CS 102: Programming1 Lecture 3 Fall 2024 PDF
Document Details
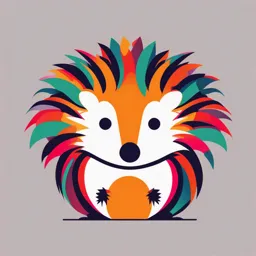
Uploaded by WorldFamousZither
Horus University
2024
Tags
Summary
This document is a lecture on Java operators, including arithmetic, assignment, relational, logical, and bitwise operators. Examples of code are presented.
Full Transcript
CS 102: Programming1 Lecture 3 Fall 2024 outlines Operators in Java Arithmetic Operators Assignment Operators Relational Operators Logical Operators Unary Operators Bitwise Operators Operators constitute the basic building block to any programming l...
CS 102: Programming1 Lecture 3 Fall 2024 outlines Operators in Java Arithmetic Operators Assignment Operators Relational Operators Logical Operators Unary Operators Bitwise Operators Operators constitute the basic building block to any programming language. Java too provides many types of operators which can be used according to the need to perform various calculation and functions be it logical, arithmetic, relational etc. They are classified based on the functionality they provide. Here are a few types: 1. Arithmetic Operators 2- Unary Operators 3- Assignment Operator 4- Relational Operators 5- Logical Operators 6- Ternary Operator 7- Bitwise Operators 8- Shift Operators Arithmetic Operators Addition(+) Operator Addition(+): This operator is a binary operator and is used to add two operands. class Addition { public static void main(String[] args) { int num1 = 10, num2 = 20, sum = 0; System.out.println("num1 = " + num1); System.out.println("num2 = " + num2); sum = num1 + num2; System.out.println("The sum = " + sum); } Output: } num1 = 10 num2 = 20 The sum = 30 Subtraction(-) Operator Subtraction(-): This operator is a binary operator and is used to subtract two operands. class Subtraction { public static void main(String[] args) { int num1 = 20, num2 = 10, sub = 0; System.out.println("num1 = " + num1); System.out.println("num2 = " + num2); sub = num1 - num2; System.out.println("Subtraction = " + sub); } Output: num1 = 20 } num2 = 10 Subtraction = 10 Multiplication(*): Multiplication(*): This operator is a binary operator and is used to multiply two operands. class Multiplication { public static void main(String[] args) { int num1 = 20, num2 = 10, mult = 0; System.out.println("num1 = " + num1); System.out.println("num2 = " + num2); mult = num1 * num2; System.out.println("Multiplication = " + mult); } } Output: num1 = 20 num2 = 10 Multiplication = 200 Division(/): Division(/): This is a binary operator that is used to divide the first operand(dividend) by the second operand(divisor) and give the quotient as result. public class Division { public static void main(String[] args) { int num1 = 20, num2 = 10, div = 0; System.out.println("num1 = " + num1); System.out.println("num2 = " + num2); div = num1 / num2; System.out.println("Division = " + div); } } Output: num1 = 20 num2 = 10 Division = 2 Modulus(%): Modulus(%): This is a binary operator that is used to return the remainder when the first operand(dividend) is divided by the second operand(divisor). public class Modulus { public static void main(String[] args) { int num1 = 5, num2 = 2, mod = 0; System.out.println("num1 = " + num1); System.out.println("num2 = " + num2); mod = num1 % num2; System.out.println("Remainder = " + mod); } } Output: num1 = 5 num2 = 2 Remainder = 1 Java Assignment Operator Java Assignment Operator These operators are used to assign values to a variable. The left side operand of the assignment operator is a variable, and the right-side operand of the assignment operator is a value. “=”: This is the simplest assignment operator which is used to assign the value on the right to the variable on the left. This is the basic definition of assignment operator and how does it function. public class Assignment { public static void main(String[] args) { int num; String name; num = 10; name = "CJCS"; System.out.println("num is assigned: " + num); Output: System.out.println("name is assigned: " + name); num is assigned: 10 }} name is assigned: CJCS “+=” operator “+=”: This operator