Chapter 3 Processing Data PDF
Document Details
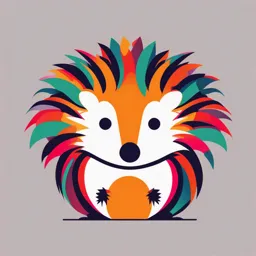
Uploaded by TougherEinsteinium
Tags
Related
- C# Basic Structure, Identifiers, Data Types, and Operators PDF
- L6-L9-Variables, Data types, sizes and constants .pptx
- L6-L9-Variables, Data types, sizes and constants .pdf
- L6-L9-Variables, Data types, sizes and constants .pdf
- SYSC 2006 Lecture 2: Variables, Data Types, and Expressions PDF
- C Programming PDF
Summary
This chapter covers basic C# data processing concepts, including inputting data using TextBox controls, variables (especially string variables), and string concatenation. Examples and explanations of these concepts are provided.
Full Transcript
ChaPtEr 3 Processing Data TOPICS 3.1 reading input with textBox Controls 3.7 Simple Exception handling 3.2 a First Look at Variables 3.8 using named Constants 3.3 numeric Data types and Variables...
ChaPtEr 3 Processing Data TOPICS 3.1 reading input with textBox Controls 3.7 Simple Exception handling 3.2 a First Look at Variables 3.8 using named Constants 3.3 numeric Data types and Variables 3.9 Declaring Variables as Fields 3.4 Performing Calculations 3.10 using the Math Class 3.5 inputting and Outputting numeric 3.11 More gui Details Values 3.12 using the Debugger to Locate Logic 3.6 Formatting numbers with the Errors ToString Method 3.1 Reading Input with TextBox Controls CONCEPT: The TextBox control is a rectangular area that can accept keyboard input from the user. Many of the programs that you will write from this point forward will require the user to enter data. The data entered by the user will then be used in some sort of operation. One of the primary controls that you will use to get data from the user is the TextBox control. A TextBox control appears as a rectangular area on a form. When the application is run- ning, the user can type text into a TextBox control. The program can then retrieve the text that the user entered and use that text in any necessary operations. In the Toolbox, the TextBox tool is located in the Common Controls group. When you double-click the tool, a TextBox control is created on the form, as shown in Figure 3-1. When you create TextBox controls, they are automatically given default names such as textBox1, textBox2, and so forth. As you learned in Chapter 2, you should always change a control’s default name to something more meaningful. When the user types into a TextBox control, the text is stored in the control’s Text prop- erty. In code, if you want to retrieve the data that has been typed into a TextBox, you simply retrieve the contents of the control’s Text property. 117 118 Chapter 3 Processing Data Figure 3-1 a textBox control NOTE: When you retrieve the contents of the Text property, you always get a string. Any operation that can be performed on a string can be performed on a control’s Text property. Let’s look at an example. Make sure you have downloaded the student sample programs from the book’s companion Web site (at www.pearsonhighered.com/gaddis). In the Chap03 folder, you will find a project named TextBox Demo. Figure 3-2 shows the form, with most of the control names specified, and Figure 3-3 shows the form’s code. (In Figure 3-3, to conserve space on the page, we have scrolled past the using directives that appear at the top of the code file.) Notice in Figure 3-3 that the readInputButton control’s Click event handler performs the following assignment statement: outputLabel.Text = nameTextBox.Text; This statement assigns the value of the nameTextBox control’s Text property to the outputLabel control’s Text property. In other words, it gets any text that has been entered by the user into the nameTextBox control and displays it in the outputLabel control. If you run the application, Figure 3-4 shows an example of how the form appears after you have entered Kathryn Smith and clicked the readInputButton control. Figure 3-2 the TextBox Demo application nameTextBox outputLabel readInputButton exitButton 3.1 reading input with textBox Controls 119 Figure 3-3 the form’s code (excluding the using directives) Figure 3-4 the user’s name displayed in the label Clearing the Contents of a TextBox Control You can clear the contents of a TextBox control in the same way that you clear the con- tents of a Label control: you assign an empty string ("") to the control’s Text property. For example, the following statement clears the contents of the nameTextBox control: nameTextBox.Text = ""; When this statement executes, the nameTextBox control will appear empty on the appli- cation’s form. Checkpoint 3.1 What control can be used to gather text input from the user? 3.2 In code, how do you retrieve data that has been typed into a TextBox control? 3.3 What type of data does a control’s Text property always contain? 3.4 How do you clear the contents of a TextBox control? 120 Chapter 3 Processing Data 3.2 A First Look at Variables CONCEPT: A variable is a storage location in memory that is represented by a name. Most programs store data in the computer’s memory and perform operations on that data. For example, consider the typical online shopping experience: you browse a Web site and add the items that you want to purchase to the shopping cart. As you add items to the shopping cart, data about those items is stored in memory. Then, when you click the checkout button, a program running on the Web site’s computer calculates the cost of all the items you have in your shopping cart, applicable sales taxes, shipping costs, and the total of all these charges. When the program performs these calculations, it stores the results in the computer’s memory. Programs use variables to store data in memory. A variable is a storage location in mem- ory that is represented by a name. For example, a program that manages a company’s customer mailing list might use a variable named lastName to hold a customer’s last name, a variable named firstName to hold the customer’s first name, a variable named address to hold the customer’s mailing address, and so forth. In C#, you must declare a variable in a program before you can use it to store data. You do this with a variable declaration, which specifies two things about the variable: 1. The variable’s data type, which is the type of data the variable will hold 2. The variable’s name A variable declaration statement is written in this general format: DataType VariableName; Let’s take a closer look at each of these. Data Type A variable’s data type indicates the type of data that the variable will hold. Before you declare a variable, you need to think about the type of value that will be stored in the variable. For example, will the variable hold a string or a number? If it will hold a number, what kind of number will it be, an integer or a real number? When you have determined the kind of data that the variable will hold, you select one of the data types that C# provides for a variable. The C# language provides many data types for storing fundamental types of data, such as strings, integers, and real numbers. These data types are known as primitive data types. We will look at several of them in this chapter. Variable Name A variable name identifies a variable in the program code. When naming a variable, you should always choose a meaningful name that indicates what the variable is used for. For example, a variable that holds the temperature might be named temperature, and a variable that holds a car’s speed might be named speed. You may be tempted to give variables short, nondescript names such as x or b2, but names such as these give no clue as to the purpose of the variable. In addition, there are some specific rules that you must follow when naming a variable. The same rules for identifiers that apply to control names also apply to variable names. We discussed these rules in Chapter 2, but we review them now: The first character must be one of the letters a through z or A through Z or an underscore character ( _ ). 3.2 a First Look at Variables 121 After the first character, you may use the letters a through z or A through Z, the digits 0 through 9, or underscores. The name cannot contain spaces. When naming variables, we use the same camelCase naming convention that we intro- duced in Chapter 2 for control names. For example, if we are declaring a variable to hold an employee’s gross pay, we might name it grossPay. Or, if are declaring a variable to a customer number, we might name it customerNumber. string Variables The first primitive data type we consider is the string data type. A variable of the string data type can hold any string of characters, such as a person’s name, address, password, and so forth. Here is an example of a statement that declares a string variable named productDescription: string productDescription; After the variable has been declared, you can use the assignment operator (=) to store a value in the variable. Here is an example: productDescription = "Italian Espresso Machine"; When this statement executes, the string literal "Italian Espresso Machine" is assigned to the productDescription variable. When writing an assignment statement, remember that the assignment operator assigns the value that appears on its right side to the variable that appears on its left side. Once you have assigned a value to a variable, you can use the variable in other opera- tions. For example, assume productLabel is the name of a Label control. The follow- ing statement assigns the productDescription string to the productLabel control’s Text property: productLabel.Text = productDescription; After this statement executes, the string that is stored in the productDescription variable is displayed in the productLabel control. The following statement shows another example: MessageBox.Show(productDescription); When this statement executes, the string that is stored in the productDescription vari- able is displayed in a message box. String Concatenation A common operation that performed on strings is concatenation, or appending one string to the end of another string. In C# you use the + operator to concatenate strings. The + operator produces a string that is the combination of the two strings used as its operands. The following code shows an example: string message; message = "Hello " + "world"; MessageBox.Show(message); The first statement declares a string variable named message. The second statement combines the strings "Hello " and "world" to produce the string "Hello world". The string "Hello world" is then assigned to the message variable. The third statement dis- plays the contents of the message variable in a message box. When the message box is displayed, it shows the string Hello world. 