Java I/O Files and Packages PDF
Document Details
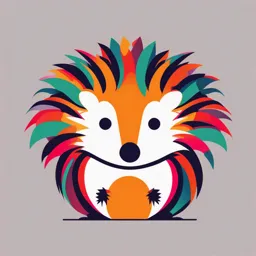
Uploaded by ImpressiveAsh1202
Tags
Summary
This document provides information about packages, streams, and file handling in Java programming, along with examples using various I/O classes and methods.
Full Transcript
MODULE III Input/ Output Input/ Output Package java.io, which supports Java’s basic I/O (input/output) system, including file I/O. Streams A stream is a sequence of data. Java uses the concept of a stream to make I/O operation fast. The java.io package contains all the classes required f...
MODULE III Input/ Output Input/ Output Package java.io, which supports Java’s basic I/O (input/output) system, including file I/O. Streams A stream is a sequence of data. Java uses the concept of a stream to make I/O operation fast. The java.io package contains all the classes required for input and output operations. Java programs perform I/O through streams. The Stream Classes Java’s stream-based I/O is built upon four abstract classes: InputStream // designed for byte streams. OutputStream // designed for byte streams. Reader // are designed for character streams. Writer //are designed for character streams. The byte stream classes and the character stream classes form separate hierarchies. Use the character stream classes when working with characters or strings. Use the byte stream classes when working with bytes or other binary objects. Java FILE class methods Byte stream classes Character stream classes File Reader class methods All Methods throws IOException Reading all data using char[] array buffer Reading and Writing to Files - using Byte Stream FileInputStream read() FileOutputStream write() File input stream methods Reading using FileInputStream File output stream methods Writing to files using FileOutputStream Reading all data using byte[] array buffer BufferedReader Java BufferedReader class is used to read the text from a character- based input stream. It can be used to read data line by line by readLine() method. It makes the performance fast. FileReader fr=new FileReader("D:/testout.txt"); BufferedReader br=new BufferedReader(fr); Reading line by line using BufferedReader BufferedWriter Java BufferedWriter class is used to provide buffering for Writer instances. It makes the performance fast. The buffering characters are used for providing the efficient writing of single arrays, characters, and strings. It provides additional method for writing “newline” and appending data. newLine() append() BufferedWriter – Example The Predefined Streams As you know, all Java programs automatically import the java.lang package. This package defines a class called System System also contains three predefined stream variables: in, out, and err. These fields are declared as public, static, and final within System. This means that they can be used with class name “System”. System.out refers to the standard output stream. By default, this is the console. System.in refers to standard input, which is the keyboard by default. System.err refers to the standard error stream, which also is the console by default. Eg. System.err.println("File opening failed:"); System.in is an object of type InputStream; System.out and System.err are objects of type PrintStream. These are byte streams, even though they are typically used to read and write characters from and to the console Reading Console Input 1. Using Scanner class Converts byte stream to character stream automatically Also, provides convenient methods to read proper data such as int, byte, long, short, float, double, boolean. Reading Console Input 2. Using BufferedReader to read console Input. This method is used by wrapping the System.in (standard input stream) in an InputStreamReader which is wrapped in a BufferedReader, we can read input from the user in the command line. Reading Console Input 3. Using Console Class It has been becoming a preferred way for reading user’s input from the command line. In addition, it can be used for reading password-like input without echoing the characters entered by the user; the format string syntax can also be used (like System.out.printf()). Advantages: Reading password without echoing the entered characters. Format string syntax can be used. Drawback: Does not work in non-interactive environment (such asin an IDE). The java string valueOf() method converts different types of values into string. By the help of string valueOf() method, you can convert int to string, long to string, boolean to string, character to string, float to string, double to string, object to string and char array to string. class ReadPasswordTest { public static void main(String args[]) { System.out.println("Enter password: "); char[] ch=System.console().readPassword(); String pass=String.valueOf(ch);//converting char array into string System.out.println("Password is: "+pass); } } WRITING CONSOLE OUTPUT Console output is most easily accomplished with print() and println() methods. These methods are defined by the class PrintStream which is the type of object referenced by System.out. Because the PrintStream is an output stream derived from the OutputStream, it also implements the low-level method write(). Thus, write() can be used to write to the console. Writing Console Output System.out – is a object of PrintStream System.out.println() System.out.print() System.out.write() Writes the bytes to output console PrintWriter It is used to print the formatted representation of data to the textoutput stream. write() print() println() PrintWriter CLASS For real-world programs, the recommended method of writing to the console when using Java is through a PrintWriter stream. PrintWriter is one of the character-based classes. Using a character based class for console output makes internationalizing your program easier. PrintWriter(OutputStream outputStream, boolean flushingOn) PrintWriter supports the print( ) and println( ) methods. To write to the console by using a PrintWriter, specify System.out for the output stream and automatic flushing. PrintWriter pw = new PrintWriter(System.out, true); PrintWriter – example to write console output System.out.write() It is used to write byte data in a console. FILE COPY PROGRAM Each time read() is called, it reads a single byte from the file and returns the byte as an integer value. read( ) returns –1 when an attempt is made to read at the end of the stream. I/O Exceptions Two exceptions play an important role in I/O handling. 