03-ci.pdf
Document Details
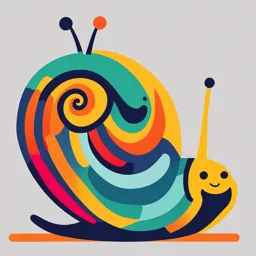
Uploaded by JubilantTellurium
Tags
Full Transcript
RW344: Software Engineering Bernd Fischer [email protected] Continuous Integration & Continuous Deployment Continuous Integration Continuous Integration (CI) is a development practice that requires developers to integrate code into a shared repository several times a day. Each...
RW344: Software Engineering Bernd Fischer [email protected] Continuous Integration & Continuous Deployment Continuous Integration Continuous Integration (CI) is a development practice that requires developers to integrate code into a shared repository several times a day. Each check-in is then verified by an automated build. By integrating regularly, we can detect errors quickly, and locate them more easily. See https://www.thoughtworks.com/continuous-integration Continuous Integration CI makes sense when working on a large project – One person’s changes might cause other’s code to fail Requires integration tests – Each developer still runs their unit tests themselves – Bugs can in theory only be in integration In reality even having only unit tests makes it worthwhile – Running on a different machine, with a different setup! – Might make small changes thinking it is fine…and it is not – Might have pushed an incomplete set of files Continuous deployment (CD) pushes this into the fielded product – doesn’t work for “shrinkwrapped software” but web and apps CI/CD in GitLab requires a runner GitLab Runner Something that can execute your pipeline Commonly using docker Specify the docker image and how to run When a commit comes in, the docker image is created and the code executed These runners can be pre-configured or you have to create your own What is Docker? container system lightweight virtual execution environment – lighter than virtual machines – platform as a service (PaaS) enables code portability: write once, run anywhere* configure your system precisely the way you want it – huge selection of base containers makes testing simple, since you know exactly what the environment is that you are testing on CI in GitLab Create a repo for your project on GitLab Add.gitlab-ci.yml file Configure a runner on GitLab – You need this to run the actual CI – settings; permissions;… ask demis Use shared runners to make life simpler – runners created so that anybody can use them – distinguished by tags must match what is in.gitlab-ci.yml default: kuber-nointernet-shared Sample.gitlab-ci.yml docker image to use as base pipeline image: maven:3-jdk-8 stage name test: stage: test script to run script: mvn test tags: [kuber-nointernet-shared] runner tag for CS GitLab shared runners Code Review key quality assurance step manual inspection of code for – logical errors – completeness of the code (no missed cases) – sufficient tests – code style adhered to (this can easily be automated) heavyweight (formal inspection following specific rules) or lightweight review after issue completion, but before merge into upstream code – no unreviewed code is pushed to main good way to mentor new developers and share expertise – form of peer pressure if you know someone will check your code GitHub support: https://github.com/features/code-review Code Review - Flow Sample A developer makes a change in their feature branch and tests it. When they’re happy they push, and make a merge request. The developer assigns the merge request to a reviewer, who looks at it and makes line and design level comments as appropriate. When the reviewer is finished, they assign it back to the author. The author addresses the comments. This stage can be repeated, but once both are happy, one assigns to a final reviewer who can merge. The final reviewer follows the same process again. The author again addresses any comments, either by changing the code or by responding with their own comments. Once the final reviewer is happy and the build is green, they will merge. See https://about.gitlab.com/2017/03/17/demo-mastering-code-review-with-gitlab/ Code Review via Pull Requests Pull Requests typically used for merging code from a fork into a repo, where you don’t have write access (GitHub) Pull Requests allow you to get feedback on your code Can also be used even when you don’t want to merge code – tag the people you want to comment on the code Build Systems Build systems are software tools designed to automate the process of program compilation. Compiling one file: javac Compiling a large system with many dependencies requires a more sophisticated build system – make (1976) – ant (2000) – Maven (2004) – Gradle (2012) – SBT for Scala and by extension Java Describing the build process becomes a program itself See https://technologyconversations.com/2014/06/18/build-tools/ Maven Provides a uniform build system – doesn’t eliminate the need to know about the underlying mechanisms, but it shields from the details – project object model (POM) and a set of plugins that are shared by all projects using Maven, providing a uniform build system If you have seen one Maven project you have seen all… Provides guidelines for best practices development – Specification, execution, and reporting of unit tests are part of the normal build cycle using Maven. – Keeping test source code in a separate, but parallel source tree – Using test case naming conventions to locate and execute tests – Having test cases setup their environment instead of relying on customizing the build for test preparation Maven POM Example za.sun.cs.rw344 stupid jar 1.0-SNAPSHOT stupid http://maven.apache.org junit junit 3.8.1 test Test-Driven Development Tests are Requirements, Requirements are Tests! 