122 Chapter 3 Processing Data Let’s look at an application that further demonstrates string concatenation. In the Chap03 folder of this book’s student sample programs (available for download at www.pearson- highered.com/gaddis), you will find a project named String Variable Demo. Figure 3-5 shows the form, with most of the control names specified, and Figure 3-6 shows the form’s code. (In Figure 3-6, to conserve space on the page, we have scrolled past the using directives that appear at the top of the code file.) Figure 3-5 the String Variable Demo application firstNameTextBox lastNameTextBox fullNameLabel showNameButton exitButton Figure 3-6 the form’s code (excluding the using directives) 1 2 3 In Figure 3-6, three statements in the showNameButton_Click event handler are pointed out: 1 This statement is a variable declaration. It declares a string variable named fullName. 2 This statement assigns the result of a string concatenation to the fullName variable. The string that is assigned to the variable begins with the value of the firstNameTextBox control’s Text property, followed by a space (" "), followed by the value of the lastNameTextBox control’s Text property. For example, if the user has entered Joe into the firstNameTextBox control and Smith into the lastNameTextBox control, this statement will assign the string "Joe Smith" to the fullName variable. 3.2 a First Look at Variables 123 3 This statement assigns the fullName variable to the fullNameLabel control’s Text property. As a result, the string that is stored in the fullName variable is displayed in the fullNameLabel control. If you run the application, Figure 3-7 shows an example of how the form appears after you have entered Chris for the first name and Jones for the last name and clicked the showNameButton control. Figure 3-7 the user’s full name displayed in the label Declaring Variables before Using Them The purpose of a variable declaration statement is to tell the compiler that you plan to use a variable of a specified name to store a particular type of data in the program. A variable declaration statement causes the variable to be created in memory. For this reason, a variable’s declaration statement must appear before any other statements in the method that use the variable. This makes perfect sense because you cannot store a value in a variable if the variable has not been created in memory. Local Variables Notice that the fullName variable in Figure 3-6 is declared inside the event handler method. Variables that are declared inside a method are known as local variables. A local variable belongs to the method in which it is declared, and only statements inside that method can access the variable. (The term local is meant to indicate that the variable can be used only locally, within the method in which it is declared.) An error will occur if a statement in one method tries to access a local variable that belongs to another method. For example, let’s go over the sample code shown in Figure 3-8: 1 This statement declares a string variable named myName. The variable is declared inside the firstButton_Click event handler, so it is local to that method. Figure 3-8 One method trying to access a variable that is local to another method 1 2 3 ERROR! 124 Chapter 3 Processing Data 2 This statement, which is also in the firstButton_Click event handler, assigns the nameTextBox control’s Text property to the myName variable. 3 This statement, which is in the secondButton_Click event handler, attempts to assign the myName variable to the outputLabel control’s Text property. This statement will not work, however, because the myName variable is local to the firstButton_Click event handler, and statements in the secondButton_Click event handler cannot access it. Scope of a Variable Programmers use the term scope to describe the part of a program in which a variable may be accessed. A variable is visible only to statements inside the variable’s scope. A local variable’s scope begins at the variable’s declaration and ends at the end of the method in which the variable is declared. As you saw in the previous example, a local variable cannot be accessed by statements that are outside the method. In addition, a local variable cannot be accessed by code that is inside the method but before the varia- ble’s declaration. Lifetime of a Variable A variable’s lifetime is the time period during which the variable exists in memory while the program is executing. A local variable is created in memory when the method in which it is declared starts executing. When the method ends, all the method’s local varia- bles are destroyed. So, a local variable’s lifetime is the time during which the method in which it is declared is executing. Duplicate Variable Names You cannot declare two variables with the same name in the same scope. For example, if you declare a variable named productDescription in an event handler, you cannot declare another variable with that name in the same event handler. You can, however, have variables of the same name declared in different methods. Assignment Compatibility You can assign a value to a variable only if the value is compatible with the variable’s data type. Only strings are compatible with the string data type, so all the assignments in the following code sample work: 1 // Declare and initialize a string variable. 2 string productDescription = "Chocolate Truffle"; 3 4 // Declare another string variable. 5 string myFavoriteProduct; 6 7 // Assign a value to a string variable. 8 myFavoriteProduct = productDescription; 9 10 // Assign a value from a TextBox to a string variable. 11 productDescription = userInputTextBox.Text; The following comments explain these lines of code: In line 2 we initialize a string variable with a string literal. This works because string literals are assignment compatible with string variables. 3.2 a First Look at Variables 125 In line 8, we assign a string variable to another string variable. This works for the obvious reason that string variables are compatible with other string variables. Assume that the application has a TextBox control named userInputTextBox. In line 11, we assign the value of the TextBox control’s Text property to a string variable. This works because the value in a control’s Text property is always a string. The following code will not work, however, because it attempts to assign a nonstring value to a string variable: 1 // Declare a string variable. 2 string employeeID; 3 4 // Assign a value to the variable. Will this work? 5 employeeID = 125; d ERROR! In line 5, we are attempting to assign the number 125 to a string variable. Numbers are not assignment compatible with string variables, so this statement will cause an error when the code is compiled. NOTE: Although you cannot store the number 125 in a string variable, you can store the string literal "125" in a string variable. A Variable Holds One Value at a Time Variables can hold different values while a program is running, but they can hold only one value at a time. When you assign a value to a variable, that value will remain in the variable until you assign a different value to the variable. For example, look at the follow- ing code sample: 1 // Declare a string variable. 2 string productDescription; 3 4 // Assign a value to the variable. 5 productDescription = "Large Medium-Roast Coffee"; 6 7 // Display the variable’s value. 8 MessageBox.Show(productDescription); 9 10 // Assign a different value to the variable. 11 productDescription = "Chocolate Truffle"; 12 13 // Display the variable’s value. 14 MessageBox.Show(productDescription); The following comments explain what we did: Line 2 declares a string variable named productDescription. Line 5 assigns the string "Large Medium-Roast Coffee" to the productDescription variable. Line 8 displays the value of the productDescription variable in a message box. (The message box will display Large Medium-Roast Coffee.) Line 11 assigns a different value to the productDescription variable. After this statement executes, the productDescription variable will hold the string "Chocolate Truffle". Line 14 displays the value of the productDescription variable in a message box. (The message box will display Chocolate Truffle.) 