BSc (Hons) Electronics Ist Sem - Programming Fundamentals using Python PDF
Document Details
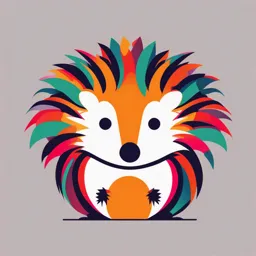
Uploaded by DauntlessFlashback
Department of Electronic Science
Tags
Related
- TK2053 Programming Paradigm - Python Interpreter PDF
- Python Programming Fundamentals PDF
- Python Programming Quiz Questions PDF
- SDU CSS 115 Programming Fundamentals 1 (Python) Fall 2024 Practice 4 PDF
- CSS 115 - Programming Fundamentals 1 (Python) Fall 2024 Lecture 5 PDF
- Cours Python - Programmations: Concepts Fondamentaux - Pratique (PDF)
Summary
This document provides a syllabus for an introductory Python programming course in a bachelor's degree program. It covers basic Python concepts like data types, operators, and control structures, and also introduces object-oriented programming concepts.
Full Transcript
# Department of Electronic Sciences ## BSc. (Hons.) Electronic Sciences ### Category-I ## CREDIT DISTRIBUTION, ELIGIBILITY AND PRE-REQUISITES OF THE COURSE | Course title & Code | Credits | Credit distribution of the course | Eligibility criteria | Pre-requisite of the course (if any) | |---|---|...
# Department of Electronic Sciences ## BSc. (Hons.) Electronic Sciences ### Category-I ## CREDIT DISTRIBUTION, ELIGIBILITY AND PRE-REQUISITES OF THE COURSE | Course title & Code | Credits | Credit distribution of the course | Eligibility criteria | Pre-requisite of the course (if any) | |---|---|---|---|---| | Programming Fundamentals using Python ELDSC-1 | 4 | Lecture: 3 Tutorial: 0 Practical/ Practice: 1 | Course Admission Eligibility | Nil | ### Learning Objectives The Learning Objectives of this course are as follows: - This course introduces the student to the fundamental understanding of the Python programming language - The main objective is to help students learn to use the Python programming language to solve problems of interest to them - It introduces the core programming basics including data types, operators, input/output, control structures, iterative and recursive constructs, compound data types, and program design with functions - The course also discusses the fundamental principles of Object-Oriented Programming (OOP), as well as comprehensive data and information processing technique ### Learning outcomes The Learning Outcomes of this course are as follows: - **CO1:** Read, write and debug python programs to solve computational problems. - **CO2:** Select and use a suitable programming construct and data objects like lists, sets, tuples and dictionaries for solving a given problem. - **CO3:** Be proficient in the handling of strings and functions - **CO4:** Use Python libraries - **CO5:** Articulate OOP concepts such as encapsulation, inheritance and polymorphism and use them in applications ## SYLLABUS OF DSC-1 - **UNIT - I Starting with Python (12 Hours)** - Introduction to Python: Python Interpreter-IDLE (script and interactive mode), Python shell, using Python as calculator, concept of data types; variables, Identifiers and keywords, Literals, Strings, Operators (Arithmetic operator, Relational operator, Logical operator, Boolean operator, Assignment operators, Membership operators(in and not in), Identity operators, Bit wise operator, Increment or Decrement operator), comments in the program, understanding error messages. - Creation of a Python Program: Input and Output Statements, Control statements -Branching (if-else, if-elif-else), indentation in python, iteration (using for, while), Conditional Statement, exit function, Difference between break, continue and pass, Nested conditionals - **UNIT - II Strings and Lists (12 Hours)** - Data objects in Python: Mutable and immutable - Strings- Creating and Storing Strings, Accessing Characters in String by Indexing (positive and negative), String Operations: concatenation, replication (*), membership, comparison, Slicing, string built-in functions, String method - Lists- Creating Lists, Accessing list elements, traversing a list, Aliasing a list, comparing list, list Operations:- concatenation, replication(*), membership, slicing, Indexing, nested list, list built-in functions List methods, del statement. - Sets: Creating sets, Sets built-in functions, Set Methods - **UNIT - III Tuples and Dictionaries (12 Hours)** - Tuples: