Parallel Algorithms Techniques PDF
Document Details
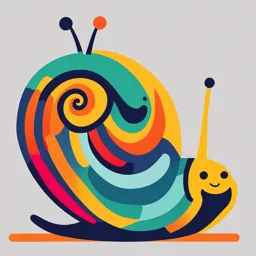
Uploaded by HeartwarmingOnyx8321
Tags
Summary
This document discusses parallel algorithms techniques. It covers divide-and-conquer, partitioning, and their application to problems like matrix multiplication and image processing. The document also provides examples and scenarios to illustrate the concepts and techniques.
Full Transcript
1. ### Divide-and-Conquer in Parallel Algorithms The **divide-and-conquer** approach is a key technique in parallel computing, enabling complex problems to be broken down into smaller, more manageable subproblems. Each subproblem is solved independently, which aligns well with parallel processing....
1. ### Divide-and-Conquer in Parallel Algorithms The **divide-and-conquer** approach is a key technique in parallel computing, enabling complex problems to be broken down into smaller, more manageable subproblems. Each subproblem is solved independently, which aligns well with parallel processing. ## Concept of Divide-and-Conquer in Parallel Algorithms The divide-and-conquer approach involves three main steps: 1. **Divide**: The problem is divided into smaller, independent subproblems. 2. **Conquer**: Each subproblem is solved, ideally in parallel. 3. **Combine**: The solutions to the subproblems are combined to form the solution to the original problem. In parallel computing, divide-and-conquer allows multiple processors or cores to work on different subproblems simultaneously. This can significantly speed up computations and is effective for problems that can be broken down into independent tasks. ### Example A classic example is **Merge Sort**: 1. **Divide**: Split the array into two halves. 2. **Conquer**: Recursively sort each half in parallel. 3. **Combine**: Merge the sorted halves. Each recursive call in Merge Sort can be handled by a separate processor, making it an ideal candidate for parallel execution. ## Characteristics of Divide-and-Conquer 1. **Parallelism**: The method enables natural parallelization since subproblems are independent and can be solved concurrently. 2. **Recursive Structure**: Divide-and-conquer algorithms are typically recursive, breaking down problems into subproblems until they reach a base case. 3. **Optimal for Multicore Architectures**: Since each core can independently process a subproblem, divide-and-conquer algorithms are optimized for multi-core processors and distributed systems. 4. **Balanced Load Distribution**: If subproblems are of similar sizes, work is balanced across processors, improving resource utilization. ## Applications of Divide-and-Conquer in Parallel Computing 1. **Sorting Algorithms**: Quick Sort, Merge Sort, and Bitonic Sort can be effectively parallelized. 2. **Matrix Multiplication**: Algorithms like Strassen’s Matrix Multiplication use divide-and- conquer to recursively split and multiply submatrices in parallel. 3. **Fast Fourier Transform (FFT)**: FFT uses divide-and-conquer to transform data, essential in signal processing. 4. **Binary Search**: The search space is halved with each step, making it an example of divide- and-conquer that can be parallelized for distributed databases. --- ## Advantages of Divide-and-Conquer in Parallel Algorithms 1. **Improved Performance**: By breaking down a problem and solving subproblems concurrently, divide-and-conquer reduces execution time, especially on large-scale problems. 2. **Enhanced Scalability**: Divide-and-conquer algorithms are highly scalable, allowing them to adapt well to an increased number of processors. 3. **Memory Efficiency**: Each subproblem can often work within a smaller memory footprint, which is efficient in parallel architectures. 4. **Reduced Complexity**: Breaking a problem into simpler subproblems can make it easier to understand and optimize. ## Complexity Analysis - **Time Complexity**: Typically, divide-and-conquer algorithms achieve a time complexity of \(O(n \log n)\) for problems like sorting. - **Space Complexity**: This depends on the level of recursion and the number of processors. It is often \(O(n)\) for algorithms like Merge Sort in sequential execution but can vary in parallel environments based on memory access patterns. --- ### Summary Divide-and-conquer in parallel computing offers a structured and efficient approach for solving large and complex problems. Its recursive, independently parallelizable nature allows for balanced load distribution and efficient resource utilization. This approach is instrumental in applications across data processing, scientific computing, and machine learning, making it a cornerstone of modern parallel algorithm design. Some other Important Techniques ### 1. Partitioning **Partitioning** in parallel computing involves dividing data or tasks into smaller chunks that can be processed independently by multiple processors or cores. This is done to reduce dependencies and allow multiple operations to be executed concurrently, increasing performance and scalability. - **Types of Partitioning**: - **Data Partitioning**: Data is divided across processors; each processor performs the same operation on its subset of data. - **Task Partitioning**: The computation tasks themselves are divided, and each processor performs a unique subset of tasks on shared or independent data. Example Example 1: In a **matrix multiplication** task, we can partition the matrix rows across multiple processors. Each processor computes the product for its assigned rows, independently, and the results are combined at the end. Example 2 Scenario: Let's say we have a large dataset of images, and we want to apply a filter (like sharpening) to each image. Data Partitioning: o We can divide the images among multiple processors, where each processor independently applies the filter to its assigned images. o Suppose there are 1000 images and 4 processors; each processor would handle 250 images. o As each processor operates independently, all processors work simultaneously on their subset, thus reducing the total processing time. Task Partitioning: o Suppose we need to perform a series of image processing tasks (resizing, filtering, and color correction) on each image. o We could assign one task (e.g., resizing) to one processor, filtering to another, and color correction to a third. o Each processor works on the same set of images, applying a specific operation, and once complete, passes the output to the next processor. o This setup ensures that each processor specializes in a specific task, which can improve performance for complex workflows. Advantages: Partitioning improves performance by spreading workload among multiple processors, thus handling more data in less time. - **Advantages**: - Increases computation speed by parallel execution. - Reduces idle time by balancing workload among processors. --- ### 2. Pipelining **Pipelining** is a parallelization technique where tasks are divided into sequential stages, and each stage is executed concurrently with different input data. This allows tasks to be processed in a "pipeline," similar to an assembly line. - **How It Works**: Each stage of the pipeline performs part of the computation and then passes the output to the next stage. Once the first stage is complete, it begins processing a new input, and each subsequent stage follows in a staggered manner. - **Example**: In a **data processing system**, pipelining can be applied to image processing: 1. Stage 1 reads image data. 2. Stage 2 applies a filter. 