126 Chapter 3 Processing Data This code sample illustrates two important characteristics of variables: A variable holds only one value at a time. When you store a value in a variable, that value replaces the previous value that was in the variable. Tutorial 3-1 gives you some practice using variables. You will create an application that uses TextBox controls to get input values, stores those values in variables, and uses the variables in operations. Tutorial 3-1: the Birth Date String application In this tutorial, you create an application that lets the user enter the following information VideoNote about his or her birthdate: Tutorial 3-1: The Birth The day of the week (Monday, Tuesday, etc.) Date String The name of the month (January, February, etc.) Application The numeric day of the month The year Figure 3-9 shows the application’s form, along with the names of all the controls. When the application runs, the user enters each piece of data into a separate TextBox. When the user clicks the Show Date button, the application concatenates the contents of the TextBoxes into a string such as Friday, June 1, 1990. The string is displayed in the dateOutputLabel control. When the user clicks the Clear button, the contents of the TextBoxes and the dateOutputLabel control are cleared. The Exit button closes the application’s form. Figure 3-9 the Birth Date String form dayOfWeekPromptLabel dayOfWeekTextBox monthPromptLabel monthTextBox dayOfmonthPromptLabel dayOfMonthTextBox yearPromptLabel yearTextBox dateOutputLabel showDateButton clearButton exitButton Step 1: Start Visual Studio and begin a new Windows Forms Application project named Birth Date String. Step 2: Set up the application’s form as shown in Figure 3-9. Notice that the form’s Text property is set to Birth Date String. The names of the controls are shown in the figure. As you place each control on the form, refer to Table 3-1 for the relevant property settings. 3.2 a First Look at Variables 127 Table 3-1 Control property settings Control Name Control Type Property Settings dayOfWeekPromptLabel Label Text: Enter the day of the week monthPromptLabel Label Text: Enter the name of the month dayOfMonthPromptLabel Label Text: Enter the numeric day of the month yearPromptLabel Label Text: Enter the year dayOfWeekTextBox TextBox No properties changed monthTextBox TextBox No properties changed dayOfMonthTextBox TextBox No properties changed yearTextBox TextBox No properties changed dateOutputLabel Label AutoSize: False BorderStyle: FixedSingle Text: (The contents of the Text property have been erased.) TextAlign: MiddleCenter showDateButton Button Text: Show Date clearButton Button Text: Clear exitButton Button Text: Exit Step 3: Once you have set up the form with its controls, you can create the Click event handlers for the Button controls. At the end of this tutorial, Program 3-1 shows the completed code for the form. You will be instructed to refer to Program 3-1 as you write the event handlers. (Remember, the line numbers that are shown in Program 3-1 are not part of the program. They are shown for reference only.) In the Designer, double-click the showDateButton control. This will open the code editor, and you will see an empty event handler named showDateButton_ Click. Complete the showDateButton_Click event handler by typing the code shown in lines 22–32 in Program 3-1. Let’s take a closer look at the code: Line 23: This statement declares a string variable named output. Lines 26–29: These lines are actually one long statement, broken up into mul- tiple lines. The statement concatenates the Text properties of the TextBox controls, along with appropriately placed commas and spaces, to create the date string. The resulting string is assigned to the output variable. For example, suppose the user has entered the following input: Friday in the dayOfWeekTextBox control. June in the monthTextBox control. 1 in the dayOfMonthTextBox control. 1990 in the yearTextBox control. The concatenation in this statement produces the string “June 1, 1990”; it is assigned to the output variable. Line 32: This statement assigns the output variable to the dateOutputLabel control’s Text property. When this statement executes, the contents of the output variable are displayed in the dateOutputLabel control. 128 Chapter 3 Processing Data Step 4: Switch your view back to the Designer and double-click the clearButton control. In the code editor, you will see an empty event handler named clearButton_Click. Complete the clearButton_Click event handler by typing the code shown in lines 37–44 in Program 3-1. Let’s take a closer look at the code: Lines 38–41: Each statement assigns an empty string ("") to the Text prop- erty of one of the TextBox controls. When these statements have finished executing, the TextBox controls will appear empty. Line 44: This statement assigns an empty string ("") to the dateOutputLabel control’s Text property. After the statement has executed, the label appears empty. Step 5: Switch your view back to the Designer and double-click the exitButton control. In the code editor, you will see an empty event handler named exit- Button_Click. Complete the exitButton_Click event handler by typing the code shown in lines 49–50 in Program 3-1. Step 6: Save the project. Then, press the F5 key on the keyboard, or click the Start Debugging button ( ) on the toolbar to compile and run the application. The form will appear as shown in the image on the left in Figure 3-10. Test the application by entering values into the TextBoxes and clicking the Show Date button. The date should be displayed, similar to the image shown on the right in the figure. Click the Clear button, and the contents of the TextBoxes and the Label control should clear. Click the Exit button and the form should close. Figure 3-10 the Birth Date String application Program 3-1 Completed Form1 code for the Birth Date String application 1 using System; 2 using System.Collections.Generic; 3 using System.ComponentModel; 4 using System.Data; 5 using System.Drawing; 6 using System.Linq; 7 using System.Text; 8 using System.Threading.Tasks; 9 using System.Windows.Forms; 10 11 namespace Birth_Date_String 3.2 a First Look at Variables 129 12 { 13 public partial class Form1 : Form 14 { 15 public Form1() 16 { 17 InitializeComponent(); 18 } 19 20 private void showDateButton_Click(object sender, EventArgs e) 21 { 22 // Declare a string variable. 23 string output; 24 25 // Concatenate the input and build the output string. 26 output = dayOfWeekTextBox.Text + ", " + 27 monthTextBox.Text + " " + 28 dayOfMonthTextBox.Text + ", " + 29 yearTextBox.Text; 30 31 // Display the output string in the Label control. 32 dateOutputLabel.Text = output; 33 } 34 35 private void clearButton_Click(object sender, EventArgs e) 36 { 37 // Clear the TextBoxes. 38 dayOfWeekTextBox.Text = ""; 39 monthTextBox.Text = ""; 40 dayOfMonthTextBox.Text = ""; 41 yearTextBox.Text = ""; 42 43 // Clear the dateOutputLabel control. 44 dateOutputLabel.Text = ""; 45 } 46 47 private void exitButton_Click(object sender, EventArgs e) 48 { 49 // Close the form. 50 this.Close(); 51 } 52 } 53 } NOTE: In Tutorial 3-1, the statement in lines 26–29 shows an example of how you can break up a statement into multiple lines. Quite often, you will find yourself writ- ing statements that are too long to fit entirely inside the Code window. Your code will be hard to read if you have to horizontally scroll the Code window to view long state- ments. In addition, if you or your instructor chooses to print your code, the state- ments that are too long to fit on one line of the page will wrap around to the next line and make your code look unorganized. For these reasons, it is usually best to break a long statement into multiple lines. When typing most statements, you can simply press the Enter key when you reach an appropriate point to continue the statement on the next line. Remember, however, that you cannot break up a keyword, a quoted string, or an identifier (such as a vari- able name or a control name). 130 Chapter 3 Processing Data Initializing Variables In C#, a variable must be assigned a value before it can be used. For example, look at this code: string productDescription; MessageBox.Show(productDescription); This code declares a string variable named productDescription and then tries to dis- play the variable’s value in a message box. The only problem is that we have not assigned a value to the variable. When we compile the application containing this code, we will get an error message such as Use of unassigned local variable ‘productDescription’. The C# compiler will not compile code that tries to use an unassigned variable. One way to make sure that a variable has been assigned a value is to initialize the vari- able with a value when you declare it. For example, the following statement declares a string variable named productDescription and immediately assigns the string literal "Chocolate Truffle" to it: string productDescription = "Chocolate Truffle"; We say that this statement initializes the productDescription variable with the string "Chocolate Truffle". Here is another example: string lastName = lastNameTextBox.Text; Assume that this statement belongs to an application that has a TextBox named lastNameTextBox. The statement declares a string variable named lastName and initializes it with the value of the lastNameTextBox control’s Text property. Declaring Multiple Variables with One Statement You can declare multiple variables of the same data type with one declaration statement. Here is an example: string lastName, firstName, middleName; This statement declares three string variables named lastName , firstName , and middleName. Notice that commas separate the variable names. Here is an example of how we can declare and initialize the variables with one statement: string lastName = "Jones", firstName = "Jill", middleName = "Rebecca"; Remember, you can break up a long statement so it spreads across two or more lines. Sometimes you will see long variable declarations written across multiple lines, like this: string lastName = "Jones", firstName = "Jill", middleName = "Rebecca"; Checkpoint 3.5 What is the purpose of a variable? 