Creating Tuples, Tuple operations: slicing, concatenation, replication, membership, comparing and deletion, tuple built-in functions - Dictionaries: Dictionary in python (key: value pairs), creating a dictionary, element accessing and traversing a dictionary, appending values, updating values, removing items from dictionary, membership, dictionary built-in functions, dictionary methods, clear statement - Object Oriented Programming: Introduction to Classes, Objects and Methods, Encapsulation, Inheritance, Polymorphism, Abstraction - **UNIT - IV Functions and Modules (12 Hours)** - Functions: Built in function (math, statistics), User defined functions: Defining Functions, arguments: positional, default, keyword, variable length arguments, scope of variables, parameter passing (string list, dictionary, tuples, sets), return statement, recursion, importing (using import) user defined function (path). - Modules in python: use of keyword from, namespacing, module aliasing, introduction to python packages (matplotlib, pandas, numpy, scikitlearn, nltk, openCV) and libraries and their applications - **Practical component (if any) - Programming Fundamentals using Python Lab (30 Hours)** - **Learning outcomes** - **CO1:** Develop algorithms and write programs in Python language for arithmetic and logical operations, conditional branching. - **CO2:** Write programs in Python language using construct and data objects like strings, lists, sets, tuples, dictionaries, Python libraries and use concept of OOP. - **CO3:** Prepare the technical report on the experiments carried. ## Essential/recommended readings 1. Allen B. Downey, "Think Python: How to Think Like a Computer Scientist", 2nd edition, Updated for Python 3, Shroff/O'Reilly Publishers, 2016 (http://greenteapress.com/wp/thinkpython/) 2. Guido van Rossum and Fred L. Drake Jr, -An Introduction to Python - Revised and updated for Python 3.2, Network Theory Ltd., 2011. 3. John V Guttag, -Introduction to Computation and Programming Using Python“, Revised and expanded Edition, MIT Press, 2013 4. Robert Sedgewick, Kevin Wayne, Robert Dondero, -Introduction to Programming in Python: An Inter-disciplinary Approach, Pearson India Education Services Pvt. Ltd., 2016. 5. Timothy A. Budd, -Exploring Pythonll, Mc-Graw Hill Education (India) Private Ltd., 2015. ## Suggestive readings 1. Kenneth A. Lambert, -Fundamentals of Python: First Programsll, CENGAGE Learning, 2012. 2. Charles Dierbach, -Introduction to Computer Science using Python: A Computational Problem-Solving Focus, Wiley India Edition, 2013. 3. Paul Gries, Jennifer Campbell and Jason Montojo, -Practical Programming: An Introduction to Computer Science using Python 311, Second edition, Pragmatic Programmers, LLC, 2013. Note: Examination scheme and mode shall be as prescribed by the Examination Branch, University of Delhi, from time to time. # DISCIPLINE SPECIFIC CORE COURSE – 2 (DSC-2): Circuit Theory & Network Analysis ## Credit distribution, Eligibility and Prerequisites of the Course | Course title & Code | Credits | Credit distribution of the course | Eligibility criteria | Pre-requisite of the course (if any) | |---|---|---|---|---| | Circuit Theory & Network Analysis ELDSC-2 | 4 | Lecture: 3 Tutorial: 0 Practical/ Practice: 1 | Course Admission Eligibility | Nil | ### Learning Objectives The Learning Objectives of this course are as follows: - To study the basic circuit concepts in a systematic manner suitable for analysis and design. - To study the steady state analysis of AC Circuits. - To study and analyse electric circuits using network theorems. - To study and design passive filters using R, L and C ### Learning outcomes The Learning Outcomes of this course are as follows: - **CO1:** Study basic circuit concepts in a systematic manner suitable for analysis and design. - **CO2:** Determine AC steady state response. - **CO3:** Analyse the electric circuits using network theorems. - **CO4:** Determine frequency response of filters ## SYLLABUS OF DSC-2 - **UNIT - I Introduction to Circuits and DC Analysis (12 Hours)** - Basic Circuit Concepts: Voltage and Current Sources, V- I characteristics of ideal voltage and ideal current sources, various types of controlled sources, passive circuit components, V-I