Lec-01.pdf
Document Details
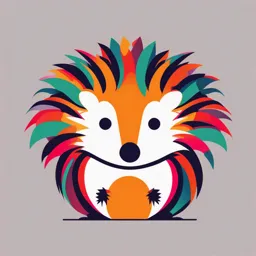
Uploaded by AccuratePrairieDog
2020
Tags
Related
- programmeren H7 Object oriented programming.pdf
- Object Oriented Programming, Lecture 06.pdf
- Object Oriented Programming, Lecture 07.pdf
- Topic 1.3 Defining Object Model_ CS 002-CS21S1 - Advanced Object-Oriented Programming.pdf
- Topic 1.6 Running Program with Objects_ CS 002-CS21S1 - Advanced Object-Oriented Programming.pdf
- B.E-in-Computer-Science-Engineering-updated-on-31.08.2018-5th-CSE-ISE-Open-Elective.pdf
Full Transcript
Programming: An Object-Oriented Approach Chapter 1 Introduction to Computers and Programming Languages...
Programming: An Object-Oriented Approach Chapter 1 Introduction to Computers and Programming Languages BEHROUZ A. FOROUZAN RICHARD F. GILBERG ©2020 McGraw-Hill Education. All rights reserved. Authorized only for instructor use in the classroom. No reproduction or further distribution permitted without the prior written consent of McGraw-Hill Education. Objectives Part 1 To discuss the two major components of a computer: hardware and software. To describe the six parts of hardware: CPU, primary memory, secondary storage, input system, output system, and communication system. To describe the two major categories of software: system software and application software. To describe the evolution of computer languages from machine languages, to assembly languages, and to high-level languages. 2 ©2020 McGraw-Hill Education Objectives Part 2 To discuss four different paradigms of computer languages: procedural, object oriented, functional, and logic. To describe the two steps of program design: understand the problem and develop a solution. To describe the multi-step procedure that transforms a program written in the C++ language to an executable program. 3 ©2020 McGraw-Hill Education COMPUTER SYSTEM We start this chapter with the idea of a computer system. A computer is a system made of two major components: hardware and software. The computer hardware is the physical equipment. The software is the collection of programs (instructions) that allow the hardware to do its job. 4 ©2020 McGraw-Hill Education Computer Hardware Figure 1.1 Basic hardware components Access the text alternative for slide images. 5 ©2020 McGraw-Hill Education Central Processing Unit Figure 1.2 Central processing unit 6 ©2020 McGraw-Hill Education ©leungchopan/Getty Images Primary Memory Figure 1.3 Primary memory 7 ©2020 McGraw-Hill Education ©Simon Belcher/Alamy Secondary Storage Figure 1.4 Some secondary storage devices 8 ©2020 McGraw-Hill Education (Left image): ©Shutterstock/PaulPaladin; (Middle image): ©David Arky/Getty Images; (Right image): ©McGraw-Hill Education Input System Figure 1.5 Some input systems 9 ©2020 McGraw-Hill Education (Left image): ©JG Photography/Alamy; (Right image): ©Keith Eng 2007 Output System Figure 1.6 Some output Systems 10 ©2020 McGraw-Hill Education (Left image): ©Ingram Publishing; (Right image): ©somnuek saelim/123RF Communication System Figure 1.7 Some communication devices 11 ©2020 McGraw-Hill Education (Left image): ©Ingram Publishing; (Right image): ©somnuek saelim/123RF Computer Software System Software. System software consists of programs that manage the hardware resources of a computer and perform required information processing. These programs are divided into three groups: operating system. system support. system development. Application Software. Application software is broken into two categories: general-purpose software. application-specific software. 12 ©2020 McGraw-Hill Education COMPUTER LANGUAGES To write a program for a computer, we must use a computer language. Over the years, computer languages have evolved from machine to symbolic to high-level languages and beyond. Figure 1.8 Computer language evolution 13 ©2020 McGraw-Hill Education Machine Languages In the earliest days of computers, the only programming languages available were machine languages. While each computer still has its own machine language, which is made of streams of 0s and 1s, we no longer program in machine language. The only language understood by a computer is it’s machine language. 14 ©2020 McGraw-Hill Education Symbolic Languages In the early 1950s, Grace Hopper developed the concept of a special computer program for converting programs into machine language. Figure 1.9 Grace Hopper Symbolic language uses mnemonic symbols to represent machine language instructions. 15 ©2020 McGraw-Hill Education ©Cynthia Johnson/Getty Images High-Level Languages The desire to improve programmer efficiency and to change the focus from the computer to the problem being solved led to the development of high-level languages. High-level languages are portable to many different computers, allowing the programmer to concentrate on the application problem at hand rather than the intricacies of the computer. The first widely used high-level language, FORTRAN, was created by John Backus and an IBM team in 1957. Following soon after FORTRAN COBOL was developed by Admiral Grace Hopper. 16 ©2020 McGraw-Hill Education LANGUAGE PARADIGMS A paradigm is a model or a framework for describing how a program handles data. Figure 1.10 Language paradigms Access the text alternative for slide images. 