3.6 Give an example of a variable declaration that will store the name of your favorite food. 3.3 numeric Data types and Variables 131 3.7 For each of the following items, determine whether the data type should be an integer, string, or real number. a. pet name b. sales tax c. mailing address d. video game score 3.8 Indicate whether each of the following is a legal variable name. If it is not, explain why. a. pay_Rate b. speed of sound c. totalCost d. 2ndPlaceName 3.9 What will be stored in the message variable after the following statement is executed? string message = "He" + "ll" + "o!"; 3.10 What is the lifetime of a variable that is declared inside of a Click event handler? 3.11 Assuming the variable greeting has not been assigned a value, what will be the result of the following statement? MessageBox.Show(greeting); 3.12 Will the following statement cause an error? Why or why not? string luckyNumber = 7; 3.13 Write a single declaration statement for the variables name, city, and state. 3.3 Numeric Data Types and Variables CONCEPT: If you need to store a number in a variable and use that number in a mathematical operation, the variable must be of a numeric data type. You select a numeric data type that is appropriate for the type of number that you need to store. In the previous section, you read about string variables. Variables of the string data type can be used to store text, but they cannot store numeric data for the purpose of per- forming mathematical operations. If you need to store numbers and perform mathemati- cal operations on them, you have to use a numeric data type. The C# language provides several primitive data types. You can read about all the C# primitive data types in Appendix A. Many of the data types provided by C# are for spe- cialized purposes beyond the scope of this book. When it comes to numeric data, most of the time you will use the three numeric primitive data types described in Table 3-2. Here are examples of declaring variables of each data type: int speed; double distance; decimal grossPay; The first statement declares an int variable named speed. The second example declares a double variable named distance. The third statement declares a decimal variable named grossPay. 132 Chapter 3 Processing Data Table 3-2 the primitive numeric data types that you will use most often Data Type Description int A variable of the int data type can hold whole numbers only. For example, an int variable can hold values such as 42, 0, and −99. An int variable cannot hold numbers with a fractional part, such as 22.1 or −4.9. The int data type is the primary data type for storing integers. We use it in this book any time we need to store and work with integers. An int variable uses 32 bits of memory and can hold an integer number in the range of −2,147,483,648 through 2,147,483,647. double A variable of the double data type can hold real numbers, such as 3.5, −87.95, or 3.0. A number that is stored in a double variable is rounded to 15 digits of precision. We use variables of the double data type to store any number that might have a fractional part. The double data type is especially useful for storing extremely great or extremely small numbers. In memory a double variable uses 64 bits of memory. It is stored in a format that programmers call double precision floating-point notation. Variables of the double data type can hold numbers in the range of ±5.0 × 102324 to ±1.7 × 10308. decimal A variable of the decimal data type can hold real numbers with greater precision than the double data type. A number that is stored in a decimal variable is rounded to 28 digits of precision. Because decimal variables store real numbers with a great deal of precision, they are most commonly used in financial applications. In this book, we typically use the decimal data type when storing amounts of money. In memory a decimal variable uses 128 bits of memory. It is stored in a format that programmers call decimal notation. Variables of the decimal data type can hold numbers in the range of ±1.0 × 10228 to ±7.9 × 1028. Numeric Literals You learned in Chapter 2 that a literal is a piece of data written into a program’s code. When you know, at the time that you are writing a program’s code, that you want to store a specific value in a variable, you can assign that value as a literal to the variable. A numeric literal is a number that is written into a program’s code. For example, the fol- lowing statement declares an int variable named hoursWorked and initializes it with the value 40: int hoursWorked = 40; In this statement, the number 40 is a numeric literal. The following shows another example: double temperature = 87.6; 3.3 numeric Data types and Variables 133 This statement declares a double variable named temperature and initializes it with the value 87.6. The number 87.6 is a numeric literal. When you write a numeric literal in a program’s code, the numeric literal is assigned a data type. In C#, if a numeric literal is an integer (not written with a decimal point) and it fits within the range of an int (see Table 3-2 for the minimum and maximum values), then the numeric literal is treated as an int. A numeric literal that is treated as an int is called an integer literal. For example, each of the following statements initializes a variable with an integer literal: int hoursWorked = 40; int unitsSold = 650; int score = −23; If a numeric literal is written with a decimal point and it fits within the range of a double (see Table 3-2 for the minimum and maximum values), then the numeric literal is treated as a double. A numeric literal that is treated as a double is called a double literal. For example, each of the following statements initializes a variable with a double literal: double distance = 28.75; double speed = 87.3; double temperature = −10.0; When you append the letter M or m to a numeric literal, it is treated as a decimal and is referred to as a decimal literal. Here are some examples: decimal payRate = 28.75m; decimal price = 8.95M; decimal profit = −50m; T I P : Because decimal is the preferred data type for storing monetary amounts, remembering that “m” stands for “money” might help you to remember that decimal literals must end with the letter M or m. Assignment Compatibility for int Variables You can assign int values to int variables, but you cannot assign double or decimal values to int variables. For example, look at the following declarations: int hoursWorked = 40; d This works int unitsSold = 650m; d ERROR! int score = −25.5; d ERROR! The first declaration works because we are initializing an int variable with an int value. The second declaration causes an error, however, because you cannot assign a decimal value to an int variable. The third declaration also causes an error because you cannot assign a double value to an int variable. You cannot assign a double or a decimal value to an int variable because such an assignment could result in a loss of data. Here are the reasons: The double and decimal values may be fractional, but int variables can hold only integers. If you were allowed to store a fractional value in an int variable, the fractional part of the value would have to be discarded. 134 Chapter 3 Processing Data The double and decimal values may be much larger or much smaller than allowed by the range of an int variable. A double or a decimal number can potentially be so large or so small that it will not fit in an int variable. Assignment Compatibility for double Variables You can assign either double or int values to double variables, but you cannot assign decimal values to double variables. For example, look at the following declarations: double distance = 28.75; d This works double speed = 75; d This works double sales = 6500.0m; d ERROR! The first declaration works because we are initializing a double variable with a double value. The second declaration works because we are initializing a double variable with an int value. The third declaration causes an error, however, because you cannot assign a decimal value to a double variable. It makes sense that you are allowed to assign an int value to a double variable because any number that can be stored as an int can be converted to a double with no loss of data. When you assign an int value to a double variable, the int value is implicitly converted to a double. You cannot assign a decimal value to a double variable because the decimal data type allows for much greater precision than the double data type. A decimal value can have up to 28 digits of precision, whereas a double can provide only 15 digits of precision. Storing a decimal value in a double