The .NET Platform PDF
Document Details
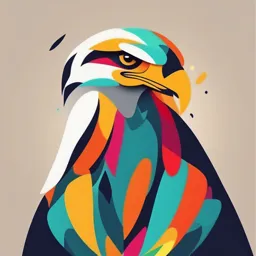
Uploaded by BeautifulIrrational
Tags
Summary
This document provides an overview of the .NET platform, covering its key components such as the Common Language Runtime (CLR), the .NET Framework Class Library (FCL), and the Common Type System (CTS). The document also touches on language integration within .NET, including cross-language features, and highlights how the .NET platform ensures language interoperability through the CLS.
Full Transcript
1 The.NET Platform Overview: Cross-Platform:.NET runs on multiple operating systems, including Windows, macOS, and Linux. Open Source:.NET is developed by Microsoft with contributions from the open- source community. Unified Platform: Combines various frameworks into a single p...
1 The.NET Platform Overview: Cross-Platform:.NET runs on multiple operating systems, including Windows, macOS, and Linux. Open Source:.NET is developed by Microsoft with contributions from the open- source community. Unified Platform: Combines various frameworks into a single platform for building different types of applications. It contains languages, compilers, runtime environments, libraries and tools. Various application types: It is an ecosystem that encompasses a wide range of technologies and tools for building various types of applications, such as desktop, web, mobile, and gaming applications. A common runtime engine shared by all.NET languages: One aspect of this engine is a well-defined set of types that each.NET language understands. Programmatically speaking, the term runtime can be understood as a collection of services that are required to execute a given compiled unit of code. For example, when Java developers deploy software to a new computer, they need to ensure the Java virtual machine (JVM) has been installed on the machine in order to run their software. The.NET platform offers yet another runtime system. The key difference between the.NET runtime and the various other runtimes I just mentioned is that the.NET runtime provides a single runtime layer that is shared by all languages and platforms that are.NET. Language integration:.NET supports cross-language inheritance, cross-language exception handling, and cross-language debugging of code. For example, you can define a base class in C# and extend this type in Visual Basic. Key Components: From a programmer’s point of view,.NET can be understood as a runtime environment and a comprehensive base class library. The runtime layer contains the set of minimal implementations that are tied specifically to a platform (Windows, iOS, Linux) and architecture (x86, x64, ARM), as well as all of the base types for.NET. 1 Common Language Runtime (CLR): o Role: Executes and manages the code written in.NET languages. o Services: Includes memory management, security enforcement (implements Code Access Security (CAS) and role-based security), exception handling, and garbage collection, language interoperability. o Just-In-Time Compilation: The CLR includes a Just-In-Time (JIT) compiler that converts Intermediate Language (IL) code into machine code that can be executed by the host operating system..NET Framework Class Library (FCL): o Base Class Library (BCL): Includes fundamental classes like System, System.Collections, System.IO, System.Net, System.Security, System.Text, etc. o System Namespace: Provides fundamental classes and base classes for other namespaces, such as System.String, System.DateTime, and System.Console. ▫ System.Collections: Provides interfaces and classes that define various collections of objects, such as lists, dictionaries, and queues. ▫ System.IO: Provides classes for synchronous and asynchronous reading and writing of data streams and files. ▫ System.Net: Provides a simple programming interface for many of the protocols used on networks, such as HTTP and FTP. ▫ System.Security: Provides the underlying structure of the CLR security system, including permissions, cryptography, and secure communications. ▫ System.Text: Provides classes representing ASCII, Unicode, and other encodings. Common Type System (CTS): Standardizes data types across different.NET languages, ensuring language interoperability. o Every.NET language supports, at the least, the notion of a class type required for object-oriented programming (OOP). In C#, classes are declared using the class keyword, like so: 2 // A C# class type with 1 method. class Calc { public int Add(int addend1, int addend2) { return addend1 + addend2; } } o CTS Interface Types Interfaces are nothing more than a named collection of abstract member definitions and/or (introduced in C# 8) default implementations, which are implemented (optionally in the case of default implementations) by a given class or structure. In C#, interface types are defined using the interface keyword. By convention, all.NET interfaces begin with a capital letter I, as in the following example: // A C# interface type is usually // declared as public, to allow types in other // assemblies to implement their behavior. public interface IDraw { 3 void Draw(); } On their own, interfaces are of little use. However, when a class or structure implements a given interface in its unique way, you are able to request access to the supplied functionality using an interface reference in a polymorphic manner. o CTS Structure Types A structure can be thought of as a lightweight class type having value-based semantics. Typically, structures are best suited for modeling geometric and mathematical data and are created in C# using the struct keyword, as follows: struct Point { // Structures can contain fields. public int xPos, yPos; // Structures can contain parameterized constructors. public Point(int x, int y) { xPos = x; yPos = y;} // Structures may define methods. public void PrintPosition() { Console.WriteLine("({0}, {1})", xPos, yPos); } } o CTS Enumeration Types 4 Enumerations are a handy programming construct that allow you to group name- value pairs. For example, assume you are creating a video game application that allows the player to select from three character categories (Wizard, Fighter, or Thief ). Rather than keeping track of simple numerical values to represent each possibility, you could build a strongly typed enumeration using the enum keyword. enum CharacterTypeEnum { Wizard = 100, Fighter = 200, Thief = 300 } The CTS demands that enumerated types derive from a common base class, System.Enum. o CTS Delegate Types Delegates are the.NET equivalent of a type-safe, C-style function pointer. The key difference is that a.NET delegate is a class that derives from System.MulticastDelegate, rather than a simple pointer to a raw memory address. In C#, delegates are declared using the delegate keyword. // This C# delegate type can "point to" any method // returning an int and taking two ints as input. delegate int BinaryOp(int x, int y); Delegates are critical when you want to provide a way for one object to forward a call to another object and provide the foundation for the.NET event architecture. Delegates have intrinsic support for multicasting (i.e., forwarding a request to 5 multiple recipients) and asynchronous method invocations (i.e., invoking the method on a secondary thread). o CTS Type Members Now that you have previewed each of the types formalized by the CTS, realize that most types take any number of members. Formally speaking, a type member is constrained by the set {constructor, finalizer, static constructor, nested type, operator, method, property, indexer, field, read-only field, constant, event}. The CTS defines various adornments that may be associated with a given member. For example, each member has a given visibility trait (e.g., public, private, protected). Some members may be declared as abstract (to enforce a polymorphic behavior on derived types) as well as virtual (to define a canned, but overridable, implementation). Also, most members may be configured as static (bound at the class level) or instance (bound at the object level). o Intrinsic CTS Data Types Although a given language typically has a unique keyword used to declare a fundamental data type, all.NET language keywords ultimately resolve to the same CTS type defined in an assembly named mscorlib.dll. Consider Table 1-2, which documents how key CTS data types are expressed in VB.NET and C#. 6 o Common Language Specification (CLS): Defines a subset of CTS that all.NET languages must support to ensure interoperability. For example, in C# you denote string concatenation using the plus operator (+), while in VB you typically make use of the ampersand (&). Even when two distinct languages express the same programmatic idiom (e.g., a function with no return value), the syntax will appear quite different on the surface. public void MyMethod() { // Some interesting code... } 7 ' VB method returning nothing. Public Sub MyMethod() ' Some interesting code... End Sub Given that the respective compilers (csc.exe or vbc.exe, in this case) emit a similar set of CIL (Common Intermediate Language) instructions. However, languages can also differ with regard to their overall level of functionality. For example, a.NET language might or might not have a keyword to represent unsigned data and might or might not support pointer types. Given these possible variations, it would be ideal to have a baseline to which all.NET languages are expected to conform. The CLS is a set of rules that describe in detail the minimal and complete set of features a given.NET compiler must support to produce code that can be hosted by the.NET Runtime, while at the same time be accessed in a uniform manner by all languages that target the.NET platform. In many ways, the CLS can be viewed as a subset of the full functionality defined by the CTS. The CLS is ultimately a set of rules that compiler builders must conform to if they intend their products to function seamlessly within the.NET universe. Each rule is assigned a simple name (e.g., CLS Rule 6) and describes how this rule affects those who build the compilers as well as those who (in some way) interact with them. Rule 1 of CLS is: CLS rules apply only to those parts of a type that are exposed outside the defining assembly. Given this rule, you can infer that the remaining rules of the CLS do not apply to the logic used to build the inner workings of a.NET type. The only aspects of a type that must conform to the CLS are the member definitions themselves (i.e., naming conventions, parameters, and return types). The implementation logic for a member may use any number of non-CLS techniques, as the outside world won’t know the difference. Ensuring CLS Compliance C# does define a number of programming constructs that are not CLS compliant. The good news, however, is that you can instruct the C# compiler to check your code for CLS compliance using a single.NET attribute. 