Java Programming: Variables & Types (PDF)
Document Details
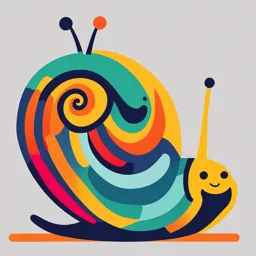
Uploaded by ImpeccableLearning
Tags
Summary
This document provides an overview of variables and their types in Java programming, including local, instance, and class variables, along with detailed explanations and examples of implicit and explicit casting/conversions. It's a great resource for students learning Java.
Full Transcript
JAVA PROGRAMMING UNIT-2 CONTROL FLOW AND ARRAY 2.1 VARIABLE AND IT’S TYPE Variable is a named storage location that holds a value...
JAVA PROGRAMMING UNIT-2 CONTROL FLOW AND ARRAY 2.1 VARIABLE AND IT’S TYPE Variable is a named storage location that holds a value of a particular type. Variables are used to store and manipulate data within a program. Java variables can be classified into different types based on their characteristics and usage. 1.Local Variables: Local variables are declared within a method, constructor, or a block of code. They are accessible only within the scope where they are declared. Local variables must be initialized before they are used. Their lifespan is limited to the block in which they are declared. EXAMPLE: public class LocalVariableExample { public static void main(String[] args) { int age = 25; // local variable System.out.println("Age: " + age); } } 2.Instance Variables (Non-Static Variables): Instance variables are declared within a class but outside of any method, constructor, or block. Each instance of a class has its own copy of instance variables. They are initialized with default values if not explicitly assigned. Instance variables are accessible throughout the class and can be accessed using the object of the class. EXAMPLE: public class InstanceVariableExample { String name; // instance variable public static void main(String[] args) { InstanceVariableExample obj = new InstanceVariableExample(); JAVA PROGRAMMING obj.name = "John"; // accessing instance variable using object System.out.println("Name: " + obj.name); } } 3.Class Variables (Static Variables): Class variables are declared with the static keyword within a class but outside of any method, constructor, or block. Unlike instance variables, there is only one copy of each class variable that is shared among all instances of the class. Class variables are initialized with default values if not explicitly assigned. They can be accessed using the class name itself, without creating an instance of the class. EXAMPLE: public class ClassVariableExample { static int count; // class variable public static void main(String[] args) { ClassVariableExample.count = 10; // accessing class variable using class name System.out.println("Count: " + ClassVariableExample.count); } } 2.2 TYPE CASTING AND CONVERSION TYPE CASTING Type casting refers to the process of converting a value from one data type to another. Java supports two types of casting: implicit casting (widening) and explicit casting (narrowing). 1.Implicit Casting (Widening): This type of casting takes place when two data types are automatically converted. It is also known as Implicit Conversion. This happens when the two data types are compatible and also when we assign the value of a smaller data type to a larger data type. EXAMPLE: public class ImplicitCastingExample { public static void main(String[] args) { JAVA PROGRAMMING int myInt = 10; double myDouble = myInt; // Implicit casting from int to double System.out.println("myInt: " + myInt); System.out.println("myDouble: " + myDouble); } } 2.Explicit Casting (Narrowing): The process of conversion of higher data type to lower data type is known as narrowing typecasting. It is not done automatically by Java but needs to be explicitly done by the programmer, which is why it is also called explicit typecasting. EXAMPLE: public class ExplicitCastingExample { public static void main(String[] args) { double myDouble = 10.5; int myInt = (int) myDouble; // Explicit casting from double to int System.out.println("myDouble: " + myDouble); System.out.println("myInt: " + myInt); } } CONVERSION Type conversion is simply the process of converting data of one data type into another. This process is known as type conversion, typecasting, or even type coercion. The Java programming language allows programmers to convert both primitive as well as reference data types. 1.Converting String to int: public class StringToIntExample { public static void main(String[] args) { String numberString = "123"; int number = Integer.parseInt(numberString); System.out.println("Number: " + number); } } JAVA PROGRAMMING 2.Converting int to String: public class IntToStringExample { public static void main(String[] args) { int number = 123; String numberString = String.valueOf(number); System.out.println("Number: " + numberString); } } 3.Converting String to double: public class StringToDoubleExample { public static void main(String[] args) { String numberString = "10.5"; double number = Double.parseDouble(numberString); System.out.println("Number: " + number); } } 2.3 WRAPPER CLASS Wrapper classes are used to provide a way to treat primitive data types as objects. Each primitive data type has a corresponding wrapper class in Java. Wrapper classes are mainly used for converting primitive types into objects, enabling them to be used in scenarios that require objects. Primitive Type Wrapper class boolean Boolean char Character byte Byte short Short int Int long Long float Float double Double JAVA PROGRAMMING 2.3.1 Auto boxing The automatic conversion of primitive data type into its corresponding wrapper class is known as auto boxing, for example, byte to Byte, char to Character, int to Integer, long to Long, float to Float, boolean to Boolean, double to Double, and short to Short. Wrapper class Example: Primitive to Wrapper: public class WrapperExample1 { public static void main(String args[]) { //Converting int into Integer int a=20; Integer i=Integer.valueOf(a);//converting int into Integer explicitly Integer j=a;//autoboxing, now compiler will write Integer.valueOf(a) internally System.out.println(a+" "+i+" "+j); } } Output: 20 20 20 2.3.2 Unboxing The automatic conversion of wrapper type into its corresponding primitive type is known as unboxing. It is the reverse process of auto boxing. Wrapper class Example: Wrapper to Primitive public class WrapperExample2 { public static void main(String args[]) { //Converting Integer to int Integer a=new Integer(3); int i=a.intValue();//converting Integer to int explicitly int j=a;//unboxing, now compiler will write a.intValue() internally JAVA PROGRAMMING System.out.println(a+" "+i+" "+j); }} Output: 333 EXAMPLE: public class WrapperExample { private int value; public WrapperExample(int value) { this.value = value; } public int getValue() { return value; } public void setValue(int value) { this.value = value; } public static void main(String[] args) { WrapperExample wrapper = new WrapperExample(10); System.out.println("Initial value: " + wrapper.getValue()); wrapper.setValue(20); System.out.println("Updated value: " + wrapper.getValue()); } } JAVA PROGRAMMING 2.4 DECISION AND CONTROL STATEMENTS Java compiler executes the code from top to bottom. The statements in the code are executed according to the order in which they appear. However, Java provides statements that can be used to control the flow of Java code. Such statements are called control flow statements. It is one of the fundamental features of Java, which provides a smooth flow of program. 