variable could potentially result in a loss of data. Assignment Compatibility for decimal Variables You can assign either decimal or int values to decimal variables, but you cannot assign double values to decimal variables. For example, look at the following declarations: decimal balance = 9280.73m; d This works decimal price = 50; d This works decimal sales = 6500.0; d ERROR! The first declaration works because we are initializing a decimal variable with a decimal value. The second declaration works because we are initializing a decimal variable with an int value. When you assign an int value to a decimal variable, the int value is implicitly converted to a decimal with no loss of data. The third declaration causes an error, however, because you cannot assign a double value to a decimal variable. A double value can potentially be much larger or much smaller than allowed by the range of a decimal. Explicitly Converting Values with Cast Operators Let’s consider a hypothetical situation. Suppose you’ve written an application that uses a double variable, and for some reason, you need to assign the contents of the double variable to an int variable. In this particular situation, you know that the double variable’s value is something that can be safely converted to an int without any loss of data (such as 3.0, or 98.0). However, the C# compiler will not allow you to make the assignment because double values are not assignment compatible with int variables. Isn’t there a way to override the C# rules in this particular situation and make the assignment anyway? The answer is yes, there is a way. You can use a cast operator to explicitly convert a value from one numeric data type to another, even if the conversion might result in a loss of 3.3 numeric Data types and Variables 135 data. A cast operator is the name of the desired data type, written inside parentheses and placed to the left of the value that you want to convert. The following code sample demonstrates: 1 // Declare an int variable. 2 int wholeNumber; 3 4 // Declare a double variable. 5 double realNumber = 3.0; 6 7 // Assign the double to the int. 8 wholeNumber = (int)realNumber; The following points describe the code: Line 2 declares an int variable named wholeNumber. Line 5 declares a double variable named realNumber, initialized with the value 3.0. Line 8 uses a cast operator to convert the value of realNumber to an int and assigns the converted value to wholeNumber. After this statement executes, the wholeNumber variable is assigned the value 3. Table 3-3 shows other code examples involving different types of cast operators. Table 3-3 Examples of uses of cast operators Code Example Description int wholeNumber; The (int) cast operator converts the value of the decimal moneyNumber = 4500m; moneyNumber variable to an int. The converted value wholeNumber = (int)moneyNumber; is assigned to the wholeNumber variable. double realNumber; The (double) cast operator converts the value of the decimal moneyNumber = 625.70m; moneyNumber variable to a double. The converted realNumber = (double)moneyNumber; value is assigned to the realNumber variable. decimal moneyNumber; The (decimal) cast operator converts the value of the double realNumber = 98.9; realNumber variable to a decimal. The converted moneyNumber = (decimal)realNumber; value is assigned to the moneyNumber variable. When you use a cast operator, you are essentially telling the compiler that you know what you are doing and you are willing to accept the consequences of the conversion. It is still possible that a loss of data can occur. For example, look at the following code sample: int wholeNumber; double realNumber = 8.9; wholeNumber = (int)realNumber; In this example, the double variable contains a fractional number. When the cast operator converts the fractional number to an int, the part of the number that appears after the decimal point is dropped. The process of dropping a number’s fractional part is called truncation. After this code executes, the wholeNumber variable contains the value 8. It’s important to realize that when a cast operator is applied to a variable, it does not change the contents of the variable. The cast operator merely returns the value that is stored in the variable, converted to the specified data type. In the previous code sample, when the (int) cast operator is applied to the realNumber variable, the cast operator returns the value 8. The realNumber variable remains unchanged, however, still contain- ing the value 8.9. 136 Chapter 3 Processing Data Checkpoint 3.14 Specify the appropriate primitive numeric data type to use for each of the follow- ing values: a. 24 dollars b. 12 bananas c. 14.5 inches d. 83 cents e. 2 concert tickets 3.15 Which of the following variable declarations will cause an error? Why? a. decimal payRate = 24m; b. int playerScore = 1340.5; c. double boxWidth = 205.25; d. string lastName = "Holm"; 3.16 Write a programming statement that will convert the following decimal variable to an int and store the result in an int variable named dollars: decimal deposit = 976.54m; 3.17 What value will the wholePieces variable contain after the following code executes? a. double totalPieces = 6.5; b. int wholePieces = (int)totalPieces; 3.4 Performing Calculations CONCEPT: You can use math operators to perform simple calculations. Math expressions can be written using the math operators and parentheses as grouping symbols. The result of a math expression can be assigned to a variable. Most programs require calculations of some sort to be performed. A programmer’s tools for performing calculations are math operators. C# provides the math operators shown in Table 3-4. Table 3-4 Math operators Operator Name of the Operator Description + Addition Adds two numbers − Subtraction Subtracts one number from another * Multiplication Multiplies one number by another / Division Divides one number by another and gives the quotient % Modulus Divides one integer by another and gives the remainder Programmers use the operators shown in Table 3-4 to create math expressions. A math expression performs a calculation and gives a value. The following is an example of a simple math expression: 12 * 2 3.4 Performing Calculations 137 The values on the right and left of the * operator are called operands. These are values that the * operator multiplies together. The value that is given by this expression is 24. Variables may also be used in a math expression. For example, suppose we have two variables named hoursWorked and payRate. The following math expression uses the * operator to multiply the value in the hoursWorked variable by the value in the payRate variable: hoursWorked * payRate When we use a math expression to calculate a value, we have to do something with the value. Normally we want to save the value in memory so we can use it again in the pro- gram. We do this with an assignment statement. For example, suppose we have another variable named grossPay. The following statement assigns the value hoursWorked times payRate to the grossPay variable: grossPay = hoursWorked * payRate Here are some other examples of statements that assign the result of a math expression to a variable: total = price + tax; sale = price - discount; commission = sales * percent; half = number / 2; The modulus operator (% ) performs division between two integers, but instead of returning the quotient, it returns the remainder. The following statement assigns 2 to leftOver: leftOver = 17 % 3; This statement assigns 2 to leftover because 17 divided by 3 is 5 with a remainder of 2. You will not use the modulus operator frequently, but it is useful in some situations. It is commonly used in calculations that detect odd or even numbers, determine the day of the week, or measure the passage of time and in other specialized operations. The Order of Operations You can write mathematical expressions with several operators. The following statement assigns the sum of 17, the variable x, 21, and the variable y to the variable answer. answer = 17 + x + 21 + y; Some expressions are not that straightforward, however. Consider the following statement: outcome = 12 + 6 / 3; What value will be stored in outcome? The number 6 is used as an operand for both the addition and division operators. The outcome variable could be assigned either 6 or 14, depending on when the division takes place. The answer is 14 because the order of operations dictates that the division operator works before the addition operator does. The order of operations can be summarized as follows: 1. Perform any operations that are enclosed in parentheses. 2. Perform any multiplications, divisions, or modulus operations as they appear from left to right. 3. Perform any additions or subtractions as they appear from left to right. 138 Chapter 3 Processing Data Mathematical expressions are evaluated from left to right. Multiplication and division are always performed before addition and subtraction, so the statement outcome = 12 + 6 / 3; works like this: 1. 6 is divided by 3, yielding a result of 2. 2. 