17 ©2020 McGraw-Hill Education Procedural Paradigm Figure 1.11 An example of a procedural paradigm 18 ©2020 McGraw-Hill Education Object-Oriented Paradigm Figure 1.12 An example of an object-oriented paradigm Access the text alternative for slide images. 19 ©2020 McGraw-Hill Education Functional Paradigm Figure 1.13 An example of a functional paradigm Access the text alternative for slide images. 20 ©2020 McGraw-Hill Education Logic Paradigm Figure 1.14 An example of a logic paradigm Access the text alternative for slide images. 21 ©2020 McGraw-Hill Education Paradigms in C++ Language After the discussion of four paradigms, one wonders where the C++ language stands. C++ is an extension to the C language and is based on the procedural paradigm. However, the existence of classes and objects allows the language to be used as an object-oriented language. In this book we use C++ mostly as a procedural paradigm in early chapters (except for input/output that are done using objects). 22 ©2020 McGraw-Hill Education PROGRAM DESIGN Program design is a two-step process that requires understanding the problem and then developing a solution. When we are given the assignment to develop a program, we are given a program requirements statement and the design of any program interfaces. In other words, we are told what the program needs to do. Our job is to determine how to take the inputs we are given and convert them to the outputs that have been specified. 23 ©2020 McGraw-Hill Education Understand the Problem The first step in program design is to understand the problem. We begin by reading the requirements statement carefully. When we fully understand it, we review our understanding with the user. Often this involves asking questions to confirm our understanding. 24 ©2020 McGraw-Hill Education Develop the Solution Figure 1.15 Find largest among five integers Access the text alternative for slide images. 25 ©2020 McGraw-Hill Education Algorithm Generalization Figure 1.16 Algorithm to find largest among n numbers Access the text alternative for slide images. 26 ©2020 McGraw-Hill Education Unified Modeling Language (UML) The Unified Modeling Language (UML) is a standard tool for designing, specifying, and documenting many aspects of a computing system. For example, it can be used to design large complex systems, programs, and objects within a program. It can also be used to show the relationship between objects in an object-oriented language such as C++. 27 ©2020 McGraw-Hill Education PROGRAM DEVELOPMENT Figure 1.17 Writing, editing, and executing a program 28 ©2020 McGraw-Hill Education Write And Edit Program The software used to write programs is known as a text editor. A text editor helps us enter, change, and store character data. The text editor could be a generalized word processor, but it is more often a special editor provided by the company that supplies the compiler. After we complete a program, we save our file to disk. This file then becomes the input to the compiler; it is known as a source file. 29 ©2020 McGraw-Hill Education Compile Programs The information in a source file stored on disk must be translated into machine language so the computer can understand it. This is the job of the compiler. 30 ©2020 McGraw-Hill Education Link Programs A program is made up of many functions. Some of these functions are written by us and are a part of our source program. However, there are other functions, such as input/output processes and mathematical library functions, that exist elsewhere and must be attached to our program. The linker assembles the system functions and ours into the executable file. 31 ©2020 McGraw-Hill Education Execute Program Once the program has been linked, it is ready for execution. To execute a program we use an operating system command, such as run, to load the program into main memory and execute it. In a typical program execution, the program reads data for processing, either from the user or from a file. After the program processes the data, it prepares the output. Data output can be written to the user's monitor or to a file. When the program is finished, it tells the operating system, which removes the program from memory. 32 ©2020 McGraw-Hill Education TESTING After we write the program, we must test it. Program testing can be a very tedious and time-consuming part of program development. As the programmer, we are responsible for completely testing it. We must make sure that every instruction and every possible situation have been tested. That is not a simple task! 33 ©2020 McGraw-Hill Education Designing Test Data Test data should be developed throughout the design and development of a program. As we design the program, we create test cases to verify the design. These test cases then become part of the test data after we write the program. One set of test data never completely validates a program. 34 ©2020 McGraw-Hill Education Program Errors There are three general classifications of errors: Specification Errors. Specification errors occur when the problem definition is either incorrectly stated or misinterpreted. Code Errors. Code errors usually generate a compiler error message. These errors are the easiest to correct. Logic Errors. The most difficult errors to find and correct are logic errors. 35 ©2020 McGraw-Hill Education