is a compound of ‘+’ and ‘=’ operators. It operates by adding the current value of the variable on left to the value on the right and then assigning the result to the operand on the left. public class Assignment { public static void main(String[] args) { int num1 = 10, num2 = 20; System.out.println("num1 = " + num1); System.out.println("num2 = " + num2); num1 += num2; System.out.println("num1 = " + num1); } } Output: num1 = 10 num2 = 20 num1 = 30 “-=” operator “-=”: This operator is a compound of ‘-‘ and ‘=’ operators. It operates by subtracting the value of the variable on right from the value of the variable on the left and then assigning the result to the operand on the left. current public class Assignment { public static void main(String[] args) { int num1 = 10, num2 = 20; System.out.println("num1 = " + num1); System.out.println("num2 = " + num2); num1 -= num2; System.out.println("num1 = " + num1); } } Output: num1 = 10 num2 = 20 num1 = -10 “*=” operator “*=”: This operator is a compound of ‘*’ and ‘=’ operators. It operates by multiplying the current value of the variable on left to the value on the right and then assigning the result to the operand on the left. public class Assignment { public static void main(String[] args) { int num1 = 10, num2 = 20; System.out.println("num1 = " + num1); System.out.println("num2 = " + num2); num1 *= num2; System.out.println("num1 = " + num1); } } Output: num1 = 10 num2 = 20 num1 = 200 Relational Operators with Examples Relational Operators with Examples ‘Equal to’ operator (==): This operator is used to check whether the two given operands are equal or not. The operator returns true if the operand at the left-hand side is equal to the right-hand side, else false. public class Relational { public static void main(String[] args) { int var1 = 5, var2 = 10, var3 = 5; System.out.println("Var1 = " + var1); System.out.println("Var2 = " + var2); System.out.println("Var3 = " + var3); System.out.println("var1 == var2: "+ (var1 == var2)); System.out.println("var1 == var3: "+ (var1 == var3)); } } Output: Var1 = 5 Var2 = 10 Var3 = 5 var1 == var2: false var1 == var3: true Relational Operators with Examples ‘Equal to’ operator (==): This operator is used to check whether the two given operands are equal or not. The operator returns true if the operand at the left-hand side is equal to the right-hand side, else false. public class Relational { public static void main(String[] args) { int var1 = 5, var2 = 10, var3 = 5; System.out.println("Var1 = " + var1); System.out.println("Var2 = " + var2); System.out.println("Var3 = " + var3); System.out.println("var1 == var2: "+ (var1 == var2)); System.out.println("var1 == var3: "+ (var1 == var3)); } } Output: Var1 = 5 Var2 = 10 Var3 = 5 var1 == var2: false var1 == var3: true Greater than’ operator(>): ‘Greater than’ operator(>): This checks whether the first operand is greater than the second operand or not. The operator returns true when the operand at the left-hand side is greater than the right-hand side. class Relational { public static void main(String[] args) { int var1 = 30, var2 = 20, var3 = 5; System.out.println("Var1 = " + var1); System.out.println("Var2 = " + var2); System.out.println("Var3 = " + var3); System.out.println("var1 > var2: "+ (var1 > var2)); System.out.println("var3 > var1: "+ (var3 >= var1)); } } Output: Var1 = 30 Var2 = 20 Var3 = 5 var1 > var2: true var3 > var1: false ‘Less than’ Operator(=): This checks whether the first operand is greater than or equal to the second operand or not. The operator returns true when the operand at the left-hand side is greater than or equal to the right-hand side. public class Relational { public static void main(String[] args) { int var1 = 20, var2 = 20, var3 = 10; System.out.println("Var1 = " + var1); System.out.println("Var2 = " + var2); System.out.println("Var3 = " + var3); System.out.println("var1 >= var2: "+ (var1 >= var2)); System.out.println("var2 >= var3: "+ (var3 >= var1)); } } Output: Var1 = 20 Var2 = 20 Var3 = 10 var1 >= var2: true var2 >= var3: false 'Less than or equal to' Operator(