12 is added to 2, yielding a result of 14. It could be diagrammed as shown in Figure 3-11. Table 3-5 shows some other sample expressions with their values. Figure 3-11 the order of operations at work outcome = 12 + 6 / 3; outcome = 12 + 2; outcome = 14; Table 3-5 Some math expressions and their values Expression Value 5 + 2 * 4 13 10 / 2 − 3 2 8 + 12 * 2 − 4 28 6 − 3 * 2 + 7 − 1 6 Grouping with Parentheses Parts of a mathematical expression may be grouped with parentheses to force some oper- ations to be performed before others. In the following statement, the variables a and b are added together, and their sum is divided by 4: result = (a + b) / 4; But what if we left the parentheses out, as shown here? result = a + b / 4; We would get a different result. Without the parentheses, b would be divided by 4 and the result added to a. Table 3-6 shows some math expressions that use parentheses and their values. Table 3-6 More expressions and their values Expression Value (5 + 2) * 4 28 10 / (5 − 3) 5 8 + 12 * (6 − 2) 56 (6 − 3) * (2 + 7) / 3 9 3.4 Performing Calculations 139 Mixing Data Types in a Math Expression When you perform a math operation on two operands, the data type of the result will depend on the data type of the operands. If the operands are of the same data type, the result will also be of that data type. For example: When an operation is performed on two int values, the result will be an int. When an operation is performed on two double values, the result will be a double. When an operation is performed on two decimal values, the result will be a decimal. It’s not uncommon, however, for a math expression to have operands of different data types. C# handles operations involving int , double , and decimal operands in the following ways: When a math expression involves an int and a double, the int is temporarily converted to a double, and the result is a double. When a math expression involves an int and a decimal, the int is temporarily converted to a decimal, and the result is a decimal. Math expressions involving a double and a decimal are not allowed unless a cast operator is used to convert one of the operands. For example, suppose a pay-calculating program has the following variable declarations: int hoursWorked; // To hold the number of hours worked decimal payRate; // To hold the hourly pay rate decimal grossPay; // To hold the gross pay Then, later in the program this statement appears: grossPay = hoursWorked * payRate; The math expression on the right side of the = operator multiplies an int by a decimal. When the statement executes, the value of the hoursWorked variable is temporarily con- verted to a decimal and then multiplied by the payRate variable. The result is a decimal and is assigned to the grossPay variable. When possible, you should avoid math operations that use a mixture of double and decimal operands. C# does not allow operations involving these two types unless you use a cast operator to explicitly convert one of the operands. For example, suppose a program that calculates the cost of a product has the following variable declarations: double weight; // The product weight decimal pricePerPound; // The price per pound decimal total; // The total cost Later in the program you need to calculate the total cost, like this: total = weight * pricePerPound; d ERROR! The compiler will not allow this statement because weight is a double and pricePerPound is a decimal. To fix the statement, you can insert a cast operator, as shown here: total = (decimal)weight * pricePerPound; The cast operator converts the value of the weight variable to a decimal, and the con- verted value is multiplied by pricePerPound. The result of the expression is a decimal and is assigned to total. 140 Chapter 3 Processing Data Integer Division When you divide an integer by an integer in C#, the result is always given as an integer. If the result has a fractional part, it is truncated. For example, look at the following code: int length; // Declare length as an int double half; // Declare half as a double length = 75; // Assign 75 to length half = length / 2; // Calculate half the length The last statement divides the value of length by 2 and assigns the result to half. Math- ematically, the result of 75 divided by 2 is 37.5. However, that is not the result that we get from the math expression. The length variable is an int, and it is being divided by the numeric literal 2, which is also treated as an int. The result of the division is truncated, which gives the value 37. This is the value that is assigned to the half variable. It does not matter that the half variable is declared as a double. The fractional part of the result is truncated before the assignment takes place. Combined Assignment Operators Sometimes you want to increase a variable’s value by a certain amount. For example, sup- pose you have a variable named number and you want to increase its value by 1. You can accomplish that with the following statement: number = number + 1; The expression on the right side of the assignment operator calculates the value of number plus 1. The result is then assigned to number, replacing the value that was previously stored there. This statement effectively adds 1 to number. For example, if number is equal to 6 before this statement executes, it is equal to 7 after the statement executes. Similarly, the following statement subtracts 5 from number: number = number − 5; If number is equal to 15 before this statement executes, it is equal to 10 after the statement executes. Here’s another example. The following statement doubles the value of the number variable: number = number * 2; If number is equal to 4 before this statement executes, it is equal to 8 after the state- ment executes. These types of operations are very common in programming. For convenience, C# offers a special set of operators known as combined assignment operators that are designed spe- cifically for these jobs. Table 3-7 shows the combined assignment operators. Table 3-7 Combined assignment operators Operator Example Usage Equivalence += x += 5; x = x + 5; −= y −= 2; y = y − 2; *= z *= 10; z = z * 10; /= a /= b; a = a / b; %= c %= 3; c = c % 3; 3.5 inputting and Outputting numeric Values 141 As you can see, the combined assignment operators do not require the programmer to type the variable name twice. Also, they give a clear indication of what is happening in the statement. Checkpoint 3.18 List the operands for the following math expression: length * width 3.19 Summarize the mathematical order of operations. 3.20 Rewrite the following code segment so that it does not cause an error. decimal pricePerFoot = 2.99m; double boardLength = 10.5; decimal totalCost = boardLength * pricePerFoot; 3.21 Assume result is a double variable. When the following statement executes, what value will be stored in result? result = 4 + 10 / 2; 3.22 Assume result is an int variable. When the following statement executes, what value will be stored in result? result = (2 + 5) * 10; 3.23 Assume result is a double variable. When the following statement executes, what value will be stored in result? result = 5 / 2; 3.24 Rewrite the following statements using combined assignment operators: a. count = count + 1; b. amount = amount – 5; c. radius = radius * 10; d. length = length / 2; 3.5 Inputting and Outputting Numeric Values CONCEPT: If the user has entered a number into a TextBox, the number will be stored as a string in the TextBox’s Text property. If you want to store that number in a numeric variable, you have to convert it to the appropriate numeric data type. When you want to display the value of a numeric variable in a Label control or a message box, you have to convert it to a string. Getting a Number from a TextBox Graphical User Interface (GUI) applications typically use TextBox controls to read keyboard input. Any data that the user enters into a TextBox control is stored in the control’s Text property as a string, even if it is a number. For example, if the user enters the number 72 into a TextBox control, the input is stored as the string "72" in the control’s Text property. If the user has entered a numeric value into a TextBox control and you want to assign that value to a numeric variable, you have to convert the control’s Text property to the desired numeric data type. Unfortunately, you cannot use a cast operator to convert a string to a numeric type. 142 Chapter 3 Processing Data To convert a string to any of the numeric data types, we use a family of methods in the.NET Framework known as the Parse methods. In computer science, the term parse typically means to analyze a string of characters for some purpose. The Parse methods are used to convert a string to a specific data type. There are several Parse methods in the.NET Framework, but because we are primarily using the int, double, and decimal numeric data types, we need only three of them: We use the int.Parse method to convert a string to an int. We use the double.Parse method to convert a string to a double. We use the decimal.Parse method to convert a string to a decimal. When you call one of the Parse methods, you pass a piece of data known as an argument into the method, and the method returns a piece of data back to you. The argument that you pass to the method is the string that you want to convert, and the piece of data that the method returns back to you is the converted value. Figure 3-12 illustrates this concept using the int.Parse method as an example. Figure 3-12 the int.Parse method Argument (the string you want to convert) An int value is returned int.Parse(string) The following code sample shows how to use the int.Parse method to convert a control’s Text property to an int. Assume that hoursWorkedTextBox is the name of a TextBox control. 1 // Declare an int variable to hold the hours worked. 