8 // Tell the C# compiler to check for CLS compliance. [assembly: CLSCompliant(true)] Web Application Development: ASP.NET framework for building dynamic web applications. ADO.NET: Provides components for data access and manipulation. Managed vs. Unmanaged Code The C# language can be used only to build software that is hosted under the.NET runtime (you could never use C# to build a native COM server or an unmanaged C/C++-style application). The term used to describe the code targeting the.NET runtime is managed code. The binary unit that contains the managed code is termed an assembly. Conversely, code that cannot be directly hosted by the.NET runtime is termed unmanaged code. The.NET platform can run on a variety of operating systems. It is quite possible to build a C# application on a Windows machine and run the program on an iOS machine using the.NET runtime. As well, you can build a C# application on Linux using Visual Studio Code and run the program on Windows. With Visual Studio for Mac, you can also build.NET applications on a Mac to be run on Windows, macOS, or Linux. Unmanaged code can still be accessed from a C# program, but it then locks you into a specific development and deployment target. Assemblies Assemblies are the building blocks of.NET applications. They are compiled code that can be executed by the CLR and have a.dll or.exe file extension..NET assemblies do not contain platform-specific instructions but rather platform-agnostic Intermediate Language (IL) and type metadata (IL is also known as Microsoft Intermediate Language (MSIL) or alternatively as the Common Intermediate Language (CIL). Thus, as you read the.NET literature, understand that IL, MSIL, and CIL are all describing essentially the same concept). 9 Unlike.NET Framework assemblies that can be either a *.dll or *.exe,.NET Core projects are always compiled to a file with a.dll extension, even if the project is an executable. Executable.NET assemblies are executed with the command dotnet.dll. New in.NET Core 3.0 (and later), the dotnet.exe command is copied to the build directory and renamed to.exe. Running this command automatically calls the dotnet.dll file, executing the equivalent of dotnet.dll. The *.exe with your project name isn’t actually your project’s code; it is a convenient shortcut to running your application. Updated in.NET 6, your application can be reduced to a single file that is executed directly. Even though this single file looks and acts like a C++-style native executable, the single file is a packaging convenience. It contains all the files needed to run your application, potentially even the.NET runtime itself! An assembly contains CIL code, which is conceptually similar to Java bytecode, in that it is not compiled to platform-specific instructions until absolutely necessary. Typically, “absolutely necessary” is the point at which a block of CIL instructions (such as a method implementation) is referenced for use by the.NET runtime. Assemblies also contain metadata that describes in detail the characteristics of every “type” within the binary. For example, if you have a class named SportsCar, the type metadata describes details such as SportsCar’s base class, specifies which interfaces are implemented by SportsCar (if any), and gives full descriptions of each member supported by the SportsCar type..NET metadata is always present within an assembly and is automatically generated by the language compiler. In addition to CIL and type metadata, assemblies themselves are also described using metadata, which is officially termed a manifest. The manifest contains information about the current version of the assembly, culture information (used for localizing string and image resources), and a list of all externally referenced assemblies that are required for proper execution. Metadata is used by numerous aspects of the.NET runtime environment, as well as by various development tools. For example, the IntelliSense feature provided by tools such as Visual Studio is made possible by reading an assembly’s metadata at design time. Metadata is also used by various object-browsing utilities, debugging tools, and the C# compiler itself. To be sure, metadata is the backbone of numerous.NET technologies including reflection, late binding, and object serialization. A.NET assembly also contains metadata that describes the assembly itself (technically termed a manifest). Among other details, the manifest documents all external 10 assemblies required by the current assembly to function correctly, the assembly’s version number, copyright information, and so forth. Like type metadata, it is always the job of the compiler to generate the assembly’s manifest. Types of Assemblies: o Private Assemblies: Used by a single application. o Shared Assemblies: Designed to be used by multiple applications. Intermediate Language (IL) Code compiled from high-level languages is converted into an intermediate language before execution. One benefit is language integration. Each.NET compiler produces nearly identical CIL instructions. Therefore, all languages are able to interact within a well-defined binary arena. Given that CIL is platform-agnostic, the.NET Framework itself is platform-agnostic, providing the same benefits Java developers have grown accustomed to (e.g., a single code base running on numerous operating systems). Because assemblies contain CIL instructions rather than platform-specific instructions, CIL code must be compiled on the fly before use. The entity that compiles CIL code into meaningful CPU instructions is a JIT compiler, which sometimes goes by the friendly name of jitter. The.NET runtime environment leverages a JIT compiler for each CPU targeting the runtime, each optimized for the underlying platform. For example, if you are building a.NET application to be deployed to a handheld device (such as an iOS or Android phone), the corresponding jitter is well equipped to run within a low-memory environment. On the other hand, if you are deploying your assembly to a back-end company server (where memory is seldom an issue), the jitter will be optimized to function in a high-memory environment. In this way, developers can write a single body of code that can be efficiently JIT compiled and executed on machines with different architectures. 11 Distinguishing Between Assembly, Namespace, and Type The C# language does not come with a language-specific code library. Rather, C# developers leverage the language-neutral.NET libraries. To keep all the types within the base class libraries well organized, the.NET platform makes extensive use of the namespace concept. A namespace is a grouping of semantically related types contained in an assembly or possibly spread across multiple related assemblies. For example, the System.IO namespace contains file I/O-related types, the System.Data namespace defines basic database types, and so on. It is important to point out that a single assembly can contain any number of namespaces, each of which can contain any number of types. Any language targeting the.NET runtime uses the same namespaces and same types. The most fundamental namespace to get your head around initially is named System. This namespace provides a core body of types that you will need to leverage time and again as a.NET developer. In fact, you cannot build any sort of functional C# application without at least making a reference to the System namespace, as the core data types (e.g., System.Int32, System.String) are defined here. 12 Accessing a Namespace Programmatically It is worth reiterating that a namespace is nothing more than a convenient way for us to logically understand and organize related types. Consider again the System namespace. From your perspective, you can assume that System.Console represents a class named Console that is contained within a namespace called System. However, in the eyes of the.NET runtime, this is not so. The runtime engine sees only a single class named System.Console. In C#, the using keyword simplifies the process of referencing types defined in a particular namespace. Assume in a Calc example program, there is a single using statement at the top of the file. using System; That statement is a shortcut to enable this line of code: Console.WriteLine("10 + 84 is {0}.", ans); Without the using statement, the code would need to be written like this: 13 System.Console.WriteLine("10 + 84 is {0}.", ans); We avoid the use of fully qualified names (unless there is a definite ambiguity to be resolved) and opt for the simplified approach of the C# using keyword. However, always remember that the using keyword is simply a shorthand notation for specifying a type’s fully qualified name, and either approach results in the same underlying CIL (given that CIL code always uses fully qualified names) and has no effect on performance or the size of the assembly. Global Using Statements (New 10.0) As you build more complex C# applications, you will most likely have namespaces repeated in multiple files. Introduced in C# 10, namespaces can be referenced globally, and then be available in every file in the project automatically. Simply add the global keyword in front of your using statements, like this: global using System; All global using statements must come before any non-global using statements. A recommendation is that you place the global using statements in a completely separate file (such as GlobalUsings.cs) for better visibility. In addition to placing the global using statements in Program.cs (or a separate file), they can be placed in the project file for the application using the following format: 14 Implicit Global Using Statements (New 10.0) 15 Another new feature included with.NET 6/C# 10 are implicit global using statements. The implicit global using statements supplied by.NET 6 varies based on the type of application you are building. The vast majority of the C# 10 project templates enable global implicit