3. Stage 3 enhances colors. 4. Stage 4 saves the processed image. While Stage 1 starts on a new image, Stages 2, 3, and 4 can work on previously started images, allowing concurrent work. Another Example Scenario: Consider a web server that handles user requests for processing images uploaded by users. Stages: o Stage 1: Accepts and reads the image file. o Stage 2: Resizes the image to standard dimensions. o Stage 3: Applies a watermark for branding. o Stage 4: Compresses the image for storage. o Stage 5: Stores the image in the database and sends back a response to the user. Pipeline Process: o As soon as Stage 1 completes processing the first image, Stage 2 can begin resizing it. While Stage 2 is resizing the first image, Stage 1 can start reading a second image. o This overlapping process continues, allowing the web server to process multiple images simultaneously in different stages. Benefit: By pipelining the tasks, the server reduces overall processing time and efficiently handles multiple user requests concurrently. Advantages: Pipelining allows each stage to continuously process new data as soon as it is free, keeping all processors busy and minimizing idle time. - **Advantages**: - Improves efficiency by keeping all stages busy. - Reduces waiting time as multiple stages process data concurrently. ### 3. Accelerated Cascading **Accelerated Cascading** is a technique used in parallel computation to quickly "cascade" or propagate computed results from one level to another. It speeds up computations by forwarding partial results to further stages, allowing for an expedited response when tasks are interconnected or hierarchical. - **How It Works**: Accelerated cascading leverages early completion of some results to start subsequent computations. Instead of waiting for all data at one level to complete before moving on, it allows for results to flow as they are ready. Example - **Example 1**: In **machine learning model training**, where layers in a neural network depend on the output of previous layers, accelerated cascading could allow certain computations in the later layers to begin before the entire previous layer is processed. - **Advantages**: - Reduces total computation time. - Makes hierarchical tasks faster by overlapping stages based on partial completion. Example 2 Scenario: Suppose we are training a deep neural network for object detection with 5 layers, where each layer depends on the output of the previous one. Process: o Usually, each layer would wait for the complete output from the previous layer. However, in accelerated cascading, partial outputs from a layer are forwarded to the next layer as soon as they are ready. o For example, once the first few neurons of Layer 1 produce output, Layer 2 can start processing based on this partial data rather than waiting for Layer 1 to finish entirely. o This continues for each subsequent layer, allowing the network to "cascade" processing across layers more quickly. Benefit: This reduces the time needed for the entire forward pass and thus shortens the overall training time. Advantages: Accelerated cascading enables higher efficiency by overlapping operations across layers, which is beneficial for deep learning models and hierarchical processes. ### 4. Symmetry Breaking **Symmetry Breaking** is a concept used in parallel and distributed computing to resolve conflicts or make decisions where identical states would otherwise lead to indefinite waiting or cycles. It "breaks" the symmetry by forcing unique decisions, ensuring progress. - **How It Works**: In distributed systems, nodes (or processors) may need to make independent choices, such as electing a leader or assigning resources. Symmetry-breaking mechanisms introduce randomness or predefined rules to resolve conflicts when nodes are in identical states. - **Example 1**: In a **distributed network** where multiple nodes attempt to become the network leader, each node might randomly generate a unique identifier or use a pre-established hierarchy to decide the leader. This breaks symmetry, ensuring only one leader is chosen. - **Advantages**: - Prevents deadlock situations where processes wait indefinitely. - Ensures fair and predictable outcomes in distributed systems. Example 2 Scenario: Imagine a distributed network of computers trying to elect a leader to manage shared resources, such as scheduling tasks or coordinating data transfers. Problem: o All nodes in the network start in the same state with identical information and could all try to elect themselves as the leader simultaneously, resulting in a deadlock. Symmetry-Breaking Solution: o To resolve this, each node generates a random unique identifier (e.g., a random number) or uses a pre-set ID. o The nodes then share their identifiers with each other, and the node with the highest identifier is elected as the leader. o If two nodes have the same identifier, they break the tie with another random number until one leader is established. Example: Suppose we have four nodes with IDs [A, B, C, D] and each generates a random identifier: A=15, B=10, C=12, and D=20. Node D has the highest identifier and becomes the leader. Advantages: Symmetry breaking prevents indefinite conflicts and ensures that distributed systems can proceed without deadlock, especially useful in decentralized networks where coordination needs to be both fair and efficient. --- Each of these techniques — **partitioning, pipelining, accelerated cascading, and symmetry breaking** — plays a crucial role in parallel computing, enhancing system performance, reducing processing time, and ensuring that concurrent processes are managed effectively. They are particularly powerful when applied in high-performance computing, distributed systems, and large-scale data processing applications.