2 int hoursWorked; 3 4 // Get the hours worked from the TextBox. 5 hoursWorked = int.Parse(hoursWorkedTextBox.Text); Let’s assume that the user has entered the value 40 into the hoursWorkedTextBox con- trol. In line 5 of the code sample, on the right side of the = operator is the expression int.Parse(hoursWorkedTextBox.Text). This expression calls the int.Parse method, passing the value of hoursWorkedTextBox.Text as an argument. Because the user has entered 40 into the TextBox, the string "40" is the value that is passed as the argument. The method converts the string "40" to the int value 40. The int value 40 is returned from the method and the = operator assigns it to the hoursWorked variable. Figure 3-13 illustrates this process. The following code sample demonstrates the double.Parse method. Assume that temperatureTextBox is the name of a TextBox control. 1 // Declare a double variable to hold the temperature. 2 double temperature; 3 4 // Get the temperature from the TextBox. 5 temperature = double.Parse(temperatureTextBox.Text); Line 5 passes temperatureTextBox.Text as an argument to the double.Parse method. That value is converted to a double, returned from the double.parse method, and assigned to the temperature variable. The following code sample demonstrates the decimal.Parse method. Assume that moneyTextBox is the name of a TextBox control. 3.5 inputting and Outputting numeric Values 143 Figure 3-13 Converting textBox input to an int The user enters 40 into the hoursWorkedTextBox control. The string "40" is stored in the control’s Text property. "40" 40 hoursWorked = int.Parse(hoursWorkedTextBox.Text); The int value 40 is returned from the int.Parse method and assigned to the hoursWorked variable. 1 // Declare a decimal variable to hold an amount of money. 2 decimal money; 3 4 // Get an amount from the TextBox. 5 money = decimal.Parse(moneyTextBox.Text); Line 5 passes moneyTextBox.Text as an argument to the decimal.Parse method. That value is converted to a decimal, returned from the decimal.parse method, and assigned to the money variable. NOTE: If you look at the top of a form’s source code in the code editor, you should see a directive that reads using System;. That directive is required for any program that uses the Parse methods. 144 Chapter 3 Processing Data Figure 3-14 Exception reported Figure 3-15 Exception reported Later in this chapter, you will learn how to catch errors like this and prevent the program from halting. Displaying Numeric Values Suppose an application has a decimal variable named grossPay and a Label control named grossPayLabel. You want to display the variable’s value in the Label control. To accomplish this, you must somehow get the value of the grossPay variable into the grossPayLabel control’s Text property. The following assignment statement will not work, however: grossPayLabel.Text = grossPay; d ERROR! You cannot assign a numeric value to a control’s Text property because only strings can be assigned to the Text property. If you want to display the value of a numeric variable in a Label control, you have to convert the variable’s value to a string. Luckily, all variables have a ToString method that you can call to convert the variable’s value to a string. You call the ToString method using the following general format: variableName.ToString() In the general format, variableName is the name of any variable. The expression returns the variable’s value as a string. Here is a code sample that demonstrates: decimal grossPay = 1550.0m; grossPayLabel.Text = grossPay.ToString(); 3.5 inputting and Outputting numeric Values 145 The first statement declares a decimal variable named grossPay initialized with the value 1,550.0. In the second statement, the expression on the right side of the = operator calls the grossPay variable’s ToString method. The method returns the string "1550.0". The = operator then assigns the string "1550.0" to the grossPayLabel control’s Text property. As a result, the value 1550.0 is displayed in the grossPayLabel control. This process is illustrated in Figure 3-16. Figure 3-16 Displaying numeric data in a Label control decimal grossPay = 1550.0m; grossPayLabel.Text = grossPay.ToString(); "1550.0" You must also convert a numeric variable to a string before passing it to the MessageBox.Show method. The following example shows how an int variable’s value can be converted to a string and displayed in a message box: int myNumber = 123; MessageBox.Show(myNumber.ToString()); The first statement declares an int variable named myNumber, initialized with the value 123. In the second statement the following takes place: The myNumber variable’s ToString method is called. The method returns the string "123". The string "123" is passed to the MessageBox.Show method. As a result, the value 123 is displayed in a message box. Implicit String Conversion with the + Operator In this chapter, you’ve learned that the + operator has two uses: string concatenation and numeric addition. If you write an expression using the + operator and both operands are strings, the + operator concatenates the strings. If both operands are numbers of compat- ible types, then the + operator adds the two numbers. But what happens if one operand is a string and the other operand is a number? The number will be implicitly converted to a string, and both operands will be concatenated. Here’s an example: int idNumber = 1044; string output = "Your ID number is " + idNumber; In the second statement, the string variable output is initialized with the string "Your ID number is 1044". Here is another example: double testScore = 88.5; MessageBox.Show("Your test score is " + testScore); The second statement displays a message box showing the string "Your test score is 88.5". In Tutorial 3-2, you will use some of the techniques discussed in this section. You will create an application that reads numeric input from TextBox controls, and displays numeric output in a Label control. 146 Chapter 3 Processing Data Tutorial 3-2: Calculating Fuel Economy In the United States, a car’s fuel economy is measured in miles per gallon, or MPG. You VideoNote use the following formula to calculate a car’s MPG: Tutorial 3-2: Calculating MPG = Miles driven ÷ Gallons of gas used Fuel Economy In this tutorial, you will create an application that lets the user enter the number of miles he or she has driven and the gallons of gas used. The application will calculate and display the car’s MPG. Figure 3-17 shows the application’s form, with the names of all the controls. When the application runs, the user enter the number of miles driven into the milesTextBox con- trol and the gallons of gas used into the gallonsTextBox control. When the user clicks the calculateButton control, the application calculates the car’s MPG and displays the result in the mpgLabel control. The exitButton control closes the application’s form. Figure 3-17 the Fuel Economy form milesPromptLabel milesTextBox gallonsPromptLabel gallonsTextBox outputDescriptionLabel mpgLabel calculateButton exitButton Step 1: Start Visual Studio and begin a new Windows Forms Application project named Fuel Economy. Step 2: Set up the application’s form as shown in Figure 3-17. Notice that the form’s Text property is set to Fuel Economy. The names of the controls are shown in the figure. As you place each of the controls on the form, refer to Table 3-8 for the relevant property settings. Step 3: Once you have set up the form with its controls, you can create the Click event handlers for the Button controls. At the end of this tutorial, Program 3-2 shows the completed code for the form. You will be instructed to refer to Program 3-2 as you write the event handlers. (Remember, the line numbers that are shown in Program 3-2 are not part of the program. They are shown for reference only.) In the Designer, double-click the calculateButton control. This opens the code editor, and you will see an empty event handler named calculateButton_ Click. Complete the calculateButton_Click event handler by typing the code shown in lines 22–38 in Program 3-2. Let’s take a closer look at the code: Line 22: This statement declares a double variable named miles. This vari- able is used to hold the number of miles driven. Line 23: This statement declares a double variable named gallons. This variable is used to hold the number of gallons used. 3.5 inputting and Outputting numeric Values 147 Table 3-8 Control property settings Control Name Control Type Property Settings milesPromptLabel Label Text: Enter the number of miles driven: gasPromptLabel Label Text: Enter the gallons of gas used: outputDescriptionLabel Label Text: Your car’s MPG: milesTextBox TextBox No properties changed gallonsTextBox TextBox No properties changed mpgLabel Label AutoSize: False BorderStyle: FixedSingle Text: (The contents of the Text property have been erased.) TextAlign: MiddleCenter calculateButton Button Text: Calculate MPG exitButton Button Text: Exit Line 24: This statement declares a double variable named mpg. This variable is used to hold the MPG, which will be calculated. Line 28: This statement converts the milesTextBox control’s Text property to a double and assigns the result to the miles variable. Line 32: This statement converts the gallonsTextBox control’s Text prop- erty to a double and assigns the result to the gallons variable. Line 35: This statement calculates MPG. It divides the miles variable