using statements by default with the ImplicitUsings element in the project’s main Property group. To disable the setting, update the project file to the following: net6.0 enable disable File Scoped Namespaces (New 10.0) Also new in C# 10, file-scoped namespaces remove the need to wrap your code in braces when placing it in a custom namespace. Take the following example of the Calculator class, contained in the CalculatorExamples namespace. Prior to C# 10, to place a class in a namespace required the namespace declaration, an opening curly brace, the code (Calculator), and then a closing curly brace. In the example, the extra code is in bold: namespace CalculatorExamples { class Calculator() {... } } As your code becomes more complex, this can add a lot of extra code and indentation. With file scoped namespaces, the following code achieves the same effect: namespace CalculatorExamples class Calculator() { 16... } Referencing External Assemblies Adding assemblies into most.NET projects is done by adding NuGet packages. However,.NET applications targeting (and being developed on) Windows still have access to COM libraries. For an assembly to have access to another assembly that you are building (or someone built for you), you need to add a reference from your assembly to the other assembly and have physical access to the other assembly. Depending on the development tool you are using to build your.NET applications, you will have various ways to inform the compiler which assemblies you want to include during the compilation cycle. Application Development o Web Applications: ASP.NET provides a framework for building dynamic web applications with server-side logic. o Windows Applications: Windows Forms and WPF allow for the creation of desktop applications with rich user interfaces. o Console Applications: Simple command-line based applications that interact with the user through text input and output. o Service Applications: Includes Windows services and web services for running background tasks and implementing service-oriented architecture. Development Tools o Visual Studio: The primary Integrated Development Environment (IDE) for.NET development. It includes features such as code editing, debugging, and project management. o NuGet: A package manager for.NET that simplifies the process of incorporating third-party libraries into.NET applications. 17 Evolution of.NET Overview of.NET Framework o Initial Release: Early 2000s. o Platform: Primarily for Windows-based applications. o Components: Included ASP.NET, Windows Forms, WPF, and more. Overview of.NET Core o Initial Release: 2016. o Platform: Designed to be cross-platform, supporting Windows, macOS, and Linux. o Open Source: Encouraged greater community involvement and allowed faster updates and iterations..NET 5 and Beyond o Unification: Combined.NET Framework and.NET Core into a single platform to simplify development. o Improvements: Focused on performance enhancements, new APIs, and better support for cloud and microservices architectures. Choosing a.NET Version o For new projects, opt for.NET ≥ 6 (formerly.NET Core) for its modern features and cross-platform support. o.NET Framework is legacy and should be used only for specific legacy projects or Windows-specific requirements. o Consider platform compatibility and project needs when selecting a.NET version. The.NET Standard was a common API specification for.NET libraries, allowing us to use it across all.NET projects. It means that different types of projects would be able to share libraries between them with.NET Standard, including implementations between.NET Framework and.NET Core projects. 18 The.NET Core and.NET Standard are different things. The first one is incompatible with original.NET Framework libraries, and the second was made to be a bridge between all.NET versions. Therefore, considering there is no compatibility between.NET Core and.NET Framework, the best strategy to migrate.NET Framework applications to.NET Core is to create libraries in.NET Standard. In that way, it is possible to keep your old implementation without breaking changes and use the same libraries in.NET Core projects in case migration gradually occurs. The.NET Standard 2.0 supports interoperability with.NET Framework 4.6.1 or later versions. Therefore, projects that use an older version of the.NET Framework, such as 2.0 or 3.5, cannot use.NET Standard libraries. It is recommended to migrate the project to the 4.6.1 version before starting any migration to.NET Core. Tools and Environment Setup Visual Studio Installation: 1. Download from the [official Visual Studio website](https://visualstudio.microsoft.com/). 2. Double-click the executable file. 3. Select desired workloads: - ASP.NET and Web development 19 - Azure development -.NET Desktop development - Universal Windows Platform development -.NET cross-platform development - Mobile development with.NET 4. Choose the language pack if necessary. 5. Complete the installation and start creating.NET projects. - Note: Visual Studio includes the latest.NET SDK, and updates are managed through the IDE. Visual Studio Code - Visual Studio Code (VSC): A lightweight, cross-platform editor for.NET projects. - Installation: 1. Download from the [official Visual Studio Code website](https://code.visualstudio.com/). 2. Double-click the executable file. 3. Install the necessary extensions for C# and Azure from the Extensions tab. 20 Introduction to Visual Studio Visual Studio (VS) - A comprehensive IDE for developing, debugging, and deploying.NET applications. - Contains project templates for various.NET applications (e.g., Console App, ASP.NET Core Web API, Blazor App). - User Interface: ▪ Solution Explorer: Access to project folders and files. 21 ▪ Editor: Develop code with syntax highlighting, code suggestions, and error messages. ▪ Server Explorer: Connect to external resources like databases and cloud services. ▪ Toolbar: Options to run applications, save changes, debug, comment code, and more. Introduction to Visual Studio Code - A flexible and performance-efficient editor available for Windows, macOS, and Linux. - User Interface: ▪ Explorer Sidebar: Access project folders and files. ▪ Editor: Customizable text editor with syntax highlighting and code suggestions. ▪ Terminal Sidebar: Execute commands and view output. 22 Overview of Principal Project Types in.NET - Project Templates: - Visual Studio provides a variety of project templates for different applications (e.g., Web, Desktop, Mobile). - Examples include Console App, ASP.NET Core Web API, and Blazor App. -.NET Core Projects: - Flexible and compatible with multiple platforms and devices. Application types we will examine in this course are: 1. Windows Forms 2. WPF (Windows Presentation Foundation) 3. WinUI 3 23 Desktop, Windows and Console Applications Native Application Development - Despite the prevalence of web-based applications, desktop applications are still crucial. - Benefits of Desktop Development: Complex Feature Integration: Access to system features like Bluetooth, USB, printers, etc. Better OS and CPU Utilization: Leverage full capabilities of the operating system and hardware. Stability: Operate without the need for an Internet connection. Terminology for “desktop applications” There is an important terminology difference that you need to understand as part of this kind of development between what is called as a Desktop App and a Windows App. Desktop Apps are applications that are built with frameworks previous to UWP, including WPF, WinForms, or any other Win32 application, and its name is meant to reflect the fact that they only run on Desktop devices. Windows Apps are instead applications that were built using UWP, and the name means that it runs on any Windows device. Windows devices refer to a variety of hardware that runs Microsoft Windows operating systems. These can include: o Personal Computers (PCs): Desktops Laptops All-in-one PCs o Tablets: Microsoft Surface devices Other tablets running Windows 1 o 2-in-1 Devices: Convertible laptops with touch screens that can function as both laptops and tablets o Workstations: High-performance computers designed for technical or scientific applications o Servers: Computers designed to manage network resources and serve multiple users simultaneously o Windows Phones (historically, though they are now largely obsolete): Smartphones running the Windows Phone operating system o Embedded Systems: Specialized devices such as ATMs, kiosks, and industrial machines running Windows Embedded or Windows IoT o Gaming Consoles: Xbox Series X|S and Xbox One (running a customized version of Windows) - Evolution of Windows Development: Win32: Introduced in late 1995. Windows Forms: Became popular in 2002 with Visual Studio. Windows Presentation Foundation (WPF): Introduced in 2006. Universal Windows Platform (UWP): Launched in 2016. WinUI 3: WinUI 3 is a native UI platform component that ships with the Windows App SDK. It’s completely decoupled from Windows SDKs. The Windows App SDK provides a unified set of APIs and tools for creating production desktop apps targeting Windows 10 and later, which can be published to the Microsoft Store1. Here’s a brief timeline: o WinUI 2 (2018): A library of Fluent-based UI controls and styles for UWP XAML apps. It leverages the Fluent Design System, Microsoft's design language aimed at creating intuitive and aesthetically pleasing user experiences across devices. 2 o WinUI 3 (2021): Expands WinUI into a full-fledged, standalone UI framework, decoupling it from the operating system and UWP. It’s part of the Windows App SDK (formerly known as Project Reunion), bridging the gap between Win32 and UWP app models. It can be used across various application models, including traditional Win32 desktop apps. Key Features of WinUI 3 o Decoupled from the Operating System: WinUI 3 is not bundled with Windows updates. Developers can integrate and update WinUI independently of the OS, ensuring access to the latest UI features without waiting for Windows releases. o Cross-Platform Application Models: Supports both UWP and Win32 (Desktop) application models, providing flexibility in choosing the appropriate model for the application. o Enhanced Performance and Modernization: Optimized for performance, enabling smoother and more responsive UIs. Incorporates the latest advancements in UI design and technology. o Comprehensive Control Set: Includes all controls from WinUI 2 and introduces new controls, enhancing the range of UI elements available to developers. o Seamless Integration with Windows App SDK: Part of a broader suite of tools and libraries designed to simplify Windows application development. Facilitates access to modern Windows APIs and services. o Backward Compatibility and Migration Support: Provides tools and guidelines to migrate existing UWP and Win32 applications to leverage WinUI 3 features. Ensures that developers can adopt WinUI 3 without extensive rewrites. Windows Forms - History: Widely used since 2002; integrates with Visual Studio for drag-and-drop development. - Support: Compatible with.NET Core,.NET 5, and.NET 6. 