1. IOException if an I/O error occurs, an IOException is thrown. Eg. EndOfStreamException // Unable to read beyond the end of the stream FileNotFoundException, DirectoryNotFoundException // Could not find a part of the path 2. FileNotFoundException if a file cannot be opened, a FileNotFoundException is thrown. It is a subclass of IOException, so both can be caught with a single catch that catches IOException. Serialization Serialization is the process of writing the state of an object to a byte stream. Useful when you want to save the state of your program to a persistent storage area, such as a file. Serialization is also needed to implement Remote Method Invocation (RMI). RMI allows a Java object on one machine to invoke a method of a Java object on a different machine. An object may be supplied as an argument to that remote method. The sending machine serializes the object and transmits it. The receiving machine deserializes it. Serialization is the process of converting the java code object into a byte stream, to transfer the object code from one java virtual machine to another and recreate it using the process of Deserialization. for serializing the object, we call the writeObject() method of ObjectOutputStream class for deserialization we call the readObject() method of ObjectInputStream class. Serializable Only an object that implements the Serializable interface can be saved and restored by the serialization facilities. The Serializable interface defines no members. It is simply used to indicate that a class may be serialized. If a class is serializable, all of its subclasses are also serializable. Why do we need Java Serialization? Serialization allows us to transfer objects through a network by converting it into a byte stream. It also helps in preserving the state of the object. Deserialization requires less time to create an object than an actual object created from a class. hence serialization saves time. One can easily clone the object by serializing it into byte streams and then deserializing it. Serialization helps to implement persistence in the program. It helps in storing the object directly in a database in the form of byte streams. This is useful as the data is easily retrievable whenever needed. Cross JVM Synchronization – It works across different JVM that might be running on different architectures(OS) Object Streams and Serialization Classes ObjectInputStream and ObjectOutputStream, which respectively implement the ObjectInput and ObjectOutput interfaces, enable entire objects to be read from or written to a stream. To use serialization with files, initialize ObjectInputStream and ObjectOutputStream objects with FileInputStream and FileOutputStream objects. ObjectOutput interface method writeObject() takes an Object as an argument and writes its information to an OutputStream. A class that implements ObjectOuput (such as ObjectOutputStream) declares this method and ensures that the object being output implements Serializable. ObjectInput interface method readObject() reads and returns a reference to an Object from an InputStream. After an object has been read, its reference can be cast to the object’s actual type. public final void writeObject(Object x) throws IOException public final Object readObject() throws IOException, ClassNotFoundException A class must implement java.io.Serializable interface to be eligible for serialization. Object serialization example Package in Java A java package is a group of similar types of classes, interfaces and sub-packages. Package in java can be categorized in two form, built-in package user-defined package. There are many built-in packages such as java, lang, awt,javax, swing, net, io, util, sql etc. Advantage of Java Package 1) Java package is used to categorize the classes and interfaces so that they can be easily maintained. 2) Java package provides access protection. 3) Java package removes naming collision. Creating packages “package” statement is used to create java packages. It must be the first line of the program. There must be only one package statement. Accessing Package “import” statement is used to include the package you want to access. There are two ways to include the package. Using fully qualified class name Ex. import Math.BasicMath; Using * to include all the classes Ex import Math.*; Example Example Example Assume there are three packages. Math package which has BasicMath class. Shape package which has Rectangle class Test package which has Main class to access the Math and Shape functionalities. Example Access Protection in Package Packages defines another levels of access protection. In java, there are 4 access specifiers private – only inside same class public - universal access protected - same package level & only for sub class of different package Default - only in same package. Here, public, protected and default plays a vital role with packages. Access Protection Package addresses five categories of visibility for class members: Access Protection:- Same class Members of the same class can access all the data including private data members Access Protection:- Same Package Sub Classes Access Protection:- Same Package Non-Sub Classes Access Protection:- Different Package Sub Classes