by the gallons variable and assigns the result to the mpg variable. Line 38: This statement converts the mpg variable to a string and assigns the result to the mpgLabel control’s Text property. This causes the value of the mpg variable to be displayed in the mpgLabel control. Step 4: Switch your view back to the Designer and double-click the exitButton control. In the code editor you will see an empty event handler named exitButton_Click. Complete the exitButton_Click event handler by typing the code shown in lines 43– 44 in Program 3-2. Step 5: Save the project. Then, press the key on the keyboard or click the Start Debugging button ( ) on the toolbar to compile and run the application. Test the application by entering values into the TextBoxes and clicking the Calculate MPG button. The MPG should be displayed, similar to Figure 3-18. Click the Exit button and the form should close. Figure 3-18 the Fuel Economy application 148 Chapter 3 Processing Data Program 3-2 Completed Form1 code for the Fuel Economy application 1 using System; 2 using System.Collections.Generic; 3 using System.ComponentModel; 4 using System.Data; 5 using System.Drawing; 6 using System.Linq; 7 using System.Text; 8 using System.Threading.Tasks; 9 using System.Windows.Forms; 10 11 namespace Fuel_Economy 12 { 13 public partial class Form1 : Form 14 { 15 public Form1() 16 { 17 InitializeComponent(); 18 } 19 20 private void calculateButton_Click(object sender, EventArgs e) 21 { 22 double miles; // To hold miles driven 23 double gallons; // To hold gallons used 24 double mpg; // To hold MPG 25 26 // Get the miles driven and assign it to 27 // the miles variable. 28 miles = double.Parse(milesTextBox.Text); 29 30 // Get the gallons used and assign it to 31 // the gallons variable. 32 gallons = double.Parse(gallonsTextBox.Text); 33 34 // Calculate MPG. 35 mpg = miles / gallons; 36 37 // Display the MPG in the mpgLabel control. 38 mpgLabel.Text = mpg.ToString(); 39 } 40 41 private void exitButton_Click(object sender, EventArgs e) 42 { 43 // Close the form. 44 this.Close(); 45 } 46 } 47 } 3.6 Formatting numbers with the ToString Method 149 Checkpoint 3.25 What method converts the string literal "40" to a value of the int data type? 3.26 Write a statement that converts each of the following string values to the decimal data type using the decimal.Parse method. a. "9.05" b. grandTotal c. "50" d. priceTextBox.Text 3.27 Suppose an application has a decimal variable named total and a Label control named totalLabel. What will be the result when the following assignment statement is executed? totalLabel.Text = total; 3.28 Write a statement that displays each of the following numeric variables in a message box. a. grandTotal d. highScore c. sum b. width 3.29 Write a statement that will store the value of an int variable named result in the Text property of a Label control named resultLabel. 3.6 Formatting Numbers with the ToString Method CONCEPT: The ToString method can optionally format a number to appear in a specific way. When you display large numbers, you usually want to format them with commas so they are easy to read. For example, 487,634,789.0 is easier to read than 487634789.0. Also, when you display amounts of money, you usually want to round them to two decimal places and display a currency symbol, such as a dollar sign ($). When you call the ToString method, you can optionally pass a formatting string as an argument to the method. The formatting string indicates that you want the number to appear formatted in a specific way when it is returned as a string from the method. For example, when you pass the formatting string "c" to the ToString method, the number is returned formatted as currency. Assuming that you are in the United States, numbers for- matted as currency are preceded by a dollar sign ($), are rounded to two decimal places, and have comma separators inserted as necessary. The following code sample demonstrates: decimal amount = 123456789.45678m; MessageBox.Show(amount.ToString("c")); Notice in the second statement that the "c" formatting string is passed to the amount variable’s ToString method. The message box that the statement displays appears as shown in Figure 3-19. There are several other format strings that you can use with the ToString method, and each produces a different type of formatting. Table 3-9 shows a few of them. 150 Chapter 3 Processing Data Figure 3-19 a number formatted as currency Table 3-9 a few of the formatting strings Format String Description "N" or "n" Number format "F" or "f" Fixed-point scientific format "E" or "e" Exponential scientific format "C" or "c" Currency format "P" or "p" Percent format Number Format Number format ("n" or "N") displays numeric values with comma separators and a deci- mal point. By default, two digits display to the right of the decimal point. Negative values are displayed with a leading minus sign. An example is −2,345.67. Fixed-Point Format Fixed-point format ("f" or "F") displays numeric values with no thousands separator and a decimal point. By default, two digits display to the right of the decimal point. Negative values are displayed with a leading minus (−) sign. An example is −2345.67. Exponential Format Exponential format ("e" or "E") displays numeric values in scientific notation. The number is displayed with a single digit to the left of the decimal point. The letter e appears in front of the exponent, and the exponent has a leading + or + sign. By default, six digits display to the right of the decimal point, and a leading minus sign is used if the number is negative. An example is −2.345670e+003. Currency Format Currency format ("c" or "C") displays a leading currency symbol (such as $), digits, comma separators, and a decimal point. By default, two digits display to the right of the decimal point. Negative values are surrounded by parentheses. An example of a negative value is ($2,345.67). Using Percent Format Percent format ("p" or "P") causes the number to be multiplied by 100 and displayed with a trailing space and % sign. By default, two digits display to the right of the decimal point. Negative values are displayed with a leading minus sign. For example, the number 0.125 would be formatted as 12.5 % and the number −0.2345 would be formatted as −23.45 %. 3.6 Formatting numbers with the ToString Method 151 Specifying the Precision Each numeric format string can optionally be followed by an integer that indicates how many digits to display after the decimal point. For example, the format "n3" displays three digits after the decimal point. Table 3-10 shows a variety of numeric formatting examples, based on the North American locale. Table 3-10 numeric formatting examples (north american locale) Number Format String ToString( ) Return Value 12.3 "n3" 12.300 12.348 "n2" 12.35 1234567.1 "N" 1,234,567.10 123456.0 "f2" 123456.00 123456.0 "e3" 1.235e+005.234 "P" 23.40 % −1234567.8 "C" ($1,234,567.80) Rounding Rounding can occur when the number of digits you have specified after the decimal point in the format string is smaller than the precision of the numeric value. Suppose, for exam- ple, that the value 1.235 were displayed with a format string of "n2". Then the displayed value would be 1.24. If the next digit after the last displayed digit is 5 or higher, the last displayed digit is rounded away from zero. Table 3-11 shows examples of rounding using a format string of "n2". Table 3-11 rounding examples using the "n2" display format string Number Formatted As 1.234 1.23 1.235 1.24 1.238 1.24 −1.234 −1.23 −1.235 −1.24 −1.238 −1.24 Using Leading Zeros with Integer Values You can use the "d" or "D" formatting strings with integers to specify the minimum width for displaying the number. Leading zeros are inserted if necessary. Table 3-12 shows examples. Table 3-12 Formatting integers using the "d" or "D" formatting strings Integer Value Format String Formatted As 23 "d" 23 23 "d4" 0023 1 "d2" 01 152 Chapter 3 Processing Data In Tutorial 3-3, you will create an application that uses currency formatting to display a dollar amount. Tutorial 3-3: Creating the Sale Price Calculator application with Currency Formatting VideoNote Tutorial 3-3: If you are writing a program that works with a percentage, you have to make sure that the Creating the percentage’s decimal point is in the correct location before doing any math with the per- Sale Price centage. This is especially true when the user enters a percentage as input. Most users will Calculator Application enter the number 50 to mean 50 percent, 20 to mean 20 percent, and so forth. Before you with perform any calculations with such a percentage, you have to divide it by 100 to move its Currency decimal point to the left two places. Formatting Suppose a retail business is planning to have a storewide sale in which the prices of all items will be reduced by a specified percentage. In this tutorial, you will create an applica- tion to calculate the sale price of an item after the discount is subtracted. Here is the algo- rithm, expressed as pseudocode: 1. Get the original price of the item. 2. Get the discount percentage. (For example, 20 is entered for 20 percent.) 3. Divide the percentage amount by 100 to move the decimal point to the correct location. 4. Multiply the percentage by the original price. This is the amount of the discount. 5. Subtract the discount from the original price. This is the sale price. 6. Display the sale price.