3 - Creating a Windows Forms Project: Start a New Project: In Visual Studio, select "Windows Forms" as the project type. Choose.NET Version: Ensure compatibility with.NET 6.0 or higher. Development Tools: Utilize the design mode and Toolbox in Visual Studio for rapid development. UI Design: Drag-and-drop controls onto the form, use property grid to configure properties. Code-Behind: Write event handlers and business logic in C#. Windows Presentation Foundation (WPF) - Introduction: Available since 2006, offers a more modern approach compared to Windows Forms. - Technology: Based on XAML (Extensible Application Markup Language), which separates UI from business logic. - Capabilities: Supports advanced 2D and 3D graphics, data binding, streaming video, flow-style documents, animation and interoperating with legacy GUI models like Windows Forms, ActiveX, etc. - Creating a WPF Project: Start a New Project: In Visual Studio, select "WPF App" as the project type. Choose.NET Version: Ensure compatibility with.NET 6.0 or higher. Development Tools: Visual Studio provides a design mode with XAML code visibility. UI Design: Design the UI using XAML for layout and styling (XAML is not limited to WPF applications. Any application can use XAML to describe a tree of.NET objects, even if they have nothing to do with a visible user interface.). Code-Behind: Implement event handlers and logic in the code-behind files (C). Data Binding: Bind UI elements to data sources using XAML. - Similar to Web development with HTML, XAML keeps a hierarchical structure for all the visual elements for a WPF screen. 4 - There are two options to change the properties: in visual Design mode or the XAML. - Most WPF developers tend to manipulate any aspect of design directly on XAML as the Design mode is quite limited and may also affect productivity. Once a developer becomes familiar with XAML, simple new screens can be quickly developed. - Each XAML file or WPF screen has the equivalent C# file code associated with it. - The C# file handles the logical implementation for the WPF screen, such as button-clicking, retrieving data from the database, calling an external API, validating data, and other operations. - Some critical applications built by Microsoft are made in WPF, such as Visual Studio. Universal Windows Platform (UWP) - Introduction: Launched in 2016, introduced in Windows 10, designed for modern Windows applications. The purpose of this platform is to help develop universal apps that run on Windows 10, Windows 10 Mobile (discontinued), Windows 11, Xbox One, Xbox Series X/S, and HoloLens without the need to be rewritten for each. - UWP apps do not run on earlier Windows versions. - UWP does not target non-Microsoft systems. 5 - Technology: Uses XAML and integrates with the Windows Runtime API. - Benefits: Apps can be published on the Windows Store, access modern Windows features. - Creating a UWP Project: Setup: Ensure UWP workload is installed in Visual Studio. Start a New Project: Select "Blank App (Universal Windows)" as the project type. Choose Target Versions: Select the minimum and target Windows versions to support. Development Mode: Enable Developer Mode in Windows settings for local development. UI Design: Design UI using XAML, taking advantage of adaptive controls for different device sizes. Code-Behind: Write application logic in C#. Packaging: Create app packages for deployment on the Windows Store or distribution. 6 - UWP is part of the Windows operating system itself. It receives constant updates throughout Nuget (the package manager for.NET that lets you produce and consume packages.) package updates and contains many additional built-in controls for development and design. - There are many ways of developing UWP applications. It is possible to use C#, C++, Visual Basic, and JavaScript, including HTML, WinUI, XAML, and DirectX, for development, despite XAML being the most used markup language for this kind of application. Therefore, UWP is more flexible compared to Windows Forms and WPF applications. - As Universal Windows Platform applications have access to a broader range of Windows APIs from the user’s machine, this kind of application follows a stricter flow in terms of security and privacy than other types of Desktop projects within the.NET platform. It is mandatory to specify a Manifest explicitly pointing out which features of the machine must be installed and run. 7 - This flow for installation is expected for any modern application due to security, privacy, and other legal and ethical concerns. Additionally, a detailed manifest helps publish the application in the Windows Store to adequately meet a relevant part of the requirements before allowing users to download and install the application on their machines. 8 WinUI 3 Applications 1. Introduction to WinUI 3 Definition: o WinUI 3 is a state-of-the-art user interface (UI) framework specifically designed for building Windows applications. o It provides a comprehensive set of controls and UI components, enabling developers to create modern, visually appealing, and highly functional applications. Development: o WinUI 3 is developed by Microsoft and the broader developer community, leveraging the advantages of open-source collaboration. o As part of the Windows App SDK, it is intended to modernize the Windows app development experience. 2. Benefits of WinUI 3 Open Source Advantages: o Reliability: Open-source development ensures continuous improvements, quick issue resolution, and regular updates, making the framework more robust and reliable. o Community Involvement: The active participation of the developer community leads to innovative solutions, enhanced features, and faster problem-solving. Backward Compatibility: o Multi-Version Support: Unlike UWP controls, which are tied to specific Windows versions, WinUI 3 controls work across various Windows versions, ensuring broader compatibility and longer application lifespan. o Consistent Updates: WinUI 3 allows for new features and controls to be added without waiting for Windows OS updates, facilitating a more agile development process. 9 Enhanced Controls: o Expanded Toolkit: WinUI 3 introduces new and improved controls that are not available in UWP, providing developers with more tools to create rich user experiences. o Modern Features: The framework includes modern UI features, such as adaptive layouts, high-DPI support, and smooth animations, enhancing the overall user experience. 3. History and Evolution of WinUI First Release (July 2018): o WinUI was initially released as two NuGet packages: Microsoft.UI.Xaml and Microsoft.UI.Xaml.Core.Direct. o These packages allowed developers to use new XAML controls and features in their applications independently of Windows OS updates. WinUI 2.0 (October 2018): Subsequent Versions: o WinUI 2.1 o WinUI 2.2 o WinUI 2.3 o WinUI 2.4 o WinUI 2.5 o WinUI 2.6 o WinUI 2.8 4. Transition to WinUI 3 Major Update: o WinUI 3 is a significant evolution from WinUI 2, transitioning from a library of controls to a full-fledged UI framework built on the Windows desktop.NET platform. 10 11 Library A library is a collection of pre-written code, functions, classes, or modules that developers can use to perform common tasks, solve specific problems, or add particular functionalities to their applications. Libraries are typically designed to be modular and reusable, allowing developers to include only the components they need. Key Characteristics: Focused Functionality: Libraries usually provide specific features or utilities. Developer Control: The developer decides when and how to call library functions. Modularity: Can be integrated into various parts of an application as needed. Framework A framework is a comprehensive, reusable platform for building software applications. It provides a structured foundation, enforcing certain design patterns and architectural principles. Frameworks often include libraries but also define the overall architecture and flow of the application. Key Characteristics: Comprehensive Structure: Offers a complete solution for building applications. Inversion of Control: The framework dictates the flow and calls the developer’s code. Opinionated: Often enforces specific conventions and patterns. Key Differences 12 o This transition marks a shift from UWP to a more flexible and powerful platform for building Windows applications. End of UWP Feature Updates: o With the release of WinUI 3, UWP UI libraries will only receive security updates, and all new features and controls will be added exclusively to WinUI. o This shift ensures that WinUI remains the primary framework for modern Windows app development, with ongoing updates and new features. 5. New Features in WinUI 3 WebView2 Control: 13 o Based on the Chromium-based Microsoft Edge browser, WebView2 provides a modern and performant way to integrate web content into Windows applications. o It offers a consistent and up-to-date web browsing experience, leveraging the latest web standards and technologies. Backward Compatibility: o WinUI 3 includes XAML and Composition features from the Spring 2019 Windows SDK that are compatible with Windows 10 version 1809 and later. o This ensures that applications built with WinUI 3 can run on a wide range of Windows versions, providing broad compatibility and reach. 6. The Windows App SDK and Project Reunion Project Reunion: o An initiative aimed at unifying Windows app development by combining Win32 and UWP APIs into a single, cohesive platform. o This project was later renamed the Windows App SDK, reflecting its broader scope and long-term vision. Scope: o The Windows App SDK includes WinUI 3 and other components that modernize and streamline the Windows development experience. o It aims to provide a unified platform for building Windows applications that run across all Windows devices, from desktops to tablets and beyond. 