characteristics, and ratings of different types of R, L, C elements. - DC Circuit Analysis: Kirchhoff's Current Law (KCL), Kirchhoff's Voltage Law (KVL), Node Analysis, Mesh Analysis, Super node & Super mesh Analysis, Star-Delta Conversion. - **UNIT – II AC Analysis (12 Hours)** - Steady State Analysis: Sinusoidal Voltage and Current, Definition of Instantaneous, Peak, Peak to Peak, Root Mean Square and Average Values. Phasor, Complex Impedance, Sinusoidal Circuit Analysis for RL, RC and RLC Circuits. Node and Mesh Analysis for AC circuits. Star-Delta Conversion for complex impedances. - Power in AC Circuits: Instantaneous Power, Average Power, Reactive Power, Power Factor. - **UNIT - III Network Theorems (12 Hours)** - Network Theorems: Principal of Duality, Superposition Theorem, Thevenin's Theorem, Norton's Theorem, Reciprocity Theorem, Millman's Theorem, Maximum Power Transfer Theorem. (Independent Sources) - AC circuit analysis using Network Theorems. - **UNIT - IV Filters (9 Hours)** - Filters and Resonance: Introduction to Passive Filters-High Pass, Low Pass, Band Pass & Band Stop Filters, Frequency response of RC Circuits-High pass and Low pass filters, Frequency response of Series and Parallel RLC Circuits. Resonance in Series and Parallel RLC Circuits, Quality (Q) Factor and Bandwidth, Band Pass and Band Stop RLC Filters. - **Practical component (if any) - Circuit Theory and Network Analysis Lab (Hardware and Circuit Simulation Software) (30 Hours)** - **Learning outcomes** - **CO1:** Verify the network theorems and operation of typical electrical circuits. - **CO2:** Choose the appropriate equipment for measuring electrical quantities and verify the same for different circuits. - **CO3:** Prepare the technical report on the experiments carried. - 1. Familiarization with Multimeter: Resistance, Capacitor and Inductor in series, parallel and series-parallel. - 2. Familiarization with Oscilloscope: Measurement of Amplitude, Frequency and phase of a sinusoidal signal - 3. Verification of Kirchhoff's Current Law. - 4. Verification of Kirchhoff's Voltage Law - 5. Verification of Norton's theorem. - 6. Verification of Thevenin's Theorem. - 7. Verification of Superposition Theorem. - 8. Verification of the Maximum Power Transfer Theorem. - 9. Design of Low Pass RC Filter and study of its Frequency Response. - 10. Design of High Pass RC Filter and study of its Frequency Response. - 11. Study of Frequency Response of a Series LCR Circuit and determination of its (a) Resonant Frequency (b) Impedance at Resonance (c) Quality Factor Q (d) Band Width. Note: Students shall sincerely work towards completing all the above listed practicals for this course. In any circumstance, the completed number of practicals shall not be less than nine. ## Essential/recommended readings 1. S. A. Nasar, Electric Circuits, Schaum's outline series, Tata McGraw Hill (2004) 2. M. Nahvi and J. Edminister, Electrical Circuits, Schaum's Outline Series, Tata McGraw Hill.(2005) 3. Robert L. Boylestad, Essentials of Circuit Analysis, Pearson Education (2004) ## Suggestive readings (if any) 1. Alexander and M. Sadiku, Fundamentals of Electric Circuits, McGraw Hill (2008) # DISCIPLINE SPECIFIC CORE COURSE-3 (DSC-3): Semiconductor Devices ## Credit distribution, Eligibility and Pre-requisites of the Course | Course title & Code | Credits | Credit distribution of the course | Eligibility criteria | Pre-requisite of the course (if any) | |---|---|---|---|---| | Semiconductor Devices ELDSC-3 | 4 | Lecture: 3 Tutorial: 0 Practical/ Practice: 1 | Course Admission Eligibility | Nil | ### Learning Objectives The Learning Objectives of this course are as follows: - To understand the Physics of semiconductor devices - To be able to plot and interpret the current voltage characteristics for basic semiconductor devices - The student should be able to understand the behaviour, characteristics and applications of power devices such as SCR, UJT, DIAC, TRIAC, IGBT ### Learning outcomes The Learning Outcomes of this course are as follows: - **CO1:** Describe the behavior of semiconductor materials - **CO2:** Reproduce the I-V characteristics of diode/BJT/MOSFET devices - **CO3:** Apply standard device models to explain/calculate critical internal