IPT FINALS PDF
Document Details
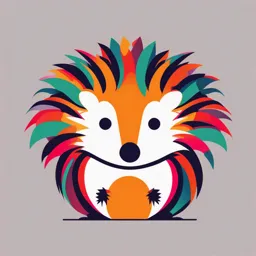
Uploaded by RealisticTangent5570
Tags
Summary
This document is a collection of notes about the Graphics class, methods like paint(), repaint(), drawString(), setFont(), setColor(), and others in the Java programming language. It includes sections on drawing lines, rectangles, arcs, and polygons in graphics applications. The document also showcases the concepts of creating graphics and graphics2D objects. It covers several topics within the field of computer science.
Full Transcript
**WEEK 13** **The Graphics Class** - provides the framework for all graphics operations within the AWT - plays two different, but related, roles: § sets the graphics context § provides methods for drawing simple geometric shapes, text, and images to the graphics destination · Bec...
**WEEK 13** **The Graphics Class** - provides the framework for all graphics operations within the AWT - plays two different, but related, roles: § sets the graphics context § provides methods for drawing simple geometric shapes, text, and images to the graphics destination · Because the Graphics class is an abstract base class, it cannot be instantiated directly. ** ** **The paint()and repaint()Methods** - The paint () method is used to display text, shapes, and graphics within the applet window. - The header for the paint() method is: o public void paint(Graphics g) · You don't call the paint () method directly. Instead, you call the repaint() method when a window needs to be updated, such as when it contains new images or you moved a new object onto the screen **The drawString()Method** · Allows you to draw a String in a JApplet window. · Requires three arguments: a String, an xaxis coordinate, and a y-axis coordinate. · A member of the Graphics class, thus you need to use a Graphics object to call it. **Using the setFont() Method** · The appearance of strings drawn using Graphics object can be modified using the setFont() method. · The setFont() method requires a Font object, which you create with a statement such as, o Font font = new Font(\"Verdana\", Font.BOLD, 26); · Then, the Graphics object is instructed to use the font by using it as the argument in a setFont() method call. **Using the setColor() Method** · The Colorclass contains 13 constants. · These constants can be used as argument to the setColor() method. Example: g.setColor(Color.BLUE); · You can create your own Colorobject with the statement: Color myColor = new Color(r,g,b); Where r, g, and b are numbers representing the intensities of red, green, and blue in the desired color. · These three numbers can range from 0 to 255, with 0 being the darkest shade of the color and 255 being the lightest. **Creating Graphics and Graphics2DObjects** · Supposed you want to display a string when the user clicks a JButton, you write the actionPerformed() method as follows: public void actionPerformed(ActionEvent e) { Graphics g = getGraphics(); g.drawString(\"You pressed the button!\", 50, 100); } **WEEK 14** **Drawing Lines and Rectangles** · The drawLine() method is used to draw a straight line between any two points on the screen. · This method takes the four arguments: x- and y-coordinates of the line's starting point and the x- and y-coordinates of the line's end. o Example: § line.drawLine(10, 10, 100, 200); · The drawRect() method is used to draw the outline of a rectangle while the fillRect() method is used to draw a solid, or filled, rectangle. · Each of these methods requires four arguments: o The first two arguments represent the x- and y coordinates of the upper-left corner of the rectangle. o The last two arguments represent the width and height of the rectangle. o Example: § drawRect(20, 100, 200, 10); · The clearRect() method also requires four arguments and draws a rectangle. · The drawRoundRect() method creates rectangles with rounded corners. · This method requires six arguments: o The first four arguments match the four arguments required to draw a rectangle: the xand y-coordinates of the upper-left corner, the width, and the height. o The two additional arguments represent the arc width and height associated with the rounded corners. · The drawOval() is one of the methods of a Graphics object that draws a circle or an oval that fits within the rectangle specified by four arguments: x- and y-coordinates, width, and height. · The oval is drawn inside a rectangle whose upper-left hand corner is at (X, Y), and whose width and height are as specified. o Example: § g.drawRect(50, 50, 100, 60); g.drawOval(50, 50, 100, 60); **WEEK 15** **Drawing Arcs** · In Java, you can draw an arc using the Graphics drawArc() method. · The drawArc() method requires six arguments: o The x-coordinate of the upper-left corner of an imaginary rectangle that