7. Comparisons to Other Frameworks WinUI 3 vs. UWP: o Control Updates: WinUI allows for new and updated controls without requiring an updated Windows SDK, offering a more flexible and agile development process. o Latest.NET Support: Supports the latest.NET versions, providing improved performance, security, and functionality. o Sandbox Restrictions: WinUI is not confined by UWP's sandbox restrictions, offering more flexibility for developers to build powerful and feature-rich applications. 14 WinUI 3 is not confined by UWP's sandbox restrictions because it is a separate framework from UWP. While UWP is designed to be a secure and restricted environment for apps, WinUI 3 is built on top of the Windows Desktop Runtime (WDR), which provides access to more system features and capabilities than UWP. Here are some key differences between WinUI 3 and UWP: Platform: WinUI 3 is built on top of WDR, which is a desktop platform, while UWP is a universal app platform that can run on both desktop and mobile devices. Capabilities: WinUI 3 has access to more system features and capabilities than UWP, such as file system access, network access, and hardware acceleration. Security: UWP has stricter security restrictions in place to protect users from malicious apps. WinUI 3, on the other hand, has a more flexible security model that allows developers to choose the level of security they need for their apps. In summary, WinUI 3's ability to bypass UWP's sandbox restrictions is due to its reliance on WDR and its more flexible security model. This makes it a suitable choice for developers who need to build desktop apps with access to a wider range of system features and capabilities. WinUI 3 vs. WPF (Windows Presentation Foundation): o Modern Features: WinUI 3 includes modern UI elements such as adaptive layouts and high-DPI support, which are more advanced compared to WPF. o Performance: WinUI 3 can leverage the latest.NET versions and modern hardware, potentially offering better performance for complex applications. o Development Experience: WPF has a mature ecosystem and extensive documentation, but WinUI 3 benefits from ongoing updates and a more modern development approach. WinUI 3 vs. Windows Forms: o UI Capabilities: WinUI 3 offers more advanced and modern UI controls and features compared to the relatively simpler and older Windows Forms. 15 o Performance and Scalability: WinUI 3 is designed for modern hardware and can handle more complex, high-performance applications. o Development Approach: Windows Forms is easier to learn and use for simple applications, but WinUI 3 offers greater flexibility and capabilities for building complex, modern applications. Cross-Platform Potential: o React Native: Integrates with React Native for building cross-platform applications with a consistent UI, extending the reach of WinUI applications beyond Windows. o Uno Platform: Enables WinUI applications to run on iOS, Android, macOS, Linux, and the web, providing true cross-platform capabilities. Console Applications - Purpose: Simplified development for learning C# and performing background tasks. - Use Cases: Ideal for automation scripts, server-side jobs, and simple utilities. - Creating a Console Application: Start a New Project: In Visual Studio, select "Console App" as the project type. Choose.NET Version: Ensure compatibility with.NET 6.0 or higher. Development: Write the main logic in the Program.cs file. Execution: Run the application directly from Visual Studio or command line. Libraries: Use NuGet packages to add functionality. Recommendations for Choosing a.NET Version 16 - Use.NET ≥ 6: For new projects, use.NET versions ≥ 6, which are essentially.NET Core and now considered "regular".NET. - Avoid.NET Framework: Unless you have a specific use case or are maintaining a legacy project, it is recommended to avoid using.NET Framework. Windows Forms Windows Forms provides access to native Windows interface elements by wrapping the existing Windows API in managed code. Managed code in the context of Windows Forms refers to code that is executed within the.NET Common Language Runtime (CLR). The CLR is a managed execution environment that provides services like garbage collection, type safety, and security. In Windows Forms: Native Windows API: This refers to the underlying Windows operating system's functions and interfaces that are directly responsible for creating and manipulating windows, controls, and other UI elements. Managed Code Wrapper: Windows Forms provides a layer of managed code that wraps these native API calls. This means that.NET developers can interact with Windows UI elements using familiar.NET constructs and classes, without needing to write directly in C++ or other native languages. Benefits of Managed Code: Simplified development: Developers can use a higher-level programming language like C# or VB.NET to create Windows Forms applications, reducing the complexity of working with native APIs. Platform independence: While Windows Forms is specifically designed for Windows, the.NET framework provides a degree of platform independence through technologies like.NET Core and.NET 5+. Garbage collection: The CLR handles memory management automatically, reducing the risk of memory leaks and improving developer productivity. Type safety: The.NET framework enforces type safety, helping to prevent common programming errors. 17 In summary, managed code in Windows Forms provides a bridge between the.NET platform and the native Windows API, making it easier for developers to create Windows applications using familiar.NET technologies. Visual Studio uses projects and solutions to manage application development. Conceptually, a project is a collection of files that produce a.NET assembly, such as a library (.dll) or executable (.exe). A solution is a collection of projects that are grouped for development or deployment purposes. When a solution has only one project, the two words are somewhat equivalent. By default, Visual Studio creates a directory for the solution, and then creates a directory for each project within this solution directory. In C#, namespaces and assemblies serve different purposes: 1. Namespaces: o A namespace is a logical grouping of related types (classes, interfaces, enums, etc.). o It helps avoid naming conflicts by organizing types under a common name. o For example, System.Data and System.Web are namespaces. o Namespaces are specified in your source code using the namespace keyword. o They are not directly tied to assemblies; multiple namespaces can exist within a single assembly, and a single namespace can span multiple assemblies. 18 2. Assemblies: o An assembly is a physical unit of code that can be executed by the.NET runtime environment. o It can contain one or more namespaces. o Assemblies define the deployment, versioning, and security boundaries for your code. o They are typically compiled into DLL or EXE files. o Examples of assemblies include System.Data.dll and System.Web.dll. o Assemblies are the building blocks of.NET applications, providing the runtime with information about types and resources. In summary, namespaces organize your code logically, while assemblies define the physical organization and deployment of your code. They work together to create a cohesive application structure. Example Project: The MyPhotos solution is stored on disk in a file called MyPhotos.sln. This solution holds a single project called MyPhotos, stored in the C# project file MyPhotos.csproj. 19.resx files: Store resource info. AssemblyInfo.cs file: Stores assembly info for the project. Form...cs files: Form class files Program.cs: The Program class file, which contains the entry point Main. What are.resx files? 20.resx files are XML-based resource files used in.NET applications to store localized resources such as: Strings: Textual content like labels, button captions, or error messages. Images: Graphics and icons. Sounds: Audio files. Custom objects: Any serializable object. Why use.resx files? Localization: Easily create different language versions of your application by creating separate.resx files for each language. Maintainability: Centralize and manage resources in a single location, making it easier to update and modify them. Reusability: Use resources across multiple parts of your application. Efficiency: Reduce code duplication and improve maintainability. Structure of a.resx file: A.resx file consists of elements, each containing a element that stores the resource data. The attribute of the element specifies the resource name. For example: XML Hello, world! The Program class in C# is defined as static. Reasons for this: 1. Single Entry Point: 21 a. A C# application can have only one entry point. The Main method within the Program class serves as this entry point. b. Defining the Program class as static ensures that the Main method can be called without creating an instance of the class. 2. No Instance Creation: a. The Program class is typically used to initialize the application and set up necessary resources. It doesn't represent a specific object or entity within the application. b. By making it static, there's no need to create an instance, saving memory and avoiding potential complexities. 3. Utility Class: a. The Program class often acts as a utility class, containing static methods for initialization, configuration, or other application-wide tasks. b. Static members are convenient for such tasks as they can be accessed directly without creating an instance. 4. Standard Convention: a. It's a widely adopted convention in C# to define the Program class as static. This makes it easier for developers to understand and maintain C# applications. A namespace defines a group, or scope, of related classes, structures, and other types. A namespace is a bit like a family: it defines a group of distinct members with a common name and some shared sense of purpose. All objects in the.NET Framework, and indeed in C# itself, are organized into namespaces. The System namespace includes obje cts related to the framework itself, and most namespaces defined by.NET are nested within the System namespace. The System.Windows namespace defines types and namespaces related to the Windows operating system. This organization into namespaces permits two objects with the same base name to be distinct—much as two people can both share the same first name. For example, the Button class in the System.Web.UI.WebControls namespace represents a button in a web application, while Button in the System.Windows.Forms namespace represents a button in a Windows application. 