1.Decision Making statements if statements switch statement 2.Loop statements do while loop while loop for loop for-each loop 3.Jump statements break statement continue statement 1.Decision Making statements As the name suggests, decision-making statements decide which statement to execute and when. Decision- making statements evaluate the Boolean expression and control the program flow depending upon the result of the condition provided. There are two types of decision-making statements in Java, i.e., If statement and switch statement. 1) If Statement: In Java, the "if" statement is used to evaluate a condition. The control of the program is diverted depending upon the specific condition. The condition of the If statement gives a Boolean value, either true or false. In Java, there are four types of if-statements given below. Simple if statement if-else statement if-else-if ladder Nested if-statement Simple if statement: It is the most basic statement among all control flow statements in Java. It evaluates a Boolean expression and enables the program to enter a block of code if the expression evaluates to true. Syntax: if(condition) { statement 1; //executes when condition is true } JAVA PROGRAMMING EXAMPLE: public class Student { public static void main(String[] args) { int x = 10; int y = 12; if(x+y > 20) { System.out.println("x + y is greater than 20"); } } } Output: x + y is greater than 20 if-else statement The if-else statement is an extension to the if-statement, which uses another block of code, i.e., else block. The else block is executed if the condition of the if-block is evaluated as false. Syntax: if(condition) { statement 1; //executes when condition is true } else{ statement 2; //executes when condition is false } EXAMPLE: public class Student { public static void main(String[] args) { int x = 10; int y = 12; if(x+y < 10) { System.out.println("x + y is less than 10"); } else { System.out.println("x + y is greater than 20"); } } } JAVA PROGRAMMING Output: x + y is greater than 20 if-else-if ladder: The if-else-if statement contains the if-statement followed by multiple else-if statements. In other words, we can say that it is the chain of if-else statements that create a decision tree where the program may enter in the block of code where the condition is true. We can also define an else statement at the end of the chain. Syntax: if(condition 1) { statement 1; //executes when condition 1 is true } else if(condition 2) { statement 2; //executes when condition 2 is true } else { statement 2; //executes when all the conditions are false } EXAMPLE: public class Student { public static void main(String[] args) { String city = "Delhi"; if(city == "Meerut") { System.out.println("city is meerut"); }else if (city == "Noida") { System.out.println("city is noida"); }else if(city == "Agra") { System.out.println("city is agra"); }else { System.out.println(city); } } } Output: Delhi JAVA PROGRAMMING Nested if-statement: In nested if-statements, the if statement can contain a if or if-else statement inside another if or else-if statement. Syntax: if(condition 1) { statement 1; //executes when condition 1 is true if(condition 2) { statement 2; //executes when condition 2 is true } else{ statement 2; //executes when condition 2 is false } } EXAMPLE: public class Student { public static void main(String[] args) { String address = "Delhi, India"; if(address.endsWith("India")) { if(address.contains("Meerut")) { System.out.println("Your city is Meerut"); }else if(address.contains("Noida")) { System.out.println("Your city is Noida"); }else { System.out.println(address.split(",")); } }else { System.out.println("You are not living in India"); } } } Output: Delhi JAVA PROGRAMMING Switch Statement: In Java, Switch statements are similar to if-else-if statements. The switch statement contains multiple blocks of code called cases and a single case is executed based on the variable which is being switched. The switch statement is easier to use instead of if-else-if statements. It also enhances the readability of the program. Points to be noted about switch statement: The case variables can be int, short, byte, char, or enumeration. String type is also supported since version 7 of Java Cases cannot be duplicate Default statement is executed when any of the case doesn't match the value of expression. It is optional. Break statement terminates the switch block when the condition is satisfied. It is optional, if not used, next case is executed. While using switch statements, we must notice that the case expression will be of the same type as the variable. However, it will also be a constant value. Syntax: switch (expression){ case value1: statement1; break;. case valueN: statementN; break; default: default statement; } EXAMPLE: public class Student{ public static void main(String[] args) { int num = 2; switch (num){ case 0: System.out.println("number is 0"); break; case 1: System.out.println("number is 1"); break; JAVA PROGRAMMING default: System.out.println(num); } } } Output: 2 2. Loop statements In programming, sometimes we need to execute the block of code repeatedly while some condition evaluates to true. However, loop statements are used to execute the set of instructions in a repeated order. The execution of the set of instructions depends upon a particular condition. In Java, we have three types of loops that execute similarly. However, there are differences in their syntax and condition checking time. for loop while loop do-while loop for loop for loop is similar to C and C++. It enables us to initialize the loop variable, check the condition, and increment/decrement in a single line of code. We use the for loop only when we exactly know the number of times, we want to execute the block of code. Syntax: for(initialization, condition, increment/decrement) { //block of statements } JAVA PROGRAMMING EXAMPLE: public class Calculattion { public static void main(String[] args) { // TODO Auto-generated method stub int sum = 0; for(int j = 1; j