represents the bounds of the imaginary oval that contains the arc o The y-coordinate of the same point o The width of the imaginary rectangle that represents the bounds of the imaginary oval that contains the arc o The height of the same imaginary rectangle o The beginning arc position o The arc angle · The fillArc() method creates a solid arc. · This method is useful for drawing pie-shaped pieces of a circle or oval. o Example: § solidArc.fillArc(10, 50, 100, 100, 20, 320); solidArc.fillArc(200, 50, 100, 100, 340, 40); **WEEK 16** **Creating Polygons** · In Java, there are two approaches in drawing polygons: a method and a class. · The drawPolygon() method requires three arguments: two integer arrays and a single integer. Example: import javax.swing.\*; import java.awt.\*; public class DrawPolyDemo extends JApplet { public void paint(Graphics g) { int xPoints\[\] = {5, 25, 50, 30, 15, 5}; int yPoints\[\] = {10, 35, 20, 65, 40, 10 }; g.drawPolygon(xPoints, yPoints, xPoints.length); } } · The second approach to drawing polygons is using the Polygon class to construct a Polygon object. · Once you have a Polygon object, you can simply pass it as a single argument to the fillPolygon() method. Example: Polygon poly = new Polygon(xPoints, yPoints, size); g.fillPolygon(poly); · You then use the addPoint() method in statements such as the following to add points to the polygon: o poly.addPoint(100,100); o poly.addPoint(150,200); o poly.addPoint(50,250); ** ** **Copying an Area** · The copyArea() method is used to copy a rectangular area of a Graphics object into another area. This method requires six parameters: o The x- and y-coordinates of the upper-left corner of the area to be copied o The width and height of the area to be copied o The horizontal and vertical displacement of the destination of the copy Example: g.copyArea(0, 0, 20, 30, 100, 50); **WEEK 17** **Introduction** · The Java Database Connectivity (JDBC) API is the industry standard for database independent connectivity between the Java programming language and a wide range of databases. · The JDBC API provides a call-level API for SQL-based database access. **JDBC Architecture** · The JDBC API contains two major sets of interfaces: o the JDBC API for application writers o the lower-level JDBC driver API for driver writers **JDBC Architecture** · Direct-to-Database Pure Java Driver o This style of driver converts JDBC calls into the vendor-specific database management system (DBMS) protocol so that client applications can communicate directly with the database server. · Pure Java Driver for Database Middleware o This style of driver translates JDBC calls into the middleware vendor\'s protocol, which is then translated to a DBMS protocol by a middleware server. ** ** **JDBC Overview** · The JDBC API makes it possible to do three things: o Establish a connection with a database or access any tabular data source o Send SQL statements o Process the results **Getting Started** · In order to create Java application that interacts with a database, you need to do the following: o Install the Java platform, which includes the JDBC API. o Install a driver. o Install your DBMS if needed. **Loading Drivers** · To manually load the database driver and register it with the DriverManager, load its class file. Class.forName(\) · For JDBC--ODBC Bridge driver, the following code will load it: Class.forName(\"sun.jdbc.odbc.JdbcOdbcD river\"); or new sun.jdbc.odbc.JdbcOdbcDriver(); **Making the Connection** · The second step is to create a Connection object. Connection con = DriverManager.getConnection( url, \"myLogin\", \"myPassword\"); Example String url = \"jdbc:odbc:adprog1\"; Connection con = DriverManager.getConnection(url, \"myLogin\", \"passwd\"); · Methods that you can use in creating a database connection: java.sql.DriverManager o getConnection(String url, String user, String password) throws SQLException · java.sql.Connection o Statement createStatement() throws SQLException void close() throws SQLException void setAutoCommit(boolean b) throws SQLException void commit() throws SQLException void rollback() throws SQLException **Creating JDBC Statements** · A Statement object is what sends your SQL statement to the DBMS. · The Connection object instance is used to create the Statement object stmt: Statement stmt = con.createStatement(); · For a SELECT statement, the method to use is executeQuery. · For statements that create or modify tables, the method to use is executeUpdate. Example Statement stmt = con.createStatement(); stmt.executeUpdate(\"INSERT INTO PRODUCTS \" + \"VALUES ('P00001\', 101, 7.99, 0, 0)\"); **Retrieving Values from Result Sets** · The JDBC API returns results in a ResultSet object. ResultSet rs = stmt.executeQuery(\"SELECT PROD\_NAME, PRICE FROM PRODUCTS\"); ** **