22 The line “static void Main()” is the entry point for the application. 23 Partial definition of Form1 class The Main method in the Program class creates a new Form1 object. The Form1 class is partially defined by the Form1.cs file. 24 25 The Windows Forms designer requires the field components in order to ensure that - components are properly managed on the form at runtime,and - specifically for components that are not also Windows Forms controls. The use of garbage collection in.NET means that you have no idea when memory and other system resources will be freed for objects that are no longer in use. The Dispose method provides a way to control how certain resources are released and reclaimed by the system. 26 27 28 29 30 Renaming MainForm.cs as Form1.cs 31 - Adding Controls to a Form You can add controls to a form by dragging and dropping controls from the Toolbox. 32 - You can add controls programmatically too. The Controls property returns an instance of the Control.ControlCollection class. This class defines an Add method that adds a control to the collection. The Controls property can be used to retrieve the controls on a form as well. using System; using System.Drawing; using System.Windows.Forms; namespace MyNamespace{ public class MyForm : Form 33 { private Button btnLoad; private PictureBox pbxPhoto; public MyForm() { // Create and configure a button btnLoad = new Button(); btnLoad.Text = "&Load"; btnLoad.Left = 10; btnLoad.Top = 10; // Create and configure a picture box pbxPhoto = new PictureBox(); pbxPhoto.BorderStyle = BorderStyle.Fixed3D; pbxPhoto.Width = this.Width / 2; pbxPhoto.Height = this.Height / 2; pbxPhoto.Left = (this.Width - pbxPhoto.Width) / 2; pbxPhoto.Top = (this.Height - pbxPhoto.Height) / 2; // Add our new controls to the form 34 this.Controls.Add(btnLoad); this.Controls.Add(pbxPhoto); } public static void Main() { Application.EnableVisualStyles(); Application.Run(new Form1()); } } } - Handling Events in Windows Forms - Event Handling Basics - Events are predefined situations like user interactions (clicks, key presses) or system actions (timers, file changes). Internally, the Windows operating system passes messages around for this purpose. When the user clicks the Load button, a message occurs that indicates the button has been pressed and released. The Application.Run method arranges for the applicati on to wait for such messages in an efficient manner. 35 The.NET Framework defines such actions as events. Events are predefined situations that may occur. Examples include the user clicking the mouse or typing on the keyboard, or an alarm going off for an internal timer. Events can also be triggered by external programs, such as a web server receiving a message, or the creation of a new file on disk. In C#, the concept of an event is built in, allowing classes to define events that may occur, and instances of that class to indicate functions that receive and process these events. For example, we want to do something when the user clicks the Load button. The Button class inherits an event called Click from the base Control class. Our program defines a method called HandleLoadClick to handle this event. We link these two together by registering our method as an event handler for the Click event. btnLoad.Click += new EventHandler(this.HandleLoadClick); Since it is possible to have more than one handler for an event, the += notation is used to add a new event handler without removing any existing handlers. When multiple event handlers are registered, the handlers are typically called sequentially in the same order in which they were added. The System.EventHandler is a delegate in C#, and specifies the signature required to process the event. In this case, EventHandler is defined internally by the.NET Framework as follows: 36 public delegate void EventHandler(object sender, EventArgs e); A delegate is similar to a function pointer in C or C++, except that delegates are type-safe. The term type-safe means that code is specified in a well-defined manner that can be recognized by a compiler. That is, an incorrect use of a delegate is a compile-time error. As of.NET 2.0, C# allows the delegate to be omitted, since the compiler already knows that the Button.Click method accepts the System.EventHandler delegate. This permits the shortened form: btnLoad.Click += this.HandleLoadClick; 37 - Example of handling a button click event 38 39 - Resizing Forms - Anchor Property - Controls can be anchored to one or more sides of the form to adjust their position and size dynamically when the form is resized. o Anchored controls maintain a fixed distance from their anchor positions when the form is resized. o To anchor a control: Use the Visual Studio Designer Properties window. Select the control, go to the Properties window, and set the Anchor property to one or more values (e.g., AnchorStyles.Bottom | AnchorStyles.Right). 40 41 You can also set the Anchor property programmatically: button1.Anchor = AnchorStyles.Bottom | AnchorStyles.Right; The control will adjust its position and size as the form resizes, maintaining the specified anchor positions. btnLoad.Anchor = AnchorStyles.Top | AnchorStyles.Left; pbxPhoto.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; - Example Code for Resizing (the revised code ensures that the picture box resizes with the form): 42 43 - Dock Property - The use of Anchor is fine when you have a set of controls and need to define their resize behavior. - In the case where you want to use as much of the form as possible, the Anchor property does not quite work. You could position the control at the edges of the form and anchor it to all sides, this is not the most elegant solution. - Instead, the Dock property is provided for this purpose. - The Dock property also affects the resizing of controls on a form. - The Dock property establishes a location for a control within its container by fixing it flush against a side of the form. - Like Anchor, the Dock property takes its values from an enumeration, in this case the DockStyle enumeration. Note that the AnchorStyles enumeration is plural since a control can have multiple anchor settings, while the DockStyle enumeration is singular since a control can have a single docked value. - We can see how the Dock property works by replacing the pbxPhoto.Anchor line in our program with a Dock setting that causes the PictureBox control to fill the entire form. 44 45 Using Controls and Properties - Common Properties - `Text`, `Left`, `Top`, `Width`, `Height`, `Anchor`, `BorderStyle`, `SizeMode` are common properties for configuring controls. 46 WPF Applications WPF Overview Windows Presentation Foundation (WPF) is a development framework used to create desktop applications. It is a part of the.NET framework. WPF provides a resolution- independent and vector-based rendering engine, which is helpful for dealing with modern graphics hardware. Additionally, WPF for.NET is an open-source framework forked from the original WPF for.NET Framework source code, with some differences between the two versions: 1. SDK-style projects:.NET uses SDK-style project files, different from the traditional.NET Framework project files managed by Visual Studio. To migrate your.NET Framework WPF apps to.NET, you’ll need to convert your projects. 2. NuGet package references: If your.NET Framework app lists NuGet dependencies in a packages.config file, migrate to the format. This change simplifies package management and eliminates the local “Packages” folder. 3. Code Access Security (CAS): CAS is not supported by.NET. WPF for.NET removes CAS-related code, assuming full trust. Publicly defined CAS-related types were moved out of the WPF assemblies and into the Core.NET library assemblies. Features: - Utilizes DirectX for rendering. - Declarative programming model using XAML (eXtensible Application Markup Language). - Clear separation of UI (XAML) and logic (C# code-behind). At runtime when a XAML element is processed, the default constructor for its underlying class is called, and the object is instantiated; its properties and events are set based on the attribute values specified in XAML. - XAML is XML-Based. It requires well-formed XML, meaning all tags must be properly closed and validated against specific schemas. - XAML is ideal for defining UI layout and static properties. C# is necessary for dynamic behaviors and business logic. - WPF's data binding capabilities allow for dynamic and complex data visualization. - It leverages the Common Language Runtime (CLR), enabling the use of languages like C# to communicate with UI elements. 1 - Traditional controls like combo boxes are limited to displaying text, but WPF enhances usability by allowing the inclusion of images, labels, text boxes, tooltips, and more within a single control. For instance, a combo box in WPF can include images, text, and even other controls, enhancing the user experience. - WPF integrates media, 2D and 3D graphics, documents, and typography into a single system. Key Concepts in WPF 1. Device Independent Units (DIUs): - WPF measures UI elements in DIUs, which are based on the system's DPI settings. This ensures a consistent look across different hardware configurations. 2. Layout System: - WPF's layout system is based on relative values and is adjusted at runtime. This dynamic layout approach allows for flexible and adaptive UIs. 3. UIElements: - In WPF, everything that appears on the screen is a `UIElement`. This includes controls, panels, and other visual elements. 