comparing values of different types (e.g. a number and a string)---the results are probably not what you expect. >>> 5 == '5' False >>> 0 < '5' Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: '<' not supported between instances of 'int' and 'str' 31 Check Your Understanding Q. What is the Python boolean expression for "twice the value of x is not equal to 100"? 32 Check Your Understanding Q. What is the Python boolean expression for "twice the value of x is not equal to 100"? A. x * 2 != 100 Or, equivalently: ◎ 2 * x != 100 ◎ 100 != x * 2 ◎ 100 != 2 * x 33 3. Logical Operators 34 Logical Operators ◎ Logical operators can be used to combine and modify boolean expressions. ◎ Python has three logical operators: and, or, not. ○ These generally behave as you would expect from the meaning of the words. 35 The and Operator (Logical And) ◎ True if both expressions are truthy. ◎ False if at least one expression is falsy. P Q P and Q True True True True False False False True False False False False >>> x = 5 >>> y = 7.5 >>> x < y and x == 5 True >>> y == 5 and x == 5 False >>> x == 0 and y <= 0 False 36 The or Operator (Logical Or) ◎ True if at least one expression is truthy. ◎ False if both expressions are falsy. >>> x = 5 >>> y = 7.5 >>> x < y or x == 5 True P Q P or Q True True True >>> y == 5 or x == 5 True True False True >>> x == 0 or y <= 0 False True True False False False False 37 The not Operator (Logical Not) ◎ Negates the expression. ◎ True if the expression is falsy. ◎ False if the expression is truthy. P not P True False False True >>> x = 5 >>> y = 7.5 >>> not x < y False >>> not y == 5 True 38 Combining Boolean Expressions ◎ Logical operators can be used to build complex boolean expressions. ◎ Other kinds of operators can be included in the expression. ◎ For example, "either x is greater than 10 and even, or y is less than 0" can be expressed as: (x > 10 and x % 2 == 0) or y < 0 39 Extended Operator Precedence OPERATOR () NAME Higher Parentheses ** Exponentiation *, /, %, // Multiplication, etc. +, - Addition, etc. ==, !=, >, <, >=, <= Comparison not Logical "not" and Logical "and" or Lower Logical "or" 40 Check Your Understanding Q. What does the following expression evaluate to? 2 * 7 > 5 and not 27 < 8 OPERATOR () NAME Higher Parentheses ** Exponentiation *, /, %, // Multiplication, etc. +, - Addition, etc. ==, !=, >, <, >=, <= Comparison not Logical "not" and Logical "and" or Lower Logical "or" 41 Check Your Understanding Q. What does the following expression evaluate to? 2 * 7 > 5 and not 27 < 8 A. True. 1. 2 * 7 > 5 and not 27 < 8 2. 14 > 5 and not 27 < 8 3. True and not 27 < 8 4. True and not False 5. True and True 6. True OPERATOR () NAME Higher Parentheses ** Exponentiation *, /, %, // Multiplication, etc. +, - Addition, etc. ==, !=, >, <, >=, <= Comparison not Logical "not" and Logical "and" or Lower Logical "or" 42 Summary 43 In This Lecture We... ◎ Discovered the boolean data type. ◎ Compared values using comparison operators. ◎ Used logical operators to construct more complex boolean expressions. 44 Next Lecture We Will... ◎ Use boolean expressions to control the flow of program execution. 45 Thanks for your attention! The slides and lecture recording will be made available on LMS. The “Cordelia” presentation template by Jimena Catalina is licensed under CC BY 4.0. PPT Acknowledgement: Dr Aiden Nibali, CS&IT LTU. 46