parameters of semiconductor devices - **CO4:** Explain the behavior and characteristics of power devices such as SCR/UJT etc. ## SYLLABUS OF DSC-3 - **UNIT - I Introduction to Semiconductors and Carrier Transport (12 Hours)** - Basic Concepts of Semiconductors: Energy Bands in Solids, Concept of Effective Mass, Direct and Indirect Bandgap Semiconductors, Density of States (Qualitative understanding), Carrier Concentration at Normal Equilibrium in Intrinsic Semiconductors and its Temperature Dependence, Derivation of Fermi Level for Intrinsic and Extrinsic Semiconductors and its Dependence on Temperature and Doping Concentration - Carrier Transport Phenomena: Drift velocity, Mobility, Resistivity, Hall Effect Conductivity, Diffusion Process, Einstein Relation, Current Density Equation, Carrier Injection, Generation and Recombination Processes (Qualitative concepts), Continuity Equation. - **UNIT - II P-N Junction Devices (12 Hours)** - P-N Junction Diode: Space Charge at a Junction, Depletion Layer, Electrostatic Potential Difference at Thermal Equilibrium, Depletion Width and Depletion Capacitance of an Abrupt Junction. Concept of Linearly Graded Junction - Diode Equation and I-V Characteristics (Qualitative), Zener and Avalanche breakdown Mechanism. - Metal Semiconductor Junctions, Ohmic and Rectifying Contacts, Zener diode, Tunnel diode, Varactor Diode, Optoelectronic Devices: LED, Photodiode, Solar cell, LDR, their Circuit Symbols, Characteristics and Applications - **UNIT - III Bipolar Junction Transistors (12 Hours)** - Bipolar Junction Transistors (BJT): PNP and NPN Transistors, Energy Band Diagram of Transistor in Thermal Equilibrium, Emitter Efficiency, Base Transport Factor, Current Gain, Relation between alpha and beta, Base-Width Modulation, Early Effect, Modes of operation, Input and Output Characteristics of CB, CE and CC Configurations and their Applications. - **UNIT - IV FET and Power Devices (9 Hours)** - Field Effect Transistors: JFET, Channel Formation, Pinch-Off and Saturation Voltage, Input, Transfer and Output Characteristics. - MOSFET, NMOS, PMOS, Types of MOSFET, Circuit symbols, Working and Characteristic Curves of Depletion mode and Enhancement mode MOSFET (both N channel and P Channel), Complimentary MOS (CMOS) as an Inverter. - Power Devices: Introduction to UJT, SCR, TRIAC, DIAC, IGBT and their Basic Constructional Features (Schematic Diagram), Characteristics and Applications. ## Practical component (if any) - Semiconductor Devices Lab (30 Hours) (Hardware and Circuit Simulation Software) ### Learning outcomes - **CO1:** Examine the characteristics of Semiconductor Devices - **CO2:** Perform experiments for studying the behaviour of semiconductor devices for circuit design applications - **CO3:** Calculate various device parameters values from their I-V Characteristics - **CO4:** Interpret the experimental data for better understanding of the device behaviour - 1. Study of the I-V Characteristics of Diode - Ordinary and Zener, Solar Cell, Photodiode - 2. Study of the I-V Characteristics of the CE, CB and CC configurations of BJT and obtain Input and Output impedances and Gains (Any one configuration to be assigned at the time of Examination) - 3. Study of the I-V Characteristics of JFET/MOSFET - 4. Study of the I-V Characteristics of the UJT - 5. Study of the I-V Characteristics of the SCR - 6. Study of the I-V Characteristics of DIAC and TRIAC - 7. Study of Hall Effect. Note: Students shall sincerely work towards completing all the above listed practicals for this course. In any circumstance, the completed number of practicals shall not be less than six. ## Essential/recommended readings 1. S.M Sze Semiconductor Devices: Physics and Technology, 2nd Edition, Wiley India Edition 2. Ben G Streetman and S. Banerjee Solid State Electronic Devices, Pearson Education 3. Dennis Le Croissette, Transistors, Pearson Education 4. Jacob Millman and Christos Halkias: Electronic Devices and Circuits, Tata McGraw-Hill Edition ## Suggestive readings 1. Nutan Kala Joshi and Swati Nagpal, Basic Electronics with Simulations and Experiments, Khanna Publishers (2021) 2. Jasprit Singh, Semiconductor Devices: Basic Principles, John Wiley and Sons 3. Kannan Kano, Semiconductor Devices, Pearson Education