2 Development Tools: - Visual Studio and Blend for Visual Studio provide comprehensive tools for designing and developing WPF applications. It is possible to switch back and forth between Visual Studio and Blend for Visual Studio, and the same project can be opened in both the IDEs at the same time. Architecture: 3 ** Differences between managed and unmanaged code: 1. Managed Code: Execution Environment: Managed code runs within a managed runtime environment, such as the Common Language Runtime (CLR) in.NET Framework. Security: It provides security features for applications written in.NET Framework. Memory Management: Memory is managed by the CLR’s Garbage Collector. Services: It offers runtime services like garbage collection, exception handling, etc. Compilation: The source code is compiled into an intermediate language (IL or MSIL or CIL). Access Level: It does not provide low-level access to the programmer. Performance: Slightly slower due to memory management overhead and Just-In-Time (JIT) compilation. Debugging: Easier due to availability of CLR’s debugging tools. Distribution: Requires installation of CLR on the target machine. Interoperability: Interoperates well with other.NET languages and libraries. 2. Unmanaged Code: 4 Execution Environment: Unmanaged code is directly executed by the operating system. Security: It does not provide any security features. Memory Management: Memory is managed by the programmer. Services: No runtime services like garbage collection or exception handling. Compilation: The source code directly compiles into native languages. Access Level: Provides low-level access to the programmer. Performance: Faster due to direct access to system resources and compiled machine-specific code. Debugging: Harder due to lack of debugging tools. Distribution: Can be distributed as a standalone executable or DLL file. Interoperability: Limited interoperability with.NET languages. Getting Started with WPF Applications Pre-requisites: - Visual Studio 2022 Community Edition (version 17.4 or later). -.NET desktop development workload installed. Creating a New WPF Project Step-by-Step Guide: 1. Create a New Project: - Navigate to File -> New -> Project... - Select "WPF Application" template. - Configure the project name, location, and solution settings. Video: https://www.youtube.com/watch?v=4DZZadT2RPs 2. Project Structure: - App.xaml and App.xaml.cs: Entry point of the application. - MainWindow.xaml and MainWindow.xaml.cs: Main application window. - Dependencies: Includes Microsoft.NETCore.App and Microsoft.WindowsDesktop.App.WPF. 5 3. Important Files in a WPF Project: XAML Basics - Namespaces and Schemas: At the root of every XAML file, you will declare the namespaces to be used: 6 This listing displays the XAML that was generated by the WPF App (.NET Framework) template in Visual Studio. - Namespaces are typically defined as an attribute within the root element of the document; the root element is the first XML tag in the XAML document. XAML uses XML syntax to define a namespace — “xmlns” means “XML namespace,” and it’s typically followed by a colon (:) and then an alias. This alias is the shorthand reference to the namespace throughout the XAML document; it’s what you use to instantiate an object from a class in that namespace. The namespace that represents the CLR objects will be distinguished in the XAML file with the x prefix. The first XML namespace, http://schemas.microsoft.com/winfx/2006/xaml/presentation, maps a slew of WPF.NET namespaces for use by the current *.xaml file (System.Windows, System.Windows.Controls, System.Windows.Data, System.Windows.Ink, System.Windows.Media, System.Windows.Navigation, etc.). This one-to-many mapping is hard-coded within the WPF assemblies (WindowsBase.dll, PresentationCore.dll, and PresentationFramework.dll) using the assembly-level [XmlnsDefinition] attribute. For example, if you open the Visual Studio Object Browser and select the PresentationCore.dll assembly, you will see listings such as the following, which essentially imports System.Windows: [assembly:XmlnsDefinition("http://schemas.microsoft.com/winfx/2006/xaml/prese ntation", "System.Windows")] The second XML namespace, http://schemas.microsoft.com/winfx/2006/xaml, is used to include XAML-specific “keywords” (for lack of a better term) as well as the inclusion of the System.Windows.Markup namespace, as follows: [assembly:XmlnsDefinition("http://schemas.microsoft.com/winfx/2006/xaml", 7 "System.Windows.Markup")] - In addition to these two necessary XML namespace declarations, it is possible, and sometimes necessary, to define additional tag prefixes in the opening 8 element of a XAML document. You will typically do so whenever you need to describe in XAML a.NET class defined in an external assembly. For example, say you have built a few custom WPF controls and packaged them in a library named MyControls.dll. Now, if you want to create a new Window that uses these controls, you can establish a custom XML namespace that maps to your library using the clr-namespace and assembly tokens. Here is some example markup that creates a tag prefix named myCtrls, which can be used to access controls in your library: The clr-namespace token is assigned to the name of the.NET namespace in the assembly, while the assembly token is set to the friendly name of the external *.dll assembly. You can use this syntax for any external.NET library you would like to manipulate in markup. - If you need to define a class in markup that is part of the current assembly but in a different.NET namespace, your xmlns tag prefix is defined without the assembly= attribute, like so: xmlns:myCtrls="clr-namespace:SomeNamespaceInMyApp". - The StartupUri value defines the window that will be displayed before the application is executed. In this case, the MainWindow.xaml window will be displayed. 9 - The x:Class attribute defines the C# code-behind file of this XAML file. If you open App.xaml.cs, you see that its class name is App and it inherits from the Application class (which is the root element of the XAML file). - XAML elements correspond to.NET classes, and attributes correspond to properties or events of those classes. The First Example for XAML and Code-Behind 10 11 12 13 14 Creating and Using Resources in a WPF App (App.xaml for the second example) - The resources of a WPF app are contained in a keyed collection of reusable objects. - Resources can be created and retrieved using both XAML and C#. - Resources can be anything — data templates, arrays of strings, or brushes used to color the background of text boxes. - Resources doesn’t define a class as most XAML elements do. A resource is actually assigning a value to the Resources property of its containing Application object. This type of tag is called a property element, an XML element that represents a property (or attribute) of an object. Property elements are used when complex objects are assigned to a property of an object that can’t be expressed as a simple string value. Property elements must be contained within the tags of the parent element — in this case, within the Application tags. 15 - Resources are also scoped, meaning that they can be available to the entire Application (global), to the Window, to the User Control, or even to only a specific control. - You can access resources in XAML using the `StaticResource` or `DynamicResource` markup extensions. For example: A StaticResource will be resolved and assigned to the property during the loading of the XAML which occurs before the application is actually run. It will only be assigned once and any changes to resource dictionary ignored. It will be resolved on object construction. A DynamicResource assigns an Expression object to the property during loading but does not actually lookup the resource until runtime when the Expression object is asked for the value. This defers looking up the resource until it is needed at runtime. A good example would be a forward reference to a resource defined later on in the XAML. Another example is a resource that will not even exist until runtime. It will update the target if the source resource dictionary is changed. It will be evaluated and resolved every time control needs the resource. - Example for defining a string resource at the application level (An application-scoped resource is available to all Windows and user controls defined in the project.): 1.Add the System namespace located in the mscorlib.dll assembly by adding the following namespace to the App.xaml root element. xmlns:sys="clr-namespace:System;assembly=mscorlib" The mscorlib.dll assembly is where the String class is located. The String class is now available for use throughout the App.xaml document. 2. Create the resource between the Application.Resource tags; add the following String class element: Hello WPF World! This element instantiates an object of type String, initialized to the value Hello WPF World!, and keyed off of the key Purpose. This resource is now available throughout the MyFirstWPFApplication application by requesting the resource “Purpose”. 16 17 The Second Example for XAML and Code-Behind 18 App.xaml is the entry point of the WPF application. Within App.xaml, the StartupUri value defines the window displayed on application startup. In this case, the StartupUri value is MainWindow.xaml. 19 20 Creating the Second Example with C# Code Only Anything that you can implement using XAML can be implemented in C#. This is not true in reverse; not everything you can do in C# can be done in XAML. C# is the obvious choice for performing business logic tasks with procedural code that can’t be expressed. Create a new project. 21 Controlling Class and Member Variable Visibility Consider the following XAML definition that makes use of the ClassModifier and FieldModifier keywords: 22 23 Fields can be marked as public, private, protected, internal, protected internal, or private protected. 24 A breakdown of the different types you can encounter in C#: 1. Classes: The most common type in C#. Encapsulate data (properties) and behavior (methods). Can be used to represent complex objects or entities. 2. Structs: Similar to classes but have value semantics rather than reference semantics. Typically used for small, immutable data structures. Often used to represent simple data types like points, colors, or rectangles. 3. Interfaces: Define a contract that classes must adhere to. Specify the methods and properties that a class must implement. Used to promote code reuse and polymorphism. 4. Enums: Define a set of named constants. Often used to represent a fixed set of values. Example: enum DayOfWeek { Sunday, Monday, Tuesday,... } 5. Delegates: Type-safe function pointers. Used to pass methods as arguments to other methods. Commonly used for event handling and callbacks. 6. Primitive Types: Built-in types that represent basic data values. Examples: int, double, bool, char, string. 