1. Add a test – each new feature begins with a test that passes iff the feature's specifications are met. 2. Run all tests – the new test should fail for expected reasons 3. Write the simplest code that passes the new test 4. All tests should now pass – if any fail, the new code must be revised 5. Refactor as needed, using tests after each refactor to ensure that functionality is preserved 6. Repeat Test-Driven Development – jUnit Example import static org.junit.Assert.*; import org.junit.Test; public class Simple { public class TestSimple { public static void main(String[] s) { @Test isEven(10); public void testSomethingEven() { } assertTrue(Simple.isEven(10)); } public static boolean isEven(int i) { @Test return true; public void testSomethingOdd() { } assertFalse(Simple.isEven(9)); } } } Test-Driven Development – jUnit Example import static org.junit.Assert.*; import org.junit.Test; public class Simple { public class TestSimple { public static void main(String[] s) { @Test isEven(10); public void testSomethingEven() { } assertTrue(Simple.isEven(10)); } public static boolean isEven(int i) { @Test return (i % 2 == 0); public void testSomethingOdd() { } assertFalse(Simple.isEven(9)); } } } Debugging This is an Art, but worth picking up Brute force and ignorance: – Take memory dumps, do run-time tracing, put print statements everywhere. – You will be swamped with data!! Backtracking: – Begin at the point where the symptom occurs, trace backwards step by step – Only feasible for small programs. Cause elimination: – Use deduction to list all possible causes. – Devise tests to eliminate them one by one. – Try to find the simplest input that shows the symptom. – Delta Debugging: remove code or versions to identify bug location Logging Fancy print statements! The sooner you learn the power of logging the better Logging is a form of abstraction – Gives a runtime view of what the program is doing Finds bugs faster than testing – Tells you what the bug is (see debugging from before) – Even if you don’t have the test to expose and issue one can see from the logs there is an issue Can form part of automated testing of long running code See http://vasir.net/blog/development/how-logging-made-me-a-better-developer Bug and Issue Tracking Bug Tracking systems allow programmers and testers to keep track of the bugs in the code: Bug reports are filed in a database (a good bug report should tell a developer how to reproduce the error for themselves) Bugs are always ranked according to severity – P1 (must fix) – P2 (not showstopper, schedule to fix) – P3 (nice to have, not scheduled to fix) – P4 (enhancement) Many tools, see http://www.aptest.com/bugtrack.html Commonly used: Bugzilla (free) and JIRA (commercial) Great essay on the topic: http://www.chiark.greenend.org.uk/~sgtatham/bugs.html Bug and Issue Tracking One can also use a bug tracking system to record outstanding features and track their progress (issue tracking). bugs and features treated uniformly tracking system becomes central management tool – widely used in agile processes Bug tracker and code repository should be cross-linked: each bug should be linked to the bug-inducing commit each bug-fixing commit should be linked to the issue → allows detailed code and process analysis – mining software repositories A Bug’s Life New version is committed to repository and tagged “v1” New build is created and QA (Quality Assurance) is notified to start testing v1 QA finds a bug and reports it in the Bug Tracking system, under tag “Bug666”. – Bug is assigned a severity, say P1, and a “developer” is assigned to fix it. – Bug666 is now OPEN. Developer is typically the engineering lead and not actually the developer that will fix the bug… … but during a triage of the bugs by the engineering lead, it gets assigned to the developer that is most likely to be able to fix it A Bug’s Life During triage (or preferably before filing the bug) it might be noticed that this bug is a duplicate of another bug and will be designated as DUPLICATE and then CLOSED If the bug report is good enough to reproduce the bug the developer will fix it and make a new check-in to the repository stating that it is to fix Bug666 and will record the bug tag in the check-in message, say this check-in is now “v2” If the bug report is not good enough, developer and QA will go back and forth (recorded in a thread attached to the bug report) QA will verify that the bug is fixed and if so will set the bug status to CLOSED, recording version v2 as the bug-fixing commit If it is not fixed the cycle repeats A Bug’s Life – Bugzilla standard bug life cycle