7. Nullable Types: Can hold a value or be null. Used to indicate that a value may be missing. Example: int? (nullable integer) 25 XAML types are the building blocks used to create elements and their properties within a XAML document. They provide a declarative way to define the user interface of a.NET application. Key Points: Correspondence to C# Types: XAML types directly correspond to C# types. When you create an element in XAML, you are essentially instantiating a C# class object. Element Tags: Each XAML element is associated with a C# class. The element tag represents the class name. Properties: The properties of a XAML element are defined by the corresponding C# class. These properties can be set using attributes within the XAML element. Content: The content of a XAML element can be defined using either plain text or nested elements. The type of content that an element can accept is determined by the C# class associated with the element. Common XAML Types: 1. FrameworkElement: A base class for many UI elements, providing common properties like Width, Height, Margin, Padding, and more. 2. Control: A derived class from FrameworkElement that represents interactive UI elements. Examples include Button, TextBox, ComboBox, ListBox, etc. 3. Panel: A derived class from FrameworkElement that is used to arrange other elements. Examples include StackPanel, Grid, Canvas, etc. 4. Shape: A derived class from FrameworkElement that represents geometric shapes like Rectangle, Ellipse, Line, etc. 5. DataTemplate: A template that defines the visual representation of data items. Used in conjunction with data binding to display data in a specific format. 6. Style: A collection of properties that can be applied to multiple elements. Used to create consistent styles and themes. Example: Code snippet In this example: Grid is a panel that arranges its children using rows and columns. 26 TextBlock and Button are controls that represent text and a clickable button, respectively. C# members are the components that make up a class or struct. They define the structure and behavior of an object. There are three main types of members: fields, properties, and methods. Fields Direct storage: Fields are directly stored within an object and can be accessed and modified directly. Data encapsulation: They are typically private to ensure data integrity and prevent unauthorized access. Initialization: Fields can be initialized either within the class or struct definition or in the constructor. Example: C# public class Person { private string name; private int age; public Person(string name, int age) { this.name = name; this.age = age; } } Properties Encapsulated access: Properties provide controlled access to the underlying data, often using getter and setter methods. Data validation: They can implement logic to validate data before setting it. Calculated properties: They can return calculated values based on other properties or fields. Example: ``csharp public class Person { private string name; private int age; public string Name { 27 get { return name; } set { name = value; } } public int Age { get { return age; } set { age = value; } } } Methods Behavior definition: Methods define the actions or operations that an object can perform. Parameter passing: They can accept parameters as input and return values as output. Method overloading: Multiple methods with the same name `csharp public class Person { //... public void Greet() { Console.WriteLine("Hello!"); } public int CalculateAge(int currentYear) { return currentYear - age; } } Additional Notes: Constructors: Special methods used to initialize objects. Destructors: Methods called automatically when an object is garbage collected (although rarely used in modern C#). Static members: Members that belong to the class itself rather than individual instances. Nested types: Classes or structs defined within another class or struct. 28 By default, all C#/XAML type definitions are public, while members default to internal. However, based on your XAML definition, the resulting autogenerated file contains an internal class type with a public Button variable. internal partial class MainWindow : System.Windows.Window, System.Windows.Markup.IComponentConnector { public System.Windows.Controls.Button myButton;... } XAML Property-Element Syntax Property-element syntax allows you to assign complex objects to a property. Here is a XAML description for a Button that makes use of a LinearGradientBrush to set its Background property: Notice that within the scope of the and tags, you have defined a subscope named. Within this scope, you have defined a custom. Any property can be set using property-element syntax, which always breaks down to the following pattern: 29 While any property could be set using this syntax, if you can capture a value as a simple string, you will save yourself typing time. For example, here is a much more verbose way to set the Width of your Button:... 100 6Processing StartUp and Exit Events In addition to the namespace definitions, the App.xaml file defines application properties such as the StartupUri, application-wide resources, and specific handlers for application events such as Startup and Exit. The StartupUri indicates which window to load on startup. Examine the markup, shown here: 30 Using the XAML designer and using Visual Studio code completion, you can add handlers for the Startup and Exit events. Your updated XAML should look like this (notice the change in bold): If you look at the App.xaml.cs file, it should look like this: 31 public partial class App : Application { private void App_OnStartup(object sender, StartupEventArgs e) { } private void App_OnExit(object sender, ExitEventArgs e) { } } The InitializeComponent() method configures the application properties, including the StartupUri and the event handlers for the Startup and Exit events. public void InitializeComponent() { #line 5 "..\..\App.xaml" this.Startup+=new System.Windows.StartupEventHandler(this.App_OnStartup); #line default #line hidden this.Exit += new System.Windows.ExitEventHandler(this.App_OnExit); #line default } XAML Attached Properties 32 In addition to property-element syntax, XAML defines a special syntax used to set a value to an attached property. Essentially, an attached property allows a child element to set the value for a property that is defined in a parent element. The general template to follow looks like this: The most common use of attached property syntax is to position UI elements within one of the WPF layout manager classes (Grid, DockPanel, etc.). There are a few items to be aware of regarding attached properties. First and foremost, this is not an all-purpose syntax that can be applied to any property of any parent. For example, the following XAML cannot be parsed without error: Attached properties are a specialized form of a WPF-specific concept termed a dependency property. Unless a property was implemented in a specific manner, you cannot set its value using attached property syntax. 33 XAML Markup Extensions There is another way to specify the value of a XAML attribute, using markup extensions. Markup extensions allow a XAML parser to obtain the value for a property from a dedicated, external class. This can be beneficial given that some property values require several code statements to execute to figure out the value. Markup extensions provide a way to cleanly extend the grammar of XAML with new functionality. A markup extension is represented internally as a class that derives from MarkupExtension. Note that the chances of you ever needing to build a custom markup extension will be slim to none. However, a subset of XAML keywords (such as x:Array, x:Null, x:Static, and x:Type) are markup extensions in disguise! A markup extension is sandwiched between curly brackets, like so: 34 In the given XAML code, several **markup extensions** are used. Markup extensions in XAML allow for more dynamic and flexible behavior by instructing the XAML parser to perform operations beyond static value assignment, similar to how you can use expressions in other programming languages. Let’s break down each markup extension used in this code: 1. `{x:Static}` Markup Extension The `{x:Static}` markup extension is used to refer to a static field or property in code. In XAML, it provides a way to assign values that are constants or static members from a type (usually from.NET types or user-defined classes) to a property. Code Example: ```xml ``` Explanation: - `x:Static CorLib:Environment.OSVersion`**: The `OSVersion` is a static property of the `Environment` class from the `System` namespace (aliased as `CorLib`). This property retrieves the current operating system version, and this value is displayed in the `Label`. - `x:Static CorLib:Environment.ProcessorCount`**: Similarly, `ProcessorCount` is a static property of the `Environment` class that retrieves the number of processors available on the system. This value will also be displayed in the `Label`. In both cases, the `{x:Static}` markup extension is used to fetch these static values and display them as the content of the `Label` control. 2. `{x:Type}` Markup Extension The `{x:Type}` markup extension in XAML is equivalent to the `typeof` operator in C#. It allows you to pass the `Type` object of a class to a property. This is often used to reference types for type-related properties or operations, such as for data templates, style selectors, etc. **Code Example:** ```xml ``` 35 Explanation: - `x:Type Button`: This returns the `Type` object that represents the `Button` class. The content of the `Label` will display the name of the type, which in this case is `Button`. 3. `{x:Type}` with `CorLib:Boolean` ```xml ``` Explanation: -`x:Type CorLib:Boolean`: This retrieves the `Type` object for the `Boolean` type, which resides in the `CorLib` namespace (usually aliased for `System` in WPF XAML). It would display the `Type` name, which in this case is `Boolean`. Summary of Markup Extensions in this Code: - `{x:Static}`: Used to fetch the value of a static field or property. In the code, it is used to display system-related information like `OSVersion` and `ProcessorCount`. - `{x:Type}`: Used to reference the type object of a class. In the code, it is used to display the name of types like `Button` and `Boolean`. These extensions help create more dynamic UIs by allowing properties to reference system properties, type information, or other static data.